FUNDAMENTALS A Complete Guide for Beginners
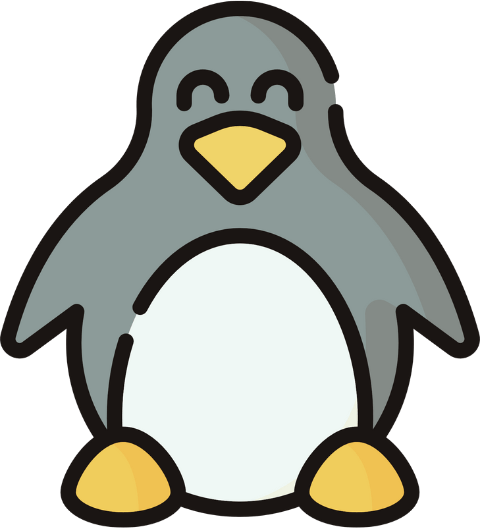
Error handling is one of the most important aspects of bash scripting. It is essential for maintaining the reliability and stability of applications. Sometimes handling errors can be difficult, but using print exit codes provides a simple way to deal with it. To handle errors in your bash script, you can check the exit code with $? variable. This will determine whether your last command’s execution is successful or not. This article will discuss how to print and handle errors with exit code in bash with a brief illustration. So let’s start!
What are Bash Exit Codes?
Bash exit code is a numeric value returned by a command or script after the execution of the program. These codes are used to indicate whether the program is executed successfully or not. In general, a bash exit code value of 0 indicates that the program executed successfully. On the other hand, a bash exit value of non–zero indicates different failure scenarios. Different values indicate different types of errors or conditions.
Here’s a table of some commonly encountered Bash exit codes along with their typical meanings:
Exit Code | Meaning |
---|---|
0 | Success |
1 | General Errors |
2 | Misuse of Shell Builtins |
126 | Permission Issues |
127 | Command Not Found |
128 | Invalid Argument to Exit |
130 | Interrupted by Ctrl+C |
255 | Exit Status Out of Range |
How to Check Exit Code of a Command in Bash?
You can retrieve the exit code of the most recent Bash command by using the special variable “$?“.
To find out the exit code of a command use the $? variable and print the value as given in the script below:
#!/bin/bash
# Execute a command
ls
# Capture the exit code
exit_code=$?
# Output the exit code
echo "Exit code: $exit_code"
#Execute another command
ls /home/LinuxSimply
#Store the exit code for 2nd command
exit_code=$?
#print the output
echo "Exit code of 2nd Command: $exit_code"
First, it runs the ls command, which lists all the files and folders in the current directory. Then, it stores the exit code of the previous command in the special variable $?
. The script then displays the exit code stored in $?
on the console using the echo command.
Next, the script runs another command, ls /home/LinuxSimply
, which tries to list the contents of a specific directory. The exit code of this second command is stored in the variable “exit_code”. Finally, the script prints the exit code of the second command.
As you can see from the image above, the ls command successfully executed, thus the exit code of it is 0. However, the $? variable only stores the output of the last executed command. Upon executing another command ls /home/LinuxSimply, the output of the previous command is lost. As the prompt says, the ls command can not access /home/LinuxSimply directory, which means it is a failed command, thus the exit code is a non–zero value.
How to Print and Handle Error with Exit Code in Bash?
To print and handle errors with exit codes in a Bash script, you can follow these steps:
- Execute Command: Begin by executing the command whose exit code you want to capture and handle.
- Capture Exit Code: Use the special variable “$?” immediately after executing the command to capture its exit code.
- Print Exit Code: Output the exit code to provide visibility into the success or failure of the command.
- Handle Error: Implement conditional logic to check the exit code and handle errors appropriately. This may include displaying error messages, performing cleanup tasks, or exiting the script with a specific exit code to indicate failure.
Here’s a practical example illustrating how to print and handle errors with exit codes in a Bash script:
#!/bin/bash
# Execute a command that may fail
ls
# Capture the exit code
exit_code=$?
# Print the exit code for visibility
echo "Exit code: $exit_code"
# Check the exit code and handle errors
if [ $exit_code -ne 0 ]; then
echo "Error: Command failed with exit code $exit_code"
# Perform error handling tasks here (e.g., logging, cleanup)
exit 1 # Exit the script with a non-zero exit code to indicate failure
else
echo "Success: Command executed without errors"
# Continue script execution or perform further actions
fi
This Bash script executes a command using the “ls” command as an example, with the potential for failure. After executing the command, it captures the exit code using the special variable $?
. Subsequently, the script prints the exit code to the console.
Following this, the script checks the exit code using a conditional statement. If the exit code is non–zero, indicating a failure, an error message is displayed. Conversely, if the exit code is zero, indicating success, a success message is printed.
Now, with the help of ls command, the provided bash script can list the file and folder of the current directory which is evident from the image given above. Thus the exit code is zero and it prints “Success: Command executed without errors”.
Checking Exit Codes from Piped Commands
In a pipeline, the exit status of the entire pipeline is determined by the exit status of its last command. However, suppose you want to access the exit status of each command within the pipeline. In that case, you need to use additional techniques such as the “PIPESTATUS” array or the “set -o pipefail” option.
1. Using PIPESTATUS Array to Handle Piped Command
To handle piped command, use the “PIPESTATUS” array, which holds the exit status of each command in the last pipeline executed. Here’s an example:
#!/bin/bash
# Execute commands with a pipe
ls /nonexist_directory | wc -l
# Capture the exit code of the first command in the pipeline
exit_code_ls=${PIPESTATUS[0]}
# Print the exit codes for visibility
echo "Exit code of ls: $exit_code_ls"
echo "Exit code of the whole command pipeline: $?"
This Bash script executes a pipeline of commands. It starts with the command ls /nonexist_directory
which tries to list the contents of a non-existent directory, followed by wc -l
which counts the number of lines generated by the first command’s output. After executing the pipeline, the script captures the exit code of the first command using the special variable ${PIPESTATUS[0]}
and prints it out to display its success or failure. Additionally, it prints the exit code of the entire command pipeline using $?
, which captures the exit status of the last command in the pipeline. Thus it determines the overall success or failure of the pipeline.
As the image suggests, the exit status of the first command ls /nonexist_directory failed because there is no file named nonexist_directory. Thus, the exit code returns 2 using the PIPESTATUS array. However, the exit code of the whole command ls /nonexist_directory | wc -l is 0, which means successful execution because the exit code returns based on the last command it executes. Since the wc -l is a valid and successful command in this case, the whole command status is 0.
Note: While using PIPESTATUS array, you will receive the exit status of each command if you have two commands in a pipeline. In such cases, PIPESTATUS[0]
will return the exit status of the first command, while $?
will return the exit value of the last command.
2. Using “set -o pipefail” to Handle Piped Command
Another approach is to use the “set -o pipefail” option, which causes a pipeline to produce a non–zero exit status if any command in the pipeline fails. Here’s how to use it:
#!/bin/bash
# Enable pipefail option
set -o pipefail
# Execute commands with a pipe
ls /nonexist_directory | wc -l
# Capture the exit code of the last command in the pipeline
exit_code=$?
# Print the exit code for visibility
echo "Exit code of the pipeline: $exit_code"
This Bash script enables the pipefail option using set -o pipefail
. This ensures that the exit status of the entire pipeline reflects the failure of any command within it. The script then executes a pipeline of commands separated by pipes (|). The pipeline consists of two commands. The first command, ls /nonexist_directory
, attempts to list the contents of a non-existent directory. The second command, wc -l
, counts the number of lines. After running the pipeline, the script captures the exit code of the last command (wc -l
) using the special variable $?
. Finally, the script prints the exit code of the entire pipeline to the console, along with a descriptive message. This provides insight into the success or failure of the pipeline as a whole.
As the image shows, The command ls /nonexist_directory failed. It is because the directory nonexist_directory does not exist. However, the command wc -l was executed successfully and returned a word count of zero. Finally, despite the successful execution of the last command, the exit code for the entire command line is 2 because the first command failed. Therefore, the entire command line is considered to have failed.
Conclusion
In conclusion, there are numerous situations to print and handle errors with an exit code in bash, depending on the situation, such as how you want to channel the error or what the possible alternatives are to rectify the issue. This article discusses some ways to do so, but if you feel that you are stuck on something, please feel free to share it with us. We will appreciate your concerns and provide solutions for them. Till then, happy Bash coding!
People Also Ask
How to print the exit code of a command in Bash?
To print the exit code of a command in Bash, you can use the special variable $? immediately after executing the command. This variable holds the exit code of the most recently executed command. Here’s how you can do it:
#!/bin/bash
# Execute a command
your_command
# Capture the exit code
exit_code=$?
# Print the exit code
echo "Exit code: $exit_code"
Here replace your_command
with the command line for which you want to check the exit status.
What is $? 0 in Bash?
$? is a variable in bash that represents the output of the most recent command. When the command ends, $? is set to its output. Usually, an output value of 0 means that the command completed successfully with no errors. The command is considered to be failed if it returns a non-zero value.
What is exit code 1 in Bash?
In bash, exit code 1 usually means that the most recent command has thrown an exception or failed to complete the job successfully. In bash, the exit code ranges from 0 to 255. A value of 0 indicates success and a value of non–zero indicates different failure conditions.
Related Articles
- How to Exit on Error in Bash Script? [6+ Methods]
- How to Handle Error with “trap ERR” in Bash? [Easy Steps]
- [Solved] “No such file or directory” Error in Bash
- [Fixed] “bad substitution” Error in Bash
- [SOLVED] /bin/bash^M: bad interpreter: No such file or directory
- [Fixed] “bash: syntax error near unexpected token” Error
- [Fixed!] “syntax error: unexpected end of file” in Bash
- [Solved!] Handling Error with TRY CATCH Block in Bash
<< Go Back to Bash Error Handling | Bash Error Handling and Debugging | Bash Scripting Tutorial