FUNDAMENTALS A Complete Guide for Beginners
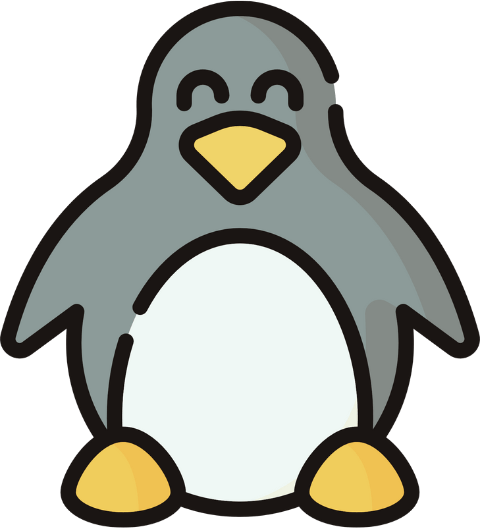
Don’t get baffled by the special variables that are internally set by the Bash shell. These special variables are crucial as users can store and manipulate valuable information related to the shell environment using them. In this article, I’ll walk you through the Bash special variables with scripting examples. Let’s dive into it!
What Are Special Variables in Bash?
Special variables are the predefined variables that get treated specially when encountered in a Bash shell. You can use these variables only for reference; not for any value assignment. The Bash shell automatically sets these kinds of variables. You can deploy these variables in Bash to explore the various aspects of the Bash shell’s behavior and execution.
Special Variables List in Bash
Here is a list of the Bash special variables and their usage:
Variable | Purpose |
---|---|
$0 | The name of the executing script. |
$1…$9 | The first nine command-line arguments. |
$# | The number of positional parameters passed to the script. |
$@ | All positional parameters as separate strings. |
$* | All positional parameters as a single string. |
$? | The exit status of the last executed command. |
$$ | The process ID of the current shell. |
$! | The process ID of the last background command. |
$- | The current options set for the shell. |
Bash Special Variables with 9 Examples
The Bash shell treats several variables specially. Here’s a detailed look with examples of the Bash special variables:
“$0” Special Variable in Bash
The $0
variable holds the name of the current shell or script. It is called the zeroth argument of the script. It allows you to reference the script’s name within the script itself. Check the following script to see how the special variable $0 works:
#!/bin/bash
echo "The script's name is: $0"
In the line echo "The script's name is: $0"
, $0
holds the name of the current shell that is being executed and the echo command prints the output.
From the above image, you can see that the value of the $0 variable is replaced with the currently executed script name.
“$1…$9” Special Variable
The special variables $1, $2, $3, …$9 contain the first nine command-line arguments provided to the script. Here, each variable corresponds to a specific positional number like $1
represents the first argument, $2
represents the second argument, etc.
Here’s an example of the special variables $1, $2, and $3:
#!/bin/bash
echo "First argument: $1"
echo "Second argument: $2"
echo "Third argument: $3"
Here, in the three lines echo "First argument: $1"
, echo "Second argument: $2"
, echo "Third argument: $3"
, $1, $2, and $3 represent the first three arguments of the current script and the echo
command prints the output.
Now, run the script by the following command:
./firstToNinth.sh Hello Linux World
From the above image, you can see that I have accessed the first three arguments. Similarly, you can access arguments from first to ninth whatever you need.
“$#” Special Variable in Bash
The special variable $#
specifies the number of command-line arguments passed to a function or script. You can use this to create Bash scripts that usually validate the user inputs. Check the bash script of special variable $#:
#!/bin/bash
echo "Argument Number: $#"
In the line echo "Argument Number: $#"
, $#
retrieves the number of command-line arguments, and the echo
command displays the output.
From the above image, you can see that $# determines the number of provided arguments, and the script displays them. As I did not put any argument after the script, it showed Argument Number 0.
“$@” Special Variable
The $@
variable represents all command-line arguments passed to a script or function as separate words or an array. It lets you access each command-line argument individually while creating Bash scripts.
To know how to work with $@ special variable, check the bash script:
#!/bin/bash
for arg in "$@"; do
echo "$arg"
done
The line for arg in “$@”; do
indicates a loop that iterates over each word in the $@ variable. Here, "$@”
retrieves all the command-line arguments as separate entities. Now, in the line echo "$arg"
, the echo
command prints the value of the arg variable.
Now, run the script by the following command:
./allarg.sh grape orange
From the image, you can see all the arguments as a space-separated list.
“$*” Special Variable in Bash
Generally, the $*
variable acts like the $@
variable when used without double quotes. But if you double-quote this variable, it behaves differently. In this case, the $*
variable stores all the command-line arguments passed to the script as a single string.
Here’s an example of a special variable $*:
#!/bin/bash
for arg in "$*"; do
echo "$arg"
done
The line for arg in “$*”; do
indicates a loop that iterates over each word in the $*
variable. Here, “$*”
specifies that the entire argument’s set as a single string meaning that the loop will iterate only once over this single entity. Now, the echo
command prints the value of the arg variable.
Now, run the script by the following command:
./singlestring.sh grape orange apple
From the above image, you can see all the assigned arguments as a single string.
“$?” Special Variable
The $?
variable stores the exit status of the last executed command. It checks the success or failure of the most recently executed command within a script. Moreover, it helps to handle errors depending on the success and failure of a command.
Here’s an example of how to check the exit status of the ping command with $? special variable:
#!/bin/bash
ping -c 1 linuxsimply.com
if [ $? -eq 0 ]; then
echo "Ping successful."
else
echo "Ping failed."
fi
Here, ping -c 1 linuxsimply.com
sends a single ping packet to the host linuxsimply.com. In the line if [ $? -eq 0 ]; then
, $?
checks the exit status of the last ping
command. If the exit status is 0, it indicates success, otherwise, it indicates failure. When the success occurs, the echo
command will execute “Ping successful.”. If the failure occurs, the echo command will execute “Ping failed.” in the else branch.
From the image, you can see that the attempt I made to ping the website linuxsimply.com is successful.
“$$” Special Variable in Bash
The $$
variable contains the process ID (PID) of the currently executed script. You can create temporary & unique files, and manage multiple background processes by using this useful variable.
See the bash script to know how to check PID with special variable $$:
#!/bin/bash
echo "Process ID (PID) of this script: $$"
In echo "Process ID (PID) of this script: $$"
, $$
holds the process ID of the currently executing script and the echo
command prints the output.
In the above snapshot, you can see that the process ID of the script I have executed is 2634.
“$!” Special Variable
The $!
variable contains the process ID (PID) of the last executed background command in the script. This variable is helpful for monitoring multiple processes and handling long-run background commands.
Let’s see an example of a special variable $!:
#!/bin/bash
echo "A background process is going to start."
sleep 1 &
background_pid=$!
echo "Background process has started with the PID: $background_pid"
#Waiting for the background process to complete
wait $background_pid
echo "Background process with the PID $background_pid has completed."
The echo "A background process is going to start."
indicates that a background process is going to start. Then, in sleep 1 &
the sleep command causes the script to wait for 1 second. Here, the &
symbol sends it to the background. Now, in the line background_pid=$!, $!
holds the process ID of the background process.
The echo "Background process has started with the PID: $background_pid"
indicate that the PID of the background process has started. Afterward, wait $background_pid
pauses the script execution until the background process with PID completes. After the completion of the background process, the echo
command prints Background process with the PID $background_pid has completed.
The above snapshot specifies the process ID of the last background command of the script.
“$-” Special Variable in Bash
The $-
variable represents the current option flags for the shell. Through this variable, you can display the current state of the shell in terms of multiple options. However, every option and its meaning can vary based on the configuration of your shell. Here are some commonly used options:
- h: Hashall option.
- m: Monitor mode.
- i: Interactive mode.
- x: Execution tracing.
- u: Unset variables as an error.
- e: Exit the shell.
- B: Brace expansion mode.
- H: History expansion.
Now, look at the bash script of the special variable $-:
#!/bin/bash
echo "Current shell options: $-"
if [[ "$-" == *i* ]]; then
echo "Enabled interactive mode."
else
echo "Disabled interactive mode."
fi
if [[ "$-" == *x* ]]; then
echo "Enabled execution tracing."
else
echo "Disabled execution tracing."
fi
Here, the line echo "Current shell options: $-"
prints the value of the $-
variable. Then, the first conditional statement if [[ "$-" == *i* ]]; then
checks if i is present in the output of $-. If i is present, the echo
command prints “Enabled interactive mode.”. Otherwise, it goes to the else block, and the echo command prints “Disabled interactive mode.”. The second conditional statement follows the same as the first but it checks if the execution tracing is enabled or not.
The above image depicts the current shell options for my system: hB meaning that the hashall option is enabled and the shell is running in brace expansion mode too. Also, the interactive mode and execution tracing are disabled for my shell.
Conclusion
So far, you have understood that Bash special variables are quite essential during Bash scripting. These variables enable users to create more robust, flexible, and dynamic Bash scripts.
People Also Ask
What are some commonly used special variables in Bash?
Some commonly used special variables in Bash are as follows:
- $0: Indicates the shell script name.
- $1, $2,…,$N: Indicates positional parameters. $1 represents the 1st argument passed to the script, $2 represents the 2nd argument passed to the script, and $N represents the Nth argument passed to the script.
- $#: Counts the number of arguments passed to the script.
- $@: Treats all the arguments as a separate entity.
- $*: Treates all the arguments as a single entity.
- $$: Represents the process ID (PID) of the current script.
- $!: Represents the process ID (PID) of the last background command.
- $?: Indicates the exit status of the last executed command.
Can I use special variables outside of Bash scripts?
No, generally, special variables are used within Bash scripts. They are not directly useable outside of the Bash environment.
Can I use special variables in arithmetic expressions?
Yes, you can use some of the special variables in arithmetic expressions. But remember that it’s not applicable to all special variables.
What is the difference between $EUID and $UID?
The $UID holds the numeric user ID that represents the users’ actual identifier, while $EUID holds the effective user ID that represents the effective permissions a user has when executing a command.
Can I use special variables in Bash variable names?
No, you cannot use special variables directly in Bash variable names. But you can use the values of these variables by assigning them to regular variables.
Related Articles
- What Are Built-in Variables in Bash [2 Cases With Examples]
- An Ultimate Guide of Using Bash Environment Variables
- The “.bashrc” Environment Variables [4 Cases]
- String Variables in Bash [Manipulation, Interpolation & Testing]
- What is Variable Array in Bash? [4 Cases]
- What is Boolean Variable in Bash? [3 Cases With Examples]
- What is HereDoc Variable in Bash? [5 Practical Cases]
- What is PS1 Variable in Bash? [3 Customization Examples]
- What is PS3 Variable in Bash? [3 Practical Examples]
<< Go Back to Types of Variables in Bash | Bash Variables | Bash Scripting Tutorial