FUNDAMENTALS A Complete Guide for Beginners
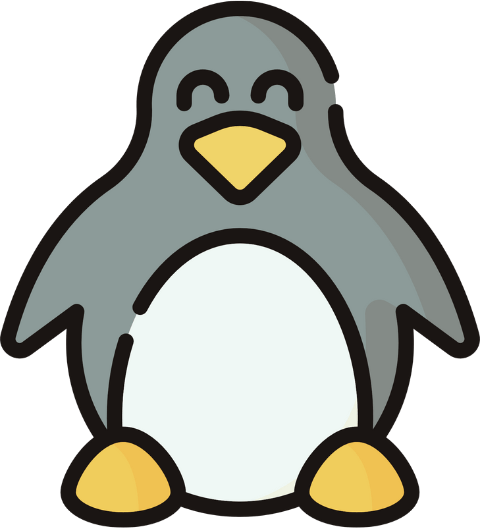
You can use the following methods to clear an array in Bash:
- Using the unset command:
unset <existing_array>
- Using the declare command:
declare -a <existing_array>
- Reassigning to an empty array:
existing_array=()
To clear an array in Bash is to remove all the elements of the array, essentially resetting it to an empty state. This is particularly useful in terms of reusing the array and memory management. This article discusses 6 effective methods of how to clear an array in Bash. It will mention commands such as unset and declare, standard array expressions as well as 2 iterative approaches using for and while loop to remove elements and clear an array in Bash. Furthermore, it will shed light on how to clear associative arrays in Bash.
1. Using the “unset” Command
The unset is very useful in removing the elements of a Bash array and making it clear. The syntax is unset array_name
. Let’s see the “unset” command in action below to clear an array in Bash:
#!/bin/bash
# the array to be clear
language=(c python Golang java)
#print the array
echo ${language[@]}
#clear the array using unset
unset language
#print the array again
echo "after clear elements: ${language[@]}"
#printing array length
echo ${#language[@]}
In the above bash script, after initializing an array language with 4 elements, the use of the unset language
command removes all the array elements; ending up clearing the array. As a result, in trying to print the array items, the script returns an empty screen and the length becomes zero.
2. Using the “unset” Command with Array Elements
The unset command is also capable of removing a specific array element using the syntax unset array[index]
where index is the position of the item to be removed. However, this can also be used to clear an array by removing all the elements using the syntax unset array[index]
for every array element like the following example:
#!/bin/bash
# Define an array
my_array=("element1" "element2" "element3")
# Print the array
echo "${my_array[@]}"
# Use unset with array elements
unset my_array[0]
unset my_array[1]
unset my_array[2]
# Print the array and length
echo "after clearance: ${my_array[@]}"
echo "length: ${#my_array[@]}"
In the above, the expression my_array=("element1" "element2" "element3")
creates an array with 3 items. Then the “unset” commands take each element individually and remove the elements to clear the array. Finally, the echo command outputs an empty line since the array has been successfully cleared.
3. By Reassigning an Empty Array with Array=() Expression
The expression array=() is generally used to declare an empty array in Bash making it ready to initialize later. However, this is also capable of clearing a Bash array. All you need to do is to reassign the same array of elements using array=()
and make it empty. Here’s an example:
#!/bin/bash
#array declaration
cars=(BMW Ferrari Accord)
#print elements
echo ${cars[@]}
#print array length
echo "original length: ${#cars[@]}"
#reassign to empty the array
cars=()
#print empty array and length
echo "after clear items: ${cars[@]}"
echo "empty array length: ${#cars[@]}"
In the above, after declaring an array called cars with 3 values, the cars=()
expression reassigns the same array but with no elements. This effectively clears the array and its elements (BMW Ferrari Accord). After printing its length and items, it’s obvious that the array has become clear.
4. Using the “declare -a” Command with Array=()
The command “declare -a” is useful to declare a Bash array explicitly. In addition, using “declare -a” in combination with “array=()” successfully clears an array in Bash. The full syntax to follow is: declare -a array=().
Here’s an example to clear an array in Bash using the syntax declare -a array=()
:
#!/bin/bash
#array declaration
brands=(BMW Puma Nokia)
#print elements
echo ${brands[@]}
#print array length
echo "original length: ${#brands[@]}"
#clear array using declare -a
declare -a brands=()
#print empty array and length
echo "clear array items: ${brands[@]}"
echo "clear array length: ${#brands[@]}"
The above Bash script creates the brands array using brands=(BMW Puma Nokia)
. Then, the declare -a brands=()
expression declares the array again and reassigns an empty array variable. Finally, after printing the array items and length again, the success of the clear operation becomes visible since echo "clear array items: ${brands[@]}"
and echo "clear array length: ${#brands[@]}"
returns empty screen with an array length of zero.
5. Using “for” Loop
The Bash for loop is an effective tool to access the array elements iteratively. Now, by using the syntax for ((index=0; index<$length; index++))
, it is possible to access each element and remove them using unset my_array[$index]
to clear the array as a result. Here’s an example:
#!/bin/bash
# Define an array
my_array=("element1" "element2" "element3" "element4" "element5")
#print array
echo "${my_array[@]}"
# array length for iteration
length=${#my_array[@]}
# Use a for loop to clear array
for ((index=0; index<$length; index++)); do
unset my_array[$index]
done
# Print the modified array
echo "clear array elements: ${my_array[@]}"
echo "array length: ${#my_array[@]}"
In the above, my_array=("element1" "element2" "element3" "element4" "element5")
declares my_array with 5 elements. Then the loop for ((index=0; index<$length; index++))
iterates over the entire length of the array and clears each item at a specified index using unset my_array[$index]
to clear the array which is evident after printing the length and items again at the end.
6. Using “while” Loop
Apart from using the for loop, Bash while loop is also a powerful tool to clear an array in Bash. Here’s how:
#!/bin/bash
# Define an array
items=("elem1" "elem2" "elem3" "elem4" "elem5")
#print array
echo "before clear: ${items[@]}"
# Determine the length of the array
array_length=${#items[@]}
# Use a while loop to unset elements until the array is empty
while [ $array_length -gt 0 ]; do
unset items[$((array_length - 1))]
array_length=$((array_length - 1))
done
# Print the modified array
echo "after clear: ${items[@]}"
In the above, after initializing the array called items with 5 elements, the loop while [ $array_length -gt 0 ]
iterates each array element and unset items[$((array_length - 1))]
removes them to effectively clear the array.
Clear a Range of Elements in Bash
As of now, the previous methods have discussed how to clear Bash arrays by removing all the elements. However, clearing a range of specific elements is also possible using for loops which might become a necessity at times. Look at the example below to clear a range of elements in Bash:
#!/bin/bash
# Define an array and print items
my_array=("element1" "element2" "element3" "element4" "element5")
echo " original array state: ${my_array[@]}"
# Range of indices to clear elements (e.g., indices 1 to 3)
start_index=1
end_index=3
# Use a loop to unset elements in the specified range
for ((i = $start_index; i <= $end_index; i++)); do
unset my_array[$i]
done
# Print the modified array
echo " specific clear state: ${my_array[@]}"
In the above, an array called my_array is declared using the code my_array=("element1" "element2" "element3" "element4" "element5")
. Then the loop for ((i = $start_index; i <= $end_index; i++))
iterates from index 1 to 3 (defined by start_index and end_index) and removes them using the unset command. This clears specific elements from my_array and the rest of the items are printed using the echo command with echo " specific clear state: ${my_array[@]}"
.
Clear an Associative Array in Bash
All the methods above have displayed how to clear an indexed array in Bash, to be more specific. In addition, it is possible to clear an associative array in Bash simply by using the unset command. Here’s an example:
#!/bin/bash
#declare an associative array
declare -A assoc_array
#value insertion to the array
assoc_array["fruit"]="apple"
assoc_array["vegetable"]="carrot"
assoc_array["drink"]="water"
#print the array values
echo "values: ${assoc_array[@]}"
#print array length
echo "length: ${#assoc_array[@]}"
#clear associative array using unset
unset assoc_array
echo "after clearing the array:"
#print the array values
echo "values: ${assoc_array[@]}"
#print array length
echo "length: ${#assoc_array[@]}"
In the above, the command declare -A assoc_array
explicitly declares a Bash associative array named assoc_array and then populates it with 3 key-value pairs. Then the command unset assoc_array
makes the array empty which, in turn, clears the entire array. Finally, printing the items and length of the array twice denotes the successful clearing of the assoc_array.
declare -A assoc_array=()
to clear a Bash associative array.Conclusion
Clearing a Bash array is useful in terms of populating it with new values but without deleting the entire array which opens creative possibilities for data integration and handling. This article sheds light on 6 simple methods for how to clear an array in Bash. It talks about commands like unset and declare to smartly clear an array. Furthermore, it mentions for loop, and while loop to clear a Bash array with ease. In addition, it shows how to clear a range of selected elements from arrays including clearing associative arrays in Bash. Hope this article empowers the users and developers to hone this skill of clearing arrays in Bash elevating scripting mastery.
Frequently Asked Questions
How to remove an element from an array completely in Bash?
To remove an element completely from an array, use the unset command with the syntax unset array[index]
. It will remove the item at index from the array. For instance, to remove the item Accord from the array cars=(BMW Ferrari Accord)
, use the syntax unset cars[2]
.
Can I make a Bash array empty?
Yes. Use the syntax array=() to reassign an existing array and set it to empty. For instance, consider the array food=(apple banana cream)
. To make this array empty use the syntax food=()
which will remove all the elements (apple banana cream) and make the array empty.
How to clear a Bash array?
To clear a Bash array, use the unset command followed by the array name you intend to clear in the format unset array_name. This will remove all the elements and make the array clear. For example, to clear the array cars=(BMW Ferrari Accord)
, use the syntax unset cars
.
Is it possible to clear a Bash array without the “unset” command?
Absolutely. To clear an array in Bash without the unset command, use the declare -a command with the array reassignment expression array=() in the full syntactical format declare -a array=()
. This clears the array by removing its elements.
Can I clear a Bash associative array using the declare command?
Yes, you can use the declare command in the format declare -A associative_array=() to clear the associaitve_array. For instance, to clear the associative array “declare -A assoc_array=( [“key1″]=”value1” [“key2″]=”value2” [“key3″]=”value3″ )”, use the code declare -A assoc_array=()
.
Related Articles
- How to Convert a JSON Array into Bash Array [5 Methods]
- Master Bash Array of Strings with Spaces [Complete Guide]
- How to Convert String into Array in Bash [8 Methods]
- How to Append to an Array in Bash? [4 Easy Methods]
- How to Filter an Array in Bash? [8 Methods]
- How to Copy an Array in Bash [6 Simple Methods]
- How to Reverse an Array in Bash? [8 Easy Methods]
- How to Slice an Array in Bash [10 Simple Methods]
- Iterate Through a Bash Array Using “foreach” Loop [5 Examples]
- How to Check If an Array is Empty in Bash? [5 Methods]
<< Go Back to Array Operations in Bash | Bash Array | Bash Scripting Tutorial