FUNDAMENTALS A Complete Guide for Beginners
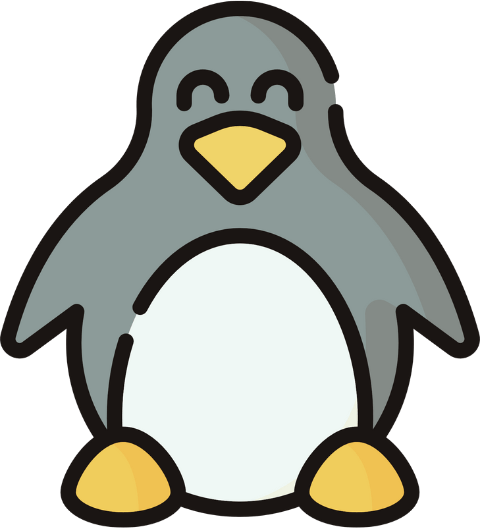
Parameters are essential for passing information to functions, allowing them to perform specific tasks with different inputs. The article will discuss the syntax for defining Bash functions and demonstrate how to use parameters with these functions. It will also illustrate various examples of using and manipulating these parameters within the function’s body, providing you with a comprehensive understanding of how to effectively utilize parameters in Bash scripting.
Parameters in Bash Scripting
A Bash parameter refers to a value or information supplied to a Bash script or function during execution. These parameters enable scripts to accept inputs, enhancing their flexibility, adaptability, and user-friendliness.
In Bash scripting, parameters come in various types: positional parameters, special parameters, and named parameters (options and flags). etc. These diverse parameter types enable scripts to receive and process information, configure behavior, and interact with the system and users effectively. However, to learn more about the bash parameters, you can follow our article, Parameters in Bash scripting, on the website.
Functions in Bash Scripting
Functions in Bash scripting are blocks of reusable code that perform specific tasks. They play a crucial role in making your scripts modular, organized, and easier to maintain. Functions allow you to combine a series of commands and execute them by simply calling the function name.
A. Syntax For Creating
Creating a Bash function follows a straightforward syntax. You have the option to define a function using either the explicit function keyword or the shorthand notation. Here is the syntax of the function using the function keyword:
function function_name() {
# Function code here
}
Alternatively, you can use the shorthand notation:
function_name() {
# Function code here
}
B. Naming Conventions
Bash functions follow a naming convention similar to variable names. Here are the naming conventions for Bash functions:
- Function names should consist of letters, digits, or underscores.
- They must start with a letter or underscore.
- Function names are case–sensitive, so myFunction and myfunction would be considered different functions.
- Choose descriptive and meaningful names for your functions that indicate their purpose or functionality.
- It’s best to avoid using Bash keywords (e.g., if, while, for) as function names to prevent confusion and potential conflicts with the Bash language.
- Use lowercase letters for function names, separating words with underscores for improved readability (e.g., calculate_average, process_data).
C. Calling a Bash Function
To call a Bash function, you simply need to use its name followed by parentheses, like this:
function_name
This will execute the code within the function. If the function accepts arguments, you can pass them within the parentheses like this:
function_name arg1 arg2
The function will then receive these arguments as positional parameters, accessible within the function as $1, $2, and so on.
D. Passing Arguments
To pass arguments to a Bash function, you include them within the parentheses when calling the function. These arguments are then accessible within the function as positional parameters, denoted as $1, $2, $3, and so on. Here’s an example:
my_function() {
echo "First argument: $1"
echo "Second argument: $2"
}
# Calling the function with arguments
my_function arg1 arg2
In this example, when you call my_function with arg1 and arg2, within the function, $1 will hold the value arg1, and $2 will hold the value arg2. You can access and manipulate these arguments as needed within your function’s code.
E. Returning Values
Returning values from a Bash function enables you to generate output based on input parameters. In Bash, you can achieve this by utilizing the return keyword. Here’s a breakdown of the process with a code:
#!/bin/bash
function calculate_sum {
local result=$(( $1 + $2 ))
return $result
}
result=$(calculate_sum 3 5)
echo "The calculated result is $result"
In this example, I establish a Bash function called calculate_sum that accepts two parameters, a and b. Within the function, I utilize the $1 and $2 references to access the values of the first and second parameters, respectively. I perform the addition operation and save the result in a local variable named result.
Subsequently, I use the return keyword to send the value of the result back from the function. When I call the calculate_sum function with arguments 3 and 5, the returned value is captured in the result variable, which is then displayed on the console.
6 Practical Examples of Using Parameters in Bash Function
Bash scripting can be made more efficient with the help of functions that take inputs, known as parameters. In this article, I’ll dive into six practical examples that illustrate how to use parameters in Bash functions. These examples will show you how to create flexible and powerful scripts for everyday tasks.
Example 01: Creating Functions With Arguments in Bash Script
As described previously, to craft a Bash function that accepts arguments, you first define the function and then access these arguments using special variables like $1, $2, $3, and so forth. These variables correspond to the positional arguments supplied when calling the function.
Here, I am going to give an illustration of a basic Bash function that employs arguments:
- At first, launch an Ubuntu Terminal.
- Write the following command to open a file in Nano:
nano function_greeting.sh
EXPLANATION- nano: Opens a file in the Nano text editor.
- function_greeting.sh: Name of the file.
- Copy the script mentioned below:
#!/bin/bash # Define a function named "greet" that takes a name as an argument function greet() { local name="$1" echo "Hello, $name!" } # Call the "greet" function with the name "LinuxSimply" greet "LinuxSimply"
EXPLANATIONThis Bash script defines a function called greet that accepts a name as an argument. Inside the function, it assigns the argument to a local variable named name and then echoes a greeting message using that name. Finally, it calls the greet function with the argument LinuxSimply resulting in the output: “Hello, LinuxSimply!“
- Press CTRL+O and ENTER to save the file; CTRL+X to exit.
- Use the following command to make the file executable:
chmod u+x function_greeting.sh
EXPLANATION- chmod: Changes the permissions of files and directories.
- u+x: Here, u refers to the “user” or the owner of the file and +x specifies the permission being added, in this case, the “execute” permission. When u+x is added to the file permissions, it grants the user (owner) permission to execute (run) the file.
- function_greeting.sh: File name.
- Run the script by the following command:
./function_greeting.sh
As the image suggests, the function_greeting.sh successfully returns the intended string “Hello, LinuxSimply” to the command line.
Example 02: Pass Multiple Arguments to the Bash Function
Passing multiple arguments to a Bash function is useful when you need to provide the function with different input values that are related to the same operation or task. In contrast to the previous section, this section involves passing multiple parameters instead of a single argument. To illustrate it further, suppose you want to create a Bash function called calculate_sum that calculates the summation of multiple numbers. Check the below code to understand the concept fully.
You can follow the steps of Example 01, to save & make the script executable.
Script (multiple_arguments.sh) >
#!/bin/bash
# Define a function named "calculate_sum" to add two numbers
function calculate_sum {
local num1=$1
local num2=$2
# Check that both arguments are numbers
if ! [[ "$num1" =~ ^[0-9]+$ ]]; then
echo "Error: Argument 1 is not a number"
exit 1
fi
if ! [[ "$num2" =~ ^[0-9]+$ ]]; then
echo "Error: Argument 2 is not a number"
exit 1
fi
local sum=$(( num1 + num2 ))
echo $sum
}
# Call the "calculate_sum" function with two arguments
result=$(calculate_sum 10 20)
echo "The result is: $result"
In this Bash script, the function named calculate_sum adds two numbers. Inside the function, it takes two arguments, namely num1 and num2, which represent the numbers to be added. The script first checks if both num1 and num2 are numeric values.
If either of them is not a number, it displays an error message and exits the script with a non–zero status code. If numeric, it calculates their sum and stores it in a local variable called sum. Following the function definition, the code calls calculate_sum with the arguments 10 and 20, capturing the result in the result variable. Finally, it prints the result with the echo command.
To execute the script in your command line, type the following command:
./multiple_arguments.sh
Upon execution, the script returns “The result is: 30”, as the output of the two given variables.
Example 03: Managing and Validating Arguments
When dealing with arguments in Bash functions, it’s crucial to verify their validity and correct formatting. For instance, here I have provided another bash code to demonstrate the concept of managing and validating arguments. Its primary objective is to check for empty arguments and identify the presence of numbers within the provided input, ensuring that your script behaves reliably and accurately, regardless of the user’s input.
You can follow the steps of Example 01, to save & make the script executable.
Script (validity_check.sh) >
#!/bin/bash
# Check if no arguments were provided
if [ $# -eq 0 ]; then
echo "No arguments provided."
else
for arg in "$@"; do
if [[ -z $arg ]]; then
echo "Argument '$arg' is empty."
elif [[ $arg =~ [0-9] ]]; then
echo "Argument '$arg' contains a number."
fi
done
fi
The code begins by checking if any arguments were provided using the $# parameter. If no arguments are given, it prints “No arguments provided.“
However, when arguments are present, the script employs a for loop with $@ to iterate through them. Within this loop, it utilizes -z to check if an argument is empty, and if so, it displays “Argument ‘[arg]’ is empty.“
Additionally, it employs =~ to check if an argument contains a number using a regular expression [0-9]. If a number is found, it outputs “Argument ‘[arg]’ contains a number.
Now, run the script by the following command:
./validity_check.sh
./validity_check.sh 23
In the first command line, as there is no argument associated with the bash file, it returns “No arguments provided” to the command line. Upon providing 23 as the argument, the code returns “Argument ‘23’ contains a number.”
Example 04: Using the Division Method in the Bash Function With an Integer Argument
In this section, I’ll explore how to create a Bash script that divides an integer and then passes the result as input to a function.
You can follow the steps of Example 01, to save & make the script executable.
Script (division_function.sh) >
#!/bin/bash
calculate_division() {
result=$(( $1 / $2 ))
echo "Dividing $1 by $2 equals: $result"
}
calculate_division 50 5
This Bash script defines a function called calculate_division that calculates the division of two numbers. Inside the function, it performs the division operation and stores the result in a variable named result. Then, it displays the result along with a message. Finally, it calls the calculate_division function with the arguments 50 and 5.
You can initiate the execution of the script by using this command in your command line:
./division_function.sh
Upon execution of the division_function.sh file, the code divides the given two arguments, resulting in the output: “Dividing 50 by 5 equals: 10“.
Example 05: Passing a Function as a Parameter in a Bash Script
In example 01, I created a function that greets based on the user input. However, for now, I want to modify that code to give you an idea about passing a function as a parameter. Suppose I want to provide our greet() function with another function that delivers distinct greetings depending on the time of day.
You can follow the steps of Example 01, to save & make the script executable.
Script (function_parameter.sh) >
#!/bin/bash
greet_based_on_hour() {
hour_now=$(date +%H)
# We use 10#$hour_now to prevent numbers with a leading zero from being interpreted as octal
if (( 10#$hour_now >= 5 && 10#$hour_now < 12 )); then
echo "Good morning. The current hour is $hour_now."
elif (( 10#$hour_now >= 12 && 10#$hour_now < 18 )); then
echo "Good afternoon. The current hour is $hour_now."
elif (( 10#$hour_now >= 18 && 10#$hour_now < 22 )); then
echo "Good evening. The current hour is $hour_now."
else
echo "Good night. The current hour is $hour_now."
fi
}
greet_based_on_hour
This Bash script defines a function called greet_based_on_hour that greets the user based on the current hour. It retrieves the current hour using date +%H, ensuring numbers with leading zeros are interpreted as decimal with 10#. Then, it employs conditional statements to determine the appropriate greeting message based on the time of day. Finally, it calls the greet_based_on_hour function to display the corresponding greeting message according to the current hour.
Now, execute the file via the command line by typing the following command:
./function_parameter.sh
As the current time during the execution time was 12:23, the code greets as “Good afternoon. The current hour is 12” upon the code execution.
Example 06: Positional and Named Arguments With a Bash Function
In Bash function with parameters, the typical approach involves using positional arguments, as demonstrated in the previous examples. Nonetheless, it’s also possible to employ named arguments, like the options commonly found in command-line utilities.
You can follow the steps of Example 01, to save & make the script executable.
Script (named_function.sh) >
#!/bin/bash
function process_input_output() {
local input_name=""
local output_name=""
while [ "$#" -gt 0 ]; do
case "$1" in
-i|--input)
input_name="$2"
shift 2
;;
-o|--output)
output_name="$2"
shift 2
;;
*)
echo "Unknown option: $1"
return 1
;;
esac
done
# Display the input and output file names
echo "Input name: $input_name"
echo "Output name: $output_name"
}
process_input_output -i "input.txt" -o "output.txt"
This Bash script defines a function named process_input_output designed to handle input and output file names as options. It uses a while loop to iterate through the provided arguments, parsing options like -i or –input for the input file name and -o or –output for the output file name. The function stores these names in local variables and then displays them. When called with -i “input.txt” and -o “output.txt”, it prints the input and output file name accordingly.
Write the following command to execute the file in your command line:
./named_function.sh
Upon giving the input and output file, the file returns the input and output file name accordingly.
Tips for Efficient Bash Scripting With Functions and Arguments
So far, you have learned various practical examples about the use of parameters in the bash function. However, here are some tips, which may guide you to develop efficient Bash scripting with functions and arguments.
- It’s essential to consistently verify arguments to guarantee your script operates correctly.
- Utilize local variables inside functions to enhance readability and prevent conflicts with global variables.
- Incorporate error–handling mechanisms within your functions to deliver informative responses when issues arise. Employ the return statement to exit a function with an appropriate exit code.
- Make sure each function has a single, clear purpose, which makes them easier to manage, understand, and reuse.
- Utilize comments and descriptive function names to enhance your code’s clarity and readability.
- Thoroughly test your functions to ensure they perform as intended in various situations.
Conclusion
In conclusion, understanding how to use parameters in Bash functions is a fundamental skill for any Bash script developer. This article has provided a thorough introduction to the concept, covering the syntax for defining functions with parameters and offering practical examples to illustrate their usage. However, if you have any questions or queries related to this article, feel free to comment below. Thank You.
People Also Ask
How do you pass parameters in bash function?
You can pass parameters to a Bash function by specifying them when calling the function. For example, if you have a function named my_function and want to pass two parameters, you would call it like this: my_function parameter1 parameter2
What is $1 in bash function?
In a Bash function, $1 refers to the first command-line argument passed when calling the function. It allows the function to access and use the first argument within its code.
What does $@ mean in shell script?
In a shell script, $@ is a special variable that represents all the command-line arguments passed to the script. It treats each argument as a separate entity, making it useful for iterating through and processing multiple input values within the script.
Can we call a function without parameters?
Yes, you can call a function without parameters in a Bash script. Bash functions can be defined with or without parameters, and you can choose to call them with or without providing arguments.
Related Articles
- How to Pass All Parameters in Bash Scripts? [6 Cases]
- Parsing Parameters in Bash Scripts [5 Examples]
- What is $0 in Bash Script? [4 Practical Examples]
- Difference Between $$ Vs $ in Bash Scripting [With Examples]
- How to Use Alias with Parameters in Bash Scripting? [6 Examples]
<< Go Back to Parameters in Bash Scripting | Bash Scripting Tutorial