FUNDAMENTALS A Complete Guide for Beginners
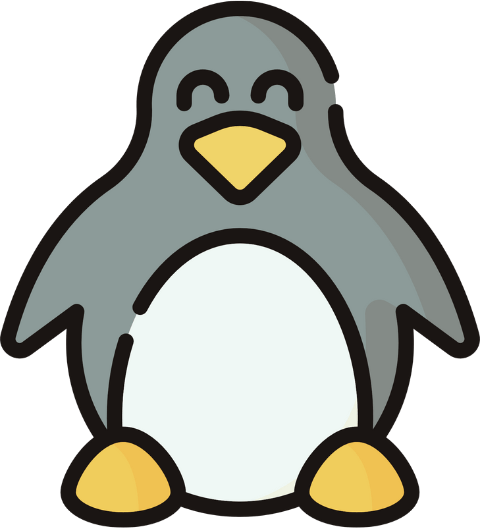
Bash scripts with effective error handling is crucial for reliable and effective process management. So, being aware of the potential errors is a prime concern. Therefore, this guide delves into 4 methods on how to exit Bash scripts with a message to notify the users of failures and decide further actions. In discussing the methods, the article mentions several commands and conditional logic-based approaches to exit scripts with messages for the successful denotation of errors. Moreover, it sheds light on the exiting of piped commands with messages in Bash.
Method 1: Using Logical “OR” Operator
The simplest approach to exit a Bash script with a message is by using the logical OR (||) operator. Using this operator, in between, ensures the printing of an error-denoting message (on the right-hand side) if the command on its left-hand side fails and returns a non-zero exit code.
Check out the example below to exit a Bash script using logical OR (||):
#!/bin/bash
# The command to run and exit with a message if an error
cat "doc.txt" 2> /dev/null || { echo "script exited with error"; exit 1; }
Here, the above Bash script tries to display the contents of the file doc.txt using the cat command. If it succeeds, the contents are simply displayed. Otherwise, the the OR (||) operator comes into action. The echo command prints the message ‘script exited with error” and subsequently exits with code 1 using exit 1
.

Method 2: Using If Statement
The Bash if statement lets users execute commands based on condition checking. This strategy helps check the exit code of an executed command to exit with a message to the user in case of errors.
Look at the example below to exit the Bash script with a message using the if statement:
#!/bin/bash
# 1st command to run
echo "script starts executing"
# check if any error occurs and exit with a message
if [ ! -f "doc.txt" ]; then
echo "Further execution terminated: check script for error"
exit 1
fi
# script continues if no error
cat "doc.txt"
echo " script ends with success"
Here, the above script starts with printing a message using the echo command. Then if [ ! -f "doc.txt" ]
checks if doc.txt exists in the system. If False, then it prints a message that denotes that the script has encountered an error. Finally, it exits with exit 1
, and the rest of the script is not executed (printing file contents using the cat command and displaying a message of success). Otherwise, the remaining part successfully executes.
Here, since the file “doc.txt” does not exist, the script exits with a message indicating an error condition to the user. Subsequently, it tells users to check for those errors within the same message.
Method 3: Using “trap” and “exit” Command
The trap command catches different signal handlers and determines action based on the signal type. One instance would be to catch any error condition and execute codes to let scripts exit with messages with the Bash exit command.
Here’s an example of how to exit with a message on error using the trap and exit commands:
#!/bin/bash
#function to print exit on error message
print_error() {
echo "script exited unexpectedly with error. Please check the script."
exit 1
}
# Trap the ERR signal and call print_error function to exit with message
trap print_error ERR
# the script commands to run that may fail
cat "landingPage.js" 2> /dev/null
# script executes if no further error
echo "Script continued to execute!"
The script first defines a function named print_error that denotes the error condition using a message and lets the script exit with code 1. Then, it sets the trap command using the code trap print_error ERR
. This catches the error signal (if occurs in execution of commands) and invokes the “print_error” function. Finally, if commands encounter errors, the script exits with the message invoked by “print_error”, while the remaining commands are not executed.
Here, the script exits with an error-conveying message since the command cat fails (the file landingPage.js does not exist). Otherwise, it would have run successfully printing the content of the file (if it existed).
Method 4: Using “trap” and “set -e” Command
The set command with the -e option lets a Bash script exit immediately as soon as it encounters any error. So, it hinders any further execution of the script. Now, smartly using it with the trap command allows for the seamless exit of scripts under any failure along with displaying messages to alert the users of such encounters. Here’s how:
#!/bin/bash
# set command to terminate script on error immediately
set -e
#function to print exit on error message
message_on_error() {
echo "An error occurred with exit code $?. Please check the script."
}
# Trap the ERR signal and call message_ on_error
trap message_on_error ERR
# The script commands to run
Cat "doc.txt" 2> /dev/null
# script executes if no further error
echo "Script completed successfully!"
Here, the script first defines the “set” command and the trap command to catch errors. Then it defines a function message_on_error that prints a message indicating the error condition ( if occurs). So, the script runs the command Cat "doc.txt" 2> /dev/null
and suppresses any error message to /dev/null. Subsequently, the “Cat” command fails (because it’s cat not Cat), and the trap catches the ERR (error) signal. This calls the message_on_error function to print the error message and set -e lets the script exit immediately hindering any further execution. Otherwise, the script would have executed all the commands successfully.
Here, since the Cat command (it should be cat) fails, the Bash script above exits with the message defined in the message_on_error function.
Exiting of Pipelines With Message in Bash
A pipeline chains two or more commands to run together which is also termed as piped commands in Bash. Such piping in Bash can occasionally introduce complexity. So, exiting with an error denoting a message is a crucial step in working with complex processes such as pipelines. So, look at the below script that uses the if statement to make Bash pipelines exit with a message:
#!/bin/bash
# message to alert the script's beginning
echo "script starts.."
echo
# Run the piped commands
echo "hello" | GREP "hello" 2> /dev/null
# Check if any command in the pipeline failed and exit with a message
if [ "${PIPESTATUS[*]}" != "0 0" ]; then
# Print an error message
echo "Error: pipeline failed...check script"
exit 1
fi
# Continue if all commands succeeded
echo "Success: All commands in the pipeline executed successfully"
First of all, the above script prints a message denoting its beginning of execution. Secondly, it attempts to run a pipeline and suppresses any error to /dev/null. Then it checks if any of the exit codes of the piped commands is non-zero using the condition if [ "${PIPESTATUS[*]}" != "0 0" ]
. If yes, then the script prints the message “Error: pipeline failed…check script” and exits with code 1. Otherwise, it executes the remaining commands. That’s how the script exits with a message denoting failure.
So, in the above, the command GREP fails (since it is grep) after the successful beginning of the script. Thus, it exits by printing the appropriate message seen on the screen.
Conversely, if the “grep” command executes successfully, then the script continues its execution like the below image:
Note: The PIPESTATUS variable used above is a Bash array storing the individual exit codes of each command in a pipeline. To know more about PIPESTATUS, read this article: “Bash Pipestatus to Handle Exit Codes of Piped Commands”
Conclusion
The above article discusses how to exit a Bash script with a message to the user employing 4 effective methods. It has mentioned several methods that incorporate commands like trap, set, etc. Additionally, it explains the use of conditional statements to exit scripts with messages in Bash delving into examples using both single and piped commands.
Frequently Asked Questions
How to exit a script immediately after encountering an error condition in Bash?
To exit a Bash script immediately after encountering an error, use the command set -e. When it is enabled using “set -e”, it ensures that the script exits as soon as any command returns a non-zero exit code.
How to exit a script in Bash?
To exit a script in Bash, use the “exit” command followed by the exit code after defining the command or stream of commands you want to execute. The full syntax is exit N where N is the exit code status. For instance, if you run the command echo "hello"
and add the line exit 0
in the next line, the script will execute the “echo” command and terminate its execution with code 0. This will also stop the further execution of the script.
Can I suppress error messages to print on the screen?
Yes, you can. To suppress any error message, just add 2> /dev/null next to the command you intend to execute. For instance, echo "hello world" 2> /dev/null
. This will ensure that the error message (if any) will not be displayed on the screen. For example, if the command cp command fails to copy a file or folder, the regular error message will not be printed on the screen.
What does it mean by /dev/null?
The /dev/null is a virtual null device that discards the output of a command or script. So, anything redirected to this file will simply vanish. For example, if you run the command echo "hello"
, it will print hello in the terminal. However, if it’s redirected by using echo "hello" > /dev/null
, then the string hello will not be displayed on the screen.
Related Articles
- Usage of “exit” Command in Bash [Explained]
- How to Set Exit Codes in Bash? [An Easy Guide]
- How to Set & Use Pipefail in Bash [Explained Guide]
- Bash PIPESTATUS to Handle Exit Codes of Piped Commands
- [4 Methods] How to Check Exit Code in Bash?
- [Explained] What Are “exit 0” and “exit 1” in Bash?
<< Go Back to Exit Codes in Bash | Bash Process and Signal Handling | Bash Scripting Tutorial