FUNDAMENTALS A Complete Guide for Beginners
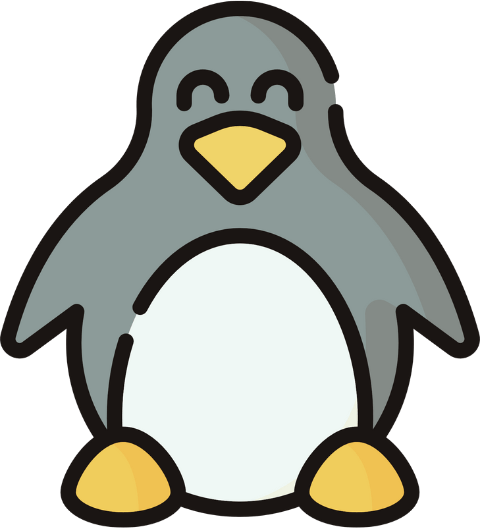
The date command in Bash scripting is widely used to retrieve and manipulate dates and times. It offers various formats to print the current, previous, or future dates. This command is useful for tasks like generating timestamps in log files or organizing files based on their creation dates. This article discusses the date command and its application in depth.
Key Takeaways
- Learning the basic syntax of the date command.
- Learning about available custom date formats.
- Arithmetic operations for getting a previous or future date using the date command.
- Application of date command (Creating timestamps in log files).
Free Downloads
The “date” Command in Bash
One can implement the date command and its options using the following command structure.
Command Syntax >
echo [OPTION]...[+FORMAT]
Useful Options
The date command offers only a few options like -d, -f -s, etc.
2 Methods of Displaying Current Date in Bash
Getting the current date is important for any system user. There are two basic ways of getting the current date in Bash. I am going to discuss both methods in this article.
Method 1: Using “date” Command in Bash
One can easily get the current date and time by executing the date command from the terminal or by substituting the date command in a Bash script.
❶ At first, launch an Ubuntu Terminal.
❷ Write the following command to open a date.sh file in the build-in nano editor:
nano date.sh
- nano: Opens a file in the Nano text editor.
- date.sh: Name of the file.
# !bin/bash
current_date=$(date)
echo “Today is: $current_date”
❹ Use the following command to make the file executable:
chmod u+x date.sh
- chmod: Changes permissions.
- u+x: Giving the owner executing permission.
- date.sh: Name of the script.
./date.sh
The program prints the current date after the text “Today is: ”.
Method 2: Using the “printf” Command in Bash
Another approach to obtaining the current date in a formatted text is by using the printf command. It allows you to print the current date with specific formatting.
Scripts (printf.sh) >
#!bin/bash
formatted_date=$(printf "%(%Y-%m-%d)T")
echo $formatted_date
The script prints the current date in year-month-day format using the printf command. The command is executed and output is stored in the formatted_date variable. Later, it echoed back using the echo command.
Run the script by the following command:
./printf.sh
The image above shows that the program prints the current date which is 2023-06-21.
Comparative Analysis of Methods
Here is a comparative analysis between the above-discussed methods. Take a look at the analysis to determine which method suits your needs best.
Method | Advantage | Disadvantage |
---|---|---|
date command |
|
|
printf command |
|
|
While both methods are suitable for the task, I personally find the date command more flexible. It provides a wide range of format specifiers, allowing for greater flexibility in displaying dates. Moreover, it allows the printing of both date and time simultaneously.
2 Basic Examples of “date” Command in Bash
The date command is primarily used for getting the current date and time of the system. However, the available options and formats give a user the flexibility to use and manipulate the command in various ways.
Example 1: Getting Past and Future Dates Using Bash Script
The options of the date command are assigned to perform various tasks. For example, one can set a new system time or get a date other than the current date using different options of the date command.
Scripts (dateoption.sh) >
#!bin/bash
# Get yesterday's date
yesterday=$(date -d "yesterday")
echo "Yesterday's date: $yesterday"
# Get date of day before yesterday
b_yesterday=$(date -d "-2 day")
echo "Date of day before yesterday: $b_yesterday"
# Date of the same day in the next week
n_week=$(date -d "next week")
echo "Yesterday's date: $n_week"
# Set a custom date
custom_date="2023-06-30"
sudo date -s "$custom_date" # Requires root privileges
Here the -d command option is used to get yesterday’s date. Later the echo command is used to print yesterday’s date that was saved in the ‘yesterday’ variable.
Later, it prints the day before yesterday using the -d option along with the given parameter “-2 day“.
Afterward, the program uses the -d option once more with the parameter “next week” to show the date of the same day in the next week.
Another command option -s is used to set a new system date and time. This requires root privilege which is given by the sudo command.
Run the script by the following command:
./dateoption.sh
The program shows yesterday’s date, date of the day before yesterday, and displays a new command prompt for the user to input the password in order to change the system’s date.
Arithmetic Operations Within “date” Command
At this point, you should know a few arithmetic operations that can be performed with the date command. The list below contains a demonstration of such operations.
Command | Example Output | Details |
---|---|---|
$ date | Wed Jun 21 12:29:10 PM +06 2023 | Display the current date. |
$ date -d “+5 days” | Mon Jun 26 12:29:54 PM +06 2023 | Display date after 5 days. |
$ date -d “1+tomorrow” | Thu Jun 22 01:00:00 AM +06 2023 | Display the date of the day after tomorrow. |
$ date -d “Jun 18, 2023 +1 week” | Sun Jun 25 12:00:00 AM +06 2023 | Display date of the day after 1 week from the date Jun 18, 2023. |
Example 2: Formatting Date With Various Format Specifiers
The date command offers a huge number of formatting options for printing date and time. The following script demonstrates a few of them. By mentioning the format specifiers, you can customize the output of the date command to suit different date and time representations.
Scripts (dateformat.sh) >
#!/bin/bash
# Get current date and time
current_date=$(date)
echo "Current date and time: $current_date"
# Get date in a specific format
formatted_date=$(date +"%Y-%m-%d")
echo "Formatted date: $formatted_date"
# Get date with day of the week
formatted_date_with_day=$(date +"%A, %B %d, %Y")
echo "Formatted date with day of the week: $formatted_date_with_day"
# Get time in a specific format
formatted_time=$(date +"%H:%M:%S")
echo "Formatted time: $formatted_time"
The script begins by capturing the current date and time using the date command and storing it in the current_date variable. Then, it prints the current date and time using echo.
The script continues by formatting the date in a specific format, YYYY-MM-DD, and storing it in the formatted_date variable. This formatted date is also displayed.
Next, it formats the date with the day of the week using %A, %B, %d, and %Y format specifier, representing the full weekday name, month name, day, and year. The output is displayed as before.
Lastly, the script formats the current time as HH:MM:SS and displays it.
Run the script by the following command:
./dateformat.sh
In this image, you can see that the program initially prints the current date and time. Later it prints the date “2023-06-20” which was specified to use the format “%Y-%m-%d“.
In the next part, it prints Tuesday and June in full form which was specified using the specifiers %A and %B. Finally, it prints the current time in HH:MM:SS format.
Format Specifiers of Date Command
The most useful format specifiers of the date command are listed below for your convenience.
Format | Description | Example code | Sample Output |
---|---|---|---|
%% | A literal | $ date +%% | % |
%a | Locale’s weekday name in shorter form; Such as Sun | $ date +%a | Sun |
%A | Locale’s full weekday name; Such as Sunday | $ date +%A | Sunday |
%b | Locale’s month name in shorter form | $ date +%b | Jun |
%B | Locale’s full month name | $ date +%B | June |
%c | Locale’s date and time | $ date +%c | Sun 18 Jun 2023 12:18:40 PM +06 |
%C | century | $ date +%C | 20 |
%e | Day of month | $ date +%e | 18 |
%F | Full date; same as %Y-%m-%d | $ date +%F | 2023-06-18 |
%g | Last two digits of the year of ISO week number | $ date +%g | 23 |
%G | year of ISO week number | $ date +%G | 2023 |
%d | Day of month | $ date +%d | 18 |
%D | Date; same as %m/%d/%y | $ date +%D | 06/18/23 |
%H | Hour | $ date +%H | 11 |
%I | Hour | $ date +%I | 11 |
%j | Day of year | $ date +%j | 169 |
%m | Month of year | $ date +%m | 06 |
%M | Minute(00 to 59) | $ date +%M | 32 |
%r | Locale’s 12-hour clock time | $ date +%r | 11:12:14 AM |
%q | Quarter of a year | $ date +%q | 2 |
%s | seconds since 1970-01-01 00:00:00 UTC | $ date +%s | 1687068749 |
%S | seconds(00 to 60) | $ date +%S | 50 |
%t | A tab | $ date +%t | |
%T | Time as same as %H:%M:%S | $ date +%T | 12:10:13 |
%u | Day of week(1 to 7); 1 is Monday | $ date +%u | 7 |
%U | Week number of the year(0 to 53); Sunday as first day of week | $ date +%U | 25 |
%V | ISO week number of the year(0 to 53); Monday as the first day of week | $ date +%V | 24 |
%w | Day of week(0 to 6); 0 is sunday | $ date +%w | 0 |
%W | Week of year(0 to 53); Monday as firstday of the week | $ date +%W | 24 |
%x | Locale’s date representation | $ date +%x | 06/18/2023 |
%X | Locale’s time representation | $ date +%X | 12:04:10 PM |
%y | Last two digits of year | $ date +%y | 23 |
%Y | Full year | $ date +%Y | 23 |
%z | +hhmm numeric time zone | $ date +%z | +0600 |
%:z | +hh:mm numeric time zone | $ date +%:z | +06:00 |
%::z | +hh:mm:ss numeric time zone | $ date +%::z | +06:00:00 |
%:::z | numeric time zone with : to necessary precision(+06, -05:30) | $ date +%:::z | +06 |
%Z | Time zone abbreviation | $ date +%Z | +06 |
Creating Timestamps for Log Files Using “date” Command
Timestamps of log files are important to notify users about the last modification time of a file. One can use the date command to create a Bash program that will append timestamps on a log file after each modification.
Scripts (timestamp.sh) >
#!/bin/bash
# Get the current timestamp
timestamp=$(date +"%Y-%m-%d %H:%M:%S")
# Log message
log_message="This is a log message."
# Append the timestamp and log message to the log file
echo "$timestamp $log_message" >> log_file.txt
This script captures the current time and date using the date command and formats it as YYYY-MM-DD HH:MM:SS. The formatted timestamp is then stored in the timestamp variable. The final line appends the timestamp and log message to a log file.
Run the script by the following command:
./timestamp.sh
The output shows that the script adds a timestamp on the log_file.txt. When visualizing the file using the cat command, it shows 2023-06-20 17:40:21 timestamp is appended with the log message.
Conclusion
In conclusion, the date command is very useful for finding present, past, and future dates. One can print those in many formats. Hope this article will help you in printing dates in whatever formats you like.
People Also Ask
Related Articles
- How to Print Time in Bash [2 Quick Methods]
- How to List Users in Bash [2 Easy Ways]
- How to Get Current Time in Bash [4 Practical Cases]
- How to Use Date Format in Bash [5 Examples]
- How to Get Timestamp in Bash [2 Practical Cases]
- How to Copy and Paste in Bash [2 Methods & Cases]
- How to Read Password in Bash [3 Practical Cases]
- How to Send Email in Bash [2 Easy Methods]
- Bash Script to Send Email with Attachment [Step-by-Step Guide]
- How to Get IP Address in Bash [3 Methods]
- How to Find and Replace String in Bash [5 Methods]
- How to Get Script Name Using Bash Script? [3 Easy Ways]
- How to Call Another Script in Bash [2 Methods]
- How to Generate UUID in Bash [3 Simple Methods]
- 3 Easy Ways to Write to a File in Bash Script
- How to Write the Output to a File in Bash Script [5 Practical Cases]
- How to Create a List in Bash Scripts? [2 Easy Methods]
- How to Clear History in Bash [2 Practical Cases]
- How to Clear Screen Using Bash Script? [2 Effective Methods]
- How to Check Ubuntu Version Using Bash Scripts? [2 Methods]
<< Go Back to Bash Script Examples | Bash Scripting Basics | Bash Scripting Tutorial