FUNDAMENTALS A Complete Guide for Beginners
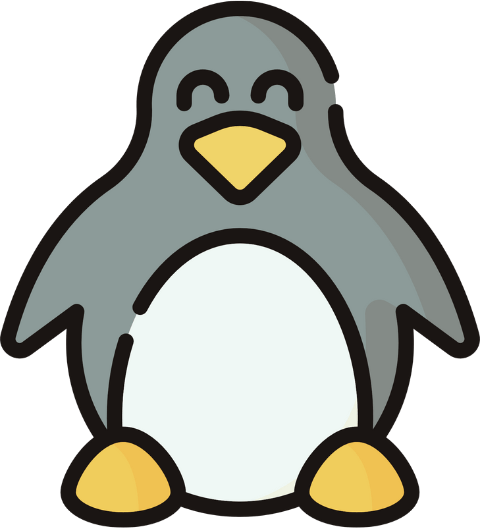
To enable “exit on error” in Bash scripts, use the “set -e” or “set -o errexit” command at the beginning of your script. This directive instructs Bash to exit immediately when a command fails, ensuring prompt error detection and termination of script execution. The following article will explore different methods to exit on error in a bash script with a detailed explanation. So let’s start!
What is “Exit on Error” in Bash?
The ‘Exit on Error’ is a technique used in bash scripting to stop the execution of the script as soon as an error is encountered. By setting this approach, your script will stop at the first error, preventing any more commands from running, and making the problem worse.
What are the Advantages of “Exit on Error” in Bash?
The advantages of “exit on error” in bash are:
- Early Detection of Issues: With “exit on error,” scripts can promptly identify and halt execution upon encountering errors, preventing further actions that could exacerbate the problem.
- Enhanced Debugging: By stopping execution at the point of failure, developers can efficiently debug scripts, pinpointing the root cause of errors and implementing necessary fixes.
- Improved Script Reliability: Implementing “exit on error” ensures script reliability by preventing partial or erroneous execution, thereby reducing the risk of unintended consequences.
- Simplified Error Handling: Rather than allowing errors to propagate throughout the script, “exit on error” simplifies error handling by focusing on isolating and addressing individual issues as they arise.
6+ Effective Techniques to Exit Bash Code on Error
When an error occurs in the bash script, you can exit in various ways. These include using the exit command, the set command, the trap command etc. Here are six effective techniques to exit bash code on error:
1. Using “set -e” Command to Exit on Error
The most handy way to close a Bash script when it encounters an error is to use the “set -e” option. This option terminates the script immediately if any command ends with a “non-zero exit code”.
Below is an example Bash script demonstrating the usage of “set -e” in error handling:
#!/bin/bash
set -e
#this command will fail
mkdirr /tmp/example
#this command will not be executed if error found
ls
The command mkdirr /tmp/example
attempts to create a directory named “example” in the “/tmp” directory but contains a typo (mkdirr
instead of mkdir). This will cause the script to terminate due to the error in the command. Consequently, the ls command after it will not be executed, as the script will exit before reaching that line.
As the picture shows above, the “mkdir” command fails, the script terminates immediately, without executing the ls command.
2. Using “exit 1” Command
When a script executes exit 1 command, it terminates the execution with a non-zero exit status, specifically 1 in this case. By leveraging this command, you can halt the execution if any error occurs.
Here are two ways you can employ the “exit 1” command to exit on an error in Bash:
A. Exit Code with “exit 1” Command
The exit code indicates the state of execution of the script. Typically, the interpreter returns two types of exit code upon execution of each code line. The zero exit code indicates the success of the code line and the non–zero code indicates an error. You can use $? variable to check the exit status of any code line and use exit 1 if finds an error. When the script encounters exit 1, it terminates immediately and returns an exit code (1) to the calling process.
To exit the code on error with “exit 1” command, use the following script:
#!/bin/bash
mkdirr /tmp/example
#Using exit code to check if the previous command failed
if [ $? -ne 0 ]; then
exit 1
fi
#this command will not be executed if error found
ls
mkdirr /tmp/example
command attempts to create a directory named “example” in the “/tmp” directory. However, there’s a typo in the command (mkdirr
instead of mkdir
), causing it to fail. It then checks its exit code using $?
variable. If the exit code is non-zero, it exits with exit 1. Subsequent commands, like ls, won’t execute upon failure.
As you see from the image above,
mkdirr /tmp/example
line fails to execute and terminates the further execution with an exit code 1. The script returns a default error line to the terminal.
B. OR Operator with “exit 1” Command
Another way to exit on error is to use the exit 1 command along with the OR Operator (||).
To apply ‘exit on error’ in a bash script, place the possibly-failing command before ‘|| exit 1’:
#!/bin/bash
# Execution will not proceed if an error is found in the first command
mkdirr /tmp/example || exit 1
#this command will not be executed if an error found
ls
In this Bash script, the first command attempts to create a directory using mkdirr /tmp/example
. If this command fails, indicated by a non-zero exit status, the script will go for the second command and exit with exit 1
. Consequently, the subsequent ls command won’t execute if an error is encountered during the execution of the first command.
As you can see from the image above, the line ‘mkdirr / tmp/example’ failed because of a typo and the script aborted the execution and returned a default error line to the terminal.
3. Using “trap” Command with Exit Argument
The trap command in bash uses the EXIT signal to execute a specific command when the script terminates. You can specify a specific action to perform when a script terminates, regardless of why it terminates.
Here is a sample code demonstrating the use of the trap command with an exit argument:
#!/bin/bash
# Set trap to exit on error
trap 'echo "Error occurred. Exiting..."; exit 1' ERR
# Example command
mkdirr /tmp/example
#this command will not be executed if an error found
ls
In this Bash script, a trap is set to exit on error using trap 'echo "Error occurred. Exiting..."; exit 1' ERR
. Afterwards, mkdirr /tmp/example
attempts to create a directory, and if it fails, the script exits with an error message. The subsequent ls command won’t execute upon encountering an error.
As in the given script,
mkdirr /tmp/example
line fails to execute due to the typo “mkdirr” instead of mkdir command, thus returning a non–zero exit code. The ERR signal is activated due to this reason and enables the echo command within the trap command. Thus the terminal provides a customized error message, “Error occurred. Exiting…” which is evident from the image given above.
4. Using “set -o errexit” Command
The “set -o errexit” command allows you to enable the “exit on error” function in bash. In Bash, if any command in a script terminates with a “non-zero status” (which indicates failure), the script terminates immediately using the “set -o errexit” command.
To enable the “exit on error” in bash, use the “set -o errexit” Command at the beginning of the script as below:
#!/bin/bash
# Enable "exit on error" feature
set -o errexit
# Example command
mkdirr /tmp/example
#this command will not be executed if error found
ls
In this Bash script, set -o errexit
enables the “exit on error” feature, causing the script to terminate immediately if any command fails. The command mkdirr /tmp/example
attempts to create a directory, and if it fails, the script exits without executing further commands. Thus, the subsequent ls command won’t be executed upon encountering an error.
As the image suggests, the
mkdirr /tmp/example
line fails due to a typo and the script stops the execution and returns a default error line to the terminal.
5. Using “set -eo pipefail” Command
The command “set -eo pipefail” in Bash enables two features simultaneously: “exit on error” (set -e) and “pipefail” (set -o pipefail). The “set -e” ensures that the script exits immediately if any command within it exits with a non-zero status, indicating failure. Additionally, the “set -o pipefail” ensures that if any command in a pipeline fails, the entire pipeline’s exit status reflects that failure, rather than just the exit status of the last command.
To use “set -eo pipefail” in a Bash script, you would include it at the beginning of your script, typically after the shebang line (#!/bin/bash). Here’s an example of how you would apply it:
#!/bin/bash
# # Enable 'exit on error' and 'pipefail' options
set -eo pipefail
# Example command
mkdirr /tmp/example | echo "LinuxSimply"
#this command will not be executed if error found
ls
In this Bash script, set -eo pipefail
enables the “exit on error” and “pipefail” options, causing the script to terminate immediately if any command fails or if a command in a pipeline fails. The command mkdirr /tmp/example
attempts to create a directory, and if it fails, the script exits without executing further commands. Additionally, the pipeline fails if mkdirr
fails, preventing the subsequent echo "LinuxSimply"
command from executing. Consequently, the ls command won’t execute if an error is encountered.
As you see, the script executes the
echo "LinuxSimply"
line but fails to execute mkdirr /tmp/example
. Overall the exit status is a non-zero value, thus the program terminated and did not execute the ls command.
6. Using “set -u” Command
The “set -u” command is a Bash option that enables the “uninitialized variable” detection feature. This setup ensures that scripts handle uninitialized variables appropriately. Whenever there is an uninitialized variable found during the execution time, the script will terminate immediately. Here’s how the “set -u” command works in a Bash script:
#!/bin/bash
# Enable 'uninitialized variable' detection
set -u
# Example using an uninitialized variable
echo "The value of variable foo is: $foo"
# This ls command will not be executed due to the error caused by the uninitialized variable
ls
In this Bash script, set -u
enables the detection of uninitialized variables. The script attempts to echo the value of the variable foo, which has not been initialized. This causes an error, and the script terminates without executing the subsequent ls command.
As you can see from the image above, the provided code detects the unbound variable foo due to the presence of the “set -u” command. The execution is terminated once the code finds the unset variable.
Conclusion
In conclusion, the article explores the significance and implementation of error handling techniques in Bash scripting. It emphasises different techniques to enhance script reliability by immediately exiting upon encountering errors. By enabling these techniques, Bash scripts can effectively handle errors, prevent unexpected behaviour, and ensure predictable execution flow. However, if you have any questions or queries related to this article, feel free to comment below. Thank you!
People Also Ask
What does Bash exit do?
The exit command terminates the bash shell session or the bash script. When the bash shell is executed with no arguments, the bash shell exits with the exit status value set to zero indicating that the bash shell has completed successfully.
However, you can specify the output of the bash script or shell session by specifying the exit status code. The output status code can be set from 0 to 255. A value of 0 indicates that the bash shell is working correctly, while a non–zero usually indicates that the shell is not working correctly.
When the bash shell encounters the exit command, it immediately pauses and returns control to the parent process or to the calling environment.
How do I exit a Bash script?
To exit a Bash script, you can use the exit command followed by an optional exit status code. For example:
#!/bin/bash
# Your script commands here
# Exit the script with a success status code
exit 0
When the exit command is encountered in a Bash script, it terminates the script’s execution immediately and returns control to the shell or parent process that invoked it. The optional exit status code (in this case, 0) can indicate the success or failure of the script to the calling environment. If no exit status code is provided, Bash assumes a successful exit with a status code of zero.
How to ignore exit code Bash?
To ignore the exit code of a command, you can use the || operator in conjunction with a command that you want to execute regardless of the exit status. For example:
command_that_might_fail || true
In this syntax, command_that_might_fail
is the command whose exit code you want to ignore. If this command fails (i.e., exits with a non-zero status), the true command is executed, which has an exit status of zero. Consequently, the entire expression evaluates to a successful exit status.
What is exit code 5 in Bash?
In Bash, exit code 5 typically indicates that the program or script encountered an error related to improper usage or command-line arguments. However, the exact meaning of exit codes can vary between different programs or scripts. It’s a convention rather than a strict rule, so the specific interpretation of exit code 5 might depend on the context in which it’s used within a particular script or program.
What is exit 1 or 0 in Bash?
In Bash, exit code 0 typically signifies the successful completion of a command or script. When a command or script finishes executing without encountering any errors, it exits with an exit status of 0.
On the other hand, exit code 1 usually indicates that the command or script encountered some form of error during execution. It’s a convention to use exit code 1 to signal general errors, such as incorrect usage of a command, failure to perform a required operation, or unexpected conditions.
Related Articles
- Print and Handle Error with Bash Exit Code [Easy Guide]
- How to Handle Error with “trap ERR” in Bash? [Easy Steps]
- [Solved] “No such file or directory” Error in Bash
- [Fixed] “bad substitution” Error in Bash
- [SOLVED] /bin/bash^M: bad interpreter: No such file or directory
- [Fixed] “bash: syntax error near unexpected token” Error
- [Fixed!] “syntax error: unexpected end of file” in Bash
- [Solved!] Handling Error with TRY CATCH Block in Bash
<< Go Back to Bash Error Handling | Bash Error Handling and Debugging | Bash Scripting Tutorial