FUNDAMENTALS A Complete Guide for Beginners
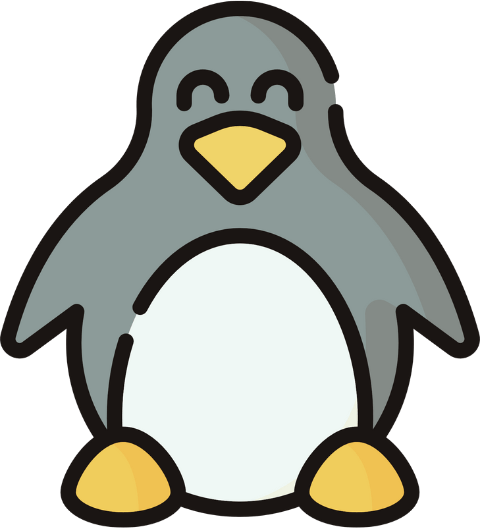
The Bash date command is multi-functional, offering various options and formats to display date and time. With this command, you can generate a timestamp that’s useful for logging and other purposes. This article demonstrates how to obtain a timestamp in Bash using the date command.
Key Takeaways
- Learning about the date command.
- Creating timestamp using the date command.
- Use of timestamp for logging purposes.
Free Downloads
2 Cases for Getting Timestamp in Bash
The date command usually shows both the date and time. But if you want time only in your timestamp, you have to use format specifiers that tell it how you want the time to appear. Take a look at the below scripts to see how you can create a customized timestamp with just the time part.
Case 1: Get Timestamp in Local Time Using Bash Script
Using the format specifier %T you can exact the time part of the date command. This will print time in %H:%M:%S format. However, you can also use %H:%M:%S format specifiers to get the same result.
❶ At first, launch an Ubuntu Terminal.
❷ Write the following command to open a file named localtime.sh in the build-in nano editor:
nano localtime.sh
- nano: Opens a file in the Nano text editor.
- localtime.sh: Name of the file.
Script (localtime.sh) >
#!/bin/bash
timestamp=$(date +%T)
echo $timestamp
In the script, the %T format specifier is used with the date command, which retrieves the current local time in the hh:mm:ss format. This local time is then stored in the timestamp variable. The echo command is used to display the variable, which effectively prints the current time to the terminal when the script is run.
❹ Use the following two commands to make both file executable:
chmod u+x localtime.sh
- chmod: Changes permissions.
- u+x: Giving the owner executing permission.
- localtime.sh: Name of the script.
./localtime.sh
As you can see the timestamp variable contains the local time which can be used as a timestamp.
Case 2: Getting Timestamp in UTC time Using Bash
To get the timestamp in UTC time you can use the -u option of date command. Here is how you can achieve it.
Script (utctime.sh) >
#!/bin/bash
timestamp=$(date -u +%T)
echo $timestamp
In the script, the -u flag is used along with the %T format specifier within the date command. This combination gives the current time in UTC and formats it as hh:mm:ss. The obtained UTC time is then stored in the timestamp variable. Finally, the echo command is used to display the value stored in the timestamp variable.
Run the utctime.sh script by the following command:
./utctime.sh
Here -u option successfully generates UTC time as shown in the image above. This can be used as a timestamp where necessary.
How to Get Timestamp in UNIX Epoch
A Unix timestamp is represented as seconds since January 1, 1970. To get a timestamp in Unix time epoch use %s option of date command.
Script (unixtime.sh) >
#!/bin/bash
timestamp=$(date +%s)
echo $timestamp
The +%s option captures the current time in Unix timestamp, which represents the number of seconds elapsed since January 1, 1970, UTC. The obtained timestamp is stored in the timestamp variable. Later the echo command is used to display it.
Run the unixtime.sh script by the following command:
./unixtime.sh
Here you can see a timestamp that basically counts time in seconds since January 1, 1970.
Effective Use of Timestamp in Bash
Let’s say you want a timestamp for logging purpose or keeping track of system updates. In the following demonstration I am going to write a similar script that can updates system in each 100 seconds epoch and add a timestamp in a log file for each update.
Script (timestamp.sh) >
#!/bin/bash
log_file="update.log"
# Check if the log file exists, if not, create it
if [ ! -e "$log_file" ]; then
touch "$log_file"
fi
while true; do
timestamp=$(date +%s.)
sudo apt update
echo "System updated at: $timestamp" >> "$log_file"
sleep 100
done
This Bash script initializes a variable named log_file with the value update.log. It checks whether the specified log file exists; if not, it creates the file using the touch command. The script then enters an infinite loop while loop. Within each iteration of the loop, it captures the current Unix timestamp in seconds using the date command.
Then it proceeds to update the system’s package information using sudo apt update. After the update, it appends the timestamp to the log file, indicating that the system was updated in the corresponding timestamp. The loop pauses for 100 seconds using the sleep command before starting the next iteration.
Run the timestamp.sh script by the following command:
./timestamp.sh
Once you run the code it will update the system in each 100s and add update notice with Unix timestamp in the update.log file.
Let’s see the timestamp of each update in update.log file.
As you can see update.log contains two timestamps in it. Last one indicates the time when the system is lastly updated using timestamp.sh script.
Conclusion
In summary, getting a timestamp in Bash is straightforward using the date command. Utilizing timestamps efficiently simplifies tasks for system administrators, enabling easy tracking of modification times and investigations into recent changes. After reading this article hope you can easily use timestamps in Bash.
People Also Ask
Related Articles
- How to Get Date in Bash [2 Methods with Examples]
- How to Print Time in Bash [2 Quick Methods]
- How to List Users in Bash [2 Easy Ways]
- How to Get Current Time in Bash [4 Practical Cases]
- How to Use Date Format in Bash [5 Examples]
- How to Copy and Paste in Bash [2 Methods & Cases]
- How to Read Password in Bash [3 Practical Cases]
- How to Send Email in Bash [2 Easy Methods]
- Bash Script to Send Email with Attachment [Step-by-Step Guide]
- How to Get IP Address in Bash [3 Methods]
- How to Find and Replace String in Bash [5 Methods]
- How to Get Script Name Using Bash Script? [3 Easy Ways]
- How to Call Another Script in Bash [2 Methods]
- How to Generate UUID in Bash [3 Simple Methods]
- 3 Easy Ways to Write to a File in Bash Script
- How to Write the Output to a File in Bash Script [5 Practical Cases]
- How to Create a List in Bash Scripts? [2 Easy Methods]
- How to Clear History in Bash [2 Practical Cases]
- How to Clear Screen Using Bash Script? [2 Effective Methods]
- How to Check Ubuntu Version Using Bash Scripts? [2 Methods]
<< Go Back to Bash Script Examples | Bash Scripting Basics | Bash Scripting Tutorial