FUNDAMENTALS A Complete Guide for Beginners
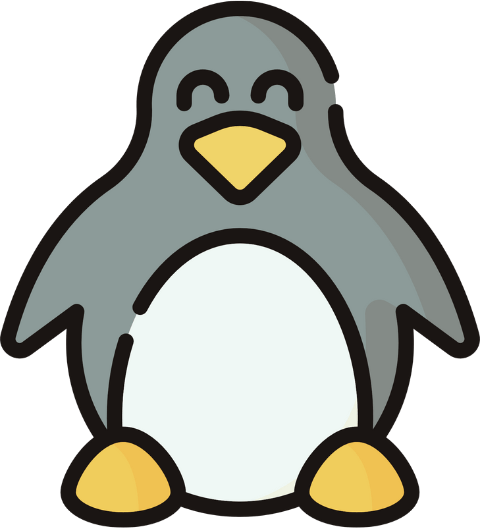
The double square brackets [[ ]] within an ‘if’ statement in Bash introduce extended capabilities for performing conditional tests compared to single square brackets [ ]. You can employ double square brackets [[ ]] in various schemes in Bash such as string comparisons, numeric comparisons, file comparisons, regex matching, validating compound conditionals and pattern matching etc.
In this article, I will demonstrate 6 different examples of using double square brackets [[ ]] within an ‘if’ condition in Bash.
What Are Double Square Brackets “[[ ]]” in Bash?
The double square brackets [[ ]] are treated as an extended command in Bash which is a convenient alternative to single square brackets [ ]. This double brackets [[ ]] structure was first introduced in Korn SHell (ksh).
However, it is now used in Bash for more complex and versatile conditional tests within ‘if’ statements. These brackets offer improved functionalities in terms of pattern matching, parameter expansion, error handling etc. Using [[ ]] structure helps in preventing word splitting in Bash scripts.
The syntax for using double brackets [[ ]] in ‘if’ statements is as follows:
if [[ condition ]]; then
#Code to execute if the condition is true
fi
6 Examples of Using Double Square Brackets “[[ ]]” With “if” Condition in Bash
Double square brackets [[ ]] in Bash provide distinct conditional tests and evaluations with additional features. Following are some examples stating how double square brackets [[ ]] can be implemented within the ‘if’ conditional statement in Bash:
Example 1: Numeric Comparison Test Using Double Brackets
The double square brackets [[ ]] offer a direct way to perform numeric comparison tests in Bash by using several numeric operators such as -eq (equal), -ne (not equal), -lt (less than), -le (less than or equal), -gt (greater than) and -ge (greater than or equal). You can use the syntax if [[ number1 OPERATOR number2 ]];
to compare two numeric values with the [[ ]] construct. Let’s see an example:
#!/bin/bash
#Defining two variables
number1=5
number2=15
#Checking if a number is less than another one
if [[ $number1 -lt $number2 ]]; then
echo "$number1 is less than $number2"
fi
In the script, if [[ $num1 -lt $num2 ]];
checks if the variable number1 is less than the variable number2. If it satisfies the condition, the script executes a true expression.
Example 2: String Comparison Test Using Double Brackets in If Statement
The double square brackets [[ ]] in Bash ‘if’ statement can be used to compare strings with various string comparison operators such as = (equal), != (not equal), > (greater than), < (less than), >= (greater than or equal) and <= (less than or equal). To accomplish these conditional tasks, use the syntax if [[ string1 OPERATOR string2 ]];
. Let’s see how it works:
#!/bin/bash
#Defining two variables
str1="Hello"
str2="Linux"
#Checking if a string is not equal to another one
if [[ $str1 != $str2 ]]; then
echo "Strings are not equal"
fi
Here, if [[ $str1 != $str2 ]];
validates whether the two strings str1 and str2 are equal or not. If it returns true, the script prints ‘Strings are not equal’.
Example 3: Checking File Existence Using Double Brackets in Bash
Multiple file and directory test operations can be done using the double square brackets [[ ]] in Bash ‘if’ conditional statements. To accomplish these tasks, you can use the file test operators within conditional statements in Bash. Here’s an example demonstrating how to check file existence by employing if [[ OPERATOR file_name ]];
syntax:
#!/bin/bash
#Defining a variable
file="tag.sh"
#Checking if the file exists
if [[ -e $file ]]; then
echo "The file exists."
fi
Here, if [[ -e $file ]];
checks if the assigned file tag.sh exists or not. If the file exists, the script executes a true expression and displays ‘The file exists.’.
The above image states that the file I have assigned in the script exists in my system.
Example 4: Matching Regular Expression (Regex) in Bash Using Double Brackets
Regular expression (Regex) inside double square brackets [[ ]] in Bash provides flexible pattern matching based on certain criteria. In this case, you can use the =~
operator along with double square brackets [[ ]] to perform regular expression matching. Take a look at the following example:
#!/bin/bash
#Defining a variable
email_id="[email protected]"
#Checking if the email address matches the pattern
if [[ $email_id =~ ^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}$ ]]; then
echo "A valid email."
fi
Here, the ‘if’ conditional statement checks if the variable email_id
matches the specified regular expression pattern for a verified email address format. If the email address matches the pattern, it executes a true expression and prints ‘A valid email.’.
You can see from the image that the assigned email_id validates the basic format of an email address successfully.
Example 5: Verifying Compound Conditions With Logical Operators
The logical operators &&
(AND) and ||
(OR) and multiple conditions can be utilized together within double square brackets [[ ]] to perform more complex conditional evaluations. Let’s see an example:
#!/bin/bash
#Defining a variable
number=20
#Checking compound conditions with logical operators
if [[ ($number -lt 25 && $number != 30) || $number -gt 40 ]]; then
echo "Satisfies the compound conditions."
fi
In this script, the compound if statement validates whether the value of the number is less than 25 and not equal to 30 or greater than 40. If all the conditions are met, it returns a true expression.
This image indicates compound conditional validation using logical operators within [[ ]].
&&
and ||
with the test operators -a
(logical AND) and -o
(logical OR), you must enclose them within single square brackets [ ]
.Example 6: Performing Globbing for Pattern Matching in Bash
In Bash scripting, the term globbing refers to the process of using wildcard characters for matching and manipulating filenames or paths effectively based on patterns. Some widely used wildcard characters for globbing in Bash are *
(Asterisk), ?
(Question Mark) and [ ]
(Square Brackets) etc.
You can match filenames with a specific prefix, extension, list files, check specific substring etc by performing globbing within double square brackets [[ ]] in Bash. Following is an example demonstrating how globbing works within [[ ]]:
#!/bin/bash
#Defining a variable
prefix_part="hash"
for file in *; do
if [[ $file == "$prefix_part"* ]]; then
echo "Valid file $file with '$prefix_part prefix."
fi
done
The script searches all files in the current working directory and verifies all the filenames (using globbing pattern *) starting with the given prefix. Every time a file matches the condition, it returns a true expression.
In this image, you can see that all the files in my current working directory have been identified that start with a prefix hash.
Difference Between Single and Double Square Brackets in Bash
In Bash scripting, both single square brackets [ ] and double square brackets [[ ]] are used to perform test operations in different conditional statements and loops. However, there are some possible differences between them in terms of functional features. Following is a tabular representation of the key differences between single and double square brackets in Bash:
Functions |
Single Square Brackets [ ] |
Double Square Brackets [[ ]] |
---|---|---|
Basic Feature |
‘[ ]’ is a built-in command in Bash. |
‘[[ ]]’ is an extended Bash command that is the enhanced version of ‘[ ]’. |
Logical Operators |
Supports not only && and || logical operators but also -a and -o operators. |
Supports only && and || logical operators. |
Regex Matching |
Does not support regex matching. |
Uses =~ operator for regex matching. |
Quoting during String Handling |
Requires explicit quoting. |
No explicit quoting. |
Parentheses ‘( )’ for Grouping Expressions |
Use an escape character before ‘(‘ and ‘)’ and a space after ‘(‘ and ‘)’. |
Use the parentheses directly without any escape character. |
Pattern Matching |
Supports limited pattern matching. |
Supports more versatile pattern matching. |
Supported Bash Versions |
Compatible for all versions. |
Does not work in older versions. |
Word Splitting |
Supports word splitting for unquoted variables. |
Does not support word splitting. |
Conclusion
To sum up, using double square brackets [[ ]] within ‘if’ statements in Bash enables users to evaluate more advanced conditional tests and create more versatile Bash scripts.
People Also Ask
Why double square brackets don’t work in if statement with grep?
Double square brackets don’t directly work with the ‘grep’ command in an ‘if’ statement because it is an external command in Bash. However, you can use the ‘grep’ command in the ‘if’ statement by using the test command or equivalent test operator ‘[ ]’. For example:
#!/bin/bash
string="Hello, Linux!"
if [ "$(echo "$string" | grep "Linux")" ]; then
echo "'Linux' exists in the string."
fi
Are double square brackets [[ ]] preferable over single square brackets [ ] in Bash?
In Bash, double square brackets ‘[[ ]]’ are preferable to use in some cases over single square brackets ‘[ ]’ due to their improved functionality, ease of use, code readability, and better error handling features.
Can I use double brackets in any version of Bash?
You can use double square brackets [[ ]] in most recent versions of Bash. However, note that ‘[[ ]]’ is not supported in older or limited versions of Bash.
Are double brackets necessary for simple comparisons?
No, double brackets are not necessary to use for simple comparisons in Bash, rather you can use single square brackets [ ] or the ‘test’ command.
Can I nest double brackets within ‘if’ statements?
Yes, you can nest double brackets within ‘if’ statements to evaluate more complex conditional expressions in Bash. For instance:
#!/bin/bash
#Defining a variable
number=30
if [[ $number -lt 50 ]]; then
if [[ $number -gt 10 ]]; then
echo "The number $number is between 10 and 50."
fi
fi
Can I use double brackets for checking if a string contains a specific substring?
Yes, you can use double brackets to check if a string contains a specific substring using the syntax [[ $string == *substring* ]]
. For example:
#!/bin/bash
string="Hello, welcome to Linuxsimply!"
if [[ $string == *"welcome to"* ]]; then
echo "The string contains 'welcome to'."
fi
Related Articles
- Mastering 10 Essential Options of If Statement in Bash
- How to Check a Boolean If True or False in Bash [Easy Guide]
- Bash Test Operations in ‘If’ Statement
- Check If a Variable is Empty/Null or Not in Bash [5 Methods]
- Check If a Variable is Set or Not in Bash [4 Methods]
- Check If Environment Variable Exists in Bash [6 Methods]
- Bash Modulo Operations in “If” Statement [4 Examples]
- How to Use “OR”, “AND”, “NOT” in Bash If Statement [7 Examples]
- Evaluate Multiple Conditions in Bash “If” Statement [2 Ways]
- 6 Ways to Check If a File Exists or Not in Bash
- How to Check If a File is Empty in Bash [6 Methods]
- 7 Ways to Check If Directory Exists or Not in Bash
- Negate an “If” Condition in Bash [4 Examples]
- Check If Bash Command Fail or Succeed [Exit If Fail]
- How to Write If Statement in One Line? [2 Easy Ways]
- Different Loops with If Statements in Bash [5 Examples]
- How to Use Flags in Bash If Condition? [With Example]
- Learn to Compare Dates in Bash [4 Examples]
<< Go Back to If Statement in Bash | Bash Conditional Statements | Bash Scripting Tutorial