FUNDAMENTALS A Complete Guide for Beginners
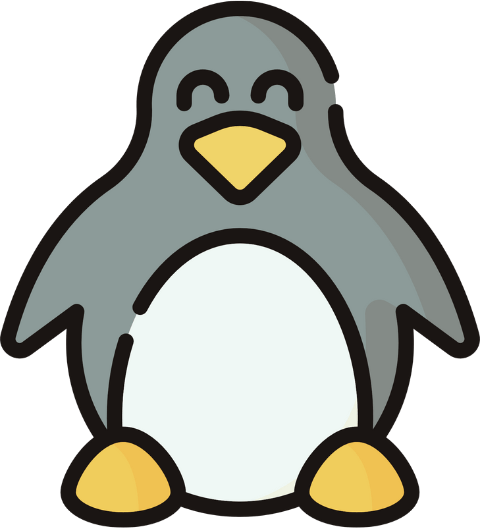
While working with Bash scripts, sometimes it may be necessary to terminate the script that provides a smooth way to error handling and flow controlling of scripts. So, knowing about the Bash exit command is vital for developing well-written scripts since it terminates the script and returns a status code for seamless control over its execution.
This guide will aid your understanding of the Bash exit command by discussing its basic to practical use cases with several examples within Bash scripts.
What is Bash “exit” Command?
The exit command is a built-in Bash command that smoothly terminates the execution of a Bash script and returns a numeric value to the parent script. Additionally, when used with an exit code, it signals the end of the script’s execution by returning the exit code status to the calling process and indicates the success or any error condition encountered by the script. Moreover, the exit command can also terminate the current shell session.
Generally, the “exit” command is used with an exit status code of N to exit the script execution. The syntax is very straightforward as follows:
exit N
Here, N is a numeric value (0-255) passed to the exit command as an argument that the script returns as an exit code to the shell. However, this is optional and when this code is not specified, the exit command displays the code returned by the last executed command. You can use the code echo $?
to display the exit code where $? is a special variable storing the exit code.
Below is a simple script to show the basic use of the exit command in Bash to terminate script execution:
#!/bin/bash
echo "Hello, from,Linuxsimply!"
exit 0
The script above prints the message “Hello, from,Linuxsimply!” using the echo command and then terminates the script with an exit status code of 0. The exit 0
command indicates the success of the script’s execution.
4 Examples to Use the “exit” Command in Bash
This section will mention 4 examples to display the Bash exit command in action. The examples will revolve around basic script termination to defining your custom Bash exit codes employing the exit command for achieving better control flow and execution of Bash scripts.
1. Terminating Bash Scripts
The exit command simply terminates the execution of a Bash script and returns the exit code status value if specified to the calling process. Below is an example of terminating a script using the exit command with the exit code 0 using the syntax exit 0
:
#!/bin/bash
echo "this line is printed on the screen"
exit 0
echo "this will not be printed"
The script above first prints “this line is printed on the screen” and then exits the script execution with an exit code of 0 using exit 0
. Since this immediately terminates the script, the last line is not printed to the prompt. The running of the echo $?
Also verifies the value of the exit code.
#!/bin/bash
echo "this line is printed on the screen"
exit
echo "this will not be printed"
So, you can see that the exit command alone is enough, and here the exit code is 0 since the script runs successfully before the exit command terminates the script preventing the printing of the last line.
2. Denoting Success or Failure in Bash Scripts
Another basic use of the exit command, apart from script termination, is to return exit status codes after execution to denote whether the script runs successfully. This leverages efficient error handling and debugging of processes.
Here’s an example of using the exit command to denote the success or failure of scripts in Bash:
#!/bin/bash
# Prompt the user to enter a file name
echo "Enter the file name:"
read file_name
# Check if the file exists
if [ -f "$file_name" ]; then
echo "File '$file_name' exists"
exit 0
else
echo "File '$file_name' does not exist"
exit 1
fi
In the above, the script takes a file name as input from the user and then checks if the file exists using if-else conditional statements. If the file exists, it prints the line of the if scope and terminates the script immediately with the exit 0
command, where code 0 denotes the successor of the script in finding the file. Otherwise, the else is executed and the script is terminated with exit 1
, denoting an error condition of file not found.
echo $?
command.
3. Controlling the Execution Flow of Scripts in Bash
The exit command lets smooth control over the execution flow of a Bash script based on the return code. Here’s how:
#!/bin/bash
#check if a user is root to control the script execution flow
if [[ "$(whoami)" != root ]]; then
echo "Only user root can run this script."
exit 1
fi
echo "hello world"
exit 0
The above script checks if the user is the root user or not employing the conditional statement if [[ "$(whoami)" != root ]]
and terminates with exit code 1 if the user is not the root user using exit 1
. Contrarily, if the user is root, then the “echo” command prints the line hello world on the screen and exits with 0 denoting success. This is how it controls the flow of the script and executes commands.
4. Using Custom Exit Codes with the “exit” Command
In using the exit codes with the exit command to terminate scripts, typically users adopt the codes according to their usual meanings. For instance, the exit 0
command terminates the script indicating success. However, it is possible to define a custom exit code for process termination like the following example:
#!/bin/bash
echo "enter username"
read username
echo "hello, $username"
exit 127
echo "the script ends here...."
The above Bash script takes a username as input from the user with the read command and stores it in the username variable. Then prints the username with a message using the “echo” command and exits successfully with the exit 127
command where code 127 denotes success instead of the conventional success code 0 and skips the last line from execution.
3 Common Pitfalls in Working with Bash “exit” Command
This section discusses 3 common pitfalls one may encounter in working the Bash exit command. Let’s explore them one by one with possible solutions:
1. Problems with Exit Statuses
Sometimes the exit status codes used with the exit command can be misleading. For instance, the script might return the code 0, indicating success, even if there’s an error. Look at the following example:
#!/bin/bash
# This script has a failing command but still exits with status 0
non_existent_command
echo "This is a script"
exit 0
In the script, the non_existent_command fails, but the echo command succeeds and the script exits with code 0, indicating success. However, it would have been better if the script immediately exited after encountering the “non_existent_command” leveraging effective error handling.
Solution: To avoid such errors, it is recommended to use exit codes and the exit command carefully. However, one solution is to use the set -e
command at the beginning of the script. This will let the script exit immediately after getting failures. Here’s how:
#!/bin/bash
# script will exit since there is non-existent command
set -e
non_existent_command
echo "This is a script"
The above script uses the set -e
command at the beginning and just after encountering the command not found an error in executing non_existent_command, the script immediately exits and the rest is not executed. Moreover, the echo $?
command returns code 127 on the screen and verifies the error.
2. Unexpected Termination of Scripts
Sometimes the use of the exit command terminates the script unexpectedly. This causes if the user is unaware of when and where to use the exit command. Let’s see an example:
#!/bin/bash
echo "script starts"
if [ -f /etc/passwd ]; then
echo 'File exists'
exit 0
fi
echo "script ends"
In the above, after printing the 1st line and checking the condition, the script exits due to the presence of the exit 0
command. Thus, the last line of the code is not executed though it seems a relevant part of the code.
Solution: The solution to this problem is simple. Carefully use the exit command only where necessary with full knowledge of its outcome.
3. “Exit: command not found” Error
One common mistake the scriptwriters make is to incorrectly capitalize the exit command which leads to errors and unexpected behaviors. Here’s an example:
#!/bin/bash
# print the current directory
pwd
# exiting the script with Exit 0 instead of exit 0
Exit 0
echo " script ends"
In the above, using “Exit 0” instead of the exit 0 command leads to an error of the “Exit: command not found” like the following image:Solution: To avoid such errors, always pay attention to the correct syntax and double-check before code execution.
Conclusion
This article has discussed the concepts of the exit command in Bash which is an essential part of well-written scripts. It has mentioned the exit command from basics to its common practical use with 4 examples. Moreover, it has mentioned 3 common pitfalls while working with the Bash exit command with solutions. So, this article will leverage your mastering the exit command in Bash. Additionally, it will help you write robust code with efficient handling of errors and control flow within scripts
People Also Ask
What does the exit command do in Bash?
The Bash exit command indicates the termination of a script’s execution immediately. It denotes when the script should exit. Moreover, it alerts if the script is executed successfully or encounters an error condition. When used with an exit status code, the script exits with that specific exit status. Without exit status, the script exits with the exit code of the last executed command in the script.
What is the difference between the special variable $? and the Exit command in Bash?
The $? (dollar question mark) is a special variable that stores the exit code status of the last executed command or process. It checks for the command or process’s success or failure, such as the Bash script.
On the other hand, the exit command explicitly leverages the termination of a script or current shell session. It can be used with an optional exit status code in the format exit [exit code] or without mentioning any custom code. This lets the command terminate the execution of a script to denote its success or failure.
What is the exit code at the end of a Bash script?
The exit code at the end of a Bash script is the exit code of the last executed command. Such code is assigned when the script finishes its execution. For instance, if the last command executes successfully, the exit code at the end of the script would be 0. Otherwise, the script returns a nonzero exit code depending on the error condition.
What is the difference between the “exit” and “return” commands?
The exit command is used to terminate the execution of a script or current shell session. When the script encounters the exit command, it immediately stops execution. Moreover, it returns the exit status to the calling process. Contrarily, the return command is used to exit from a Bash function. When a function encounters the return command, it exits the function and passes the specified value to the caller.
Can we exit from a Bash script using the return command?
No. We cannot exit from a Bash script using the return command. This is because it is used to exit from a Bash function; not a Bash script. To exit from a Bash script, you can use the exit
command either alone or with an exit status code depending on the success or error condition of the script.
What do exit 0, exit 1, and exit 2 mean in a Bash script?
exit 0
, exit 1
, and exit 2
are commands returning the exit status of a Bash script denoting success or failure. For example, the command exit 0 typically means the script has been executed successfully. On the other hand, the exit 1 command denotes errors in a Bash script. This usually occurs when an incorrect argument is passed to a command or it misses any argument. In addition, the command exit 2 denotes the error condition encountered by the script. For example, when the command used within the script cannot find the targeted file, it returns exit code 2.
Related Articles
- 4 Methods to Exit Bash Scripts on Error with Message
- How to Set Exit Codes in Bash? [An Easy Guide]
- How to Set & Use Pipefail in Bash [Explained Guide]
- Bash PIPESTATUS to Handle Exit Codes of Piped Commands
- [4 Methods] How to Check Exit Code in Bash?
- [Explained] What Are “exit 0” and “exit 1” in Bash?
<< Go Back to Exit Codes in Bash | Bash Process and Signal Handling | Bash Scripting Tutorial