FUNDAMENTALS A Complete Guide for Beginners
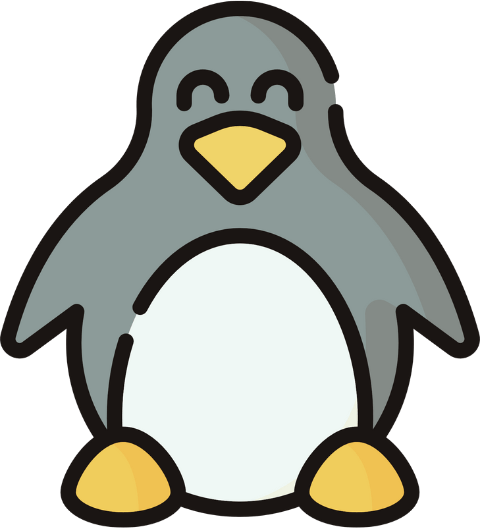
Bash read array is the process of appending an array with data obtained from various sources such as files, command outputs, or standard inputs within scripts. This functionality lets fill up an empty array with values or even add elements into an array along with the existing ones making data processing more effective.
You can use the following 3 methods to read an array in Bash:
- Using read Command:
read -a <your_array>
- Using readarray Command:
readarray -t your_array < sample.txt
- Using mapfile Command:
mapfile -t your_array < sample.txt
The remaining part of the article will walk you through the in-depth discussion of these 3 methods to read arrays in Bash.
1. Using “read” Command
The read command in Bash scripting is a primary tool for reading an array with contents from user inputs or any external resources. With the -r
option and while loop, it accesses the file contents and reads them into the specified array using the syntax, array+=($file_name)
. Here, the += is the “compound assignment operator” for arrays in Bash which appends elements to the end of the “array”.
Let’s have a look at a complete example of how to read an array using the read -r command:
#!/bin/bash
# Initialize an empty array
teams=()
# Read lines from file into the array
while IFS= read -r line; do
teams+=("$line")
done < "sample.txt"
# Print the array elements
echo "the elements of array: ${teams[@]}"
The script begins by initializing an empty array called teams. It then proceeds to read lines from a file named sample.txt while setting the Internal Field Separator (IFS) to a null value and ensuring that leading and trailing whitespace is preserved (IFS= read -r line)
. For each line read, it’s appended as a new element to the teams array using teams+=("$line")
. Finally, it displays all elements stored in the array using "the elements of array: ${teams[@]}"
.
A. Read From Command Output
In Bash, one common operation is to capture the output of a command and redirect it to an array for storage and further manipulation. The read command seamlessly does this by accepting the specified command output as its input using the process substitution with a while loop for element-wise iteration and reading into arrays.
Look at the following example to read a Bash array from a command output using the read command:
#!/bin/bash
# declare empty array
Arr=()
# Command whose output to store in the array
command_output=$(pwd) # Replace this with your desired command
# Read command output into the array
while IFS= read -r line; do
Arr+=("$line")
done <<< "$command_output"
# Print the array elements
echo "the array element: ${Arr[@]}"
echo
#print the array details
echo "the array details:"
declare -p Arr
After declaring an empty array Arr, the script stores the output of the pwd command into a variable named command_output using command_output=$(pwd)
. Using a while loop, it reads each line from command_output and appends it as an element to the Arr array. Finally, it displays all elements stored in the array using echo "the array element: ${Arr[@]}"
. Also, it uses the declare -p Arr
command to show array details to further verify the process of reading the Bash array from the command output.
B. Read From Standard User Input
The read command is also capable of taking user-defined input to read into a Bash array. The syntactical format, read -a <array_name>, uses whitespace as the default delimiter. When users insert elements using whitespaces, the read command differentiates and stores them into the specified array with contiguous indices. Here’s how:
#!/bin/bash
#declare an array
declare -a arr
#take std input from user
echo "enter whitespaced elements. Press Enter to finish.."
read -a arr
echo
#wait for 2 second to reflect changes
echo "The entered elements are:"
sleep 2
#print elements after 2 seconds
echo "${arr[@]}"
Here, the Bash script above initializes an array arr with declare -a arr
. Then it uses the command read -a arr
to prompt the users to input whitespace-separated elements. After that, upon hitting the ENTER button, it reads them into arr by storing them at contiguous indexed positions. Next, it takes a pause for 2 seconds using sleep 2
to provide a clear separation between the input prompt and output of displayed elements. Finally, it prints the user inputs as array elements in a single line using echo "${arr[@]}"
.
2. Using “readarray” Command
The readarray command empowers Bash scripts to efficiently read an array with contents from external resources such as files or standard user input. The common syntax is readarray -t your_array < data.txt which takes the contents of the data.txt file as input and reads your_array with the contents. The flag -t denotes readarray to trim the trailing newline characters from the lines to read into an array.
Here’s an example to read into a Bash array from a file using the command readarray -t:
#!/bin/bash
#declare an empty array
declare -a myArr
#read into array from sample.txt
readarray -t myArr < sample.txt
#print all the elements
echo "the elements are: ${myArr[@]}"
The above script uses the readarray -t myArr < sample.txt
command to read lines from the sample.txt file and read the array myArr with those contents. Finally, it prints the file contents as array elements using the command echo "the elements are: ${myArr[@]}"
.
The readarray command reads the array elements (Argentina France Moroccro Netherlands) from the sample.txt file.
A. Read From Command Output
In this case, Bash passes the standard output of a command as an input to the readarray command which stores the final output as elements in the declared array.
Here’s an example to read the contents from a standard command output into a Bash array:
#!/bin/bash
#read the specified command output into “the numbers” array
readarray -t numbers < <(seq 5)
#print all the elements
echo "the elements are: ${numbers[@]}"
echo
#print specific elements
echo "first one: ${numbers[0]}"
echo "last one: ${numbers[-1]}"
In the script above, the readarray command uses the output of the (seq 5) command and reads the array numbers with those contents (1 2 3 4 5) in the format readarray -t numbers < <(seq 5)
. where < <(seq 5) redirects its output 1 2 3 4 5 as standard input to readarray. It then prints the array elements using echo "the elements are: ${numbers[@]}"
to verify the reading process. Additionally, the script prints the 1st and last array values.
As you can see, the script takes the output of the (seq 5) command as input and reads into the numbers array with values 1 2 3 4 5 which are then displayed on the terminal.
B. Read From Standard User Input
The readarray -t <my_array> command prompts the user to input contents and the “readarray” command takes those as contents of an input file and reads my_array with the lines. Here’s an example:
#!/bin/bash
# Initialize an empty array
declare -a my_array
echo "Enter elements (press Enter and then Ctrl+D to end):"
# Read lines from standard input into the array
readarray -t my_array
sleep 1 # wait for 1 second
# Print the array elements
echo "array elements you entered: ${my_array[@]}"
This script first declares a Bash array called my_array. Then the command readarray -t my_array
prompts the user to enter elements signaling the end of giving input by pressing ENTER and CTRL+D afterward. The sleep 1
command makes the script wait for 1 second before it prints the array elements using echo "array elements you entered: ${my_array[@]}"
.
As you can see, the readarray command prompts the user to input data and reads the given data (1 2 3) into the array my_array.
3. Using “mapfile” Command
The mapfile command is often interchangeable with readarray command and facilitates the direct reading of input lines from standard inputs or file descriptors into an array. The basic syntax to read an array in Bash from a file is mapfile -t <your_array>. The -t
option tells the mapfile
command to truncate the trailing newline characters from the lines to read into an array.
Let’s see an example to understand how the mapfile command works:
#!/bin/bash
# Initialize an empty array
declare -a myArr
# Read lines from file into the array
mapfile -t myArr < "sample2.txt"
# Print the array elements
printf '%s\n' "${myArr[@]}"
The above code uses the mapfile -t myArr < "sample2.txt"
command to read lines (apple banana pie) from the sample2.txt file and read the array myArr with those contents. Finally, it prints the array elements using the command echo"${myArr[@]}"
.
From the image, you can see that the mapfile command has read the sample2.txt file lines to the array myArr.
Conclusion
So far, this tutorial has discussed the ins and outs of 3 methods (using read, readarray, and mapfile command) to read a Bash array from external sources along with hands-on illustrations. With a solid grasp of this topic, you will be efficient in data management within Bash Arrays enhancing scripting capabilities. Happy Scripting!
People Also Ask
What’s the use of delimiter in Bash “readarray”?
The “delimiter” in the readarray command is used to denote the beginning or end of the data item. Working with delimiters provides a feature to decide when the next element of the array will be inserted into the specified array. To set a custom delimiter use the -d flag followed by the delimiter character. Here’s an example:
#!/bin/bash
readarray -d "n" myArr < sample3.txt
echo ${myArr[0]} #Index 0 will contain strings till the first character ‘n’ is found
echo ${myArr[1]} #Index 1 will contain strings after the first ‘n’ till the second ‘n’ is found
Here the readarray command will add data into myArr until it finds the delimiter “n” and will increment the array index just after finding “n” and so on.
Contents of sample3.txt:
France
Singapore
Output:
Fran
Ce Sin
How can I remove a newline character in reading a file into an array?
You can use the -t option with the readarray command to remove a new line character while reading a file into an array in Bash. Take the array your_array, for example, and use the syntax:
readarray -t your_array < "file.txt"
How to access array elements in Bash “readarray”?
Use the index numbers in the format echo ${your_array[index]} to access elements after reading them into a Bash array from a file using the readarray command. You can also use for loop to iterate over the elements. Moreover, it is possible to access all the elements in one go using the length expressions in the format: echo ${your_array[@]} or echo ${your_array[*]}.
How to read the elements of a Bash array?
To read a Bash array, use the length expression command echo ${array[@]}. For example, to read an array named nums=(1 2 3 4)
, the command is: echo ${nums[@]}
. You can also use length expression ${nums[*]} prefixing with the echo command to read the Bash array and print the contents.
Can I use a file as a standard input to read into a Bash array?
Yes, you can. Use the readarray command to read an array in Bash from a standard input file. For instance, to read an array named myArr using the file sample.txt, “use the following syntax”:
Readarray myArr <<< $(cat sample.txt)
What is “readarray” in Bash?
The readarray is a Bash built-in command that reads contents into an array. The “readarray” command reads the lines from the standard input source into the indexed array. For example, to read a command output into an array named list, use the syntax:
readarray -t list < <(ls -l)
The above command reads the array list with the current files available in your system log by locating each file at contiguous index numbers.
Related Articles
- Declare Array in Bash [Create, Initialize]
- Index Array in Bash [Explained]
- Bash Associative Arrays [Explained]
- Bash Array of Arrays [Explained]
- Elements of Bash Array
<< Go Back to Bash Array | Bash Scripting Tutorial