FUNDAMENTALS A Complete Guide for Beginners
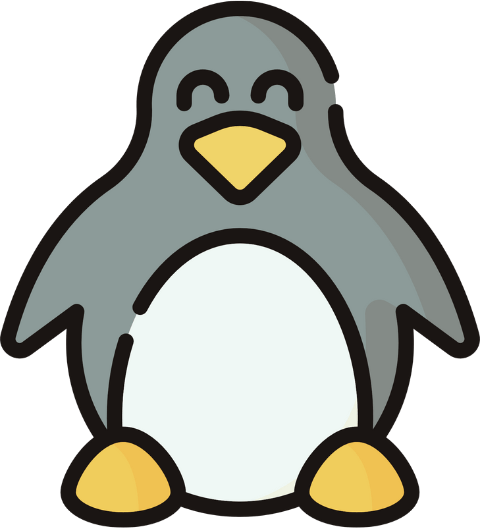
Bash parameters serve as a means for users to supply inputs to running scripts. Leveraging bash parameters/arguments enables the creation of reusable scripts, minimizing repetitive code. In this article, I will explore some cases of passing all parameters in bash scripts. So let’s start.
Key Takeaways
- Getting familiar with all bash parameters.
- Learning on passing parameters to the bash algorithm.
- Calculating mathematical operations using the bash parameters.
Free Downloads
List of All Bash Parameters
Here is a list of all the parameters typically used in Bash scripting.
Parameter’s Syntax | Description |
---|---|
$0 | The name of the script or command. |
$1, $2, … | The positional parameters representing arguments are provided to the script or command. |
$# | The count of positional parameters. |
$@ | All positional parameters as separate quoted strings. |
$? | The exit status of the last executed command. |
$$ | The process ID (PID) of the current shell. |
$! | The PID of the last background command. |
$- | The current shell options. |
$* | All positional parameters as a single string. |
$IFS | The internal field separator, is used for word splitting. |
However, in the following section of my article, I will use $@ and $# parameters to give you some overall demonstration of passing all parameters in bash scripting.
6 Practical Cases of Passing All Parameters in Bash Scripting
To demonstrate the concept of my article, especially how to employ bash parameters to pass the value in a code, I will write 6 different scripts in the following section which will be started from iterating through parameters using loops, storing them in arrays for manipulation, and even employing conditional checks to ensure the presence of necessary arguments. So let’s start with one by one.
Case 01: Passing all Parameters as String in a Bash Script
Passing all parameters as a string in a Bash script involves collecting all command-line arguments into a single string. This approach treats the entire set of arguments as a unified entity. Using $* or $@ within the script allows for capturing all parameters. However, in this specific code, I will use $* to get all parameters in a string format.
❶ At first, launch an Ubuntu Terminal.
❷ Write the following command to open a file in Nano:
nano string_parameters.sh
- nano: Opens a file in the Nano text editor.
- string_parameters.sh: Name of the file.
❸ Copy the script mentioned below:
#!/bin/bash
# $* to get all the parameters from the command line
echo "Ready to take all the arguments as string."
echo "$*"
The first line #!/bin/bash is called the shebang line indicates that the Bash shell executes the script. Then, it’s stated that the script is prepared to gather all input arguments as a continuous string. It uses the echo command with $* to display all parameters provided through the command line as a unified string.
❹ Press CTRL+O and ENTER to save the file; CTRL+X to exit.
❺ Use the following command to make the file executable:
chmod u+x string_parameters.sh
- chmod: Changes the permissions of files and directories.
- u+x: Here, u refers to the “user” or the owner of the file and +x specifies the permission being added, in this case, the “execute” permission. When u+x is added to the file permissions, it grants the user (owner) permission to execute (run) the file.
- string_parameters.sh: File name.
❻ Run the script by the following command:
./string_parameters.sh Hello LinuxSimply 33
As the image depicts above, the command line returns all of the positional arguments I provided in the command line: Hello LinuxSimply 33 as string form followed by the “Ready to take all the arguments as string” string.
Case 02: Passing All the Bash Parameters in an Array
An array comprises a series of characters or strings. To obtain an array of arguments passed, $@ is utilized, followed by processing them through a loop. This approach is beneficial when there’s a need to repetitively execute a task on the given input.
You can follow the steps of Case 01, to save & make the script executable.
Script (array_parameters.sh) >
#!/bin/bash
# Store all parameters in an array
params=("$@")
# Display all parameters using the array
echo "All Parameters: ${params[@]}"
# Loop through the array and display each parameter
for param in "${params[@]}"; do
echo "Parameter: $param"
done
Here, an array named params is created to store all the positional parameters passed to the script. The syntax (“$@”) expands the special variable $@ into an array, with each parameter as a separate element. The parentheses ensure that individual parameters are treated correctly, even if they contain spaces. Following this, a for loop iterates through the array, individually processing each element. Within the loop, the value of each parameter is printed with the label “Parameter:”.
Run the script by using the following command.
./array_parameters.sh Hello LinuxSimply 1200
Executing the script with arguments like ./array_parameters.sh Hello LinuxSimply 1200 yields an output that lists all parameters as a cohesive string and subsequently displays each parameter with the designated label as like Parameter: Hello Parameter: LinuxSimply Parameter: 1200.
Case 03: Reading All the Parameters Using Loop in a Bash Script
In a bash script, you can read all the parameters passed to the script using the special variable $@. This variable holds all the arguments passed to the script as separate words. To read these parameters using a loop, you can use a for loop that iterates over each parameter in $@. Here’s an example of how you can do this:
You can follow the steps of Case 01, to save & make the script executable.
Script (loop_parameters.sh) >
#!/bin/bash
# Loop through all positional parameters
for arg in "$@"; do
echo "Positional Parameter: $arg"
done
The provided Bash script employs a for loop to iterate through all the positional parameters supplied to the script. The loop is initiated with the declaration for arg in “$@”; do, where “$@” holds the individual arguments. Within the loop, the echo statement prints each parameter’s value preceded by the label “Positional Parameter:“.
Run the script by using the following command.
./loop_parameters.sh LinuxSimply Ubuntu Hello
Upon executing the bash file, the command line returns: Positional Parameter: LinuxSimply, Positional Parameter: Ubuntu, Positional Parameter: Hello.
Case 04: Read All Parameters After the Nth Position
The concept of reading the parameter after the nth position in Bash scripting involves manipulating the sequence of positional parameters to focus on a subset of values. By utilizing the shift command within a loop, you can discard the initial parameters and effectively shift the script’s attention to those positioned after the specified point.
You can follow the steps of Case 01, to save & make the script executable.
Script (shift_nth_parameters.sh) >
#!/bin/bash
# Define the position after which you want to start reading parameters
echo -n "From which position you want to start: "
read n
# Shift to remove the first 'n' parameters
for ((i=1; i<=n-1; i++)); do
shift
done
# Display the parameters after the nth position
echo "Parameters after the $n-th position: $@"
The Bash script begins by prompting the user to input a position value n from which they wish to start reading parameters. The read command collects this value. Subsequently, a for loop is employed, using the input n to shift the parameters to the left, discarding the first n-1 parameters. Lastly, the script prints the parameters that remain after the n-th position using the $@ variable.
Copy and paste the following command to run the script.
./shift_nth_parameters.sh 4 5 2 23 7 8
Now if you execute the script with ./shift_nth_parameters.sh 4 5 2 23 7 8, the result will be Parameters after the 3-th position: 2 23 7 8.
Case 05: Displace All the Parameters Using the Shift Command
In a bash script, the shift command is used to shift the positional parameters to the left, effectively discarding the first parameter and moving each subsequent parameter from one position to the left. This is useful when you want to process parameters in groups or remove the first parameter to focus on the rest.
You can follow the steps of Case 01, to save & make the script executable.
Script (shift_parameters.sh)>
#!/bin/bash
#displaying the output before shifting value
echo "Current values of parameter 1 and 2: " $1 and $2
shift
#displaying the output after shifting.
echo "After making 1 shift: " $1 and $2
shift 3
echo "After 3 more shifts: " $1 and $2
Initially, The code displays the values of the first and second positional parameters using $1 and $2. After invoking the shift command, the parameters shift to the leftward by one position, causing the value of $2 to take the place of $1. Subsequently, the script prints the updated values. Then, an additional shift 3 command discards the first three parameters, moving the values in positions beyond $3 to $1 and $2. The script concludes by printing the final values of the repositioned parameters.
Run the script by using the following command.
./shift_parameters.sh 1 2 3 4 5 6 7 8
Upon execution the script returns, Current values of parameter 1 and 2: 1 and 2, After making 1 shift: 2 and 3, and After 3 more shifts: 5 and 6.
Case 06: Using Bash All Parameters to Perform Arithmetic Operations
Now by iterating through the positional parameters using a loop, you can apply arithmetic operations to each parameter individually or to a series of parameters collectively. Follow the script given below.
You can follow the steps of Case 01, to save & make the script executable.
Script (arithmetic_parameters.sh) >
#!/bin/bash
# Check if at least two parameters are provided
if [ "$#" -lt 2 ]; then
echo "Please provide at least two parameters."
exit 1
fi
# Store the first parameter
result="$1"
shift
# Loop through the remaining parameters and perform addition
while [ $# -gt 0 ]; do
result=$((result + $1))
shift
done
# Display the result
echo "Sum of parameters: $result"
The provided Bash script focuses on performing arithmetic addition on a set of input parameters. It first ensures that a minimum of two parameters are provided, displaying an error message and exiting if the condition is not met. The script then captures the initial parameter, shifting it out to concentrate on the remaining ones. Within a while loop, it iterates through the remaining parameters, progressively adding each to the result using arithmetic expansion. After processing all parameters, the script displays the sum with the help of an echo command.
Copy and paste the following command to run the script.
./arithmetic_parameters.sh 45 5 2
Finally, executing the script with ./arithmetic_parameters.sh 45 5 2 yields the output “Sum of parameters: 52“.
Conclusion
In conclusion, this article has explored various approaches to pass all the parameters, such as iterating through parameters using loops, storing them in arrays for manipulation, and even employing conditional checks to ensure the presence of necessary arguments. Hope it will work as a complete guide to learn the bash language. However, if you have any questions or queries, feel free to comment below. Thank You!
People Also Ask
Related Articles
- How to Use Positional Parameters in Bash Script? [2 Examples]
- Parsing Parameters in Bash Scripts [5 Examples]
- 4 Methods to Pass Named Parameters in a Bash Script
- What is $0 in Bash Script? [4 Practical Examples]
- Difference Between $$ Vs $ in Bash Scripting [With Examples]
- How to Use Alias with Parameters in Bash Scripting? [6 Examples]
- How to Use Bash Function with Parameters? [6 Examples]
<< Go Back to Parameters in Bash Scripting | Bash Scripting Tutorial