FUNDAMENTALS A Complete Guide for Beginners
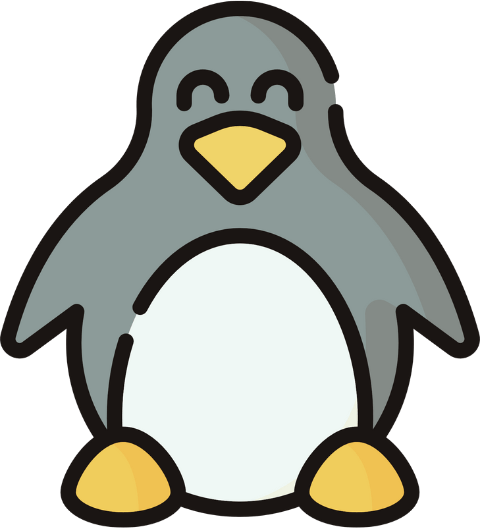
When writing shell scripts in Bash, choosing appropriate names for variables is essential for code readability and maintainability. By adhering to consistent naming conventions, you can enhance the clarity and understandability of your scripts, making them easier to debug and modify. This article will state some rules for Bash Variable naming conventions in the shell script.
Key Takeaways
- Learning of Bash Variable Naming Conventions.
- Creating your own variable name using the provided materials.
- An assignment to test your understanding of the Variable Naming Convention.
Free Downloads
6 Different Bash Variable Naming Conventions in Shell Script
To build a comprehensive and easily understandable code, naming the variables with a proper guideline is crucial. This also allows one to avoid any unexpected errors occurring during Debugging time.
While Bash doesn’t enforce any strict naming conventions, developers often follow certain conventions to enhance code clarity and consistency. Down below, I have discussed six different rules that should be followed while choosing your variable name.
Rule 01: Naming a Variable Using Alphabets and Numbers
While alphabets are commonly used in variable names, incorporating numbers can add a level of specificity and clarity to your code. However, bash script is a case-sensitive language which means you can store different data in the same variable having different case combinations.
❶ At first, launch an Ubuntu Terminal.
❷ Write the following command to open a file in Nano:
nano var_alphabet.sh
- nano: Opens a file in the Nano text editor.
- var_alphabet.sh: Name of the file.
❸ Copy the script mentioned below:
#!/bin/bash
#Variable naming using only alphabets
var=35
#Using Uppercase
VAR="VAR variable Contains Upper Case Letter"
#Variable naming using alphabets and numbers
var123=34.4
echo $var
echo $VAR
echo $var123
The first line #!/bin/bash specifies the interpreter to use (/bin/bash) for executing the script. Then, there are three variables var, VAR, and var123 created to store some data in it. Later, the echo command was used to print their stored value to prove that all those variables’ naming are correct and in compliance with the bash script naming convention.
❹ Press CTRL+O and ENTER to save the file; CTRL+X exit.
❺ Use the following command to make the file executable:
chmod u+x var_alphabet.sh
- chmod: is used to change the permissions of files and directories.
- u+x: Here, u refers to the “user” or the owner of the file and +x specifies the permission being added, in this case, the “execute” permission. When u+x is added to the file permissions, it grants the user (owner) of the file permission to execute (run) the file.
- var_alphabet.sh: is the name of the file to which the permissions are being applied.
❻ Run the script by the following command:
./var_alphabet.sh
From the above image, you can see that all three variables have returned their respective values: 35, VAR variable Contains Upper Case Letter, and 34.4.
Rule 02: Avoid Using Number at the Beginning of the Variable Name
When it comes to naming variables in Bash shell scripts, one important convention to follow is to avoid using a numeric digit as the first character of a variable name. Here is a sample example demonstrated below where I created several variables placing the number digit at the beginning and end of the variable name. The variable with the number digit at the beginning does not return its corresponding value in the execution time.
You can follow the steps of rule 01, to save & make the script executable.
Script (var_number.sh) >
#!/bin/bash
#variable with number first (invalid way)
123var="Wrong Way to name"
#correct way
var123="Correct Naming"
echo $123var
echo $var123
Here, #! /bin/bash: ‘#!’, is called shebang or hashbang. It indicates the interpreter to be used for executing the script, in this case, it’s bash. Next, a random variable 123var has been created by placing the number digit first. Another variable var123 is also named to show the correct way of naming. Later, the echo command displays the corresponding value contained in the variable.
Rule 03: Using Underscore to Avoid Whitespace
If a variable name consists of multiple words, it is advisable to separate them using underscores (_). For instance, user_name is advisable instead of using username
You can follow the steps of rule 01, to save & make the script executable.
Script (var_underscore.sh) >
#!/bin/bash
#bad way to name your variable
var name="jhon"
#correct way to name your variable
var_name="Tom"
echo $var name
echo $var_name
The #!/bin/bash is called a shebang, and it specifies the interpreter (in this case, /bin/bash) that should be used to execute the script. Next, the var_name variable is created and tried to display using the echo command. Similarly, another var_name variable is created to store a string inside it and displayed later.
As you can see from the above image, only the var_name variable returns its string value “Tom” during execution time.
Rule 04: No Whitespace on Either Side of the Assignment Operator(=)
When using this operator, it is recommended to have no whitespace (spaces or tabs) immediately before or after the operator. The interpreter takes it as a command instead of assigning value to the variable otherwise. Here is a relevant example stated below.
You can follow the steps of rule 01, to save & make the script executable.
Script (var_whitespace.sh) >
#!/bin/bash
#Wrong way to declare assign data
var1 =23
var2= 25
#Correct way
var3="Great"
#print the variables
echo $var1
echo $var2
echo $var3
Here, #! /bin/bash: ‘#!’, is called shebang or hashbang. It indicates the interpreter to be used for executing the script, in this case, it’s bash. Then a variable var1 is created and a whitespace is there followed by the var1. In the case of the second variable, var2, there is another whitespace followed by the equal sign. Both cases are wrong and thus will occur an error in execution time. Later another variable var3 has been created in the correct way and then displayed with the echo command.
Rule 05: Avoiding Special Characters (Except Underscore)
In general, it is best to stick to alphanumeric characters (letters and numbers) and underscores when naming variables. Avoid using special characters, whitespace, or punctuation marks, as they can cause syntax errors or introduce unexpected behaviour. For instance, using any special character such as @, $, or # anywhere while declaring a variable is not legal.
You can follow the steps of rule 01, to save & make the script executable.
Script (var_special_character.sh) >
#!/bin/bash
#wrong way to name a variable
var@=5
var#=8
var$=23
#To display the variable data
echo $var@
echo $var#
echo $var$
#Correct way to name
var=45
echo $var
Here, #! /bin/bash: ‘#!’, is called shebang or hashbang. It indicates the interpreter to be used for executing the script, in this case, it’s bash. Next, three different variables are created using special characters such as @, #, $. Next, all three variables are called using the echo command to display their individual data. Later, another variable var is also created and displayed using the echo command.
Rule 06: Avoid Reserved Keywords or Built-in Command Names as Variable Names
Bash has a set of reserved keywords that have special meanings and functionalities within the shell. Although you will get the variable data, to prevent conflicts and unexpected behaviour, it is crucial to avoid using these reserved keywords as variable names. Examples of reserved keywords include if, else, case, do, while, etc.
You can follow the steps of rule 01, to save & make the script executable.
Script (var_Reserved_keyword.sh) >
#!/bin/bash
#Wrong way to name your variable
while=56
if=34
elif=67
#To display the variable value
echo $while
echo $if
echo $elif
#correct way to name
variable=50
echo $variable
Here, #! /bin/bash: ‘#!’, is called shebang or hashbang. It indicates the interpreter to be used for executing the script, in this case, it’s bash. Some reserved words such as while, if, elif, etc store data in this code. Then it displays the value using the echo command.
Comparison of Correct and Incorrect Variable Naming Conventions
Lastly, I draw a summary of the discussion in a table to describe the permissible and non-permissible ways to name the variable. Check this out to get the overall view.
Correct Naming Convention | Incorrect Naming Convention |
---|---|
Use lowercase letters and underscores. | Start with a number or special character (except underscore). |
Start with a letter or underscore. | Contain spaces or special characters like !, @, #, %, etc. |
Consist of lowercase letters, underscores, and digits. | Use uppercase letters. |
Can include numbers and underscores within the name (except as the first character). | Incorporating reserved keywords or built-in command names as variable names. |
Use descriptive and meaningful names. | Use inconsistent or ambiguous names that hinder code readability. |
Assignment on Bash Variable Naming Convention
Here I have also made a list of assignments to practice by yourself. Don’t forget to share your answer in the comment section.
- Using uppercase letters in variable names is acceptable. (True/False)
- We can use hyphens (-) in variable names in Bash. (True/False)
- Bash is case-sensitive, so myVar and myvar are considered different variables. (True/False)
- We can use Whitespace in variable names in Bash. (True/False)
- Variable names can contain special characters like !, @, and # in Bash. (True/False)
- Provide a valid variable name in Bash that includes both uppercase and lowercase letters, as well as an underscore.
- Determine whether the following variable names are correct or not:
- myVariable
- 123abc
- User_name
- My variable
- MyVar
- myVar!
Conclusion
In conclusion, adhering to proper variable naming conventions is essential when working with Bash variables in shell scripts. In this article, I have tried to give you some must known rules to follow while choosing your variable name. Things are easy if you remember however if you have any query related to this article. Feel free to comment below. Thank you.
People Also Ask
Related Articles
- How to Declare Variable in Bash Scripts? [5 Practical Cases]
- How to Assign Variables in Bash Script? [8 Practical Cases]
- How to Check Variable Value Using Bash Scripts? [5 Cases]
- How to Use Default Value in Bash Scripts? [2 Methods]
- How to Use Set – $Variable in Bash Scripts? [2 Examples]
- How to Read Environment Variables in Bash Script? [2 Methods]
- How to Export Environment Variables with Bash? [4 Examples]
<< Go Back to Variable Declaration and Assignment | Bash Variables | Bash Scripting Tutorial