FUNDAMENTALS A Complete Guide for Beginners
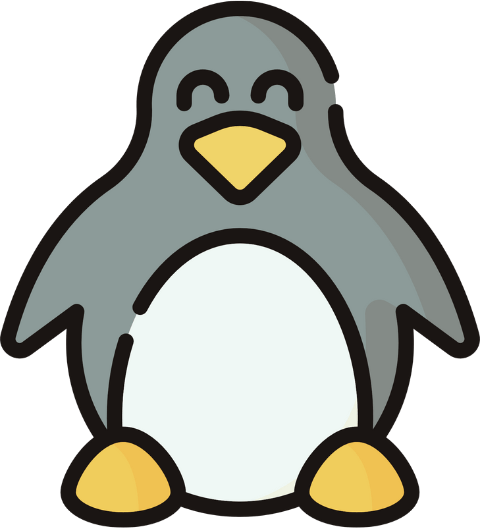
The bash variable holds important data that carries vital information. Sometimes programmers might need to compare bash variables for processing and manipulation of data. In such cases, you need to know some techniques for comparing Bash variables. Some techniques are useful for both string and numeric variables, and some techniques are useful for only numeric variables. In this article, I have explored a bunch of techniques to compare bash variables. I have also included the syntax for these comparison techniques. So without further delay, let’s get started and deep dive into the realm of comparing bash variables.
Key Takeaways
- Knowing syntax for checking equality, inequality, greater than, less than etc comparison.
- Getting familiar with the process of equality, inequality, and greater than comparison.
Free Downloads
Operators to Compare Variable String
Here I have listed some common conditional expressions used for variable comparison with syntax and explanation.
Comparison Operator | Syntax | Explanation |
---|---|---|
is equal to | (( $x == $y )) or -eq | Returns true if both the integers in comparison are equal. |
is not equal to | (( $x != $y )) or -ne | Returns true if both the integers in comparison are not equal. |
is greater than | (( $x > $y )) or -gt | Returns true if x is greater than y. |
is less than | (( $x < $y )) | Returns true if x is less than y. |
is greater than or equal to | (( $x >= $y )) | Returns true if x is greater than or equals to y. |
is less than or equal to | (( $x <= $y )) | Returns true if x is less than or equals to y. |
3 Cases to Compare Variables in Bash Script
Variables store data and sometimes you might need to compare Bash variables to process and manipulate their data. In this section, I have listed some cases of comparing variables. I hope after going through these cases, you will be able to compare variables productively.
Case 01: Compare String Variables Using the ‘==’ Operator or “-eq” Command
You can easily string variables using the “==” operator. If the variable is numerical, you can use the “eq” command in place of the “==” operator. And syntax for using the -eq command is if [[ $variable_1 -eq $variable_2 ]], Here, I will compare two string variables with the “==” operator. To do so, follow the below procedures.
❶ At first, launch an Ubuntu Terminal.
❷ Write the following command to open a file in Nano:
nano script1.sh
- nano: Opens the nano text editor.
- sh: Bash script name.
➌ Copy the script mentioned below:
#!bin/bash
#setting value of name1 and name2 variable
name1="Mark"
name2="Jhon"
#checking the equality of the name1 and name2 variable.
if [ "$name1" == "$name2" ]; then
echo "The names are the same."
else
echo "The names are different."
fi
#! /bin/bash ‘#!’, is called shebang or hashbang. It indicates the interpreter to be used for executing the script, in this case, it’s bash. After that, name1=”Mark” name2=”Jhon” command has assigned value to the name1 and name2 variable. Afterward, if [ “$name1” == “$name2” ]; the command compares the name1 and name2 variables and prints the “The names are the same” message if the comparison result is true otherwise prints the “The names are different.” message on the terminal. Finally, fi denotes the end of the if command.
❹ Press CTRL+O and ENTER to save the file; CTRL+X to exit.
❺ Use the following command to make the file executable:
chmod +x script1.sh
- chmod: Changes the permissions of files and directories.
- +x: Argument with the chmod command to add the executable permission.
- sh: File that you want to make executable.
❻ Run the script by the following command:
./script1.sh
The image shows that the Bash script has compared two string variables and printed a message accordingly.
Case 02: Compare Variables Using the ‘!=’ Operator or the “-ne” Command
The programmers can easily compare two variables using the != operator or -ne command. The syntax for using the -ne command is if [[ $variable_1 -ne $variable_2 ]]. If the variable is a string, the only way is to use the != operator. Here I will develop a Bash script that will utilize the != operator to compare if two numeric variable is not equal or not. To know more, follow the below script.
Script (script2.sh) >
#!/bin/bash
#assigning value to code1 and code2 variable
code1="7845"
code2="9632"
#checking the inequality of the code1 and code2 variable
if [ "$code1" != "$code2" ]; then
#output of the if condition will be true if the two variable is unequal
echo "The codes are different."
else
echo "The codes are the same."
fi
The code1=”7845″ code2=”9632″ command has assigned value to the code1 and code2 variables. Then the if [ “$code1” != “$code2” ]; command has checked the inequality of values of the code1 and code2 variables and returns exit status as true if the values are unequal otherwise false. If the exit status is true, it prints the “The codes are different.” message on the terminal otherwise it prints the “The codes are the same.” message on the terminal. Finally, the fi command denotes the end of the if condition.
Run the script by executing the following command
./script2.sh
The image shows that the Bash script has compared two numeric variables whether they are not equal or equal and printed a message accordingly.
Case 03: Check if a Variable is Greater than Another Variable
You can check whether a variable is greater than other variables simply just using the “>=” operator or “-gt” command. If the variables are string then using >= operator is the only option. Here I have developed a Bash script to check whether a numeric variable is greater than another numeric variable using the -gt command. To know more, follow the below script.
Script (script3.sh) >
!/bin/bash
#assigning values to the x and y variables
x=3
y=1
#checking whether x is greater than y. If true, the comparison will return zero as the exit status
if [[ $x -gt $y ]]
then
echo "x is greater than y!"
else
echo "x less than or equal to y!"
fi
The x=3 y=1 command has assigned values to the x and y variables. After that the if [[ $x -gt $y ]] command has started an if condition where it checks whether x is greater than y. If yes then it returns a zero exit status. And in such conditions, it prints the “x is greater than y!” message on the terminal. Otherwise, it prints the “x less than or equal to y!” message on the terminal. Finally, fi denotes the end of the if condition.
Run the script by executing the following command
./script3.sh
The image shows that the Bash script has checked whether variable x is greater than y or not. And print a message on the terminal accordingly.
Conclusion
Comparing Bash variables is an important task for the programmer as it enables the programmer to compare the valuable data stored inside a variable. In this article, I have tried to explore the various techniques for comparing bash variables and I believe these will be helpful for you to learn the process of comparing bash variables and use it effectively.
People Also Ask
Related Articles
- How to Echo Variables in Bash Script? [4 Practical Examples]
- How to Use String Variables in Bash Script? [4 Cases]
- How to Append String to Bash Variable? [2 Effective Ways]
- How to Check If Bash Variable Exists? [2 Effective Methods]
- How To Check if Bash Variable is Empty? [2 Easy Methods]
- How to List and Set Bash Environment Variables? [3 Methods]
- 2 Ways to Unset Environment Variables Using Bash Script
- 5 Methods to Check If Environment Variable is Set in Bash Script
- How to Set Bash $PATH Variable? [Easiest Configuration]
- 2 Cases to Execute Command Stored in Bash Variable
- How to Store Command Output to Bash Variable? [3 Examples]
- How to Read a File into Bash Variable? [2 Simple Methods]
- How to Write Bash Variable to File? [3 Effective Methods]
- Increment Variable Value in Bash Scripts [4+ Examples]
- Adding 1 to Bash Variable [3 Examples]
- Decrement Variable Value in Bash Scripts [4+ Examples]
- Addition of Bash Variable [4+ Examples]
- How to Subtract Two Bash Variables? [4+ Easy Approaches]
- How to Multiply Variable in Bash [6+ Practical Examples]
- Variable Substitution in Bash [Replace Character & Substring]
<< Go Back to Using Variables in Bash Scripting | Bash Variables | Bash Scripting Tutorial