FUNDAMENTALS A Complete Guide for Beginners
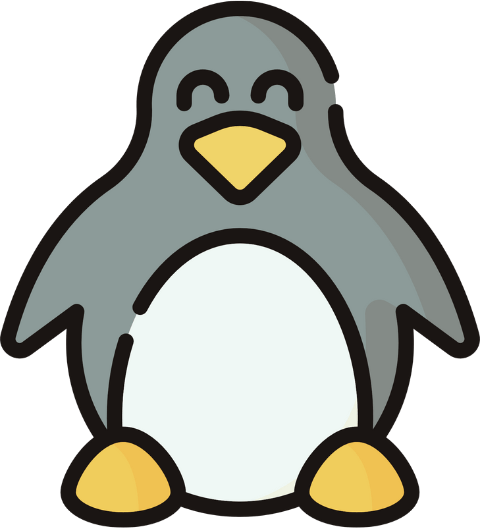
A Bash array is a dynamic data structure that stores data values of diversified types as its elements. For a successful array manipulation to avail efficient data storage, and management, it’s crucial to know about the different array operations involved within the arrays in the Bash scripting language.
This article discusses the concepts of 7 essential Bash array operations from print operations, and element insertion operations to even array removal operations. It also mentions necessary commands and Bash scripts for seamless array operations.
1. Print Operation
The print operation is the process of displaying the array elements in the terminal. The echo command with the length expression syntax ${array[@]} or ${array[*]} prints the entire bash array on the screen.
Here’s how to print an array in Bash employing the syntaxecho ${array[@]}
:
#!/bin/bash
#Array creation
metallica=(james lars kirk trujilo)
#printing array length to the terminal using both expressions
echo "the array elements printed using \${metallica[@]}: ${metallica[@]}"
echo # for adding extra spaces between the outputs
echo "the array elements printed using \${metallica[*]}: ${metallica[*]}"
${metallica[@]}
and ${metallica[*]}
access the array metallica with 4 elements. Finally, the echo command displays all the array items in a single line to the prompt.Print the Length of an Array in Bash
The length of a Bash array is the total number of elements present in that array. The process to retrieve the array length is very straightforward in Bash. Just use the length expressions ${#array[@]} or ${#array[*]} with the echo command to display the length of the array. The hash (#) tells the expressions to expand the array and find the number of elements which is the array length.
Here’s an example to print the Bash array length using the syntaxecho ${#array[@]}
:
#!/bin/bash
#Array creation
cards=(hearts diamonds spades clubs)
#print the array
echo "elements: ${cards[@]}"
#printing array length to the prompt
echo "length:${#cards[@]}"
${#cards[@]}
gets the array length. On an end note, the commandecho "length:${#cards[@]}"
prints the array length which is 4. Print the Length of Nth-Indexed Element in an Array
To print the length of only a specific array element, the printing operation expression ${#array[@]} is enough. Just replace the @ sign with the index number, say, N, of the element to get the length of the element. The full syntax is:echo ${#array[N]}
.
Below is a Bash scripting example to print the length of the Nth-indexed element:
#!/bin/bash
#Array creation
cards=(hearts diamonds spades clubs)
#printing the length of the elements “diamonds”
echo ${#cards[1]}
The above Bash script creates an array named cards with 4 elements. Then theecho ${#cards[1]}
syntax fetches the length of the string stored in the index 1, which is diamonds, and outputs the length of that element string, “diamonds”, which is 8 characters long.
2. Append Operation
To append to an array is to add a new element to an existing Bash array. By default, the append operation adds a new element to the array at the very end. The syntax to append an array involves the array name and the element to append with the+=
operator in between like the following:
your_array+=(element_to_append)
Let’s see an example of the array append operation:
#!/bin/bash
#The main array
distros=(ubuntu kali arch)
#prints array with 3 existing elements
echo "array before appending: ${distros[@]}"
#new value to append
distros+=(redHat)
#Prints array with newly added element redHat
echo "array after appending: ${distros[@]}"
In the script, the array named distros initially had 3 elements. Then, using the expressiondistros+=(redHat)
, appends the new element redHat to the array. As a result, the distros array now has 4 elements which is verified by printing the array using the standard echo command.
3. Insert Operation
The insert operation in a Bash array is useful since it lets the element be added at a specific position of the array. It is adding but with an index assignment that leverages the element insertion at the specified place. The general syntax to insert an element into an array is:array_name[index_number]=element_to_insert
Here’s an example of how to insert elements to specific positions of an array in Bash:
#!/bin/bash
#The primary array
bd_bands=(artcell rockStrata warfaze)
#inserting elements into the array at different positions
bd_bands[3]=vibe
bd_bands[8]=powersurge
# loop through the indices and print elements
for position in ${!bd_bands[@]};
do
echo "band ${bd_bands[$position]} is at index: $position"
done
In this script, after defining the array bd_bands with 3 elements [positioned at index 0,1, 2 respectively.], the expressionsbd_bands[3]=vibe
andbd_bands[8]=powersurge
insert 2 more elements into that array at indices 3 and 8 (position 4 and position 9 since the bash array is 0-indexed). Then, the loopfor position in ${!bd_bands[@]}
iterates over the array indices and displays the elements along with their indices employing the expressionecho "band ${bd_bands[$position]} is at index: $position"
.
4. Copy Operation
To copy an array in Bash is very simple. Just expand the array of elements and store them in a new array-type variable. Here’s how:
#!/bin/bash
#declare array
Unix=('Debian' 'Red hat' 'Ubuntu' 'Suse' 'Fedora' 'UTS' 'OpenLinux')
#array expansion and copy
Linux=("${Unix[@]}")
#print the 2 Array
echo "1st array: ${Unix[@]}"
echo
echo "copied array: ${Linux[@]}"
Linux=("${Unix[@]}")
expands the array elements of Unix. It copies the whole array to the new array-type variable Linux. In the end, the echo command prints both the arrays Unix and Linux to verify the copy operation.5. Bash String to Array Operation
In Bash, it is possible to convert a string into an array of elements. The easiest way to do this is by using the IFS (Internal Field Separator) to split the whole string based on a specific delimiter and store them as elements in a Bash array. Here’s an example:
#!/bin/bash
# Your string
my_string="Apple,Orange,Banana,Grapes"
#print the string
echo "$my_string"
# Set the delimiter
IFS=',' read -r -a my_array <<< "$my_string"
echo
# Print array elements
for element in "${my_array[@]}"
do
echo "$element"
done
my_string="Apple,Orange,Banana,Grapes"
with comma-separated entities. The IFS=','
sets the delimiter to a comma (,). When theread
command is used with the-a
flag, it splits my_string at every comma and stores the individual parts as elements of the array my_array usingIFS=',' read -r -a my_array <<< "$my_string"
. Finally, the for loop iterates over the elements to print them on the prompt.6. Search and Replace Operation
In this operation, you can search for a specific pattern and substitute it with another value within a Bash array. Using the syntax${array[@]/pattern/replacement}
, it’s possible to find the pattern and replace it with the value of replacement.
Here’s an example to search and replace within an array:
#!/bin/bash
Unix=('Debian' 'Red hat' 'Ubuntu' 'Suse' 'Fedora')
#print the array
echo "w/o replacement: ${Unix[@]}"
#replace element
replace=${Unix[@]/Ubuntu/KALI}
echo "with replacement: $replace"
${Unix[@]/Ubuntu/KALI}
performs a pattern substitution. First, it searches for the element Ubuntu inside the array Unix. Upon finding the element, it replaces it with KALI. The echo command twice prints the Unix array to reflect on the changes of the search and replace operation. 7. Delete Operation
The delete operation lets the user remove any specified array element from the array. To remove array elements, use the unset command followed by the array_name with the index assignment operator=
and index number of the element to remove.
Here’s how to delete an array element in Bash using theunset
command:
#!/bin/bash
# the array
shoes=(adidas puma nike)
#print array
echo "before removal: ${shoes[@]}"
# Deleting nike from the array
unset shoes[1]
#printing the array after removal of the element nike
echo "after removal: ${shoes[@]}"
Delete an Entire Array
Apart from deleting specific array elements, the unset command is capable of deleting an entire array of elements in Bash and makes the array length zero. The syntax is the unset command followed by the array name to deleteunset array_2_delete
Let’s hone the process of an entire array deletion operation with an example:
#!/bin/bash
# the array to be deleted
language=(c python Golang java)
#print the array
echo ${language[@]}
#deleting the entire array
unset language
#printing array length
echo ${#language[@]}
unset language
deletes the entire array named language with 4 elements. As a result, in printing the array length, the output is zero denoting the deletion operation of en entire Bash array. Conclusion
This article sheds light on 7 popular yet essential array operations in Bash. It mentions the concepts of array printing, element addition, insertion, and deletion along with some advanced array operations such as converting string to array and search and replace operations providing hands-on examples. Hope this article boosts your confidence in array handling to efficiently avail data management within Linux systems using Bash scripts.
People Also Ask
How to clear an array in Bash?
To clear an array in Bash, use the unset command followed by the array name. For example, to clear the array cars=(bmw bugati)
, use the command unset cars
in your script. Upon executing the script, it will remove all the elements to clear the array. In addition, you can reassign the array using the syntax cars=()
, for example, to clear the bash array.
How to print the last element of an indexed array in Bash?
To print the last element of an indexed array in Bash, use the concept of negative index -1 in the syntactical format ${your_array[-1]}
to extract the last element and prefix it with the echo command to display on the screen. For instance, to print the last element of the array food=(pizza burger orange)
, use the command echo ${food[-1]}
. The output will be “orange”.
Can I print array elements in newlines in Bash?
Yes. To print the Bash array elements in a new line, use the printf command with the newline separator character \n. For instance, to print the array elements, my_array=("Apple" "Orange" "Banana" "Grapes")
, in new lines, use the expression printf "%s\n" "${my_array[@]}"
after declaring the array.
Output:
Apple
Orange
Banana
Grape
How to print a specific range of array elements in Bash?
To print a specific range of elements, use the c-style for loop in the format for ((i = start_index; i <= end_index; i++))
to iterate over a range of elements in a Bash array. Here, start_index is the starting position and end_index is the last position to iterate. For instance, to iterate from the 2nd element to 4th element of the array num=(1 2 3 4 5 6 7)
:
num=(1 2 3 4 5 6 7)
start_index=1
end_index=3
# C-style for loop
for ((i = start_index; i <= end_index; i++))
do
echo "${num[i]}"
done
OUTPUT:
2
3
4
How can I merge two arrays in Bash?
You can merge two Bash arrays by expanding them and storing them into a new array. It’s called array concatenation. For example, to merge the 2 arrays first=(virat Tamim)
and last=( Iqbal kohli)
into a new array named players, use the expression players=("${first[@]}" "${last[@]}")
.
Output: virat Tamim Iqbal kohli
What is a Bash array?
A Bash array is a data structure used to store information in an indexed way. The indexed array in Bash has numbered indices while the associative arrays have string indices called keys. Unlike other programming languages, Bash arrays can store elements of various data types.
How to echo a Bash array?
To echo a Bash array, use the length expression command echo ${array[@]}
. For example, the command is echo ${nums[@]}
to echo an array nums. You can also use length expression ${nums[*]} prefixing with the echo command.
Related Articles
- How to Convert a JSON Array into Bash Array [5 Methods]
- Master Bash Array of Strings with Spaces [Complete Guide]
- How to Convert String into Array in Bash [8 Methods]
- How to Append to an Array in Bash? [4 Easy Methods]
- How to Clear an Array in Bash [6 Methods]
- How to Filter an Array in Bash? [8 Methods]
- How to Copy an Array in Bash [6 Simple Methods]
- How to Reverse an Array in Bash? [8 Easy Methods]
- How to Slice an Array in Bash [10 Simple Methods]
- Iterate Through a Bash Array Using “foreach” Loop [5 Examples]
- How to Check If an Array is Empty in Bash? [5 Methods]
<< Go Back to Bash Array | Bash Scripting Tutorial