FUNDAMENTALS A Complete Guide for Beginners
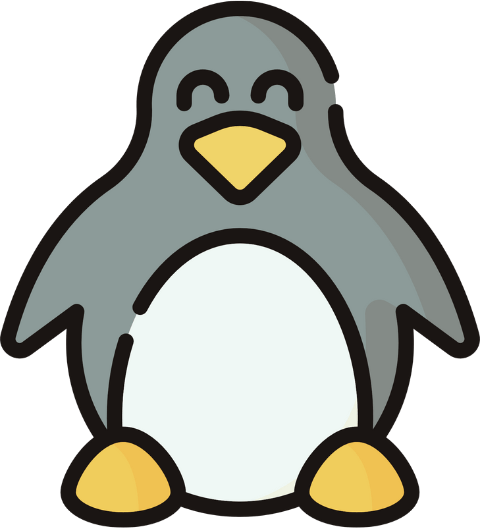
In Bash scripting, knowing how to print output accurately & efficiently is a must-have skill. Whether you need to display simple text, formatted numbers, variable values, or complex data, Bash provides several methods to meet your output needs. In this article, I will discuss basic ways to print in Bash & then I will discuss how you can print customized outputs using the printf command with some practical examples.
Different Ways to Print in Bash
There are several ways to print output in Bash. Here are some different methods:
A. “echo” Command
The echo command is a built-in command in bash scripting that is used to display text to the terminal. This is the simplest command to print any output in the bash scripting. Also, it automatically adds a new line character at the end of the output.
B. “cat” Command
The cat command is a which displays the contents of files or concatenates multiple files and displays the combined output.
C. “printf” Command
The printf command is used to format and print text in a specific manner. It allows you to control the formatting of the output, including the use of placeholders for variables. This command provides more control over the output format compared to the ‘echo’ command.
D. Here Document
A here document allows users to print multiple lines or a block of text by specifying it directly in the script. Generally, it is useful when a user wants to print formatted or multiline text.
Out of all these ways, in this writing, I will discuss how you can print output by executing Bash scripts using the printf command with some practical examples.
The “printf” Command in Bash
In Bash, ‘printf’ is a built-in shell command used to format & print text or data. It allows you to customize outputs by specifying format specifiers for various types of data and formatting options. It’s more powerful than the ‘echo’ command in terms of formatting output.
Command Syntax >
printf [FORMAT] [Argument]...
Here,
- FORMAT → Specifies the format string, which contains format specifiers and optional formatting instructions.
- Argument → Refers to the data or variables to be substituted into the format specifiers defined in the format string.
Format Specifiers >
- %d → Considers the input as a decimal (integer) number.
- %c → Considers the arguments as a single character.
- %i → Holds an integer number as an input.
- %s → Considers the input as a string of characters.
- %x → Holds a hexadecimal number as an input.
- %f → Holds a floating number as an input.
- %% → Prints a percent sign.
- \n → Prints a new line.
- \t → Prints a horizontal tab.
6 Examples of the “printf” Command
In the following article, I will present you with 6 practical examples of the ‘printf’ command. Where you can see how to use format specifiers with the command arguments to customize the desired output.
Example 1: Printing a Simple String Using Bash Script
To print a simple string using the ‘printf’ command, check out the following script:
#! /bin/bash
printf "Hello, world! \n"
Here, the printf
command simply prints the quoted message followed by a newline character \n
.
The output is simply printing a string ‘Hello, world!’.
Example 2: Printing a String With Variable Substitution Using Bash Script
The ‘printf’ command helps users print a simple string with the value assigned to a variable.
Review the script below to print a string with variable substitution:
#! /bin/bash
name= "Jane"
printf "Hello, %s!\n" "$name"
At first, a value “Jane” is assigned to the variable named “name”. Then, the printf
command prints a message where %s
is a placeholder for a string & the value of the variable $name
is substituted into the placeholder.
In the image, the command ‘printf’ is outputting the string along with the variable ($name) value, that is Jane.
Example 3: Printing Formatted Numbers Using Bash Script
The ‘printf’ command allows users to print formatted numbers using different format specifiers. For example, the format specifier %d is used to place a decimal (integer) number as input.
Here’s a script to print formatted numbers in Bash:
#! /bin/bash
num=42
printf "The answer is %d. \n" "$num"
Here, %d
is a placeholder for a decimal (integer) number. The variable ‘$num’ value is substituted into the placeholder to print the output.
The output displays a decimal number 42, which was placed inside the ‘$num’ variable.
Example 4: Formatting Floating-Point Numbers Using Bash Script
The ‘printf’ command helps in formatting floating-point numbers using the format specifier %f. Here’s how to do it:
#! /bin/bash
pi=3.14159
printf "The value of pi is %.2f. \n" "$pi"
In this script, %2f
is a placeholder for a floating-point number with two decimal places. The variable ‘$pi’ value is substituted into the placeholder, to display in the output.
The output is showing a floating number 3.14, with two decimal places. This value was placed inside the $pi variable.
Example 5: Specifying a Width and Alignment for a String
Navigate through the script below to specify a width and alignment for string and number placeholders in ‘printf’ command:
#! /bin/bash
name= "Austen"
age=06
printf "Name: %-10s Age: %02d\n" "$name" "$age"
Here, %-10s
specifies a left-aligned string with a width of 10 characters, and %02d
specifies a zero-padded decimal number with a width of 2 characters. The values of the variables ‘$name’, and ‘$age’ are substituted into the respective placeholders to print the output with alignment.
Here, the outputs are presented in the way they were supposed to be. The first variable value Austen is a left-aligned string that can have a width of 10 characters & the second variable value 06 is a zero-padded decimal number.
Example 6: Printing Hexadecimal Value Using Bash Script
The ‘printf’ command prints hexadecimal numbers using the format specifier %x. In this case, a decimal number is used as an input variable value & the command with its specifier substitutes the decimal value into the hexadecimal output value.
Here’s a script to print hexadecimal value in Bash:
#! /bin/bash
num=105
printf "Hexadecimal: %x\n" "$num"
Here %x
is a placeholder for a hexadecimal number & the value of the variable ‘$num’ is substituted into the placeholder to display the output.
The output displays 69, which is the corresponding hexadecimal value for the decimal number 105 that was placed in the $num variable.
Conclusion
In conclusion, throughout this writing, I tried to discuss the basic ways of printing in Bash. Among them, I gave a thorough description of the ‘printf’ command with some practical examples. Hope this article helps you learn how to print output in Bash.
People Also Ask
How do you print in bash?
To print in Bash, you can use either the echo or printf command. For example, you can use the ‘echo’ command followed by a text like the syntax echo "Hello, Linux!"
or use the ‘printf’ command using the syntax printf "Hello, Linux! \n"
.
How do I print a line in bash?
To print a line in Bash, you can use the echo command followed by the text you want to print. The command will output the specified text followed by a newline character. That means it will be displayed as a separate line in the terminal.
How to use printf command in bash?
To use the printf command in Bash, follow the basic command syntax printf format_string [arguments]
. Here, the format_string defines the format of the output and the arguments are the values to be printed based on the format specifiers.
How do I print a user in bash?
To print the username of the currently logged-in user in Bash, you can use the command whoami
. You can store the command’s output as a variable for further use in your Bash script using username=$(whoami)
.
Related Articles
- What is Echo Command in Bash [With 3 Practical Examples]
- How to Echo New Line in Bash [6 Practical Cases]
- Cat Command in Bash [With 4 Practical Examples]
- How to Save Bash Output to File? [3 Practical Cases]
- How to Save Bash Output to Variable? [With Practical Cases]
- How to Set Command Output to Variable in Bash [2 Methods]
- How to Suppress Output in Bash [3 Cases With Examples]
- How to Change Color of Output Using Bash Script? [Easy Guide]
<< Go Back to Bash Output | Bash I/O | Bash Scripting Tutorial