FUNDAMENTALS A Complete Guide for Beginners
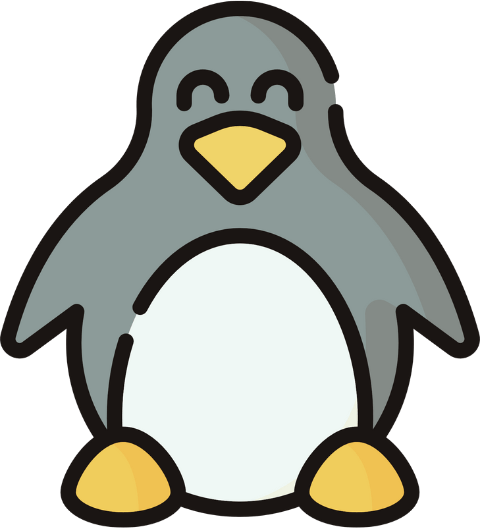
In Bash scripting, handling variables and ensuring they have appropriate values is crucial for writing effective and reliable scripts. One powerful feature that can greatly simplify this task is the use of default values. This article serves as a comprehensive guide to understanding and utilizing default variable value in Bash scripts. Throughout this article, I will explore the syntax, practical examples, and best practices for leveraging how to use default values in Bash scripts. So Let’s Start.
Free Downloads
2 Different Methods to Use Default Value in Bash Scripts
In this article, I will talk about two different methods consisting of ${VARNAME:-default} and ${VARNAME:=default} syntaxes to assign the default value.
You can read our Comparative Analysis of Methods to distinguish between these two methods and choose the best one according to your need. The first method I am going to discuss is ${VARNAME:-default} syntax. Here, I have provided three different case scenarios to understand the concept briefly. When the variable does not contain any value in it, it will take its default value to return the output. Check the steps below to understand it practically. ❶ At first, launch an Ubuntu Terminal. ❷ Write the following command to open a file in Nano: ❸ Copy the script mentioned below: The first line #!/bin/bash specifies the interpreter to use (/bin/bash) for executing the script. Next, It defines the variable VARNAME as an empty string, then uses the ${VARNAME:-LinuxSimply} syntax to assign the value “LinuxSimply” to VARNAME if it is empty. Finally, it displays the value of VARNAME using the echo command. ❹ Press CTRL+O and ENTER to save the file; CTRL+X to exit. ❺ Use the following command to make the file executable: ❻ Run the script by the following command: If you want to assign a default value to a variable only if it is set but not empty, you can also use the ${VARNAME:-default} syntax. Here’s an updated script that demonstrates the case. You can follow the steps of Case 01 of method 01, to save & make the script executable. Script (not_empty.sh) > The first line #!/bin/bash specifies the interpreter to use (/bin/bash) for executing the script.. Then the variable VARNAME contains the value “Ubuntu“. The next line checks if the variable VARNAME is empty or unset. If it is, the default value “LinuxSimply” is assigned to it using the syntax ${VARNAME:-LinuxSimply}. If VARNAME already has a value, it remains unchanged. Finally, the value of the variable VARNAME is displayed using the echo command. Run the script by using the following command: Now if the value is assigned but unset later, the variable will return its default value. You can use the following script to do so. You can follow the steps of Case 01 of method 01, to save & make the script executable. Script (unset_not_empty.sh) > The first line #!/bin/bash specifies the interpreter to use (/bin/bash) for executing the script. Firstly, the variable VARNAME contains the value “Ubuntu“. Then, the unset command unset the value of the variable VARNAME. This means the variable becomes empty. The next line checks if the variable VARNAME is empty or unset. Since it is empty at this point, the default value “LinuxSimply” is assigned to it using the syntax ${VARNAME:-LinuxSimply}. Finally, the value of the variable VARNAME is displayed using the echo command. Run the script by using the following command: There is another method you can use to assign your default value in the Bash script. In Bash scripting, the “${VARNAME:=default}” syntax also allows you to assign a default value to a variable if it is unset or empty. You can follow the steps of Case 01 of method 01, to save & make the script executable. Script (VARNAME:=default.sh) > The first line #!/bin/bash specifies the interpreter to use (/bin/bash) for executing the script. The first echo command displays the value of the variable VARNAME using the syntax ${VARNAME:=LinuxSimply}. The next line assigns a new value “Custom Value” to the variable VARNAME. The second echo command prints the value of VARNAME using the same syntax ${VARNAME:=LinuxSimply}. Then, the unset command is to unset the value of VARNAME, making it empty. The third echo command prints the value of VARNAME using ${VARNAME:=LinuxSimply}. Run the script by using the following command: Here’s a comparison table outlining the pros and cons of the two methods described above:Method 1: Using the “${VARNAME:-default}” Syntax to Assign Default Value
Case 1: If the Variable is Empty
nano empty_var.sh
#!/bin/bash
# Define the variable
VARNAME=""
# Check if the variable is empty and assign a default value
VARNAME="${VARNAME:-LinuxSimply}"
# Display the value of the variable
echo "VARNAME: $VARNAME"
chmod u+x empty_var.sh
./empty_var.sh
As the VARNAME is empty, the echo command returns LinuxSimmply as its default value.
Case 2: If the Variable is Set and Not Empty
#!/bin/bash
# Define the variable
VARNAME="Ubuntu"
# Check if the variable is empty and assign a default value if it is set
VARNAME="${VARNAME:-LinuxSimply}"
# Display the value of the variable
echo "VARNAME: $VARNAME"
./not_empty.sh
As the VARNAME is not empty, the variable return its assigned value Ubuntu instead of the default value LinuxSimply.
Case 3: Variable is Unset and Not Empty
#!/bin/bash
# Define the variable
VARNAME="Ubuntu"
#unset the VARNAME value
unset VARNAME
# Check if the variable is empty and assign a default value if it is set
VARNAME="${VARNAME:-LinuxSimply}"
# Display the value of the variable
echo "VARNAME: $VARNAME"
./unset_not_empty.sh
As the variable VARNAME is unset and thus empty, it returns the default value LinuxSimply in this specific case.
Method 2: Utilizing the “${VARNAME:=default}” Syntax to Assign Default Value
#!/bin/bash
# VARNAME is not set, so it will be assigned the default value "LinuxSimply"
echo "VARNAME: ${VARNAME:=LinuxSimply}"
# Assign a value to VARNAME
VARNAME="Custom Value"
# VARNAME is already set, so its value will be used
echo "VARNAME: ${VARNAME:=LinuxSimply}"
# Unset VARNAME
unset VARNAME
# VARNAME is unset, so it will be assigned the default value "LinuxSimply" again
echo "VARNAME: ${VARNAME:=LinuxSimply}"
./VARNAME:=default.sh
In the first output line, since VARNAME is not set, it returns the default value LinuxSimply. Upon assignment of VARNAME data, it returns its assigned value. Finally, since VARNAME is unset, the default value LinuxSimply is then displayed.
Comparative Analysis of the Methods
Methods
Pros
Cons
Method 1
Method 2
Now the question is which method you should choose to set your default value. Well, there is no specific answer to this question. However, if I want to generalize the statement, the choice between the two syntaxes depends on your specific use case and requirements. If you want to assign a default value without modifying the variable and it is already set, method 1 is preferable. On the other hand, if you want to enforce a default value and ensure VARNAME always has a non-empty value, method 2 is more suitable.
Conclusion
In conclusion, learning to use default values in Bash scripts is a valuable skill that simplifies variable handling and enhances script robustness. I hope this article will work as a user guide to set your default value whenever you work with Bash Script. However, if you have any specific questions related to this article, do not hesitate to comment below. Thank You!
People Also Ask
How do I pass a value to a bash script?
To pass a value to a Bash script, you can use command-line arguments. These arguments are provided when executing the script and can be accessed within the script using special variables. The most commonly used variable is $1, which represents the first command-line argument.
How do I set a default environment variable in Bash?
You can set a default environment variable in Bash by using the ${VARNAME:=default} syntax, where VARNAME is the name of the variable, and default is the default value you want to assign.
How to use set command in a bash script?
You can use the set command in a Bash script to modify shell options, change script behavior, and manage script parameters.
What is set in a bash script?
The set command in Bash script configures shell options and manages script parameters. Moreover, the set command enables you to set specific flags to control the bash script to maintain certain characteristics.
Related Articles
- How to Declare Variable in Bash Scripts? [5 Practical Cases]
- Bash Variable Naming Conventions in Shell Script [6 Rules]
- How to Assign Variables in Bash Script? [8 Practical Cases]
- How to Check Variable Value Using Bash Scripts? [5 Cases]
- How to Use Set – $Variable in Bash Scripts? [2 Examples]
- How to Read Environment Variables in Bash Script? [2 Methods]
- How to Export Environment Variables with Bash? [4 Examples]
<< Go Back to Variable Declaration and Assignment | Bash Variables | Bash Scripting Tutorial