FUNDAMENTALS A Complete Guide for Beginners
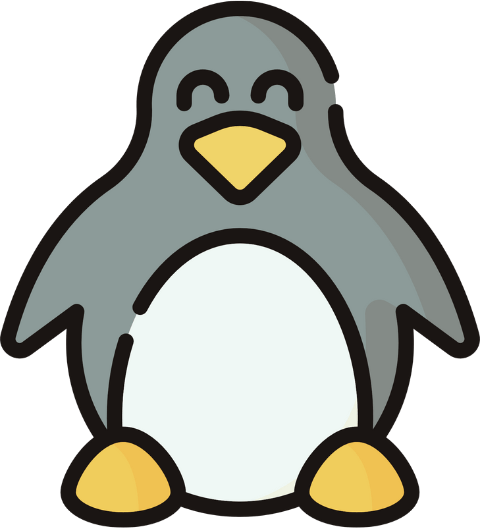
Bash commands often come with options and the output of a command varies depending on the options provided. Therefore, it is important to know how to incorporate options into a Bash script when you are creating your own commands. This article explores the use of options to get the desired output while controlling the execution path of a script.
What Are Bash Script Options?
Bash script options are flags or switches, that enable users to tailor the behavior of a script during execution. These options typically follow the script name or command name and are denoted by a hyphen followed by a single character. For example, in the ls -l
command, “-l” signifies the “list” option. Additionally, in verbose mode, options can be specified after a double hyphen using their full names, such as ls --list
.
2 Methods to Add Options in Bash Script
Options can be added to a bash script in a couple of ways. 2 popular methods of adding options are parsing options as arguments and parsing options using the getopts command.
1. Parsing Options as Argument of a Bash Script
A brute-force way of parsing options to bash scripts could be the utilization of positional parameters. This process involves iterating through each argument, determining if it’s an option and then shifting to the next argument. This continues until no options remain. Here is an example:
#!/bin/bash
# Iterate through each argument
while [[ $# -gt 0 ]]; do
case "$1" in
--verbose|-v)
echo "Verbose Mode Activated"
;;
--help|-h)
echo "Displaying help..."
# Add help instructions here
;;
*)
echo "Unknown option: $1"
;;
esac
shift 1 # Move to the next argument
done
The bash script parses command-line options by iterating through each argument provided to the script. Using a while loop, it processes each argument using a case statement. If the argument matches a defined option, such as --verbose
or -v
, it prints the message “Verbose Mode Activated”.
Similarly, if the argument matches --help
or -h
, it prints that it is displaying help instructions. For any argument that doesn’t match a known option, it prints a message indicating an unknown option.
Finally, shift 1
causes all arguments to shift to the left 1. It means the second positional argument ($2
) becomes the first positional argument and so on.
-v
and --verbose
. Moreover, it doesn’t execute the code that is written under the case --help
or -h
.
2. Using “getopts” to Parse Options
The getopts command is the default choice in Bash for parsing command-line options. It is easy to manage options in scripts using the getopts command. Here’s a basic example:
#!/bin/bash
while getopts ":ab" opt; do
case ${opt} in
a)
echo "Option -a is triggered."
;;
b)
echo "Option -b is triggered."
;;
?)
echo "Invalid option: -${OPTARG}."
exit 1
;;
esac
done
This Bash script uses a while loop which iterates through each option provided to the script. The case statement handles each option (a or b) separately. If option -a is provided, it echoes a message indicating that -a is triggered.
Similarly, if option -b is detected, it echoes a message indicating that -b is triggered. If an invalid option is provided, it prints an error message specifying the invalid option and exits the script using exit 1
.
The getopts command utilizes the OPTARG environment variable. OPTARG always holds the current option detected by getopts. For example, if the optstring provided to getopts is “:ab”, then in the first iteration, OPTARG contains the option a. And in the second iteration, OPTARG contains the option b.
Options with Parameter in Shell Script
As a Linux user, you should be familiar with shell commands that take additional parameters or values under options like this:
command_name -op1 value1 -op2 -op3 value1 value2
For instance, recall the command for printing the three-month numeric calendar:
ncal -B1 -A1
Here, -A and -B are options and 1 following these options are parameters or values for those options.So there must be a way to pass additional parameters or values to options in a bash script. The trick is the shift command. Here is an example:
#!/bin/bash
while [ -n "$1" ]; do
case "$1" in
-a) echo "-a option passed"
;;
-b) echo "-b option passed, with value $2"
shift 1
;;
-c) echo "-c option passed"
;;
*) echo "Option $1 not recognized"
;;
esac
shift 1
done
In the script, the while loop continues as long as there is a non-empty argument to process. [ -n "$1" ]
checks whether the first positional parameter is non-empty. Then, the case statement examines the value of the first parameter, $1. If it matches -a, it echoes a message indicating that the -a option is passed. If it matches -b, it echoes a message indicating that the -b option is passed, and similarly for the -c option.
Furthermore, the -b option takes an additional parameter that is provided as the subsequent argument $2. The script shifts the arguments accordingly using shift 1
, ensuring proper processing of options and their values. For any argument that doesn’t match a known option, it prints the message “Option not recognized”.
Bash Shell Options to Change Shell Settings
Some options are crucial for modifying shell settings, allowing you to customize shell behavior. These options can be set or unset to activate or deactivate specific features within the shell. One such commonly used option is -e of the set command. This option tells the shell to exit immediately if any invoked command exits with a non-zero status. It’s a handy technique to catch errors in a shell script:
#!/bin/bash
set -e
echo "This will run"
fictional_command
echo "The program should not go here"
The script begins by enabling the -e option, which means that the shell exits immediately if any command fails. The first echo command should execute successfully. However, upon encountering the non-existent “fictional_command”, the script exits abruptly due to the -e option, bypassing the last echo command entirely.
The options to set or unset shell attributes are listed below:
Option | Description |
---|---|
-a | Marks variables and functions for export to subsequent commands in the environment. |
-b | Immediately reports status of terminated background jobs. |
-e | Exits immediately if a command in a pipeline returns a non-zero status. |
-f | Disables filename expansion (globbing). |
-h | Remember commands for execution lookup. |
-k | Place all assignment statement arguments in the environment for a command. |
-m | Enables job control, runs processes in separate process groups, and prints exit status of background jobs. |
-n | Reads commands without executing them, useful for syntax error checking. Ignored by interactive shells. |
-x | Prints commands before execution. |
Standard Option Notation
You always have the freedom to choose letters for the options passed to your script. However, some letters are commonly used by Bash users. Here is a list of such options with their conventional purpose:
Options | Purpose |
---|---|
-a | List all items. |
-c | Get the count of something. |
-d | Output directory. |
-f | To specify a file. |
-h | To show the help page. |
-i | To ignore character case. |
-n | To say no to a question. |
-o | To send output to a file or so. |
-q | Keep silent; don’t ask the user. |
-r | To process something recursively. |
-v | Verbose mode |
-x | Specify executable. |
-y | To say yes without prompting the user. |
It’s good practice to follow the standards. It will help fellow Bash users to understand your script. Also, maintaining the standard notation allows you to seek help from the community more effectively.
How to Force Execution of a Script Using Options
Bash Scripts often require a force execution option to override default behaviors or confirm potentially destructive actions. Options make the task easy. Look at a simple example:
#!/bin/bash
force=false
while getopts ":f-:" opt; do
case $opt in
f | - -force)
force=true
;;
\?) echo "Invalid option: -$OPTARG" >&2; exit 1;;
esac
done
if $force; then
echo "Forcing execution..."
elif ! $force; then
read -p "Do you want to proceed without forcing execution? (Y/N): " confirm
if [ "$confirm" == "Y" ] || [ "$confirm" == "y" ]; then
echo "Proceeding without forcing execution..."
# Add your additional actions here...
else
echo "Execution aborted."
exit 0
fi
fi
This Bash script defines a variable force
initialized as false and utilizes getopts to handle command-line option -f
including its long form --force
. It toggles the “force” variable based on whether the -f
or --force
option is provided. If force is true, it displays a message indicating the execution is being forced. If “force” is false, it prompts the user for confirmation to proceed without forcing execution. Depending on the user’s response, it either continues with default actions or aborts the execution accordingly.
-f
or --force
option, it requires user’s confirmation to proceed further. On the other hand, with any form of the option, the script forcefully executes without requiring user confirmation.
How to Create a User-Defined Command Option And Parameter
The idea is to create a simple command called “calc” that can perform basic arithmetic calculations. It should take options like -a
or --addition
for adding two numbers. -m
or --multiplication
for multiplying numbers. Similarly, -s for subtraction and -d for division and also their long forms. So that user can run a command like below to add two numbers, say 50 and 30:
calc -a 50 30
The command should also have a help option for giving instructions to its new user. First of all, the instructions users should receive by pressing the help option can be put in a function like below:
Portion 1 >
#!/bin/bash
help() {
echo "Usage: calc [options] operand1 operand2"
echo "Options:"
echo " -a, --addition Perform addition"
echo " -s, --subtraction Perform subtraction"
echo " -m, --multiplication Perform multiplication"
echo " -d, --division Perform division"
echo " -h, --help Display this help message"
exit 0
}
The help
function provides user instructions for the “calc” command. The function displays that arithmetic operations are performed based on the specified options (-a, -s, -m, -d for addition, subtraction, multiplication, and division respectively). When the “help” function is called inside a script it should exit after displaying the message written in the function.
Now, the following “calc” function is defined using a case statement to perform different arithmetic operations based on user provided options:
Portion 2 >
calc() {
case $1 in
-a | --addition) echo "Result of addition: $(($2 + $3))";;
-s | --subtraction) echo "Result of subtraction: $(($2 - $3))";;
-m | --multiplication) echo "Result of multiplication: $(($2 * $3))";;
-d | --division)
if [ $3 -eq 0 ]; then
echo "Error: Division by zero!"
else
echo "Result of division: $(($2 / $3))"
fi
;;
-h | --help) help;;
*) echo "Invalid option: $1"
;;
esac
}
The calc
function uses a case statement to handle different options: a or addition, s or subtraction, m or multiplication, d or division, and h or help. Depending on the option provided, it performs the corresponding mathematical operation on the two operands passed as arguments. If the option is -d
or --division
, it checks if the divisor is zero to avoid division by zero errors. On the contrary, If the option is -h
or --help
, it calls the help function defined earlier to display usage instructions. If an invalid option is provided, it prints an error message.
Finally, ensure that the “calc” function can be called as a command in the terminal after sourcing it using the source command. This can be done using a single if statement as follows:
Portion 3 >
# Call the calc function only if the script is executed directly
if [[ "${BASH_SOURCE[0]}" == "${0}" ]]; then
calc "$@"
fi
This conditional statement checks whether the name of the current Bash script matches the name of the script being executed ("${0}"
). If they match, it means the script is being executed directly, so it calls the calc function with any arguments passed to the script ("$@"
).
At this point, put all three portions in a single script and source the script before calling the calc function as a command:
source calculator.sh
Once I run the command calc with -a option it performs addition of the arguments 50 and 2 provided after the option.
When the long form option --subtraction
is supplied for subtraction, it performs subtraction of two provided arguments 25 and 20.
At last, while running the command with -h option, it prints an user manual of the command “calc”.
Conclusion
To sum up, I discussed positional parameters and the getopts command to handle options in a Bash script. When using positional parameters, the shift command becomes necessary to properly parse arguments as options or values of options by shifting them to the left. Additionally, I’ve listed Bash shell options, enabling and disabling which changes the shell settings. Furthermore, I’ve created a function named calc to demonstrate how a user-defined command processes options and arguments provided to it.
People Also Ask
How do I add options to a bash script?
To add options to a Bash script, use the getopts command. Here’s a basic example of how you can add options:
#!/bin/bash
# Parse options
while getopts ":ab:" opt; do
case $opt in
a)
echo "Option a is triggered"
# your code here for option a
;;
b) echo "Option b is triggered"
# your code here for option a
;;
esac
done
Now, you can run the script with option -a or option -b or both as follows:
./scritptname -a
./scritptname -b
./scritptname -b -a
What is the purpose of the OPTIND variable in getopts command?
The OPTIND variable in the getopts command serves the purpose of indicating the index of the next argument to be processed. For example, if getopts has already processed some arguments, OPTIND will hold the index of the next argument that has not yet been processed.
How to add verbosity option in Bash script?
To add a verbosity option in a Bash script, you can initialize a variable say verbose to 0 to indicate verbosity level off by default. Then, use getopts to parse options, such as -v for verbosity. Inside the option handling loop, when -v is encountered, set verbose to 1 to indicate verbosity is on. How is a script to do this:
#!/bin/bash
verbose=0
while getopts "v" OPTION
do
case $OPTION in
v) verbose=1
;;
esac
done
Why should I use getopts to parse options to bash script?
You should use the getopts command to parse options to bash script as it has several advantages over other techniques. A single optstring in getopts command can handle options with and without values. For instance, to provide multiple options without value, use:
getopt "abc" -a -b -c
Here, “abc” is the opstring and the options don’t take any value.
In addition, one can customize the opstring for taking additional values to options as follows:
getopt "ab:c::" -a -b123
In this case, a is an option that doesn’t take any value. b is an option that takes a mandatory value. And finally c is an option that takes a value but it is not mandatory.
What is the difference between option and argument in Bash?
Options are flags that begin with a hyphen (-) and modify the behavior of a command or script. Whereas arguments also known as parameters are used to provide required info into a script. Sometimes arguments are provided after specifying an option if that option takes further information. Think about the command ls -l /home
. Here, -l
is an option that defines how the ls command should behave. And /home
(the home directory) is an argument that provides specific data to the ls command.
Related Articles
- How to Define a Function in Bash [2 Methods]
- Variables in Bash Function [Easy Reference]
- How to Call a Function in Bash [7 Cases]
- How to Use Alias And Functions in Bash [Reference Manual]
- Argument in Bash Script
- Return Values From Bash Function
- Overriding Commands in Bash
- Bash Function Examples
<< Go Back to Bash Functions | Bash Scripting Tutorial