FUNDAMENTALS A Complete Guide for Beginners
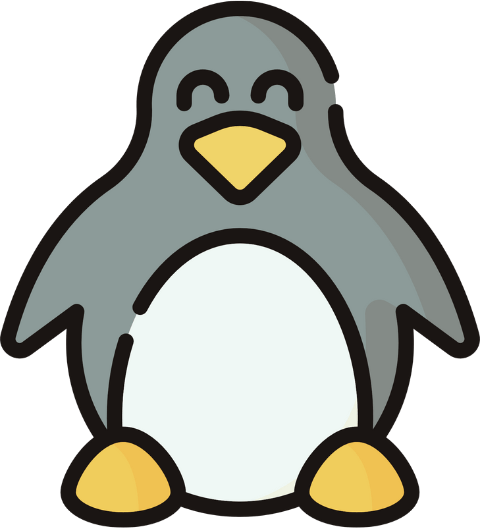
Learning how to redirect stderr (standard error) is a must-know knowledge for every Linux or Unix system administrator or developer while Bash scripting. Cause, it makes it simpler to debug, log, and monitor your Bash scripts and programs since it lets you manage error messages separately from stdout (standard output). This writing will cover the basics of stderr redirection & how you can redirect stderr with some practical Bash script examples.
Key Takeaways
- Learning the concept of the stderr redirection process.
- Getting to learn how to redirect stderr with practical cases.
Free Downloads
What is Stderr?
‘Stderr’ stands for ‘standard error’. It is one of the three standard I/O (input/output) streams used by programs in Unix-like operating systems, including Linux. This is the stream through which a program sends error messages. By default, it sends data to the terminal just like stdout (standard output). However, it lets programs separate regular output from error messages too. With the help of the redirection process, it can be redirected to a log file, discarded, or even sent to a variable for further processing.
5 Practical Cases to Redirect Stderr in Bash
In the following article, I will show 5 practical cases of how you can redirect stderr (error messages) generated by different command-line tools while creating Bash scripts.
Case 1: Redirecting Stderr to a File in Bash
In Bash, you can redirect Stderr to a file using the ‘2>’ redirection operator. Follow the below steps to see how I used the redirection process while generating a Bash script:
❶ At first, open your Ubuntu Terminal application.
❷ Now, open a file, let’s say, named ‘file.sh’ in the nano editor by using the following command:
nano file.sh
➌ Next, write the following script inside the Bash file in the nano text editor:
#! /bin/bash
cd /nonexistent_directory 2> log.txt
cat log.txt
➍ Then, press CTRL+S to save the file & press CTRL+X to exit the nano editor.
➎ After that, use the following command to make the script executable:
chmod u+x file.sh
- chmod: Changes the permission of files and directories.
- u+x: Argument with chmod command to add the executable permission for the user.
- file.sh: File which you want to make executable.
➏ Finally, run the script by the following command:
./file.sh
From the image, you can see that stderr messages are redirected to the ‘log.txt’ file and I viewed the contents of the ‘log.txt’ file using the cat command in the script.
Case 2: Appending Stderr to a File in Bash
Normally, when you redirect stderr to a file with the ‘2>’ redirection operator, it overwrites the file. But if you want, you can append the stderr to the existing file contents while redirecting by using the ‘2>>’ redirection operators. Check out the following script:
You can follow the Steps of Case 01, to see how to save, make the script executable, and run it.
Script (append.sh) >
#! /bin/bash
whoami -l 2>> log.txt
See from the output image, the stderr messages are appended to the ‘log.txt’ file and I viewed the contents of the ‘log.txt’ file using the cat command in the script. Where the new message is stored at the bottom of the previous file content.
Case 3: Redirecting Stderr to “/dev/null” in Bash
A common technique in Unix-like systems is redirecting stderr to ‘/dev/null’ when you want to discard error messages or prevent them from cluttering your terminal or log files.
The null device, ‘/dev/null’ is a special file in Unix-like systems that discards all data written to it.
You can follow the Steps of Case 01, to see how to save, make the script executable, and run it.
Script (null.sh) >
#! /bin/bash
{
date # Display the current date & time
date -r /var/empty/foo # Attempt to display the modification timestamp of a non-existent file
} 2> /dev/null
Only the stdout of the executed commands of the script is displayed, while stderr is discarded.
Case 4: Redirecting Stderr to a Variable in Bash
You can redirect stderr to a variable in a Bash script, for that you can use command substitution along with the ‘2>&1’ redirection operator. Go through the following script for further clarification:
You can follow the Steps of Case 01, to see how to save, make the script executable, and run it.
Script (var.sh) >
#! /bin/bash
# Prompt the user for a command
read -p "Enter a Command: " user_command
# Run the user-entered command and store both stdout & stderr
result=$(eval "$user_command" 2>&1)
# Check whether an error occurred or not
if [ $? -ne 0 ]; then
error_message="$result"
echo "$error_message"
else
echo "Executed successfully."
fi
After that, the script checks the exit status of the executed command using ‘$?’. If the exit status is non-zero, it indicates an error occurred & user inserted a wrong command. Then the content of the ‘result’ variable is assigned to the ‘error_message’ variable, and an error message is displayed. Otherwise, a success message is displayed.
I ran the script twice so that you can see the difference between the outputs. At first, I entered a valid command ‘date’. So it was executed successfully. But the second time, I entered the date command with option -r to view the modification timestamp of a non-existent file /var/empty/foo. As a result, the script outputs the error message.
Case 5: Redirecting Stderr to Stdout in Bash
One quite common scenario while Bash scripting is to redirect stderr to stdout while storing a program’s output to a file. That way you can have everything in one file.
You can follow the Steps of Case 01, to see how to save, make the script executable, and run it.
Script (to_stdout.sh) >
#! /bin/bash
{
whoami
whoami -l
} > output.txt 2>&1
echo "The output is '$(cat output.txt)'"
From the image, you can see the stderr is redirected to the same location stdout is, that is the output.txt file. And, inside the file, notice the stdout & stderr of the executed commands.
Importance of Redirecting Stderr in Bash
Redirecting stderr (standard error) in Bash is important for several reasons:
- Error Handling → Allows you to separate error messages so that you can handle errors more effectively.
- Logging → While automating, redirecting stderr to a log file helps to make it easier to diagnose issues.
- Cleaner Terminal → It’s an essential process for those times when you want to keep your terminal clean & only want to display outputs. You can just redirect error messages to the null device to be discarded.
- Data Integrity → When processing important data, having a clean separation between regular data and error messages ensures data integrity.
- Troubleshooting → Handling error messages separately helps in troubleshooting and improving the script.
Conclusion
To sum up, whether you want to store, append, display, or discard the Stdout and Stderr of commands, the Bash redirection process helps to manage them. The discussed practical cases in this article should help you to learn how to handle them. Happy Bash scripting!
People Also Ask
Related Articles
- How to Read From Stdin in Bash? [4 Cases With Examples]
- Bash Input Redirection [3 Practical Cases]
- What is Output Redirection in Bash [With 4 Practical Cases]
- How to Redirect Stdout and Stderr to File in Bash [5 Cases]
- Redirect Stderr to Stdout in Bash [5 Examples]
- 5 Ways to Echo to Stderr in Bash
<< Go Back to Bash Redirection | Bash Redirection and Piping | Bash Scripting Tutorial