FUNDAMENTALS A Complete Guide for Beginners
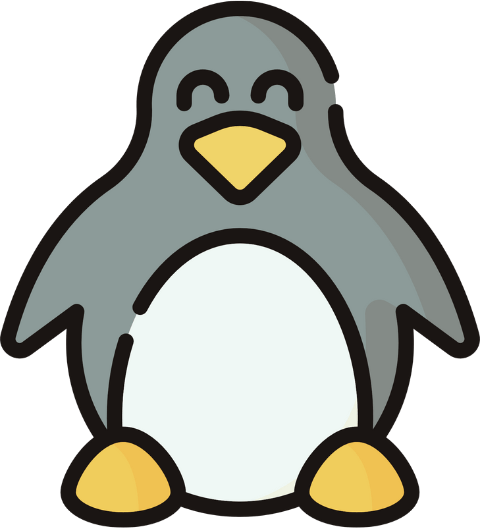
Exit codes are pivotal elements for the successful handling of command-line operations and Bash scripts with effective error handling, debugging, and script control flow–providing success or failure status of the commands or scripts. This guide will provide a comprehensive view of Bash exit codes discussing their basics, and usage in practical scenarios. It will shed light on several frequently encountered exit codes with meanings for successful adoption in command operations and Bash scripts.
What is an Exit Code in Bash?
Exit code, also called “ exit status” or “return code” is an integer value from 0-255 used by a command or script to indicate its success or failure during execution. It typically denotes whether a program has run successfully or has encountered any error condition or failure. Exit code is a traditional way of handling errors in Bash in the execution of scripts or commands in the terminal.
When a program finishes running, it returns an exit code to the calling process to indicate success or failure for further decision-making. For example, the exit code 0 typically means that the last executed command or script has run successfully while the exit status of 1 or any non-zero numerical value means that the process has encountered an error condition.
How to Get Bash Exit Codes Using the Terminal?
To get the exit code after executing a command, use the special variable $? (the dollar question mark variable) which holds the exit code of the last executed command by default or the most recently executed process. Using the echo command within the syntax echo $?
it displays the exit code on the terminal.
Get Exit Codes Using Bash Scripts
Alternatively, you can find the exit codes using Bash scripts after executing commands within the scripts employing echo $?
. Here’s a simple example:
#!/bin/bash
#the command
date
#the exit code of the date command
echo "the exit code of date: $?"
# the command to run again
cp
#exit code
echo "the exit code for cp: $?"
The above script first runs the date command and prints the exit code using echo $?. The value of 0 denotes the successful execution of the date command. In addition, after running the cp command, the exit code is returned as 1 which indicates that the cp command is missing arguments. That’s how it is possible to get the exit codes of the executed command using Bash scripts.
Common Bash Exit Codes with Meaning
The table below shows 7 commonly encountered exit codes along with mentioning their meanings and typical examples of where one might encounter such codes:
Exit code | Meaning | Examples |
---|---|---|
0 | Success of execution | |
1 | Generic errors | “Permission denied”
“Divide by zero” “Missing operand” |
2 | Improper command usage | “Missing keyword”
“No such file or directory” |
126 | Unable to execute the command | Permission issue |
127 | Problem in path resolution | Command not found |
130 | Fatal error signal | When interrupted by CTRL+C |
255 | Exit status out of range |
7 Examples to Use Exit Codes on the Command Line
This section will shed light on 7 examples to show how to use the exit codes on the command line. These examples will cover the most common exit codes (0, 1, 2, 126, 127, 130, 255) with their basic meanings and command-specific manipulations.
Example 1: Exit Code 0
The typical exit code after the successful run of a command is zero (0). For example, run the following command in the terminal:
whoami
In the above image, the command echo $? returns 0 since the whoami command runs with success (returns the current user name).
Example 2: Exit Code 1
As stated in the table above, the exit code 1 signifies generic errors such as missing operands. To see this in action, execute the command below:
mv
Since the mv command expects arguments, the exit code 1 is returned by the echo $?
command after running only the mv command like the following image:In addition, one example is to divide a number by zero which leads to the exit code being 1 due to the divide by zero generic error. Below is an example specific snapshot for clarity:
Example 3: Exit Code 2
The exit code 2 denotes failure such as the “no such file or directory error” when a user attempts to access a file or directory that is not present in the system. For instance, to list the items of a directory named players that does not exist, run the following command:
ls players
The image states that listing the players directory contents throws an error which is evident as the exit code is 2.
Example 4: Exit Code 126
When the system encounters an issue related to permission, Bash returns an exit code of 126.
Run the following command in the terminal to create a script named file.sh:
touch file.sh
As you can see, in executing the touch.sh script without permission, it threw a “permission denied” error with the exit code 126.
Example 5: Exit Code 127
The exit code 127 is returned by the system if the command to be executed doesn’t exist or is even not present in the PATH environment variable. For example, you can run the below line of code on the terminal to get a hands-on illustration:
yuki
Here, you see that the system returns exit code 127 since the command named yuki doesn’t exist.
Example 6: Exit Code 130
If any command or process execution is terminated by pressing CTRL+C, then the system returns exit code 130. Here’s an example:
The image states that the code_130.sh script should have taken a name as input by the read command. However, when the script execution is terminated by pressing CTRL+C, the printing of the exit code returns 130 due to the fatal error of process termination.
Example 7: Exit Code 255
The exit code of 255 also refers to an error condition but the nature of the error varies on the context in which it occurs. To produce the exit code 255, you can run the following command:
ssh
Here, the exit code 255 signifies that the
ssh
command encounters an error when it attempts to establish a connection with the local host as no argument (hostname) is passed to it.
How to Use Exit Codes in Bash Scripts
In Bash scripting, one can utilize exit codes to determine whether a set of commands has been executed successfully or encountered issues. These codes can be employed directly or stored in a variable for later evaluation.
Below is an example script to use exit codes directly:
#!/bin/bash
# command to be run
date
# exit status
echo "the exit code is: $?"
# command-2 to be run
mkdir
# exit status
echo "the exit code is: $?"
The above script takes two commands (date and mkdir) to execute and also uses the command echo $? to get the respective exit statuses. After executing the script, the date command returns 0 since it runs successfully within the script. However, the mkdir command has no arguments; so it is executed but with the missing operand error. That’s why its exit status is 1.
1. Control Bash Script Flow Using Exit Codes
The exit codes are very useful in Bash scripts to control the flow of execution depending on the success or failure of the previous command. This can be done easily by using if-else conditional statements and exit codes as conditions. Here’s how:
#!/bin/bash
# command to run
cd polash
#storing the exit code for cd in a shell variable
status=$?
#perform further action based on the exit code
if [ $status -eq 0 ] ; then
echo "directory remains"
else
echo "no directory in existence"
The above script uses the command cd polash
to change the directory to polash and stores the exit code in the status variable using the special variable $?. Then it decides to proceed further or not by checking the existence of the polash directory using the if conditionals with exit code value as a condition and selects to execute either the if block or the else block. This is how the above script controls its execution flow using exit codes.
2. Check if a User is Valid Using Exit Codes
You can make use of Bash exit codes to check if a user exists in the system as a valid user or not. See the below scripting example to see exit codes in action to check whether a user is valid:
#!/bin/bash
#taking a user name as input
echo "Enter a username"
read username
# fetching the usename from the /etc/passwd file
entry=$(cat /etc/passwd | grep $username)
#storing the exit code
user_exist=$?
#check if user is valid and switch user using exit code
if [ $user_exist -eq 0 ]
then
echo "Valid user"
su $username
else
echo "Invalid user"
fi
The script above prompts the user to enter a username, then searches for that username in the /etc/passwd file. If the username is found, it prints “Valid user” and attempts to switch to that user using the su command and with the provided user password. If the username is not found, it prints “Invalid user”.
For instance, in the image below, the script prompts the user to enter a password to switch to user rafid since the user is valid.On the other hand, the following snapshot states that the username yuri is not present in the system and thus the message of the else block is displayed on the terminal.
Custom Exit Codes in Bash
In working with Bash scripts, you can also define your exit codes to control the execution flow of the script. To do so, use the Bash exit command with the status code afterward. The syntax is exit [n] where n is the custom exit code you want to assign. Here’s an example:
#!/bin/bash
if [ -f /path/to/somefile ]; then
echo "File found"
exit 0
else
echo "File not found"
exit 1
fi
The above script checks if a file exists at the specified path /path/to/somefile. If the file is found, it prints “File found” to the standard output and exits with a custom exit code of 0, indicating success. If the file is not found, it prints “File not found” to the standard output and exits with a custom exit code of 1, indicating failure. That’s how the exit command with the custom exit codes denotes success or failure.
How to Use Logical Operators with Exit Codes in Bash
The logical operators (and) && and || (or) can be used with exit codes to control the execution flow of a process. For example, open the terminal and run the following command to check the validity of the user sowad using exit codes and logical operators:
cat /etc/passwd | grep sowad && su sowad || echo "invalid user"
The above image states that the user sowad is valid (returns exit code 0) and the system uses the su command to switch the user to “sowad” with the use of the && (and) logical operator.
On the other hand, the system will print “invalid user” using logical OR like the below image since the user yuki is not present in the system:
Bash Exit Codes in Piped Commands
Sometimes handling exit codes in piped commands may become necessary in Bash. In the case of the piped commands, the exit code of the entire pipeline is the exit code of the last executed command. Below is an example command to run on the terminal for exploration:
ls no_directory | wc -l
In the above case, the ls no_directory
command fails, still the exit code is 0 due to the successful run of the wc -l
command.
In addition, the PIPESTATUS array is useful in working with piped commands in Bash since it stores the exit codes of all the commands used in the pipeline. For example, to check for the exit code of the first command (ls no_directory) that failed in the previous pipeline, use the below code:
echo ${PIPESTATUS[0]}
Conclusion
This article discusses exit codes in Bash that play an integral part in command-line operations or scripts by facilitating error handling, debugging, conditional execution, etc. It has mentioned some common exit codes with their usual meanings. It also discusses multiple examples to provide a clearer view of the exit code concept for both command and scripting-based scenarios.
People Also Ask
What does the exit code do in Bash?
The exit code in Bash notifies if a command or script has run with success or not. It is a numerical value returned by Bash for any command executed in the terminal. The value can be either zero or non-zero to indicate success or any failure after execution. For instance, a command or script which typically succeeds in execution, has an exit code of zero. On the other hand, any non-zero exit code (1-255) indicates failure.
What does $? mean in Bash?
The $? mark (dollar question mark) is a special variable that holds the exit code or exit status of the last executed command. It is a specific shell variable reserved to find out if the command has been executed with success or not. You can use the command echo $?
to print the code held by $? on the screen.
How to check the exit code of the last executed command?
To check the exit code of the last executed command, open the terminal and run the command echo $?
. This returns the proper exit status of the very last command you executed.
What does the exit code 0 mean in Bash?
The exit code 0 in Bash means the successful execution of a command or a script. It signifies that the specified command or script has run but without errors. This you can find by running the command echo $?. For instance, after running the command whoami
, you can run the command echo $?
to get 0 and ensure that it runs with success
How do exit code 0 and exit code 1 differ in Bash?
Exit code 0 and exit code 1 differ primarily in what they indicate after the command or script execution. For example, exit code 0 signifies a successful execution. When a command or script runs without encountering errors, the exit code 0 is returned. On the other hand, exit code 1 is returned when the command fails to execute properly denoting the error condition.
Can I find the exit status of a command?
Yes, you can find the exit status of a command. To find the exit status of a command, use the special variable $? With the echo command after execution. The full syntax is echo $?. For example, after running the pwd
command in the terminal, you can use the command echo $?
to find the exit status of pwd. This will return a numeric value to indicate whether the “pwd” command has run with success or encountered errors.
Do the terms “exit code” and “exit status” refer to the same meaning in Bash?
Yes. The terms “exit code” and “exit status” typically refer to the same concept. Both terms are used interchangeably to denote a numerical value returned by a command when it terminates. This, in turn, indicates if the command has run successfully or has encountered any errors while executing. Typically the exit code 0 indicates success while a non-zero value indicates an error condition or failure.
Does an exit code display the output of a command?
No. The exit codes do not display the output of a command. It’s a numerical value returned by a command upon its completion to indicate that the command has been executed with success or encountered an error. The code is accessed by $? in Bash and you can run the command echo $? to display the code on the terminal.
Is it possible to store the exit status of a command to a shell variable?
Yes, it is possible to store the exit status of a command to a shell variable. To do so, assign the exit status to a shell variable by using the syntax variable_name=value. For example, after running the ls
command, you can use the code status=$?
to store the exit status or exit code of the ls command in the status variable.
Are there any alternative methods to handle exit codes in Bash?
Yes. There are commands like set -e
and trap that are useful tools to alternatively handle exit codes in Bash.
Related Articles
- 4 Methods to Exit Bash Scripts on Error with Message
- Usage of “exit” Command in Bash [Explained]
- How to Set Exit Codes in Bash? [An Easy Guide]
- How to Set & Use Pipefail in Bash [Explained Guide]
- Bash PIPESTATUS to Handle Exit Codes of Piped Commands
- [4 Methods] How to Check Exit Code in Bash?
- [Explained] What Are “exit 0” and “exit 1” in Bash?
<< Go Back to Bash Process and Signal Handling | Bash Scripting Tutorial