FUNDAMENTALS A Complete Guide for Beginners
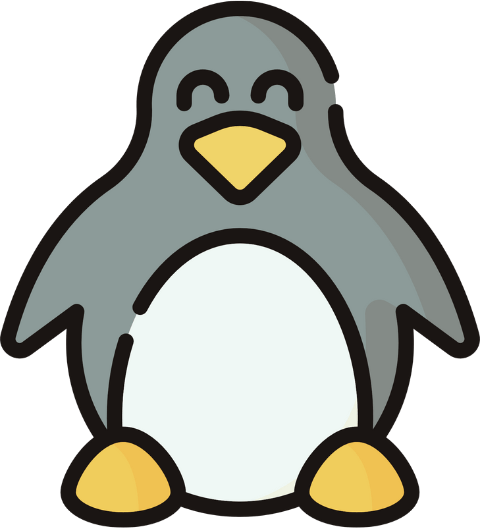
Find and replace is a fundamental task that is needed for changing things in files. Fortunately, Bash offers multiple alternative ways to find and replace. Some people like using the built-in nano text editor. Others prefer to directly replace text using Bash commands like sed. No matter what you like, you’ll find the answers to queries regarding replacing the content of a file in this reading.
Key Takeaways
- Find and replace in nano editor.
- Find and replace using the sed command.
- Find and replace using the awk command.
- Find and replace text across multiple files at a time.
Free Downloads
Find and Replace Text in the Nano Editor
Suppose you have a file and you want to replace certain text or words with new text from the file in the basic nano editor. First open the file in nano editor to successfully find and replace text. The syntax is:
nano filename
After opening the file you can replace any text using CTRL+\. In my file, I want to replace the word “hidden” with “protected” if there is any.
After selecting, a bottom menu appears as follows. Type the word or text you want to replace and press ENTER. I searched for the word “hidden” to replace it. This will automatically perform a case-insensitive search of the given text.
After pressing the ENTER key the bottom menu will ask you to put the new text. I inserted “protected” to replace the word “hidden” and pressed ENTER.Just after pressing the ENTER, another menu appears at the bottom and asks you whether you want to replace the first instance only. You can replace all instances of the given text by pressing A. In my case, I press A to replace all instances of the word “hidden”.
Once you press A for All or Y for Yes, this will instantly replace the matched text with new text. As I pressed A, I got a pop-up message stating that in 4 occurrences the word “hidden” is replaced with “protected”.
Find and Replace Text in File Using the “sed” Command in Bash
The sed command is used to find and replace certain strings in a file. The -i option of the sed command is used to replace a string in place where it matches the search. The basic syntax of the sed command to find and replace string is sed -i s"/old_string/new_string/" filename
. Look at the script below to see how it works:
#!/bin/bash
# Specify the path to the config file
config_file="/etc/ssmtp/ssmtp.conf"
# Ask user for the new mailhub value
read -p "Enter the new mailhub value: " new_mailhub
# Check if the config file exists
if [ -f "$config_file" ]; then
# Perform find and replace using sed
sed -i "s/^mailhub=.*/mailhub=$new_mailhub/" "$config_file"
echo "Replacement done in $config_file"
else
echo "$config_file does not exist."
fi
This Bash script allows users to modify the “mailhub” value in a configuration file located at “/etc/ssmtp/ssmtp.conf“. The script checks if the configuration file exists and, if so, uses the sed command to locate lines starting with “mailhub=” and replaces the value portion with the user-provided new value. The -i option enables in-place editing. Upon successful replacement, the script confirms the change with a message using the echo command.
See the content of the configuration file using the command below:
sudo cat /etc/ssmtp/ssmtp.conf
Now, run the script to see changes:
The program displays a message that it successfully changed the mailhub value to Email which was previously mail.
Let’s see the configuration file again. As you can see the value of mailhub has been changed to Email.
/g
at the end of the search. It means you want to replace the text globally. The syntax of the command will be as follows:
sed -i 's/old_text/new_text/g' filename
Find and Replace Text Using the “awk” Command in Bash
One can use the awk command to replace the text of a file. But this command can not perform in-place replacement. The basic syntax of the find-and-replace operation of the awk command is awk '{sub(/old_text/,new_text); print}' filename
. Look at the following example:
#!/bin/bash
read -p "New Email password:\n" -s new_pass
awk -v new_pass="$new_pass" '/^Email : / {sub($3, new_pass)} 1' password.txt
mv temp.txt password.txt
cat password.txt
This Bash script prompts the user to store a new email password in a secure manner using the -s flag with the read command. It then utilizes the awk command to search for lines starting with “Email : ” in a file named password.txt.
When such a line is found, it substitutes the third field (the existing password) with the newly entered password using the sub function. The modified content is then printed and redirected to a temporary file named temp.txt.
Afterward, the original password.txt file is replaced with the contents of temp.txt, effectively updating the password.
First, look at the content of the password file.
cat password.txt
These are the passwords stored in the password.txt file. Now, run the script to replace the current Email password and store a new one.
Once the program is executed it replaces the Email password with the newly inputted one which is 1445djshsy in this case.
This way one can replace the first occurrence of old text only. To replace all the occurrences use gsub instead of the sub function within the awk command.
Find and Replace Text in File With the “perl” Command
The perl command in Bash is old but extremely powerful. One can find and replace text in a single line of code using the perl command. See the codes below:
#!/bin/bash
perl -pi -e 's/hidden/protected/g' find_replace.txt
This Bash script utilizes the perl command to perform an in-place search and replace operation on the contents of the file named find_replace.txt. The script employs a regular expression-based substitution using the -pi flag, which stands for in-place and loops over files. It replaces all occurrences of the word “hidden” with “protected” in the find_replace.txt file.
First, let’s look at the find_replace.txt file.
Cat find_replace.txt
As you can see the content of the find_replace.txt file has the word “hidden” multiple times.
Now, run the above script:Once executed the program replaces the word “hidden” in all occurrences with the word “protected”.
Find and Replace Across Multiple Files Using the “find” and “sed” Commands
To find and replace text across multiple files use the find and sed commands. In such a case, the find command with exec is efficient to replace text from a couple of files at a time:
#!/bin/bash
for i in {1..10}; do
touch "dspace$i"
echo "It is a bash script file" > "dspace$i"
done
find . -type f -name "dspace*" -exec sed -i 's/It is bash script file/It is a text file with diskspace information/g' {} \;
The provided Bash script creates ten files named “dspace1” through “dspace10” using a loop. It then adds the text “It is a bash script file” into each of these files. After that, the script uses the find command to locate all files in the current directory with names starting with “dspace“.
For each of these files, the sed command is executed with the -i flag for in-place editing.
This command replaces the text “It is bash script file” with “It is a text file with diskspace information” in each of the located files, effectively modifying their contents.
Conclusion
To conclude, you can find and replace certain things in a file in various ways. The methods of this article cover almost all the default choices to find and replace text in Bash. Hope you enjoy reading this article.
People Also Ask
How do I replace a character in Bash?
To replace a character use a regular expression to match the single character. After that use any of the ways of replacing text.
How to find and replace in a shell script?
Shell Scripts are nothing but a file with the ‘.sh’ extension. You can find and replace text or string of a Bash script using any of the ways discussed in this article.
How to change the value of a variable by find and replace operation?
To change the value of a variable in any file use a regular expression to match the field and then replace the old value by new one.
Related Articles
- How to Get Date in Bash [2 Methods with Examples]
- How to Print Time in Bash [2 Quick Methods]
- How to List Users in Bash [2 Easy Ways]
- How to Get Current Time in Bash [4 Practical Cases]
- How to Use Date Format in Bash [5 Examples]
- How to Get Timestamp in Bash [2 Practical Cases]
- How to Copy and Paste in Bash [2 Methods & Cases]
- How to Read Password in Bash [3 Practical Cases]
- How to Send Email in Bash [2 Easy Methods]
- Bash Script to Send Email with Attachment [Step-by-Step Guide]
- How to Get IP Address in Bash [3 Methods]
- How to Get Script Name Using Bash Script? [3 Easy Ways]
- How to Call Another Script in Bash [2 Methods]
- How to Generate UUID in Bash [3 Simple Methods]
- 3 Easy Ways to Write to a File in Bash Script
- How to Write the Output to a File in Bash Script [5 Practical Cases]
- How to Create a List in Bash Scripts? [2 Easy Methods]
- How to Clear History in Bash [2 Practical Cases]
- How to Clear Screen Using Bash Script? [2 Effective Methods]
- How to Check Ubuntu Version Using Bash Scripts? [2 Methods]
<< Go Back to Bash Script Examples | Bash Scripting Basics | Bash Scripting Tutorial