FUNDAMENTALS A Complete Guide for Beginners
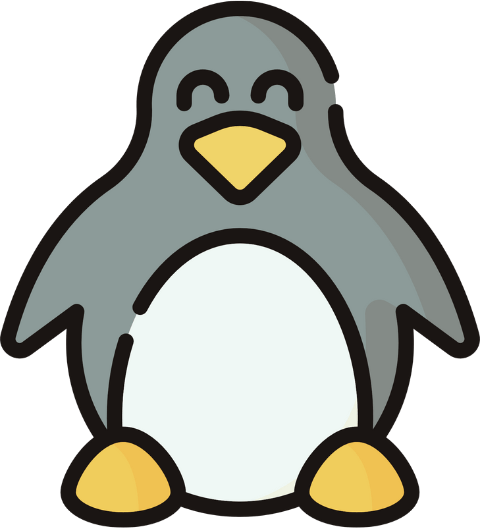
To resolve the “bad substitution” error in Bash scripts, start by reviewing the code where the error occurs. Look for parameter expansions, such as ${variable}, and ensure they are properly formatted without any syntax errors. Correctly use curly braces {} to enclose variable names, ensuring they contain only the variable name without additional characters or whitespace. Verify that there are no special characters within parameter expansions or variable assignments that could cause unexpected behaviour, and if present, escape or quote them appropriately.
Why Does the “bash bad substitution” Error Occur?
The “bash bad substitution” error message appears when there is an issue with variable replacement or expansion in the script. In bash, parameter expansion is used to manipulate variables and their value using special symbols such as ${…}. Whenever Bash encounters an error in the syntax or an operation not supported within parameter expansion, the error message “bad substitution” appears in Bash. The bad substitution error message indicates that Bash failed to understand or execute the required substitution operation because of the problem encountered.
Common reasons for encountering this error include:
- Incorrect syntax within parameter expansions: Using unsupported characters, improper nesting, or invalid syntax within ${…} can trigger this error.
- Repetition of special characters: Special characters within parameter expansions, such as quotes, braces, or dollar signs, should not be repeated and must be properly escaped or quoted to avoid unexpected behaviour.
- Extra Whitespace in parameter expansion: Additional whitespace is included within the parameter expansion, leading to unexpected behaviour and potential errors in the script.
Finding and fixing these errors in the script’s parameter expansions usually eliminates “bash bad substitution”.
3 Ways to Fix “bad substitution” Error in Bash
There are some effective ways of fixing the “bash bad substitution” error discussed below:
1. Using Regular Brackets instead of Curly Brackets
One common cause of “bad substitutions” in Bash scripts arises from using incorrect syntax, such as employing curly brackets instead of regular brackets. For instance, this error occurs when the script attempts to retrieve information, like displaying the list of files and folder of the current directory using the ls -l command but mistakenly applying improper syntax:
#!/bin/bash
echo "Here is the list of files and folders of current directory:"
# Incorrect usage of variable expansion with regular brackets
echo ${ls -l}
In this Bash script, the objective is to display a list of files and folders in the current directory using the ls -l
and echo command. However, the line echo ${ls -l}
contains incorrect usage of variable expansion with regular brackets which may lead to a “bash bad substitution” error in bash.
When you run the above script, you will get the error “bad substitution”:
The reason why the error occurs in this case is that ${…} is used for parameter expansions in bash, not for executing commands. The correct syntax for executing a command and capturing its output is either backticks (`) or $(…), known as command substitution.
To prevent the “bad substitution” error in Bash, use simple brackets instead of curly brackets. Let’s apply this correction to the script and observe the execution:
#!/bin/bash
echo "Here is the list of files and folders of current directory:"
# Incorrect usage of variable expansion with regular brackets
echo "$(ls -l)"
In this given code, the echo ${ls -l}
line has been changed to echo “$(ls -l)”
. The double quotation is used to break the line for the output result.
As you see, the modified code does not show any “bad substitution” error and allows the list of files and directories to the command line.
2. Avoid Repetition of Undesirable Characters
Another reason why you’ll see “bad substitutions” in bash scripts is because of the repeated characters. This happens when there are repeated or duplicate characters in the script, resulting in syntax errors or unpredictable behaviour. For instance, if you’re trying to define a variable with repeated characters like a dollar sign ($) inside another dollar sign, you’ll get a “bad substitution” error:
#!/bin/bash
echo "Here is the path of current directory:"
# Incorrect usage of variable expansion
echo ${$pwd}
This Bash script aims to display the path of the current directory. However, it contains incorrect usage of variable expansion, specifically in the line echo ${$pwd}
. In Bash, variable expansion is done using the $
symbol followed by the variable name. However, in this case, the $ is followed by another $
, which is incorrect syntax.
As expected, the command line returns “bad substitution” error for the
echo ${$pwd}
line.
To resolve the “bad substitution” of repetition of unwanted characters in Bash scripts, remove the redundant characters from the script:
#!/bin/bash
echo "Here is the path of current directory:"
# Correct usage of variable expansion
echo $(pwd)
echo $($pwd)
is changed to echo $(pwd)
to avoid the “bash bad substitution” error. As you see from the image, the command line does not return any bash bad substitute error, which ensures that there is no redundant syntax in the script.
3. Avoid Extra White Spaces
The third situation that may lead to encountering the “bash bad substitution” error is the presence of extra whitespace. This occurs when there are unnecessary spaces or blank characters within parameter expansions, variable assignments, or command executions in the Bash script. These extra whitespace characters can disrupt the syntax and cause Bash to misinterpret the intended operations, resulting in errors such as “bad substitution”:
#!/bin/bash
echo "Here is the list of files and folders of current directory:"
list=$(ls -l)
echo "${list }"
This Bash script lists the files and folders in the current directory using the ls -l
command and stores the output in the variable list. The echo command then prints the content of the list variable. However, the echo statement has an extra space in ${list }
, which might lead to a “bad substitution” error.
To avoid this bad substitution error in bash, make sure the script does not contain extra whitespace:
#!/bin/bash
echo "Here is the list of files and folders of current directory:"
list=$(ls -l)
echo "${list}"
For this given code, the white space in
echo "${list }"
has been removed. Now as you see, the code does not return any “bash bad substitution” error and can return the list of files and directories to the command line without any error.
Conclusion
To sum up, the “bash bad substitution” error is one of the most important errors that you face when trying to maintain the correctness and performance of their bash scripts. By thoroughly checking and fixing the syntax errors, making sure that there are no duplicate characters, and parameter expansions are correctly used. This article has discussed some possible causes and solutions of “bash bad substitution” errors. Hope this will help you to troubleshoot the script. However, if you have any questions or queries related to this article, feel free to comment below. Thank you!
People Also Ask
How to fix “bash bad substitution”?
To fix the “bash bad substitution” error, look for any wrong variable substitution or parameter expansion. Also, make sure they are enclosed and formatted correctly. Also, make sure there are no duplicate characters or repeating statements in the script. Make sure all variables are well-defined before using them. Lastly, test the script, use some debugging tools if necessary, and consult bash documentation or online sources for help.
What is process substitution in bash?
Process substitution in Bash allows to use of the output of a command or pipeline as if it were a file. It provides a way to pass the output of a command directly as input to another command or program, without the need for temporary files.
In Bash, process substitution is denoted by <(…) syntax, where the command or pipeline inside the parentheses is executed. Also, its output is treated as a temporary file-like object. This object can then be used as an argument to another command, typically for cases where the command expects a file as input.
What is command substitution?
Command substitution in Bash runs a command within another command and captures its output as a string. It’s typically done using ($(…)) syntax. This feature is useful for dynamically inserting command outputs into command lines. Also assigning them to variables for further processing in Bash scripts.
How do I stop a bash execution?
To stop the execution of a Bash script or command, you have several options at your disposal. One method is to utilize a keyboard interrupt by pressing CTRL + C, which sends a SIGINT signal to the running process. Thus it typically terminates its execution promptly. Within a Bash script, you can also use the exit command to stop execution at any point. This command terminates the script’s execution and ensure that no further commands are executed beyond the exit point.
How do I run a bash file?
To run a Bash file, follow these steps:
- Open Terminal: Launch your terminal application.
- Navigate to Directory: Use the cd command to navigate to the directory where your Bash file is located.
- Check Permissions: Ensure that the Bash file has executable permissions. If not, you can use the chmod command to add executable permissions:
chmod +x your_script.sh
- Run the Script: Execute the Bash file by typing its name preceded by ./:
./your_script.sh
- Press the Enter key to execute the command.
The Bash file will now run, and you’ll see the output in your terminal window. If there are any errors, they will be displayed in the terminal as well.
Related Articles
- Print and Handle Error with Bash Exit Code [Easy Guide]
- How to Exit on Error in Bash Script? [6+ Methods]
- How to Handle Error with “trap ERR” in Bash? [Easy Steps]
- [Solved] “No such file or directory” Error in Bash
- [SOLVED] /bin/bash^M: bad interpreter: No such file or directory
- [Fixed] “bash: syntax error near unexpected token” Error
- [Fixed!] “syntax error: unexpected end of file” in Bash
- [Solved!] Handling Error with TRY CATCH Block in Bash
<< Go Back to Bash Error Handling | Bash Error Handling and Debugging | Bash Scripting Tutorial