FUNDAMENTALS A Complete Guide for Beginners
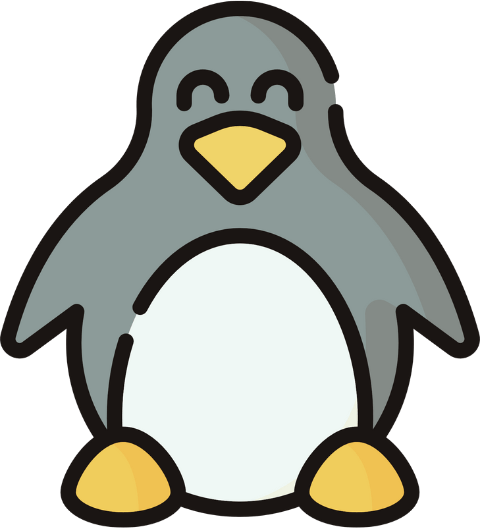
Checking a file’s existence in Bash refers to verifying if the specified file is present in the defined path or not. So, whenever working with files, it’s recommended to check the file’s existence before accessing or manipulating it. With the help of file test operators within different conditional test expressions, you can easily check the existence of a file in Bash scripting.
In this article, I will show you 6 ways to check if a file exists in Bash.
File Test Operators in Bash
The file test operators are used to determine various attributes and properties of files in Bash scripting. To check whether a file exists in Bash, you can generally use the file test operator “-e” with any conditional test syntax. Moreover, you can use the “-f” operator with an ‘if’ conditional statement to check if a file exists and if it is a regular file, excluding directories.
6 Ways to Check If a File Exists in Bash
While working with files, you can not only check the existence of a single file but also verify multiple files’ existence. You can do this by using “-e” or “-f” option with the help of the “test” command, “[ ]” and “[[ ]]” constructs. You can also check using the “stat” command, loop iteration, and logical operators along the ‘if’ statement.
1. Using “test” Command With “-e” Operator
The “test” command is a built-in utility in Bash that is used to evaluate different conditional expressions where if the condition is satisfied, it returns an exit status of zero (0) but if the condition is not met, it returns a non-zero exit status.
You can use the “test” command along with the file test operator “-e” within an ‘if’ conditional as the most straightforward way to check if a file exists in Bash. Here’s an example demonstrating the checking of a file’s existence regardless of the type:
#!/bin/bash
file_name="/home/nadiba/tag.sh" #Providing information in /path/to/file_name
#Checking if a file exists
if test -e "$file_name"; then
echo "'tag.sh' file exists."
fi
In this script, the “test” command within the ‘if’ conditional checks whether the specified file in the $file_name variable exists. If the file exists in the location, it returns a zero exit status and the script executes a true expression.
In the above image, you can see that the file tag.sh exists in my ‘home’ directory.
if ! test OPERATOR FILE_NAME;
syntax.2. Using Single Square Brackets “[ ]” With “-f” Operator
Another built-in utility, the single square brackets [ ] is treated as the equivalent syntax of the “test” command. So, you can use this “[ ]” construct as well to check a file’s existence in any POSIX-compliant shell. Here’s an example to show you how to use single square brackets to evaluate the existence of a file:
#!/bin/bash
echo -n "Enter a filename: "
read file
#Checking if the file exists
if [ -f "$file" ]; then
echo "The file exists."
fi
Here, if [ -f "$file" ];
checks if the file entered by the user exists in the system and if it is a regular file. If the file exists and is a regular file, the script returns a true expression and displays ‘The file exists.’.
if ! [ OPERATOR FILE_NAME ];
syntax, you can also check if a file does not exist.3. Using Double Square Brackets “[[ ]]”
Double square brackets [[ ]] are addressed as a shell keyword in Bash that serves similarly to the previous two syntaxes. But it is not a part of the POSIX standard and is more powerful and robust than the single square brackets.
However, you can use double square brackets to verify the existence of a file in a specified file path in Bash scripting. Here’s how:
#!/bin/bash
file="/home/nadiba/boolean.txt" #Providing information in /path/to/file_name
#Checking if a file exists
if [[ -e "$file" ]]; then
echo "'boolean.txt' file exists."
fi
Here, if [[ -e "$file" ]];
checks whether the mentioned file exists within the given location. If the file is found in the path, the script displays ”boolean.txt’ file exists.’.
You can see from the image that the file boolean.txt exists in my ‘home’ directory.
4. Using “stat” Command in Bash
The stat command in Bash is a very useful tool that provides a lot of information about files and directories such as file permissions, size, timestamps, system information etc. In addition, you can use this utility with conditional logic to test for file existence indirectly and gather additional information about that file. Here’s an example:
#!/bin/bash
#Checking if a file exists using ‘stat’ command
if stat "/home/nadiba/Desktop/dlt.sh" &> /dev/null; then #Providing information in /path/to/file_name
echo "The file 'dlt.sh' exists."
fi
Here, the ‘if’ statement using the ‘stat’ command gathers information about the file specified by the file path. If the file exists and is accessible, the script returns an exit status of zero and prints a message indicating that the file exists.
In this image, you can see that the file dlt.sh exists in the ‘Desktop’ directory of my system and it is accessible.
5. Using “for” Loop to Check Multiple Files’ Existence
In Bash scripting, sometimes you may need to check the existence of multiple files. To do so, you can use a for loop along with the ‘if’ conditional statement to iterate through a list of file paths and perform conditional checks for each iteration to verify the presence of specified files you need. For instance:
#!/bin/bash
#Defining file paths with an array
filepaths=(
#Providing information in /path/to/file_name
"/home/nadiba/assign.sh"
"/home/nadiba/ascii.sh"
"/home/nadiba/backslash.sh"
)
#Looping through each file path to check the existence
for file in "${filepaths[@]}"; do
if [ -f "$file" ]; then
echo "File '$file' exists."
fi
done
Here, the for loop iterates through each file in the filepaths array and inside the loop, the ‘if’ conditional statement evaluates whether the current file specified by $file exists and is a regular file. If the file exists, the script executes a true expression by printing each output message for each file.
From the image, you can see that all the mentioned files ‘assign.sh’, ‘ascii.sh’ and ‘backslash.sh’ exist in the ‘home’ directory of my system.
6. Using Logical Operators to Check Multiple Files’ Existence
Using logical operators for checking multiple files’ existence is not a straightforward method. However, you can use && ( logical AND) and || (logical OR) with conditional expressions to evaluate the existence of multiple files where the script performs different actions based on different combined criteria as follows:
#!/bin/bash
#Defining file paths
#Providing information in /path/to/file_name
filepath1="/home/nadiba/file1.sh"
filepath2="/home/nadiba/file2.sh"
filepath3="/home/nadiba/file3.sh"
#Checking if all files exist using && (logical AND) operator
if [ -e "$filepath1" ] && [ -e "$filepath2" ] && [ -e "$filepath3" ]; then
echo "Great! all files exist."
fi
In this script, the ‘if’ statement checks if all three files are present in the defined path using the && operator. If all the conditions are satisfied, the script prints ‘Great! all files exist.’.
From the image, you can see that all the files ‘file1.sh’, ‘file2.sh’ and ‘file3.sh’ are present in my ‘home’ directory.
Common Mistakes During File Existence Check
In Bash, it is very simple to check a file’s existence. However, some common mistakes lead to confusing errors during the check. These are:
-
Using a wrong file test operator for file existence check.
if test -d "/path/to/my_file.sh"; then
#Code to execute
fi
-
Forgetting to quote the file path while having spaces between them.
if test -f /path/to/my file.sh; then
#Code to execute
fi
-
Forgetting to put spaces between the “[ ]” construct.
if [-e "$my_file"]; then
#Code to execute
fi
Conclusion
Now that you know how to check the existence of a file in bash scripting, you can choose which method you want to use. However, the most important thing to remember is that you should check if the file you are searching for actually exists in your system before trying to access and use it. Otherwise, you may run into errors.
People Also Ask
What’s the difference between using test -e file_path and [[ -e file_path ]] to check file existence?
While checking file existence, test -e file_path
and [[ -e file_path ]]
perform similar conditional tests. So, basically, there is no operational difference between these two syntaxes. However, the [[ -e file_path ]] syntax is more flexible than the syntax test -e, providing extended features and easier string manipulation.
Can I check for the absence of a file in Bash scripting?
Yes, you can check for the absence of a file in Bash scripting by using a negation (!) operator along with the file existence check. For example:
#!/bin/bash
file_path="/path/to/filename.txt"
#Checking if a file does not exist
if [[ ! -e "$file_path" ]]; then
echo "The file does not exist."
fi
How can I create a file only if it doesn’t exist in Bash?
You can create a file only if it doesn’t exist in Bash by using the touch command. For example:
#!/bin/bash
file_path="/path/to/filename.txt"
#Checking if a file exists
if [ ! -e "$file_path" ]; then
touch "$file_path" #Creating a file
echo "A file is created."
fi
Can I check for file existence in a directory and its subdirectories?
Yes, you can check for file existence in a directory and its subdirectories by using the ‘find’ command that performs recursive searching through directories. For example:
#!/bin/bash
directory_path="/path/to/directory_name"
find_file="file_name.txt"
if [ -d "$directory_path" ]; then
if find "$directory_path" -type f -name "$find_file" | grep -q "$find_file"; then
echo "'$find_file' exists in the directory '$directory_path' or its subdirectories."
else
echo "'$find_file' does not exist in the directory '$directory_path' or its subdirectories."
fi
else
echo "Directory '$directory_path' does not exist."
fi
What happens if I try to access a file that doesn’t exist in Bash?
If you try to access a file that doesn’t exist in Bash, the operations may fail and lead to unexpected errors in your scripts.
How to check if a file exists in Bash in one line?
To check if a file exists in Bash in one line, you can use the ‘test’ command or the equivalent test single square bracket [ ] syntax. For example:
#!/bin/bash
test -e "/path/to/filename.txt" && echo "The file exists." || echo "The file does not exist."
Related Articles
- Mastering 10 Essential Options of If Statement in Bash
- How to Check a Boolean If True or False in Bash [Easy Guide]
- Bash Test Operations in ‘If’ Statement
- Check If a Variable is Empty/Null or Not in Bash [5 Methods]
- Check If a Variable is Set or Not in Bash [4 Methods]
- Check If Environment Variable Exists in Bash [6 Methods]
- Bash Modulo Operations in “If” Statement [4 Examples]
- How to Use “OR”, “AND”, “NOT” in Bash If Statement [7 Examples]
- Evaluate Multiple Conditions in Bash “If” Statement [2 Ways]
- Using Double Square Brackets “[[ ]]” in If Statement in Bash
- How to Check If a File is Empty in Bash [6 Methods]
- 7 Ways to Check If Directory Exists or Not in Bash
- Negate an “If” Condition in Bash [4 Examples]
- Check If Bash Command Fail or Succeed [Exit If Fail]
- How to Write If Statement in One Line? [2 Easy Ways]
- Different Loops with If Statements in Bash [5 Examples]
- How to Use Flags in Bash If Condition? [With Example]
- Learn to Compare Dates in Bash [4 Examples]
<< Go Back to If Statement in Bash | Bash Conditional Statements | Bash Scripting Tutorial