FUNDAMENTALS A Complete Guide for Beginners
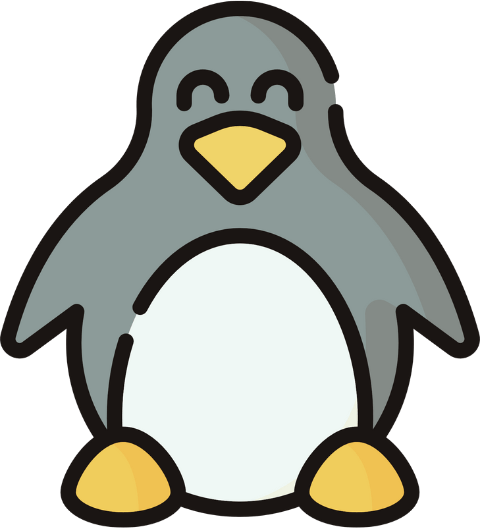
In Bash, Output redirection is a powerful feature that allows you to control where the output of commands or scripts is sent. By default, any command output (standard output or stdout) is displayed on the terminal. However, with output redirection, you can send the output to a file, append it to an existing file, or even merge it into a file. In this article, I will discuss the basics of Bash output redirection & then show some practical Bash script examples of it for further explanation.
Key Takeaways
- Learning basics of Bash output redirection.
- Getting a practical overview of the process with Bash script examples.
- Learning the importance of the output redirection process in Bash scripting.
Free Downloads
4 Practical Cases of Bash Output Redirection
The fundamental method for altering the source or destination of a program’s file descriptors is through Bash redirection. In the following article, I will show how you can use output redirection in different practical scenarios while generating Bash scripts.
Case 1: Redirecting Output to a File in Bash
You can redirect the stdout of a command to a file using the output redirection procedure. Now if the file doesn’t exist, it will be created. If it exists, its contents will be overwritten. Please follow the below steps to check the entire process yourself:
❶ At first, open your Ubuntu Terminal application.
❷ Now, open a file, let’s say, named ‘file.sh’ in the nano editor by using the following command:
nano file.sh
➌ Next, write the following script inside the Bash file in the nano text editor:
#! /bin/bash
echo “Hello World!” > Output.txt
In the script’s first line, ‘#!’ is shebang or hashbang. It indicates the interpreter to be used for executing the script, in this case, it’s bash situated in the /bin directory. In the next line, the output of the ‘echo command’ is redirected to a file named ‘Output.txt’ using the ‘>’ operator. If ‘Output.txt’ already exists, it will be overwritten with the new output. Otherwise, a new fill will be created.
➍ Then, press CTRL+S to save the file & press CTRL+X to exit the nano editor.
➎ After that, use the following command to make the script executable:
chmod u+x file.sh
- chmod: Changes the permission of files and directories.
- u+x: Argument with chmod command to add the executable permission for the user.
- file.sh: File which you want to make executable.
➏ Finally, run the script by the following command:
./file.sh
From the output image, you can see after executing the script it displays no output as it is redirected to the ‘Output.txt’ file. However, later I viewed that file using the cat command. And you can see the output string ‘Hello World!’ inside it.
Case 2: Appending Output to a File in Bash
Normally, when you redirect a stdout to a file with the ‘>’ redirection operator, it overwrites the file. But if you want, you can append the stdout to the existing file contents while redirecting by using the ‘>>’ redirection operators. Check out the following script:
Script (append.sh) >
#! /bin/bash
echo "Hello World!" >> Output.txt
Now, run the script by the following command:
./append.sh
The script appends the output of the script to the ‘Output.txt’ file. As you can see from the output of the ‘cat command’.
Case 3: Redirecting Output to “/dev/null”
While working in Bash scripts, you may need to suppress your certain command output instead of redirecting it inside a file. Just to check if the command works or maybe for a cleaner terminal you want to discard the output. Now the simplest and most common approach to suppress output in Bash is by redirecting it to the null device, ‘/dev/null’. This special file in Unix-like systems discards all data written to it.
Check out the following script, where I will perform the execution of the curl command in silence while suppressing its output to the null device.
Script (discard.sh) >
#! /bin/bash
url="https://linuxsimply.com"
if curl -s "$url" > /dev/null; then #Checks the URL request validity
echo "Request succeeded"
else
echo "Request failed"
fi
Here, the script uses the ‘curl’ command to make an HTTP request to the specified URL “https://linuxsimply.com”. The command option ‘–silent’ suppresses the progress meter & then the command output messages are redirected to ‘/dev/null’ using ‘> /dev/null’ instead of displaying on the terminal.
The ‘if’ statement checks the exit status of ‘curl’. If the request succeeds (i.e. the exit status is 0), it echoes “Request succeeded”. Otherwise, echoes “Request failed”.
Now, run the script by the following command:
./discard.sh
Executing the script only gives the “Request succeeded” message. While it suppresses all the output messages of the curl command.
Case 4: Redirecting Output & Input Simultaneously
Redirecting input is a process where the standard input (stdin) of a command is changed from the keyboard to a file. By, using the ‘<’ symbol you can take input data from a file instead of typing it interactively. In this case, let’s see how input & output redirection works concurrently.
Script (combo.sh) >
#! /bin/bash
sort <input.txt> sorted_output.txt
Later, the ‘>’ symbol is used for output redirection where it tells the shell to take the stdout of the sort command to the ‘sorted_ourput.txt’ file.
Now, run the script by the following command:
./combo.sh
From the image, first, you can see the contents of the input.txt file, and after executing the script the sorted output of the input.txt file which was redirected to the sorted_output.txt file.
Importance of Bash Output Redirection
Bash output redirection is a fundamental concept in shell scripting and command-line usage that provides several benefits and use cases. Here are some key reasons highlighting the importance of Bash output redirection:
- Control Output Destination: Redirecting output allows you to specify where the output of a command goes, whether it’s a file, another command, or nowhere (discarded)
- File Creation & Logging: Enables the creation and maintenance of log files, facilitating error tracking, debugging, and record-keeping.
- Silencing Output: Useful for quiet execution, keeping the terminal clean & managing error messages separately.
- Parallel Execution: Allows multiple commands to run concurrently and manage their outputs, vital for automation & scripting.
- Script Automation: Essential for enabling the automation of repetitive processes.
Conclusion
To sum up, output redirection is a powerful technique in Bash that allows you to control where command outputs are directed. By using various redirection operators and techniques, you can save, discard, or manage command output as needed. This writing covered the basics of output redirection and provided practical cases to help you get started!
People Also Ask
Related Articles
- How to Read From Stdin in Bash? [4 Cases With Examples]
- Bash Input Redirection [3 Practical Cases]
- How to Redirect Stderr in Bash [5 Practical Cases]
- How to Redirect Stdout and Stderr to File in Bash [5 Cases]
- Redirect Stderr to Stdout in Bash [5 Examples]
- 5 Ways to Echo to Stderr in Bash
<< Go Back to Bash Redirection | Bash Redirection and Piping | Bash Scripting Tutorial