FUNDAMENTALS A Complete Guide for Beginners
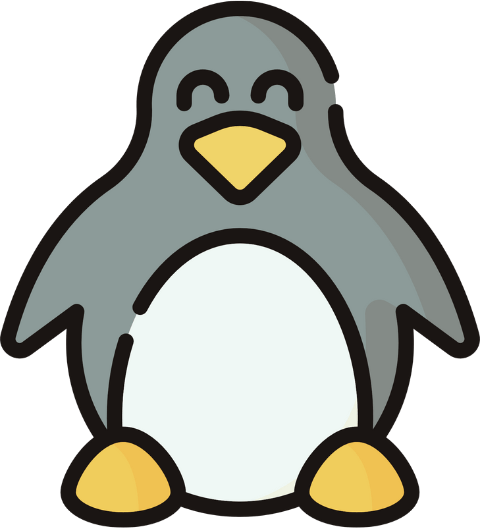
The date command in Bash offers multiple different formats for printing dates in different ways. This article provides you with an extensive list of all the available formatting options while using the date command. Along with the list, I will show you a few examples to demonstrate how to use a date format in Bash to achieve the desired result.
Different Formatting Options
The date command prints the current date and time in the following format by default:
‘Weekday_name Month_name Day_of_month Hour:Minutes:Seconds AM/PM Timezone(HH) Year’. For example, Tue Jul 11 12:14:01 PM +06 2023
See the following output, when the date command is run in the Ubuntu terminal: To achieve similar output manually, you can use the following command with different date formats:
date "+%a %b %d %I:%M:%S %p %:::z %Y"
Note: Place a plus sign (+) before the formatting options. If multiple formatting options are in action, put them within quotes.
To know more about each formatting option of the command and other available formatting options, see the list of Date Formatting Options.
5 Practical Examples of Using Date Format in Bash
At this point, I hope you are quite familiar with different formatting options. Now, I am going to show how to use those options and print the date in the required formatting.
1. Printing Date in “YYYY-MM-DD” Format
This first example is for making you more familiar with the format specifiers such as %Y and %d. These format specifiers are useful for printing dates in YYYY-MM-DD format.
To print date in YYYY-MM-DD format, use the following Bash script:
#!/bin/bash
today=$(date +%Y-%m-%d)
echo $today
The script utilizes the format %Y
to retrieve the full year (YYYY), %m
for the month (MM), and %d
for the day (DD). After substituting the date command in the variable “today“, it outputs the value of the variable using the echo command.The script prints today’s date 2023-07-11 in the expected format.
Note: Instead of using multiple format specifiers, you can use %F format to print the date in YYYY-MM-DD format
2. Printing Date in “YY-MM-DD” Format
In this example, I am going to run a Bash script that will ultimately print the date in YY-MM-DD format. Check the following bash script for the practical approach:
#!/bin/bash
today=$(date +%y-%m-%d)
echo $today
The script utilizes the format %y
to retrieve year (YY), %m
for the month (MM) and %d
for the day (DD). After substituting the date command in the variable “today“, it outputs the value of the variable using the echo command.
The program prints the current date 23-07-11 as specified by the format specifiers.
3. Printing Date in Weekday, Month Day, Year Format
Now, I want to print the date in a customized format where weekday and year should be separated by a comma (,). You can also achieve this using format specifiers of the following script:
#!/bin/bash
today=$(date “+%A, %B-%d, %Y”)
echo $today
The script specifies the format Finally, to get the full year (YYYY) it uses the format specifiers %A
to get the full name of the weekday. Then %B
and %d
to get the full name of the month and day of the month separated by a hyphen (-).%Y
. After substituting the date command in the variable “today“, it outputs the value of the variable using the echo command.
After executing the script, it displays the current date in the following format: Tuesday, July-11, 2023. The output includes the weekday, the month with the day of the month, and the year, with each item separated by a comma.
4. Printing the Day of the Current Week
Using the format specifiers of the date command you can print the day of the current week. The %u format specifier starts counting days of the current week from Monday. So, Monday is day 1 of the current week.
To print the day of the current week, use the following bash script:
#!/bin/bash
today=$(date +%u)
echo “Day of the week is: $today”
The script utilizes %u
to get the day of the current week. After substituting the date command in the variable “today“, it outputs the value of the variable using the echo command.After executing the script, it shows the Day of the week is 2. As the %u format specifier starts counting days of the week from Monday, 2 means Tuesday.
5. Printing Date in 24-Hour Format
While printing date someone may stumble in printing time in 24-hour format. Here is how you can use the date command to print time in 24-hour format:
#!/bin/bash
c_time=$(date +%H:%M:%S)
echo $c_time
The script utilizes the format %H
to retrieve the current hour in 24-hour format, %M
for minutes, and %S
for running second. After substituting the date command in the variable “c_time“, it outputs the variable’s value using the echo command.
When executing the program shows the current time 17:25:14 in a 24-hour time format.
Date Formatting Options
Apart from the examples in this article, you can achieve the desired date format using the wide variety of format specifiers given in the table below:
Format
Description
Example code
Sample Output
%%
A literal
$ date +%%
%
%a
Locale’s weekday name in shorter form; Such as Sun
$ date +%a
Sun
%A
Locale’s full weekday name; Such as Sunday
$ date +%A
Sunday
%b
Locale’s month name in shorter form
$ date +%b
Jun
%B
Locale’s full month name
$ date +%B
June
%c
Locale’s date and time
$ date +%c
Sun 18 Jun 2023 12:18:40 PM +06
%C
century
$ date +%C
20
%e
Day of month
$ date +%e
18
%F
Full date; same as %Y-%m-%d
$ date +%F
2023-06-18
%g
Last two digits of the year of ISO week number
$ date +%g
23
%G
year of ISO week number
$ date +%G
2023
%d
Day of month
$ date +%d
18
%D
Date; same as %m/%d/%y
$ date +%D
06/18/23
%H
Hour
$ date +%H
11
%I
Hour
$ date +%I
11
%j
Day of year
$ date +%j
169
%m
Month of year
$ date +%m
06
%M
Minute(00 to 59)
$ date +%M
32
%r
Locale’s 12-hour clock time
$ date +%r
11:12:14 AM
%q
Quarter of a year
$ date +%q
2
%s
seconds since 1970-01-01 00:00:00 UTC
$ date +%s
1687068749
%S
seconds(00 to 60)
$ date +%S
50
%t
A tab
$ date +%t
%T
Time as same as %H:%M:%S
$ date +%T
12:10:13
%u
Day of week(1 to 7); 1 is Monday
$ date +%u
7
%U
Week number of the year(0 to 53); Sunday as first day of week
$ date +%U
25
%V
ISO week number of the year(0 to 53); Monday as the first day of week
$ date +%V
24
%w
Day of week(0 to 6); 0 is sunday
$ date +%w
0
%W
Week of year(0 to 53); Monday as firstday of the week
$ date +%W
24
%x
Locale’s date representation
$ date +%x
06/18/2023
%X
Locale’s time representation
$ date +%X
12:04:10 PM
%y
Last two digits of year
$ date +%y
23
%Y
Full year
$ date +%Y
23
%z
+hhmm numeric time zone
$ date +%z
+0600
%:z
+hh:mm numeric time zone
$ date +%:z
+06:00
%::z
+hh:mm:ss numeric time zone
$ date +%::z
+06:00:00
%:::z
numeric time zone with : to necessary precision(+06, -05:30)
$ date +%:::z
+06
%Z
Time zone abbreviation
$ date +%Z
+06
Conclusion
In conclusion, the date command in Bash is useful for printing dates and times. One can use different format specifiers along with the date command to display or format the date as per requirement.
People Also Ask
How to set date format in shell script?
To set the date format in a shell script, you can use the date command with its different formatting characters. The most common of these characters are:
- %D: Date; same as %m/%d/%y
- %H: Hour
- %j: Day of year
- %m: Month of year
- %M: Minute(00 to 59)
- %s: seconds since 1970-01-01 00:00:00 UTC
- %S: seconds(00 to 60)
- %u: Day of week(1 to 7); 1 is Monday
- %U: Week number of the year(0 to 53); Sunday as first day of week
- %y: Last two digits of the year.
- %Y: Full year
How do you format dates in bash?
By using the date command, along with a specified format string, you can format dates in Bash. For example, executing date +%Y-%m-%d
will display the current date formatted as YYYY-MM-DD
.
How to get date of a day before the current date?
To get the date of a day before, use ‘yesterday’ or ‘1 day ago’ with the date command along with command option -d
. Use the syntax, date -d "yesterday" "+%Y-%m-%d"
.
How to increment a day in Bash script?
To increment a day from a specific date use +1 day
with the date command along with the -d
option.
Is placing the format specifiers within quotes in mandatory?
No. If you use single format specifiers you don’t need to place the specifier within quotes. However, when you use multiple specifiers at a time you need to put those specifiers within a single or double quote.
Related Articles
- How to Get Date in Bash [2 Methods with Examples]
- How to Print Time in Bash [2 Quick Methods]
- How to List Users in Bash [2 Easy Ways]
- How to Get Current Time in Bash [4 Practical Cases]
- How to Get Timestamp in Bash [2 Practical Cases]
- How to Copy and Paste in Bash [2 Methods & Cases]
- How to Read Password in Bash [3 Practical Cases]
- How to Send Email in Bash [2 Easy Methods]
- Bash Script to Send Email with Attachment [Step-by-Step Guide]
- How to Get IP Address in Bash [3 Methods]
- How to Find and Replace String in Bash [5 Methods]
- How to Get Script Name Using Bash Script? [3 Easy Ways]
- How to Call Another Script in Bash [2 Methods]
- How to Generate UUID in Bash [3 Simple Methods]
- 3 Easy Ways to Write to a File in Bash Script
- How to Write the Output to a File in Bash Script [5 Practical Cases]
- How to Create a List in Bash Scripts? [2 Easy Methods]
- How to Clear History in Bash [2 Practical Cases]
- How to Clear Screen Using Bash Script? [2 Effective Methods]
- How to Check Ubuntu Version Using Bash Scripts? [2 Methods]
<< Go Back to Bash Script Examples | Bash Scripting Basics | Bash Scripting Tutorial