FUNDAMENTALS A Complete Guide for Beginners
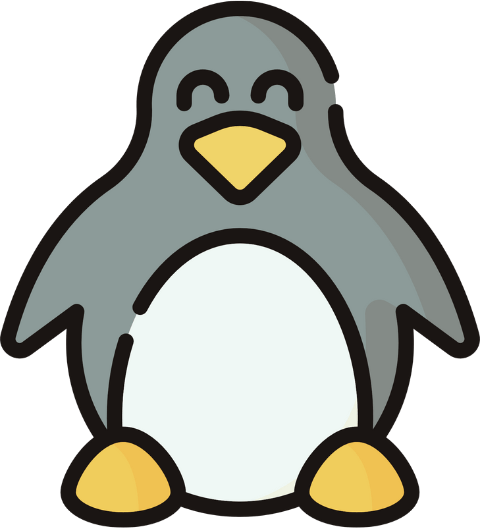
If you want to master shell scripting, saving Bash output to variables is a must-know process. It will give you the ability to write shorter and more manageable codes, and you will have better control over your data processing. In this article, you can learn how to save Bash output to variable using the command substitution method. Also, I have demonstrated some cases that will give you a better idea of how to use this method practically.
Key Takeaways
- Saving bash output to a variable using command substitution by $() or backticks (`).
- Using command substitution in a loop.
- Saving outputs in variables using nested commands.
- Storing output to a variable by specifying the command path.
- Saving output to a variable by using a command line argument.
Free Downloads
Process to Save Output to Variable Using Bash Script
Using the command substitution method, you can save bash output to variables. Command substitution is a method in Linux that allows users to use the output of one command as the input of another or as a variable.
There are two command substitution syntaxes, you can follow:
Syntax
- Using ‘($)’ notation:
variable=$(command)
- Using backticks (`):
variable=`command`
➊ First, launch an Ubuntu terminal. You can use the shortcut keys CTRL+ALT+T to do so.
➋ Then create a file with the extension .sh using the nano command and open the script in the text editor like the following.
nano example1.sh
- nano: Opens and creates a file in the nano text editor.
- sh: File for writing the bash script.
➌ After that, copy the following script into your text editor.
Script (example1.sh) >
#!/bin/bash
calendar=$(ncal -m August)
echo -e "Here is the calendar of August 2023:\n$calendar"
In this code, the first line specifies the bash interpreter. Then the calendar variable stores a value from a command ncal -m August, and to print the variable, with a message I have used the echo command.
➍ To save and exit the text editor, press CTRL+ O and CTRL+X.
➎ Then, to make the bash script file executable, run the following line in the terminal.
chmod +x example1.sh
- chmod: Changes the permission of files and directories.
- +x: Argument with the chmod command to add the executable permission.
- sh: File which you want to make executable.
➏ Finally, you can simply run the script from your command line by typing:
./example1.sh
In the above image, the ncal command was executed and stored in the calendar variable while running the script. That’s why, when the calendar variable was called it showed the stored output.
4 Practical Cases of Saving Output to Variable Using Bash Scripts
You have been familiarised with how to save the output to variables using command substitution. Now I will demonstrate some cases where you will use this command substitution method in various ways to save the output to a variable.
Case 1: Using Command Substitution in Loop to Store Multiline Output to Variable
Suppose you want to assign files and their sizes to a directory as an output in a variable that you can call later. In this case, you should use the command substitution but in the loop. The loop should be used for those types of variables that store more than one line. An example may make it easy for you.
Here, this code lists all directories and their subdirectories and prints their names. You can use the following scripts to do the same.
You can follow the steps of Command Substitution method to know about creating and saving shell scripts.
Script (loop_variable.sh) >
#!/bin/bash
# Loop through each directory
for dirname in $(find . -maxdepth 2 -type d ! -name . -printf "%f\n")
do
echo "$dirname"
done
Here, the for loop is used to go through each directory, find is to list directories, -maxdepth 2 is to limit the search to all directories and subdirectories, and don’t allow sub_sub directories in this list. -type d is to specify that only directories are listed and ! -name excludes the current directory from the list. Finally, it prints directory names with -printf with a new line and prints each directory name on each line until the end of the loop.
To simply execute the script from your command line, run the following command:
loop_variable.sh
In this picture, you can see that I have used loop to store multiple output of a command to a variable .
Case 2: Using Nested Commands
You can also store more than one command’s output in a variable using nested commands in the command substitution method. Here, the first command’s output fully depends on 2nd command’s output.
Command Syntax >
variable_name=`command1\`command2\``
The script that I have used to print today’s date and time is given below. You can follow the scripts to do the same.
You can follow the steps of Command Substitution method to know about creating and saving shell scripts.
Script (example2.sh) >
#!/bin/bash
variable1=`echo \`date\``
echo $variable1
Here, the date command is assigning the output to the echo command and echo command is taking that output and echoing it, The output from echo is assigned in variable1, which was printed in the next line.
To simply run the script from your command line, run the following command:
./example2.sh
In the above image, you can see that the nested command substitution has printed the date after executing the script and calling the variable as it was assigned in the variable.
Case 3: Specifying Command Path in Command Substitution
Here, in the command substitution method, I am going to specify the path of the command. And the output of the command will be stored as a variable. You can follow the scripts to do the same.
You can follow the steps of Command Substitution method to know about creating and saving shell scripts.
Script (example3.sh) >
#!/bin/bash
variable=$(/usr/bin/uname)
echo $variable
The uname command’s path is specified by /usr/bin and the output will be assigned in the variable. Then we printed the variable, so the uname command gets executed.
To simply run the script from your command line, run the following command:
./example3.sh
In this image, you can see that the Linux is the output as the command was uname, and the output was stored in a variable. When I executed the script the output was echoed.
Case 4: Using Command Line Argument
You can also use command line arguments within command line substitution and generate a personalised greetings. Here, we have stored a greeting line “Welcome” as a variable, then used a command line argument to call it with a string. You can follow the scripts to do the same.
You can follow the steps of Command Substitution method to know about creating and saving shell scripts.
Script (example4.sh) >
#!/bin/bash
variable=$(echo "Welcome, $1!")
echo "$variable"
In this bash script, a command line argument $1 is used within the $(), and the value of $1 is concatenated with the string “Welcome” and an exclamation mark ! using the command echo.
Note: When you run the script with a string, it will capture that as argument and generate the greeting “Hello, the string you entered within quote”
Now, run the script with the following command
./example4.sh "Maria"
In the image above, you can see that Maria was captured as an argument while executing the script, and the greeting was generated with it.
Conclusion
Finally, knowing how to save output to a variable in Bash is essential because you can use these variables, later on, to analyse the data or use them as inputs for other commands. Using this, a program can become flexible, and you can also use it to automate a task. You’ll be able to handle and manipulate data with ease if you master this method.
People Also Ask
Related Articles
- How to Print Output in Bash [With 6 Practical Examples]
- What is Echo Command in Bash [With 3 Practical Examples]
- How to Echo New Line in Bash [6 Practical Cases]
- Cat Command in Bash [With 4 Practical Examples]
- How to Save Bash Output to File? [3 Practical Cases]
- How to Set Command Output to Variable in Bash [2 Methods]
- How to Suppress Output in Bash [3 Cases With Examples]
- How to Change Color of Output Using Bash Script? [Easy Guide]
<< Go Back to Bash Output | Bash I/O | Bash Scripting Tutorial