FUNDAMENTALS A Complete Guide for Beginners
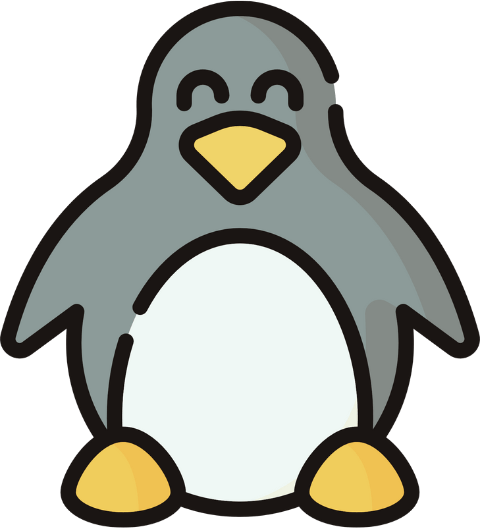
Bash scripting is a versatile way to integrate your algorithm with the Linux operating system. Central to its flexibility are parameters, which allow scripts to accept various inputs, adapt to different scenarios, and enhance reusability. This article explores the different aspects of parameters in Bash scripting, including positional, named, even alias parameters, and so on. So let’s start.
What is Bash Parameter?
A Bash parameter is a value or piece of information that is passed to a Bash script or function when it is executed. Parameters enable scripts to receive inputs, making them more versatile, adaptable, and user-friendly.
Think of Bash parameters as the secret ingredients you toss into a recipe to give it that extra specialty. They’re the values you pass to your scripts when you run them, opening up possibilities. Moreover, you can customize your scripts, make them user-friendly, and even handle unexpected twists and turns.
Let’s say you want to create a script that will greet someone whenever you pass any name to the script. Copy the following code in your nano editor and execute the file afterwards.
#!/bin/bash
name=$1 # The first parameter passed to the script
echo "Hello, $name!"
The first line of our code is a shebang, and it tells the system to execute the script using the Bash interpreter located at /bin/bash. By using the name=$1, you’re assigning the value of the first positional parameter (provided when the script is run) to the variable name. The variable $1 holds the value of the first parameter passed to the script. Then, echo “Hello, $name!” line uses the echo command to print out a message. The string then takes the value of the $name variable and it displays the value.
Types of Parameters in Bash Scripting
In Bash, parameters refer to the values or arguments provided to a script or command when it is executed. There are several ways to access these parameters within a script and you can divide all the parameters into several categories. Down below, I am going to discuss four different types of bash parameters.
1. Bash Positional Parameters
Positional parameters in Bash are a set of variables that capture and retain values passed as arguments when a script or command is invoked. The foremost among these parameters is $0, which signifies the name of the script or command itself. It is essential for self-reference. Accompanying this is a sequence of $1, $2, $3, and so on, representing the arguments provided in the order they appear. For instance, let’s have a look at the following code.
./script.sh arg1 arg2
when executing the ./script.sh arg1 arg2, $1 corresponds to arg1, and $2 corresponds to arg2. The script will do certain types of work using those positional values. Additionally, $# holds the count of arguments passed, offering a numeric perspective on the provided inputs.
2. Bash Optional Parameters
In Bash scripting, optional parameters can also be implemented using flags or options. This approach allows users to provide specific options to the script, and the script responds accordingly. This is also known as bash parameter parsing. The getopts command is often used to handle such flags. It’s a built-in Bash command that simplifies the process of parsing command line options.
Optional parameters are often indicated through short or long options. Short options consist of a single character with a preceding dash (-), while long options are comprised of words with two preceding dashes (–). The syntax for denoting an optional parameter in Bash takes the form:
script_option.sh [-s|--longoption] [argument]
In this context, script_option.sh represents the script’s name, -s serves as a short option, –longoption functions as a long option, and argument stands as an optional argument that can be provided alongside the option. To use optional parameters in a Bash script, you can use the getopts command.
The basic syntax for using getopts is:
while getopts <options> <variable>
do
case $<variable> in
<option1>) <command1> ;;
<option2>) <command2> ;;
...
<optionN>) <commandN> ;;
esac
done
In this context, <options> represents a sequence of permissible options, <variable> denotes the variable designated for holding the current option, <option1> through <optionN> provides the potential options that the script is capable of receiving, and <command1> through <commandN> signify the actions that the script should carry out upon receiving the respective option.
By following the abovementioned syntax, you can also create named parameters to specify options and their corresponding values in a more intuitive and human-readable manner.
Named Parameters in Bash Scripts
Before going to delve into the topic, let’s first understand why you need to use the named parameter instead of using the positional parameter in the first place. As I have already discussed that positional arguments like $1, $2 pass the command-line arguments. However, the effectiveness of positional arguments is inefficient as it can become unclear which argument corresponds to which data type. In contrast, named arguments offer a more meaningful option, allowing a precise understanding of the data each argument represents. The basic syntax of the named parameters is as follows:
scriptname.sh --ParameterName ParameterValue
Named parameters typically involve using flags (short or long options i.e ParameterName in the above syntax) to denote specific parameters and then associating values with those flags. This makes it easier for users to remember and provide only the necessary inputs, even if the script has a complex set of options.
3. Bash Special Parameters
Special parameters in Bash are variables that hold specific information, like the script’s name, the number of arguments passed, exit status of the last command, and process IDs. Here is a list of special parameters in Bash:
Parameter’s Syntax | Description |
---|---|
$0 | The name of the script or command. |
$1, $2, … | The positional parameters representing arguments are provided to the script or command. |
$# | The count of positional parameters. |
$@ | All positional parameters as separate quoted strings. |
$? | The exit status of the last executed command. |
$$ | The process ID (PID) of the current shell. |
$! | The PID of the last background command. |
$- | The current shell options. |
$* | All positional parameters as a single string. |
$IFS | The internal field separator, is used for word splitting. |
These special parameters collectively offer insight into the script’s environment and execution outcomes, thereby facilitating the development of more responsive and adaptable Bash scripts.
2 Practical Cases of Using Bash Parameters
Bash parameters are valuable when you want to add flexibility and customization to your scripts. Here are 2 practical cases that I am going to discuss below. Don’t forget to run the provided code in your distribution to understand the concept fully.
1. Using Bash Parameters in a Function
Bash parameters in functions are indispensable when creating versatile and interactive scripts. By using parameters, you can dynamically customize the behavior of your functions based on input values. Let’s have a practical example that demonstrates how the function works with Bash parameters.
Suppose you need a function to perform arithmetic addition operations. You want to pass two numbers as parameters to the function. You can use the following code to accomplish the task:
#!/bin/bash
# Define a function to calculate the sum of two numbers
calculate_sum() {
local num1=$1
local num2=$2
local sum=$((num1 + num2))
echo "The sum of $num1 and $num2 is: $sum"
}
calculate_sum $1 $2
Here, $1 and $2 bother are bash parameters. Now if you want to run the bash scrip by the following command, you will get an output as given below.
./bash_function.sh 5 6
Upon applying two command line arguments 5 and 6, the bash_function returns their arithmetic summation by displaying “The sum of 5 and 6 is: 11”.
2. Creating Bash Alias with Parameters
In Bash, aliases don’t support parameters, yet you can work around this by crafting functions that can process command–line arguments. These functions can subsequently be employed as aliases within your Linux environment. To illustrate, Let’s have a look at the following command:
lsdir(){ ls -l $1 | grep "^d"; }
The given alias configuration establishes an alias labeled lsdir, which accepts an argument represented by ($1) denoting the directory that we want to list. When engaging this alias, you can provide the desired directory as an argument during its invocation. For instance:
lsdir /home/miran
As the image shows, a detailed listing of the folders located in the /home/miran directory and refine the displayed output to exclusively reveal the directory entries.
Conclusion
In conclusion, the bash parameter is an effective way to communicate and pass the required argument to the bash script. In this article, I have provided an overall understanding of what is bash parameters. I have also discussed the different practical cases to use and manipulate the bash parameter in your script. However, if you have any questions or queries relevant to this article, don’t forget to comment below. Thank you!
People Also Ask
How to assign bash parameters?
In Bash, parameters are assigned using special variables. The most common ones are “$1” for the first parameter, “$2” for the second, and so on. To assign a value to a variable, you use the “=” operator without spaces. For example:
#!/bin/bash
# Assigning values to variables using parameters
first_param=$1
second_param=$2
# Printing the assigned values
echo "First parameter: $first_param"
echo "Second parameter: $second_param"
In this script, the values of the first and second parameters passed to the script are assigned to variables first_param
and second_param
, respectively. The echo statements then print out these assigned values.
How to pass parameters to bash command?
To pass parameters to a Bash command, simply provide the parameters after the command in the command line. For example command parameter1 parameter2 parameter3. Replace the command with the actual command you’re running, and replace parameter1, parameter2, and parameter3 with the specific parameters you want to pass to the command.
What is $0, $? and $# in shell scripting?
In shell scripting, $0 represents the script’s name or the current shell command, $? stores the exit status of the last executed command, and $# indicates the count of arguments passed to a script or function.
What is $$ variable in bash?
In Bash scripting, the $$ variable represents the process ID (PID) of the currently running script or shell. It provides a unique identifier for the specific instance of the script or shell session.
What is $* in shell script?
In shell scripting, $* represents all the command–line arguments passed to a script as a single string, with each argument separated by spaces.
Related Articles
- How to Pass All Parameters in Bash Scripts? [6 Cases]
- How to Use Positional Parameters in Bash Script? [2 Examples]
- Parsing Parameters in Bash Scripts [5 Examples]
- 4 Methods to Pass Named Parameters in a Bash Script
- What is $0 in Bash Script? [4 Practical Examples]
- Difference Between $$ Vs $ in Bash Scripting [With Examples]
- How to Use Alias with Parameters in Bash Scripting? [6 Examples]
- How to Use Bash Function with Parameters? [6 Examples]
<< Go Back to Bash Scripting Tutorial