FUNDAMENTALS A Complete Guide for Beginners
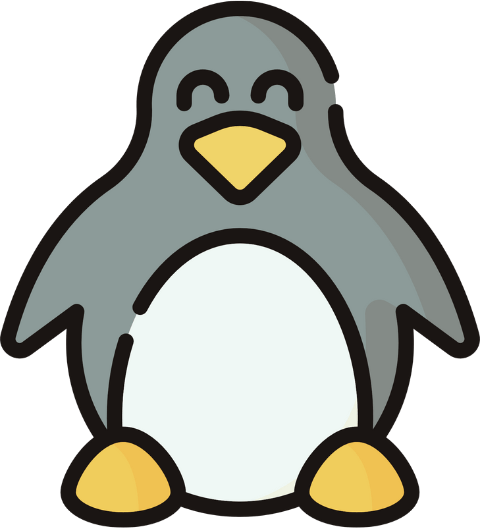
To print the current time in Bash, use the command:
echo $(date +%T)
Printing current time is useful for logging purposes, timing operations as well as displaying real-time information. Using the date
command with proper format specifiers one can display the time in various formats such as hours, minutes, and seconds, either in 24-hour or 12-hour clock notation. In this article, I am going to demonstrate how to print time in Bash and customize it.
Format Specifiers for Printing Time in Bash
Some useful format specifiers for printing time are listed below:
Format | Description | Example code | Sample Output |
---|---|---|---|
%H | Hour in 24-hour format | $ date +%H | 23 |
%I | Hour in 12-hour format | $ date +%I | 12 |
%M | Print minutes of current hour | $ date +%M | 46 |
%s | Print seconds of the current minute | $ date +%s | 35 |
%z | +hhmm numeric time zone | $ date +%z | +0600 |
%:z | +hh:mm numeric time zone | $ date +%:z | +06:00 |
%::z | +hh:mm:ss numeric time zone | $ date +%::z | +06:00:00 |
%:::z | numeric time zone with : to necessary precision(+06, -05:30) | $ date +%:::z | +06 |
%p | AM/PM designation |
1. Printing Time Using the “date” Command
The date command by default prints the current date and time. Also, it provides many options and format specifiers that allow users to print the current time without printing the date. For example:
- Print time with date:
echo $(date)
- Print time:
echo $(date +%T)
- Print hour and minute:
echo $(date +%H:%M)
- Print time with AM/PM notation:
echo $(date +%r)
The following script prints time in all available formats:
#!/bin/bash
today=$(date)
echo "Today's date: $today"
time_with_seconds=$(date +%T)
echo "Current time (with seconds): $time_with_seconds"
time_without_seconds=$(date +%H:%M)
echo "Current time (without seconds): $time_without_seconds"
with_Am_Pm=$(date +%r)
echo "Current time (12-hour format): $with_Am_Pm"
current_hours=$(date +%H)
echo "Current hours: $current_hours"
Executing this script will output as:
2. Printing Time Using the “timedatectl” Command
The timedatectl command is useful to configure the system’s date and time settings. But one can creatively use this command to get the system’s time. Follow the script below to visualize this:
#!bin/bash
time=timedatectl | awk '/Local time:/ {print $5}'
echo $time
The given Bash script captures the local time by executing the timedatectl command and extracting the fifth field from the line containing “Local time:” using the awk command. The extracted time value is assigned to the time variable using command substitution. Finally, the script displays the value stored in time using the echo command.
How to Get the Run Time Using the “time” Command in Bash?
To get the run time of a Bash script, use time
command before the commands to execute the script. The syntax is:
time ./example.sh
This will print the time required to execute the example.sh script.
Let’s check the execution time of the following script:
#!/bin/bash
echo "Enter a number:"
read n
val=0
power=1
while [ $n -ne 0 ]
do
r=$(( $n % 2 ))
val=$(( $r * $power + $val ))
power=$(( $power * 10 ))
n=$(( $n / 2 ))
done
echo "Binary output: $val"
This script takes a decimal number from the user and converts that into a binary equivalent, Now, execute this using time command before:This time I run the script by adding the time command as a prefix. You can see that the given input is again 4 and it successfully converts the number into its binary representation. Moreover, it shows three different times namely real, user, and sys time.
Conclusion
In conclusion, printing the local time or CST time is easier with the date command. On the other hand, one can use the time command to print system time. I believe with the knowledge of the above discussion, you can now print the local time in your machine as well as the system time of a Bash script.
People Also Ask
How to print time in Linux?
To print time in the terminal of Linux or any Unix operating system, you must go to the terminal and type the date
command.
How to get currenct time up to milliseconds in Bash?
To get current time up to milliseconds, use echo $(date +%H:%M:%S:%3N)
command.
How to print timestamp in Bash?
To print timestamp in Bash use the date +"%s"
command.
Related Articles
- How to Get Date in Bash [2 Methods with Examples]
- How to List Users in Bash [2 Easy Ways]
- How to Get Current Time in Bash [4 Practical Cases]
- How to Use Date Format in Bash [5 Examples]
- How to Get Timestamp in Bash [2 Practical Cases]
- How to Copy and Paste in Bash [2 Methods & Cases]
- How to Read Password in Bash [3 Practical Cases]
- How to Send Email in Bash [2 Easy Methods]
- Bash Script to Send Email with Attachment [Step-by-Step Guide]
- How to Get IP Address in Bash [3 Methods]
- How to Find and Replace String in Bash [5 Methods]
- How to Get Script Name Using Bash Script? [3 Easy Ways]
- How to Call Another Script in Bash [2 Methods]
- How to Generate UUID in Bash [3 Simple Methods]
- 3 Easy Ways to Write to a File in Bash Script
- How to Write the Output to a File in Bash Script [5 Practical Cases]
- How to Create a List in Bash Scripts? [2 Easy Methods]
- How to Clear History in Bash [2 Practical Cases]
- How to Clear Screen Using Bash Script? [2 Effective Methods]
- How to Check Ubuntu Version Using Bash Scripts? [2 Methods]
<< Go Back to Bash Script Examples | Bash Scripting Basics | Bash Scripting Tutorial