FUNDAMENTALS A Complete Guide for Beginners
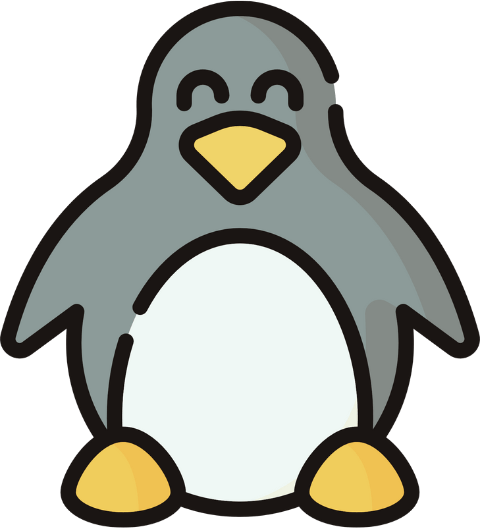
The double pipe (‘||’) is a logical operator that is often used in various programming and scripting languages, including Bash. It is known as the ‘OR’ operator that enables conditional execution & allows you to execute a command or a series of commands only if the preceding command fails. In this article, I will show you how to use double pipe “||” in Bash scripts with 3 practical examples.
What is Double Pipe (||) in Bash?
The double pipe (||) is a logical OR operator used in control flow structures to execute a command or a series of commands only if the preceding command returns a non-zero exit status, indicating a failure.
In a more simple context, every process returns an exit status (either “0” or “non-zero”). A code of 0 means the process ran successfully while a non-zero indicates an error. And, a double pipe causes commands to be executed only if the preceding command returns a non-zero exit status. You can understand the process even better if you look at it through the Truth Table of the OR operator:
Command 1 | Command 2 | Result |
---|---|---|
True | True | Command 1 |
False | True | Command 2 |
True | False | Command 1 |
False | False | Command 1 |
If Command 1 is true the result will always be the output of Command 1, regardless of the value of Command 2. And, if Command 1 is false, the result will be the output of Command 2.
Now, if I explain the basic syntax of using the double pipe (||) in commands, then I could just simply write:
<Command 1> || <Command 2>
Here, if Command 1 succeeds (returns a zero exit status), then Command 2 will not be executed. And, if Command 1 fails (returns a non-zero exit status), then Command 2 will be executed.
Check out this simple example of using ‘Double Pipe’:
Suppose you want to create a backup of a file and be sure it was successfully backed up. You can use the double pipe operator for a situation like this:
cp file.txt backup_file.txt || echo “Backup Failed”
See from the image, as the cp command fails to create the backup, the echo command displays Backup Failed.
3 Examples of Using Double Pipe “||” in Bash Scripts
Using the double pipe operator ‘||’ for simple operations in a shell script is a much easier approach to handling errors and failures more effectively. While other methods (i.e. if-else) might be verbose with increased code length & prone to more errors, double pipe allows for concise, one-liner error-handling actions when a specific command fails. In the following article, I will explain how the operator works with practical Bash script examples, so read along:
1. Create a Directory If it Doesn’t Exist
You can test the existence of a directory using a conditional expression [ condition ], where the conditional test operator -d
checks if the directory with the same name stored in a variable exists or not. And, if this file doesn’t exist (returns a non-zero exit status), the next part of the double pipe ||
will be executed & a new directory with the name will be created.
Here’s how to create a directory if it doesn’t exist using double pipe in Bash:
#! /bin/bash
directory_name="test"
[ -d "$directory_name" ] || mkdir "$directory_name"
The script checks if the directory “test” exists, and if it doesn’t, it creates a same-named directory using the mkdir command.
Upon execution of the script, you can see that the test directory did not exist in my system, & from the output of the ls -l command, was created (recently after I ran the script).
2. Install a Package If Not Already Installed
Suppose you need to install a package but not if it is already installed. In that case, you can create a conditional script using the ||
logical operator that will search for the package (if installed) in your system & if the result is non-zero then it will install the package.
To install a package if it’s not already installed use the following script :
#! /bin/bash
package_name="curl"
dpkg -l | grep -q "ii $package_name" || sudo apt install "$package_name"
This script will quietly search (grep command with the option –quiet) the package name from the installed packages (represented by ii
) from the output of the dpkg -l command. If the package is installed the second command sudo apt install $package name
will not run, otherwise, it will install the package ( curl command line tool).
3. Delete a File If it Exists
Sometimes you need to create a file but you’re not sure if any file with the same name already exists in your system & you just want to create a new one with that name. You can do that just by deleting the existing ones.
For that, first, check the existence of the file with the test operator -e
& if this part is true (returns ‘0’ exit status), run the next part of the command using the && operator (executes the command only if the preceding one returns true).
To delete a file if it exists using Bash script, use the following code snippet:
#! /bin/bash
file_name="input.txt"
[ -e "$file_name" ] && rm "$file_name" || echo "File not found: $file_name"
The script defines a variable with the file name to be checked as value & -e
checks the file’s existence. If it exists, then the rm command removes it & as the first two parts of the commands will be executed the last part (after the ||
operator) won’t get executed. But, if the first two-part returns a non-zero value, the last part will be executed & display File not found: $file_name.
The file input.txt wasn’t removed as it doesn’t exist in my system.
Conclusion
To sum up, the double pipe (||) operator is a simple yet powerful tool for conditional execution in Bash scripts. By understanding how it works & combining it with other operators, you can create much more effective & flexible scripts. Hope this writing helps you to understand the operator perfectly!
People Also Ask
What Does “||” in Bash Mean?
In Bash, the double-pipe ||
symbol is a logical operator used for the conditional execution of commands. Specifically, it is used to execute a command only if the command preceding it fails. This means if a command on the left side of ||
succeeds (exits with a status code of 0), the command on the right side of ||
will not be executed & vice versa.
What is a Pipe in Bash?
A ‘pipe’ is a technique used in Bash & other Unix-like shell environments for connecting the output of one command to the input of another command. It is represented by the vertical bar symbol |
. The simple syntax for using pipe between 3 commands is, Command 1 | Command 2 | Command 3
.
What is the Difference Between a Single Pipe and a Double Pipe?
The single pipe |
is used for creating pipelines that allow the output of one command to be passed as input to another command, more like for chaining commands together. Whereas, the double pipe ||
is a logical OR operator used in conditional statements, in a simple context, to execute the second command only if the first command fails.
Related Articles
- How to Read From Pipe in Bash [5 Cases]
- How to Pipe Output to File in Linux [4 Ways]
- Send Pipe Output to Two Commands in Bash [3 Methods]
<< Go Back to Bash Piping | Bash Redirection and Piping | Bash Scripting Tutorial