FUNDAMENTALS A Complete Guide for Beginners
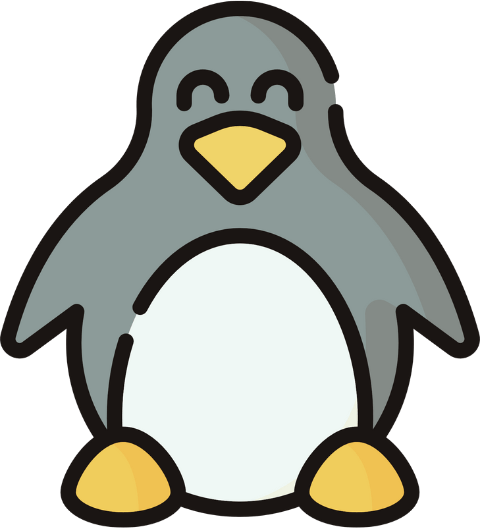
An empty array in Bash is an array data structure that contains no data elements or that’s already declared but with no initialized elements. It’s important to verify whether the array is empty especially when it comes to adding elements to the array. This article discusses 5 methods of how to check if an array is empty in Bash. Moreover, it sheds light on methods to declare an empty array in Bash and to make an existing array with elements empty in Bash.
5 Methods to Check If an Array is Empty in Bash
Checking whether an array in Bash is empty or not is beneficial in terms of appending elements and working with data within Bash arrays. This section will mention an in-depth analysis of 5 useful methods to check if a Bash array is empty. It will mention array expressions and commands like wc and grep along with if-else conditionals to check if an array is empty in Bash.
1. Using ${#array[@]} Expression
While working with arrays, it might become pivotal to check if the array is empty in Bash before making any necessary manipulation. You can do that by checking the length of the specified array with an if-else conditional statement. The full syntax is if [ ${#array[@]} -eq 0 ]
that checks if the array length equals 0 and executes the corresponding if/else block. Here’s an example:
#!/bin/bash
# array creation
cards=()
# check if array is empty
if [ ${#cards[@]} -eq 0 ]; then
echo "The array is empty"
else
echo "The array is not empty"
fi
In the script, the cards=()
expression creates the empty Bash array cards, Then the if statement checks if the array is empty using the syntax if [ ${#cards[@]} -eq 0 ]
and prints the message of the if block if the array length is 0. Otherwise, the else block is executed.
Note: you can also use the conditional if [ ${#arr[@]} -gt 0 ]
to check if the array above is empty or not where the expression checks if the array length is greater than zero to decide if it’s empty.
2. Using ${#array[*]} Expression
The asterisk (*) sign can also be employed within the expression ${#array[*]} to find the array length. Just use it with the if statement in the format if [ ${#array[*]} -gt 0 ] that checks whether the Bash array is empty or not and decides whether to execute the if block or the else block.
Here’s an example to check if a Bash array is empty using if [ ${#array[*]} -gt 0 ]
:
#!/bin/bash
arr=("hello" "world")
if [ ${#arr[*]} -gt 0 ]
then
echo "array Not empty"
else
echo "array empty"
fi
arr2=() #empty array
if [ ${#arr2[*]} -gt 0 ]
then
echo "array Not empty"
else
echo "array empty"
fi
In this script, the expression arr=("hello" "world")
declares a Bash array with 2 elements. Then if [ ${#arr[*]} -gt 0 ]
checks if the array length is greater than 0. Since the array length is 2, the if block executes the message “Not empty”. On the other hand, the 2nd array arr=()
is empty, and since if [ ${#arr2[*]} -gt 0 ]
finds its length to be 0, the message empty of the else block is executed.
The script outputs “array Not empty” for the first array & “array empty” for the second array.
3. Using the “-z” Flag
The -z flag with the if conditional operator within the syntax if [[ -z "${array[@]}" ]]
checks if a string is empty or not. In Bash arrays, the -z option with the expression ${array[@]} checks if the entire array (which is technically a string of strings) is empty or not.
To check if an array is empty using the -z flag, see the below Bash script:
#!/bin/bash
array1=()
array2=('1' '23' '4' '56')
#print the arrays
echo "array1: ${array1[@]}"
echo "array2: ${array2[@]}"
#check if array1 is empty
if [[ -z "${array1[@]}" ]]; then
echo "array1 is empty"
else
echo "array1 is full"
fi
#check if array2 is empty
if [[ -z "${array2[@]}" ]]; then
echo "array2 is empty"
else
echo "array2 is full"
fi
In the script, the array1=()
and array2=('1' '23' '4' '56')
respectively declares 2 arrays (one empty and another with elements). Then the expression if [[ -z "${array1[@]}" ]]
checks and executes the message array1 is empty since it has no elements. On the other hand, array2 has 4 elements, and thus the script prints the message that the else block array2 is full.
As you can see, the -z option finds that array1 is without elements, and the echo command prints that array1 is empty. On the other hand, array2 is full of elements and thus the message array2 is full printed on the screen.
4. Using the “wc” Command
Apart from using the standard expressions and syntax, several commands are also capable of checking whether an array is empty or not in Bash and this example will discuss how to do that using the wc command. The expression `echo ${arr[@]} | wc -w`
counts the number of words (array elements in this case) and decides if the array is empty or not.
Here’s how to use the wc command to check whether an array in Bash is empty or not:
#!/bin/bash
arr=("hello" "world" "universe")
#print array
echo ${arr[@]}
#check if array is empty
result=`echo ${arr[@]} | wc -w`
if [ $result -gt 0 ]
then
echo array Not empty
else
echo array empty
fi
arr2=()
#print array
echo ${arr2[@]}
#check if array is empty
result=`echo ${arr2[@]} | wc -w`
if [ $result -gt 0 ]
then
echo array Not empty
else
echo array empty
fi
The above script first creates an array named arr with 3 elements using arr=("hello" "world" "universe")
. Then in the expression, `echo ${arr[@]} | wc -w`
, the wc command takes the output of echo ${arr[@]} as input with the pipe operator and the -w option tells wc to count the number of words in the provided input stream and store it into the result variable. Since the final result is not zero, the if block is executed. Otherwise, the else block is executed which is evident in the case of the 2nd array called arr2 which happens to be empty.
The terminal output above states that the wc command counts the number of elements to check that the first array is not empty successfully. Contrarily, the second array is empty which is also found by the wc command.
5. Using the “grep” Command
The grep command in Linux is another useful tool to check if an array in Bash is empty or not. Usually, the grep command is a powerful tool to filter search queries based on different types of keywords such as strings, regular expressions, or any particular pattern, etc. Since array elements are also strings (words separated by spaces), the grep command is capable of checking if an array is empty. Here’s how:
#!/bin/bash
arr=("hello" "world" "multiverse")
result=$(echo ${arr[@]} | grep -o " " | grep -c " ")
echo ${arr[@]}
#check if array is empty using grep command
if [ $result -gt 0 ]
then
echo "Not empty"
else
if [ ! -z "${arr[0]}" ]
then
echo "Not empty"
else
echo "empty"
fi
fi
arr2=() #empty array
result=$(echo ${arr2[@]} | grep -o " " | grep -c " ")
echo ${arr2[@]}
#check if array is empty using grep command
if [ $result -gt 0 ]
then
echo "Not empty"
else
if [ ! -z "${arr2[0]}" ]
then
echo "Not empty"
else
echo "empty"
fi
fi
In the above script, after declaring the array named arr with 3 elements using arr=("hello" "world" "multiverse")
, the expression $(echo ${arr[@]} | grep -o " " | grep -c " ")
expands the array and the grep commands check for the number of blank spaces by taking blank space as a pattern to match using grep -o and the grep -c command gets the count of the occurrences matching that pattern and stores them into the result variable. The result is 1 less than the number of elements of the array. Now, if the result is greater than zero then the array is not empty, and the if block is executed. In addition, if the result is 0, it means the array has only 1 element. In this case, the primary else block is executed and checks for a non-zero string using if [ ! -z "${arr[0]}" ]
within the nested if-else statement and finally decides whether to execute the if or the else block.
On the other hand, since the second array named arr2 is empty, the result will be 0, after further checking by if [ ! -z "${arr2[0]}" ]
, the else block will execute the message “empty”.
The Linux grep command decides if the array in Bash is empty or not by calculating the number of spaces in between the array elements.
Declare an Empty Array in Bash
To declare an empty array, simply use the array name and assign it an empty parenthesis like the syntax array_name=()
. Here’s a complete example:
#!/bin/bash
#Array creation with empty ()
cards=()
#printing array length to the prompt
echo "length:${#cards[@]}"
In this script, the cards=()
expression declares an empty array called cards. Finally, in printing the length using the echo command with the syntax echo "length:${#cards[@]}"
, the program outputs 0 which verifies that the empty Bash array has been declared.
The above image states that the length of zero denotes the declaration of an empty Bash array.
Note: In addition, the commands declare -a array_name=()
and declare -a array_name
also lets you declare an empty array like the above.
Empty an Existing Array in Bash
Apart from the declaration above, it is possible to make an extant array with elements empty. One common method to make an array empty is to use the syntax array_name=()
. This simply reassigns the array and makes it empty by removing all the existing elements. Here’s how:
#!/bin/bash
#array declaration
cars=(BMW Ferrari Accord)
#print elements
echo ${cars[@]}
#print array length
echo "original length: ${#cars[@]}"
#reassign to empty the array
cars=()
#print empty array length
echo "empty array length: ${#cars[@]}"
In the script, the cars=(BMW Ferrari Accord)
expression creates the array cars, and printing its length and elements denotes that it contains 3 elements. Then the expression cars=()
merely reassigns the same cars array but with no elements. This effectively removes array elements making it an empty array.
declare -a cars=()
to make the existing array empty.Conclusion
To sum up, this article discusses the 5 effective methods of how to check if an array is empty in Bash. It also discusses the methods on how to declare an empty array and how to make an entire Bash array empty. Hope this guide clears your vision on Bash empty arrays ensuring smooth handling and validation of arrays based on the array’s emptiness.
People Also Ask
Can I declare an empty Bash array?
Yes, you can. Use the declare command within the syntax declare -a array. For instance, declare -a numbers
will declare an empty Bash array called Numbers.
How to set an empty array elements to zero?
To set empty array elements to zero in Bash, you can iterate the array and assign zero to all indices like the following:
#!/bin/bash
# Define an array with some elements and empty indices
myArray=()
# Loop through the indices and set empty elements to zero
for ((i=0; i<5; i++)); do
if [[ -z ${myArray[$i]} ]]; then
myArray[$i]=0
fi
done
# Print the updated array
echo "${myArray[@]}"
Output: 0 0 0 0 0
What’s the best way to clear all the elements of an array in Bash?
The best way to clear all the elements of a Bash array is to reassign it to an empty array using the syntax array_name=(). Take the array, cars=(BMW Bently Civic)
, for instance. The expression cars=()
will create an empty array cars and clear all the elements (BMW Bently Civic) from it.
Can I iterate through an empty array in Bash?
Absolutely. You can use for loop to iterate over an empty Bash array. However, the program will not return any output since the array contains no elements and the loop body will not be executed. Look at the following example:
#!/bin/bash
# Initialize an empty array
myArray=()
# Iterate through the empty array (won't execute as the array is empty)
for element in "${myArray[@]}"; do
echo "Element: $element"
done
echo "done"
Output: done since the array is empty and the loop body is not executed for any iteration.
How to remove an element from a Bash array?
To remove an element from an array in Bash, use the syntax unset array[index]. This will remove the element located at the specified index from array. For instance, to remove the element two from the array digit=(one two three)
, use the syntax unset digit[1]
.
Can I empty an existing array in Bash using the unset command?
Yes, you can. To empty an existing array of elements with the unset command, follow the syntax unset array_name. This will remove all the elements of array_name and make it empty. For instance, to empty the array named food=(burgers fries shakes)
, use the command unset food
.
How to create empty associative arrays in Bash?
To create an empty associative array, use the declare command with the -A flag employing the syntax declare -A associative_array_name. For example, the command declare -A employee
will create an empty associative array named employee.
Can I add elements to an empty array?
Absolutely. Just use the syntax array+=(element to add) to add an element to an empty array. In addition, to add multiple elements, use the syntax array+=(element1 element2 elementN). For example, in the array cars=()
, the syntax cars+=(BMW)
adds the single element BMW to the array while the expression cars+=(BMW Civic)
adds multiple elements BMW and Civic to the cars array.
Related Articles
- How to Convert a JSON Array into Bash Array [5 Methods]
- Master Bash Array of Strings with Spaces [Complete Guide]
- How to Convert String into Array in Bash [8 Methods]
- How to Append to an Array in Bash? [4 Easy Methods]
- How to Clear an Array in Bash [6 Methods]
- How to Filter an Array in Bash? [8 Methods]
- How to Copy an Array in Bash [6 Simple Methods]
- How to Reverse an Array in Bash? [8 Easy Methods]
- How to Slice an Array in Bash [10 Simple Methods]
- Iterate Through a Bash Array Using “foreach” Loop [5 Examples]
<< Go Back to Array Operations in Bash | Bash Array | Bash Scripting Tutorial