FUNDAMENTALS A Complete Guide for Beginners
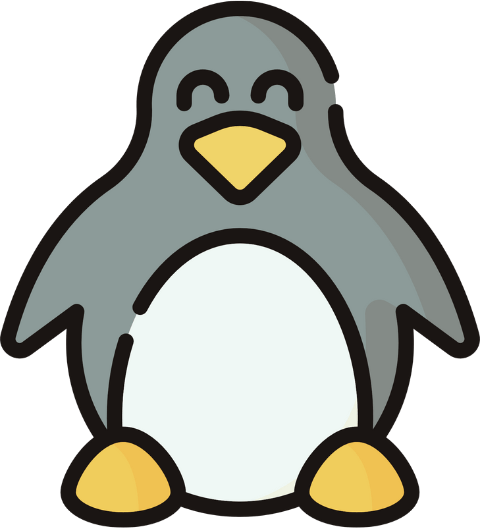
Boolean variables serve as the essential tool for creating flexible, dynamic, and condition-driven Bash scripts. These variables empower users to make Bash scripts that are evenly responsive to the changing environment & user interactions. In this guide, I’ll demonstrate the adaptable context of Bash Boolean variables for different cases.
Key Takeaways
- Learning about Bash Boolean variables.
- Experiencing Boolean variables for different cases in Bash.
Free Downloads
What Are Bash Boolean Variables?
Boolean variables are the crucial variables in Bash that contain only two possible conditions i.e., true or false for decision-making. Actually, Bash does not include the Boolean data type itself, instead, the users have to simulate these variables by using strings, integers, or by appending variable names directly in conditionals. With the explicit decision-driven capability, Bash Boolean variables enable users to control the flow of a Bash program very efficaciously.
3 Cases for Assigning Boolean Variables in Bash
You can declare and then assign values to the Boolean variables in Bash. To represent the variables you can use strings (‘true’ or ‘false’) or integers (0 for false or true for otherwise) or the variable names directly. I have described three cases below to assign the Boolean variables in Bash:
Case 1: Assigning Bash Boolean Variables Using Strings
Follow the steps below if you want to assign Bash Boolean variables using string values:
Steps to Follow >
➊ Open your Ubuntu Terminal.
➋ To open a script in the nano text editor, write the command below:
nano string.sh
- nano: A text editor.
- string.sh: This is a script. Here, I have named the script ‘string.sh’. You can name any of your choices.
➌ Hereafter, write the following script inside the editor:
Script (string.sh) >
#!/bin/bash
#Assigning boolean variables using strings
is_active="true"
is_admin="false"
In #!/bin/bash, ‘#!’ is called ‘Shebang’ or ‘Hashbang’. In ‘is_active=”true”’, the value to the Boolean variable is_active is assigned to true representing the system is active. Also, in ‘is_admin=”false”’, the value to the Boolean variable is_admin is assigned to false representing the user is not an admin.
From the image, you can see that I have assigned string values to the Boolean variables.
Case 2: Assigning Bash Boolean Variables Using Integers
Another way of assigning Boolean variables is to use integer values. Here’s how to assign Bash Boolean Variables using integers:
Script (integer.sh) >
#!/bin/bash
#Assigning boolean variables using integers
is_logged_in=5
has_permission=20
In ‘is_logged_in=5’, the integer value 5 is assigned to the variable is_logged_in indicating a non-zero integer that is considered true. Also, in ‘has_permission=20’, the integer value 20 is assigned to the variable has_permission which is also considered true.
From the image, you can see that I have assigned integer values to the Boolean variables.
Case 3: Assigning Bash Boolean Variables Using Variable Names Directly
You can use variable names directly within conditionals as Boolean values. Here’s a similar approach to the Bash Boolean Variables assignment:
Script (direct.sh) >
#!/bin/bash
#Assigning boolean variables using variable names directly
is_logged_in=true
has_permission=false
Here, in ‘is_logged_in=true’ & ‘has_permission=false’, the variable names is_logged_in & has_permission are directly assigned as Boolean values.
From the image, you can see that I have assigned the variable names as the Boolean values directly.
5 Examples of Using Boolean Variables in Bash
You can use Bash Boolean variables to declare, manipulate, and evaluate variables that specify only two conditions: true or false. Here are some examples of using Boolean variables in Bash:
Example 1: Bash Boolean Variable in Nested Conditional Statements
Typically, you can use Boolean variables in nested conditional statements. A similar demonstration is given below:
Steps to Follow >
➊ Repeat steps 1 & 2 of case 1 of assigning Bash Boolean variables and write the following script inside the editor:
Script (conditional.sh) >
#!/bin/bash
is_it_sunny=false
enjoying=true
if $is_it_sunny; then
if $enjoying; then
echo "Today is a sunny day and I'm enjoying it."
else
echo "Today is a sunny day and I'm not enjoying."
fi
else
echo "It's raining outside and I'm enjoying the rain!"
fi
Here, ‘is_it_sunny=false’ & ‘enjoying=true’ specify two Boolean variables with the assigned value ‘false’. The script uses nested conditional statements. The outer ‘if’ statement checks if the ‘is_it_sunny’ variable is true. If it becomes true, then it enters into the inner ‘if’ statement to check if the ‘enjoying’ is true.
If the ‘enjoying’ variable is true, the echo command prints ‘“Today is a sunny day and I’m enjoying it.”’, or if the ‘enjoying’ variable is false, the echo command prints ‘“Today is a sunny day and I’m not enjoying.”’. However, if the ‘is_it_sunny’ variable is false, the echo command prints ‘“It’s raining outside and I’m enjoying the rain!”’.
➋ Then, press CTRL+S to save the file & press CTRL+X to exit.
➌ After that, use the command below to make the script executable:
chmod u+x conditional.sh
- chmod: Changes the permission of the files and directories.
- u+x: Adds the executable permission for the user.
- conditional.sh: The file which you want to make executable.
➍ Finally, run the script by the following command:
./conditional.sh
In the above image, you can see the output of the matched condition.
Example 2: Bash Boolean Variable With Logical Operators
A simple example of Boolean variables with logical operators is given below:
Script (logic.sh) >
#!/bin/bash
#Applying logical OR with boolean variables
is_vip_member=true
has_free_offer=true
if $is_vip_member || $has_free_offer; then
echo "Either you are vip member or you have free offer."
fi
#Applying logical AND and NOT with boolean variables
is_active=true
has_permission=false
if $is_active && ! $has_permission; then
echo "Your account is active but you have no permission."
fi
Here, the values of two Boolean variables ‘is_vip_member=true’ and ‘has_free_offer=true’ are set to true. The if statement checks if the two variables are true by using the logical operator OR (||). If any of these two conditions become true, the echo command prints ‘“Either you are vip member or you have free offer.”’.
Again, the values of two boolean variables ‘is_active=true’ and ‘has_permission=false’ are set to true and false accordingly. Here, the if statement checks if is_active variable is true and has_permission is false by using the two logical operators AND (&&) and NOT (!). If it matches, then the echo command prints ‘“Your account is active but you have no permission.”’.
Now, run the script by the following command:
./logic.sh
The above snapshot depicts the outputs of the Boolean variables with the logical operators AND, OR, and NOT.
Example 3: Bash Boolean Variable Within Case Statements
The following example demonstrates how you can use Bash Boolean variables in nested case statements to handle multiple combinations:
Script (case.sh) >
#!/bin/bash
age=15
get_vaccine=true
case $get_vaccine in
true)
case $age in
[0-14])
echo "You are not allowed for the vaccine."
;;
*)
echo "You are allowed for the vaccine."
;;
esac
;;
false)
echo "You must be vaccinated to enter here."
;;
esac
Here, two values 15 and true are assigned respectively to the Boolean variables ‘age’ & ‘get_vaccine’. The outer case statement checks if the value of ‘get_vaccine’ is true. If true, the inner case statement checks the ‘age’ variable. When ‘age’ is between 0-14, the echo command prints ‘“You are not allowed for the vaccine.”’. If ‘age’ is more than 14, the echo command prints ‘“You are allowed for the vaccine.”’. Now, if ‘get_vaccine’ is false, the echo command prints ‘“You must be vaccinated to enter here.”’.
Now, run the script by the following command:
./case.sh
From the image, you can see the output of the Boolean variables within the case statements.
Example 4: Bash Boolean Variable for Loop Control
You can control an infinite loop by using the Bash Boolean variables. Here’s an example:
Script (loop.sh) >
#!/bin/bash
loop_running=true
count=1
while $loop_running; do
echo "Student: $count"
count=$((count + 1))
if [ $count -eq 6 ]; then
loop_running=false
echo "End of the students' loop."
fi
done
Here, two variables ‘loop_running’ & ‘count’ are assigned two values true & 1 respectively. Now, the while loop iterates until the ‘loop_running’ variable is true. Inside the loop, the echo command prints the current student count using the ‘count’ variable. Next, ‘count=$((count + 1))’ indicates the increment of the ‘count’ variable by 1.
In the line ‘if [ $count -eq 6 ]; then’, the if statement checks if the value of ‘count’ equals 6. When the ‘count’ becomes 6, it sets ‘loop_running’ to false and stops the loop to iterate after 5 times (1-5). Then, the echo command prints ‘“End of the students’ loop.”’.
Now, run the script by the following command:
./loop.sh
The screenshot above dictates a loop of students which I have limited using the Boolean variables.
Example 5: Reading & Validating Boolean Input From Users
You can easily read and validate Boolean inputs from users and respond properly according to the inputs.
Script (input.sh) >
#!/bin/bash
read -p "Are you ready to continue? (yes/no): " user_input
if [ "$user_input" = "yes" ]; then
echo "User is ready to continue."
else
echo "User is not ready to continue."
fi
In ‘read -p “Are you ready to continue? (yes/no): ” user_input’, the read command prompts the user input, the ‘-p’ flag provides the prompt to the user, and the input is stored in ‘user_input’ variable. Then, in ‘if [ “$user_input” = “yes” ]; then’, the if statement checks if the ‘user_input’ variable is yes. Now, if the ‘user_input’ is yes, the echo command prints ‘“User is ready to continue.”’, or if the ‘user_input’ is no, the echo command prints ‘“User is not ready to continue.”’.
Now, run the script by the following command:
./input.sh
The above image illustrates different outputs according to the response of the different user inputs.
Conclusion
Boolean variables are a versatile approach to control the flow of scripts in Bash. By using these variables effectively, you can append various tasks and make decisions based on different conditions regarding your script.
People Also Ask
Related Articles
- What Are Built-in Variables in Bash [2 Cases With Examples]
- An Ultimate Guide of Using Bash Environment Variables
- The “.bashrc” Environment Variables [4 Cases]
- String Variables in Bash [Manipulation, Interpolation & Testing]
- What is Variable Array in Bash? [4 Cases]
- An Extensive Exploration of Bash Special Variables [9 Examples]
- What is HereDoc Variable in Bash? [5 Practical Cases]
- What is PS1 Variable in Bash? [3 Customization Examples]
- What is PS3 Variable in Bash? [3 Practical Examples]
<< Go Back to Types of Variables in Bash | Bash Variables | Bash Scripting Tutorial