FUNDAMENTALS A Complete Guide for Beginners
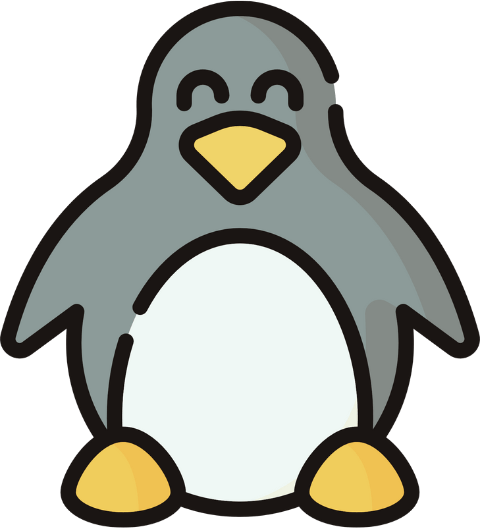
The date command in Bash is used to get the current time. The current time may differ depending on which current time it refers to. For example, someone may want the local current time, others may want the UTC time irrespective of timezone. Moreover one may be interested in the component of current time let’s say the current hour or minute. This article walks you through the use of the date command to get the time details you need.
Key Takeaways
- Printing current time in different formats.
- Getting UTC time using date command.
- Printing Unix time and converting it to UTC.
Free Downloads
4 Cases of Printing Current Time in Bash
Getting current time can be different based on the necessity of the user. One can print the current time in many ways using different format specifiers and options of date command. Now I am going to discuss four different cases of getting current time.
Case 1: Getting Current Time in “HH:MM:SS (12 Hour)” Format
To get the current time in HH:MM:SS format use %r format specifier in the date command. %T format specifier also works well however it will print time in 24-hour format. Now, I am going to print time in 12-hour format using %r.
❶ At first, launch an Ubuntu Terminal.
❷ Write the following command to open a file named currtime.sh in the build-in nano editor:
nano currtime.sh
- nano: Opens a file in the Nano text editor.
- currtime.sh: Name of the file.
Script (currtime.sh) >
#!/bin/bash
echo "Current time: $(date +"%r")"
The date command with the format specifier %r, retrieves time in a 12-hour format with AM/PM indicators. The echo command is used to display the time.
❹ Use the following two commands to make the file executable:
chmod u+x currtime.sh
- chmod: Changes permissions.
- u+x: Giving the owner executing permission.
- currtime.sh: Name of the script.
❺ Run the currtime.sh script by the following command:
./currtime.sh
Once executed the echo command prints the current time in 12-hour format with AM/ PM as shown in the image above.
Case 2: Getting Component of Current Time Using Bash Script
If you are interested only in a component of current time then you can use format specifiers like %I, %M, %S and %3N. For example, %I is used to get the current hour in a 12-hour format. %M is used to get the running minute of the current hour. %S is used to print current seconds and %3N can be used to print the second up to 3 decimal points precision. Look at the implementation of these in the Bash script below:
Script (component.sh) >
#!/bin/bash
current_hour=$(date +"%I")
current_minute=$(date +"%M")
current_second=$(date +"%S")
current_millisecond=$(date +"%3N")
echo "Current Hour: $current_hour"
echo "Current Minute: $current_minute"
echo "Current Second: $current_second"
echo "Current Millisecond: $current_millisecond"
This Bash script captures the current hour, minute, second and millisecond from the system’s time using the date command. It prints out each of these components separately using the echo command. The script retrieves the hour in 12-hour format (%I), the minute (%M), the second (%S) and milliseconds (%3N) and displays them as individual pieces of information.
Run the component.sh script by the following command:
./component.sh
As you can see the %I retrieve the current hour in a 12-hour format which is 2. %M retrieve the running minute(0 to 59) which is 57. Finally, %S and %3N are used to get the current second (0 to 59) and milliseconds (0 to 999) which are 44 and 859 respectively.
Case 3: Getting UTC Time Using Bash
UTC or Coordinated Universal Time is a time which is the same worldwide regardless of timezones. This time is important and used in many fields. The -u option of date command can effectively retrieve the current UTC time.
Script (utctime.sh) >
#!/bin/bash
current_utc_time=$(date -u +"%H:%M:%S %Z")
echo "Current UTC Time: $current_utc_time"
This Bash script obtains the current Coordinated Universal Time (UTC) by using the date command with the -u option, which indicates that the output should be in UTC.
The format specifier %H represents the hour, %M the minute, %S the second and %Z the time zone abbreviation. The script then echoes the current UTC time in [hh:mm:ss] [UTC] format.
Run the utctime.sh script by the following command:
./utctime.sh
Once executed the program successfully prints the current UTC time which is 09:04:46 UTC in case of the above execution.
Case 4: Printing Current Unix Time
Unix time or POSIX time is a way of tracking time. It represents the number of seconds that have elapsed since the Linux epoch- a specific point in time defined as 00:00:00 UTC on January 1, 1970. %s specifier is used to retrieve the current Unix time.
Script (currunix.sh) >
#!/bin/bash
current_unix_time=$(date +%s)
echo "Current Unix Time: $current_unix_time"
The date command with %s format specifier captures the Unix time and assigns it to the current_unix_time variable.
The echo command is used to display the current Unix time with necessary messages.
Run the currunix.sh script by the following command:
./currunix.sh
Once executed the script will print the current Unix time. In the above execution it prints 1692781661 as the running Unix time.
How to Get Current Time Using timedatectl Command
The timedatectl command extracts information about the time and date settings of the system. From the extracted information one can easily find out the local time, UTC time etc using the awk command.
Script (timedatectl.sh) >
#!bin/bash
curr_time=timedatectl | awk '/Local time:/ {print $5}'
echo $curr_time
The script first employs timedatectl to fetch information about the system’s date and time settings. Through the use of the awk command it targets the line containing “Local time“. The fifth field (column) of the targeted line corresponds to the local time value. {print $5} is used to store the extracted value within the curr_time variable. Finally, the echo command is utilized to print the value of curr_time.
Run the timedatectl.sh script by the following command:
./timedatectl.sh
As you can see, the current time 15:24:37 is printed in 24-hour format.
How to Convert UTC Unix Time to a Specific Timezone Using Bash Script
Let’s say you have a Unix time. Now you want to convert this into human readable UTC time. -d option of date command is useful for this purpose. The below script is designed to convert a given Unix timestamp into UTC time of a specified timezone using the -d option.
Script (converttime.sh) >
#!/bin/bash
utc_unix_time=1665248424
timezone="America/New_York"
echo "Unix time: $utc_unix_time"
converted_time=$(TZ=$timezone date -u -d "@$utc_unix_time" +"%Y-%m-%d %H:%M:%S %Z")
echo "Converted Time: $converted_time"
The utc_unix_time variable has a value of 1665248424, which is actually a Unix timestamp. The timezone variable is set to America/New_York. The TZ environment variable is temporarily set to the specified timezone. The date command is used with the -u option to indicate the input is in UTC. The -d option is utilized to convert the UTC Unix timestamp into the desired timezone.
The output format is defined using the format specifier %Y-%m-%d %H:%M:%S %Z, which represents year, month, day, hour, minute, second, and timezone abbreviation respectively.
Run the converttime.sh script by the following command:
./converttime.sh
Running the script will output the original Unix time and the corresponding UTC time in the designated time zone. As you can see Unix time 1665248424 is actually referring to 2022-06-08 13:07:04 UTC time.
Conclusion
In conclusion, getting the current time in Bash is important and useful. Maintaining a log, scheduling a specific task and keeping track of recent backups are inherently related to current time of various forms. I believe after reading this article, you will be nothing if not a pro in handling time-related issues in Bash.
People Also Ask
Related Articles
- How to Get Date in Bash [2 Methods with Examples]
- How to Print Time in Bash [2 Quick Methods]
- How to List Users in Bash [2 Easy Ways]
- How to Use Date Format in Bash [5 Examples]
- How to Get Timestamp in Bash [2 Practical Cases]
- How to Copy and Paste in Bash [2 Methods & Cases]
- How to Read Password in Bash [3 Practical Cases]
- How to Send Email in Bash [2 Easy Methods]
- Bash Script to Send Email with Attachment [Step-by-Step Guide]
- How to Get IP Address in Bash [3 Methods]
- How to Find and Replace String in Bash [5 Methods]
- How to Get Script Name Using Bash Script? [3 Easy Ways]
- How to Call Another Script in Bash [2 Methods]
- How to Generate UUID in Bash [3 Simple Methods]
- 3 Easy Ways to Write to a File in Bash Script
- How to Write the Output to a File in Bash Script [5 Practical Cases]
- How to Create a List in Bash Scripts? [2 Easy Methods]
- How to Clear History in Bash [2 Practical Cases]
- How to Clear Screen Using Bash Script? [2 Effective Methods]
- How to Check Ubuntu Version Using Bash Scripts? [2 Methods]
<< Go Back to Bash Script Examples | Bash Scripting Basics | Bash Scripting Tutorial