FUNDAMENTALS A Complete Guide for Beginners
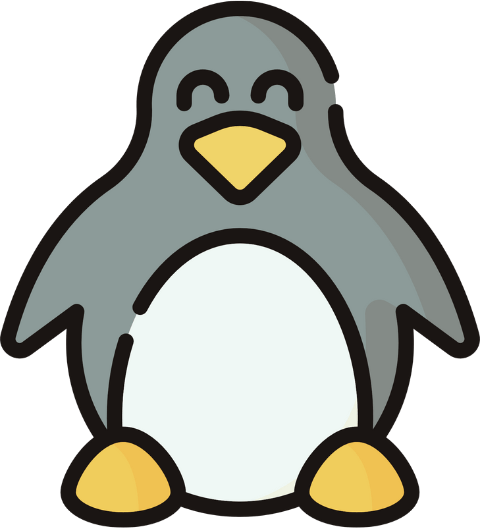
Bash programmers do the replacement, the substitution, and the removal of the bash variable too often. It helps them print necessary text on the terminal, customize a command with the necessary option to get their necessary output, replace unnecessary words from a text, concatenate two or more variables and print, and do arithmetic operations. In this article, I will demonstrate to you these types of tasks which will help you to get a holistic idea of this topic.
Syntax to Substitute, Replace, Remove Variable and Substring
- To substitute the Bash variable:
echo "String, $variable_name!"
- To replace substring from string:
new_string="${original/old_substring/new_substring}"
- To remove a substring from a variable:
result="${original_string/$substring_to_remove/}"
Variable Substitution in Bash
Bash variable substitution is a process where the value of a variable substitutes or replaces another variable. The $(..) syntax is commonly used for variable substitution.
I have listed some examples related to this topic below. The examples are simple variable substitution, concatenation of string using variable substitution, arithmetic substitution using arithmetic expansion, and command substitution by creating customized commands.
Example 01: Simple Variable Substitution
To print a text with the variable substitution, use the following script:
#!/bin/bash
#assigning the value of the name variable
name="John"
#substituting name variable
echo "Hello, $name!"
Here, name="John"
assigns the value ‘John’ to the name variable. Then, echo "Hello, $name!"
prints a text on the terminal using the echo command where the name variable is replaced by the assigned value.
The image shows that the bash script has printed a text on the terminal where the name variable is substituted by its assigned value.
Example 02: Concatenation of String Utilizing Variable Substitution
To concatenate string within two variables using variable substitution, use the following script:
#!/bin/bash
#assigning first_name and last_name variable
first_name="John"
last_name="Doe"
#concatenating two variables utilizing variable substitution
full_name="$first_name $last_name"
#printing the concatenated result
echo "Full Name: $full_name"
first_name="John"
and last_name="Doe"
assign value to the first_name and last_name variables. Then, full_name="$first_name $last_name"
concatenates the value of the first_name and the last_name variables and kept it on the full_name variable. Finally, echo "Full Name: $full_name"
prints the value on the terminal.
The Bash script has concatenated two variables using variable substitution and printed on the terminal. Here John and Doe from the output line come from the first_name and the last_name variable respectively. After concatenation, I got Jogn Doe on the terminal.
Example 03: Arithmetic Substitution Using Arithmetic Expansion
Arithmetic substitution helps in performing mathematical executions. Follow the script provided below to do arithmetic substitution using arithmetic expansion:
#!/bin/bash
#assigning value to the num1 and the num2 variable
num1=5
num2=3
#arithmetic Substitution
result=$((num1 + num2))
#printing the result
echo "Sum of $num1 and $num2:$result"
num1=5
and num2=3
assigns value to the num1 and the num2 variables. Then, result=$((num1 + num2))
calculates the summation of the num1 and the num2 variables with the help of arithmetic substitution. Finally, echo "Sum $num1 and $num2:$result"
displays the result on the terminal.
The bash script has calculated the summation of the num1 and the num2 variables utilizing the arithmetic substitution method.
Example 04: Command Substitution by Creating Customized Command
Users can create a customized command and then print the output of the customized command on the terminal.
To create a customized command and print its output, use the following script:
#!/bin/bash
#creating a customized date
current_date=$(date +%Y-%m-%d)
#printing the output of the customized command
echo "Current Date: $current_date"
current_date=$(date +%Y-%m-%d)
creates a customized date where Y
specifies year, m
specifies month and the d
specifies day. Then, echo "Current Date: $current_date"
prints the output of the customized command.
Replacing Substring in a Variable
Bash variable replacement is a process where the value of a variable replaces or variable. I have listed some examples related to this topic below.
Example 01: Replace a Single Occurrence Using Single Slash (/) Operator
In this example, I will develop a bash script that will look for a match of a specific substring for a single time and will replace it with another substring.
To replace a specific substring with another substring using a single slash (/) operator, follow the below script:
#!/bin/bash
#original variable is assigned
original="Hello, World!"
#”World” will be replaced by “Everyone” from the original variable and kept on the new_string
new_string="${original/World/Everyone}"
#new_string will be printed
echo "$new_string"
Here, original="Hello, World!"
assigns a value to the original variable. Then, new_string="${original/World/Everyone}"
replaces World with Everyone. Finally, echo "$new_string"
prints the new_string variable value on the terminal.
The image shows that the bash script has replaced the “World” with “Everyone” and printed the updated string on the terminal.
Example 02: Replace All Occurrences Using Double Slash (//) Operator
Following the previous method, I will develop another bash script in a different way that will look for a match of a specific substring and replace it with another substring. This is just another approach to using a single slash(/).
To replace a specific substring with another substring using the double slash (//) operator, follow the below script:
#!/bin/bash
#string assigning
original="She sells seashells by the seashore. She shells seashells for sure."
#sh will be replaced by SH
new_string="${original//sh/SH}"
#printing the result
echo "$new_string"
original="She sells seashells by the seashore. She shells seashells for sure."
assigns a string to the original variable. Next, new_string="${original//sh/SH}"
replaces all sh with SH and keeps it on the new_string. Finally, echo "$new_string"
prints the value of the new_string variable on the terminal.
The image shows that the Bash script has replaced the character “SH” with the character “sh” and printed the new_string on the terminal.
Example 03: Replace One Character with Another Using tr Command
One might use one character in place of another character. In such a situation, the programmer can replace one character with another using the tr command. To know more, follow the below script.
To replace one character with another using the tr command, follow the script provided below:
#!/bin/bash
#assigning main string
Main_string="Linux Simply"
#replacing n by p and save it to New_string
export New_string=$(echo "$Main_string" | tr 'n' 'p')
#printing the value of Main_string
echo "Main_string:" $Main_string
#printing the value of Modified_string
echo "Modified_string:" $New_string
Here, Main_string="Linux Simply"
assigns value to Main_string. Then, export New_string=$(echo "$Main_string" | tr 'n' 'p')
replaces n with p with the help of the tr command and the export command. Next, echo "Main_string:" $Main_string
prints the value of the Main_string. Finally, echo "Modified_string:" $New_string
prints the value of the New_string.
The image shows that the Bash script has replaced the n character with the p character with the help of the tr command.
An alternative to using the tr command is parameter expansion. The syntax for parameter expansion is:
New_string="${Main_string//(replaced character)/(new character)}"
Removing Substring from a Variable
Bash variable removal is a process where some words or sub-strings from the main string is deleted. I have listed some examples related to this topic below.
Example 01: Remove a Specific Substring From a Given String by Using Single Slash (/) Operator
In this example, I will develop a Bash script that will remove a substring from a sentence utilizing the single slash(/).
To remove a specific substring from a given string, use the following code:
#!/bin/bash
# Original sentence
sentence="Hello, world! This is an example."
# Substring to remove
substring="world"
# Remove substring using parameter expansion
result="${sentence/$substring/}"
# Print the result
echo "Original Sentence: $sentence"
echo "Substring to Remove: $substring"
echo "Result after removal: $result"
sentence="Hello, world! This is an example."
and substring="world"
assign strings to the sentence and the substring variables. Then, result="${sentence/$substring/}"
removes the substring from the sentence utilizing the single slash(/). Finally, echo "Original Sentence: $sentence"
, echo "Substring to Remove: $substring"
, and echo "Result after removal: $result"
print the original sentence, the substring, and the result on the terminal.
The Bash script has replaced the sub-string from the main string by utilizing the single slash(/) and printed the new string.
Example 02: Using Double Slash(//) Operator to Remove a Specific Substring from a Given String
Here, I will develop a Bash script that will remove a substring from a sentence utilizing the double slash(//). This is an alternative approach of the single slash(/) approach.
To remove a specific substring from a given string, follow the below script:
#!/bin/bash
#original and substring_to_remove string assigned
original_string="Hello, world!"
substring_to_remove="world"
#sub-string will be removed from the original string and kept on the result
result="${original_string//$substring_to_remove/}"
#result will be printed
echo "$result"
original_string="Hello, world!"
and substring_to_remove="world"
assign value to the original_string variable and the substring_to_remove variable. Then, result="${original_string//$substring_to_remove/}"
removes the substring_to_remove from the original_string utilizing the double slash(//). Finally, echo "$result"
prints the result on the terminal.
The Bash script has replaced the sub-string from the main string with the utilizing the double slash(//) and printed the new string.
Example 03: Remove Substring by Using sed (Stream Editor) Command
Here, I will develop a Bash script that will remove a substring from a original string utilizing the sed command.
To remove a substring using the sed command, follow the below script.
#!/bin/bash
#value assigning
original_string="Hello, world!"
substring_to_remove="world"
#removing substring_to_remove from original_string using sed command
result=$(echo "$original_string" | sed "s/$substring_to_remove//g")
#printing the result on the terminal
echo "$result"
original_string="Hello, world!"
and substring_to_remove="world"
assign value to the original_string and the substring_to_remove variable. Then, result=$(echo "$original_string" | sed "s/$substring_to_remove//g")
removes the substring_to_remove from the original_string utilizing the sed command. Finally, echo "$result"
displays the result on the terminal.
The Bash script has removed the sub-string “world” from the main string with the help of the sed command and printed the new string Hello on the command line.
Example 04: Removing of a Substring by Using the awk Command
In this example, I will develop a Bash script that will remove a substring from an original string utilizing the awk command.
To remove the substring by using awk command, follow the below script:
#!/bin/bash
# Remove "world" from the variable
original_string="Hello, world!"
substring_to_remove="world"
#removing substring_to_remove from original_string using awk command
result=$(echo "$original_string" | awk -v var="$substring_to_remove" '{gsub(var,"")}1')
#printing the result on the terminal
echo "$result"
original_string="Hello, world!"
and substring_to_remove="world"
assign value to the original_string and the substring_to_remove variable. Then, result=$(echo "$original_string" | awk -v var="$substring_to_remove" '{gsub(var,"")}1')
command has piped the output of echo command to awk command which removed the substring_to_remove from the original_string. Finally, echo "$result"
prints the result on the terminal.
The Bash script has removed the sub-string from the main string with the help of the awk command and printed the new string.
Conclusion
In this article, I have tried to explore the full process of bash variable substitution and replace characters. I believe, after going through this article, you will be productive enough to remove or a sub-string from the main string, customize a command, or replace a variable by its value.
People Also Ask
Which expression is used in bash for command substitution?
The $(..) syntax is the basic syntax for command substitution where the command to be executed is enclosed between the parentheses. This form is the recommended syntax of command substitution.
Can you use substitution with 3 variables?
Yes, you can use substitution with 3 variables. Concatenating strings from 3 variables which is the basic demonstration of the usage of variable substitution with three variables.
Are bash variables case-sensitive?
Yes, bash variables are case-sensitive. Variables in Bash Scripts are untyped and declared on the definition. Bash also supports some basic type declaration using the declare option, and bash variables are case-sensitive.
Can Bash variables have numbers?
Yes, Bash variables can have numbers. A variable in Bash can contain a number, a character, or a string of characters. Variable names can contain uppercase, and lowercase letters, numbers, underscores, and digits.
How do I replace a character in a variable in bash?
To replace a character in a variable in Bash, use the syntax below:
${original/character_to_be_replaced/new_character}
Here, the original is the main string, character_to_be_replaced is the substring to be replaced, and finally the new_character is the new substring which will be in place of character_to_be_replaced.
Related Articles
- How to Echo Variables in Bash Script? [4 Practical Examples]
- How to Use String Variables in Bash Script? [4 Cases]
- How to Append String to Bash Variable? [2 Effective Ways]
- How to Check If Bash Variable Exists? [2 Effective Methods]
- How To Check if Bash Variable is Empty? [2 Easy Methods]
- How to List and Set Bash Environment Variables? [3 Methods]
- 2 Ways to Unset Environment Variables Using Bash Script
- 5 Methods to Check If Environment Variable is Set in Bash Script
- How to Set Bash $PATH Variable? [Easiest Configuration]
- 2 Cases to Execute Command Stored in Bash Variable
- How to Store Command Output to Bash Variable? [3 Examples]
- How to Read a File into Bash Variable? [2 Simple Methods]
- How to Write Bash Variable to File? [3 Effective Methods]
- Compare Variables in Bash Scripts [3 Practical Cases]
- Increment Variable Value in Bash Scripts [4+ Examples]
- Adding 1 to Bash Variable [3 Examples]
- Decrement Variable Value in Bash Scripts [4+ Examples]
- Addition of Bash Variable [4+ Examples]
- How to Subtract Two Bash Variables? [4+ Easy Approaches]
- How to Multiply Variable in Bash [6+ Practical Examples]
<< Go Back to Using Variables in Bash Scripting | Bash Variables | Bash Scripting Tutorial