FUNDAMENTALS A Complete Guide for Beginners
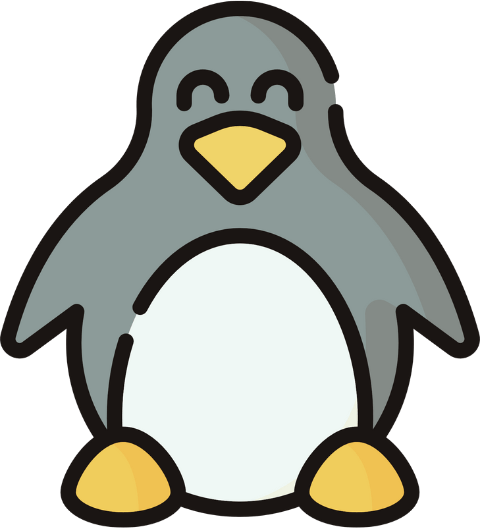
In bash scripting, $0 is a special parameter. It represents the name of the script or the currently executing shell script. When you run a bash script, $0 holds the name of the script file itself. This variable can be useful for self-referencing within the script or for displaying the script’s name in messages or logs. In this article, I am going to show you 4 different examples by using $0 in bash script. So let’s start.
Key Takeaways
- Learning about the $0 special parameter and its usability in a bash script.
- Retrieving the path directory of the file using the $0 parameter.
Free Downloads
Meaning & Purpose of “$0” in Bash Scripting
The special variable $0 in the bash code represents the name of the currently executed script or shell. This variable is particularly useful for self-referencing within scripts, allowing you to access the script’s name to create dynamic behavior. For instance, you might use it to display the script’s name in error messages or to derive the script’s directory path. By referencing $0, you can make your script more adaptable and informative.
4 Practical Examples of Using “$0” Parameter in Bash Script
As I have said earlier, you can retrieve the script name or path location by using the $0 parameter. To give you a hands-on experience, I am going to discuss 4 different examples of using $0 in a bash script. Let’s check these out.
Example 01: Displaying the Current Shell Script Name by Using the “$0” Parameter
In bash scripting, you can utilize the $0 parameter to display the name of the currently running shell script. To display the script’s name, you can simply use the $0 parameter within your script, like this:
❶ At first, launch an Ubuntu Terminal.
❷ Write the following command to open a file in Nano:
nano LinuxSimply.sh
- nano: Opens a file in the Nano text editor.
- LinuxSimply.sh: Name of the file.
❸ Copy the script mentioned below:
#!/bin/bash
# Display the script name
echo "The name of the current shell script is: $0"
The Bash script begins with a shebang (#!/bin/bash) indicating that it should be interpreted and executed using the Bash shell. The echo command is used to print the text “The name of the current shell script is: ” followed by the value of the special variable $0, which represents the name of the script itself. When the script is executed, it will output the name of the script to the terminal.
❹ Press CTRL+O and ENTER to save the file; CTRL+X to exit.
❺ Use the following command to make the file executable:
chmod u+x LinuxSimply.sh
- chmod: Changes the permissions of files and directories.
- u+x: Here, u refers to the “user” or the owner of the file and +x specifies the permission being added, in this case, the “execute” permission. When u+x is added to the file permissions, it grants the user (owner) permission to execute (run) the file.
- LinuxSimply.sh : File name.
❻ Run the script by the following command:
./LinuxSimply.sh
As the image shows, the code returns “The name of the current shell script is: ./LinuxSimply.sh in the command line.
Example 02: Displaying Script Location Using “$0” Parameter
Following the previous example, I am going to provide another bash code by using the $0 parameter. The script’s purpose is to determine and display the directory containing the script itself. When the script is executed, it will output the absolute path to the directory containing the script.
You can follow the steps of Example 01, to save & make the script executable.
Script (file_location.sh) >
#!/bin/bash
# Get the directory containing the script using readlink
script_directory=$(dirname "$(readlink -f "$0")")
# Print the script location
echo "Script location: $script_directory"
First, the readlink -f “$0” command retrieves the absolute path of the script, and the dirname command extracts the directory from the obtained path. The result is stored in the variable script_directory. The echo command prints the message “Script location: ” followed by the value stored in script_directory.
Run the following command in your terminal.
./file_location.sh
As the image shows above, the command line returns Script location: /home/miran as the absolute directory of the current shell script.
Example 03: Read a File Using “$0” Parameter in Bash Script
Apart from displaying the file name, we can also read the content of the file using the $0 parameter in the bash script. Here, I am going to give another bash script. The script’s purpose is to read and process each line from a file named “filename.txt” located in the same directory as the script. In filename.txt, it contains Hello LinuxSimply in it. Now I will read this content with the help of a bash code. So let’s see.
You can follow the steps of Example 01, to save & make the script executable.
Script (read_file.sh) >
#!/bin/bash
# Extract the directory path of the script
script_dir=$(dirname "$0")
# Define the file path relative to the script directory
file_path="$script_dir/filename.txt"
# Read and process each line from the file
while IFS= read -r line; do
echo "Line: $line"
done < "$file_path
The script starts by using the dirname “$0” command to extract the directory path of the script itself and stores it in the variable script_dir. Then, the file_path variable is defined by concatenating script_dir with “/filename.txt“.
The script enters a while loop that reads each line from the file specified by $file_path. The IFS= read -r line command reads each line while preserving leading and trailing whitespace. The loop continues until all lines have been read from the file. The < “$file_path” at the end of the loop ensures that the loop’s input comes from the specified file. When executed, the script will read filename.txt line by line and display each line along with the “Line: ” prefix.
Execute the following command in your terminal.
./read_file.sh
As you can see, the command line returns Line: Hello LinuxSimply as data contained in the filename.txt.
Example 04: Using the “$0” Parameter in the Bash Function
You can also incorporate the $0 parameter in a bash function. Here I am going to provide a sample bash code to illustrate the idea. The purpose of this Bash script is to define a function called func that takes two parameters, calculates their multiplication, and then displays the result along with the name of the script.
You can follow the steps of Example 01, to save & make the script executable.
Script (bash_function.sh) >
#!/bin/bash
func() {
Mul=$(( $1 * $2 ))
echo "The multiplication of the two given numbers is: $Mul"
echo "The name of the script is:" $0
}
func $1 $2
This Bash script begins with a shebang (#!/bin/bash) indicating it’s executed using Bash. It defines a function func that takes two parameters. Inside the function, the parameters are multiplied and stored in the variable Mul. The script then echoes the multiplication result and the script’s name using the special variable $0. Finally, the function is invoked with the provided arguments from the command line ($1 and $2).
Use the following command to run the script.
./$0_function.sh 4 3
As the image depicts above, I passed 4 and 3 integers along with the script name. Then, the script returns The multiplication of the two given numbers is: 12 and The name of the script is: ./bash_function.sh as the output.
Conclusion
In conclusion, the $0 parameter in bash scripting serves as a valuable tool for referencing the name of the currently executing script or shell. By incorporating $0 into your script, you can easily retrieve and utilize the script’s name, handle errors etc. In this article, I have provided 4 different examples to illustrate the idea fully. However, If you have any questions or queries, don’t forget to comment below. Thank You!
People Also Ask
Related Articles
- How to Pass All Parameters in Bash Scripts? [6 Cases]
- How to Use Positional Parameters in Bash Script? [2 Examples]
- Parsing Parameters in Bash Scripts [5 Examples]
- 4 Methods to Pass Named Parameters in a Bash Script
- Difference Between $$ Vs $ in Bash Scripting [With Examples]
- How to Use Alias with Parameters in Bash Scripting? [6 Examples]
- How to Use Bash Function with Parameters? [6 Examples]
<< Go Back to Parameters in Bash Scripting | Bash Scripting Tutorial