FUNDAMENTALS A Complete Guide for Beginners
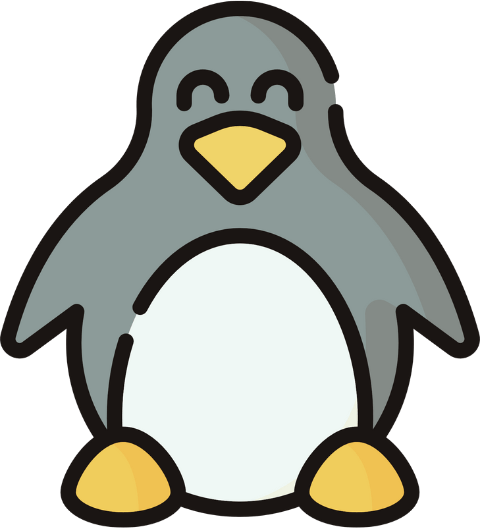
Aliases are used to wrap up long commands under a single name. Sometimes, they serve the purpose of a function. Moreover, they have some unique features when used in a Bash function. This article talks about aliases in Bash and their behavior within and outside of a function.
What is an Alias in Bash?
An alias in Bash or Linux OS is a nickname for a command or set of commands. It allows users to choose a short and easy name for frequently used long and complex commands. Users can then use the alias name as a command in the terminal to execute the grouped commands under that name. They are particularly useful for saving time and reducing typing effort for repetitive tasks.
How to Create an Alias in Bash
To create an alias in Bash, use the alias command with the following syntax:
alias alias_name="commands"
For instance, the command for creating an alias named “ll” for listing files in long format would be:
alias ll='ls -l'
As you see, after defining the alias typing “ll” in the terminal is interpreted as
ls -l
.
Note that, the declaration of an alias in Bash ends up with an error if there is any space around the =
symbol.Here, the space after the equal sign causes the error.
An alias replaces its name exactly with its contents on the command line. So one can append additional arguments or options after the alias name. For instance, in the example above, adding options such as -h after the alias name ll
would translate to “ls -l -h”.
Alias of Multiple Commands
An alias can combine multiple commands by separating them with a semicolon (;) or &&. Therefore, the syntax structure of aliasing multiple commands can be:
alias alias_name="command1; command2; command3"
Or,
alias alias_name="command1 && command2 && command3"
Let’s create an alias named “update” to update the package manager and upgrade installed packages:
alias update="sudo apt update && sudo apt upgrade"
The image shows that typing the alias name “update” in the terminal executes both sudo apt update
and sudo apt upgrade
sequentially.
If a command within the multi-command alias fails to execute, the execution of subsequent commands will be halted. For instance, if sudo apt update
fails for some reason, sudo apt upgrade
will not be executed.
How to Create Aliases to Take Arguments Using Function
Bash aliases don’t take arguments or parameters in their definition. To incorporate arguments in an alias, first define a function with arguments. And then make the function an alias. For a better understanding look at the following example:
alias newdir='dir() { mkdir -p -- "$1" && cd -P -- "$1"; }; dir'
This command defines a Bash alias named “newdir”, which has a function named “dir” that takes one argument (a directory name). Inside the function, it creates a directory using mkdir -p
to ensure parent directories are created if needed, and then changes the current directory to the newly created one using cd -P
. Finally, the function is called within the definition of the alias.
Salient Features of Aliases in Bash Function
Aliases have some interesting features within Bash functions. They are expanded during function declaration, not when the function is executed. Look at the following example for a better visualization:
echo "Calling alias within function." > file1.txt
alias myalias="cat"
func1() {
myalias file1.txt
}
func1
Here,
func1
is defined to call myalias
which is nothing but the cat command. Therefore calling “func1” reads the content of file1.txt.
Let’s redefine “myalias” as follows:
alias myalias="ls -l"
Now, call “func1” again and see what happens:
func1
It is evident that “func1” executes the previous definition of “myalias”. Because myalias was expanded when the function was defined. Even the shell no longer knows that func1 calls myalias; cause the alias name is replaced by its content. Hence the function only knows that “func1” calls the cat command. You can see the definition of “func1” using the type command:
type func1
The image shows that func1 doesn’t have the alias myalias in its definition. Rather it has the cat command in the place of myalias.
Another important thing to note is that an alias defined in a function is not available until after the function is executed. This is easy to discern with the following example:
echo "Defining alias within a function." >file2.txt
func2() {
alias ll="ls -lh"
}
ll file2.txt
Calling the alias
ll
defined in func2
ends up with an error because the alias isn’t available until the func2 has been executed.
Let’s execute the function func2 and try to access the alias “ll”:
func2
ll file2.txt
This time the alias “ll” lists the file “file2.txt”
How to Make an Alias Permanent to Shell Sessions
Aliases defined in the terminal are available to that terminal session only. To make an alias permanent to shell sessions, write it in a file that is read when a shell session begins. One such file is ~/.bashrc. Open the file in any editor such as nano and write the alias at the end of the file to make it permanent:
sudo nano ~/.bashrc
Consider, the following “update” alias. Now, to make it permanent write it at the bottom of the bashrc file:
alias update="sudo apt update && sudo apt upgrade"
Finally, save and close the file. Any aliases added in the bashrc file will be available to the new shell sessions. If you open a new terminal and run the update alias you will see the effect:
How to Bypass an Alias in Bash
An alias takes precedence over a command of the same name. Therefore, if an alias is named after a shell command, it will override the command. For example, if an alias is named “ls”, it will override the built-in ls command. To execute the built-in shell command and bypass the alias, put a backslash before the command name:The image shows that an alias is defined as ls. Running “ls” in the terminal executes the ls alias not the ls command. However, placing a backslash before the name of the command executes the built-in shell command.
How to Remove an Alias in Bash
To remove an alias write the alias name as an argument after the unalias command:Here, alias “update” is not available after deleting it using the unalias command.
5 Frequently Used Aliases
Certain commands are used by Bash users so often that they should be permanent aliases. One such alias that I have previously discussed is “update” for updating and upgrading packages. There are a ton of others as well.
1. Change Directory to the Top Level of a Git Repository
To change the directory to the top level of a Git repository, you can define the following alias:
alias gd="cd $(git rev-parse --show-toplevel)"
2. Copy the Current Directory to the Clipboard on MacOS
To copy the current directory to the clipboard use the following ccd alias on your macOS:
alias ccd='pwd | tr -d "\n"| pbcopy'
The alias ccd copies the current directory path to the clipboard by first retrieving the path using pwd, removing the newline character with tr command, and then copying it to the clipboard with pbcopy.
3. Alias to Climb up Multiple Directories
One can define aliases to move up multiple directories as follows:
alias ...='cd ../..'
alias ....='cd ../../..'
These aliases allow you to move up two or three directory levels at once by typing “…” and “….” respectively.
4. Alias for Searching in the History List
The following alias will help to search from the history list directly using the grep command:
alias hist="history | grep"
5. Alias for Finding the Largest Files/Directories
To find most largest files or directories within the quickest amount of time, use the following alias:
alias large='du -hsx * | sort -rh | head -10'
Conclusion
Hopefully, this article gives you some insights into aliases and functions in Bash. I believe from now on you can create your aliases and functions. But be cautious while defining aliases with the same name as existing commands. And, never forget the behavior of aliases in a function.
People Also Ask
What advantages does a Bash alias offer?
The main advantage of Bash alias is it saves time by reducing the typing effort. Moreover, an alias is more memorable than a long-winded command.
How can I list all the aliases defined in a shell session?
You can easily list all the aliases defined in the current shell session by executing the alias command without any additional arguments.
How can I use an alias in a function?
You can use an alias in a function only if it is defined and available in the current shell session. However, redefining an alias used in a function doesn’t replace the definition used in the function declaration.
Which one is better bash function or alias?
Both bash function and aliases are useful. So determining whether a function or alias is better depends on the use case. For example, if it is required to parse arguments then the function has more flexibility. On the other hand, if it is just a frequently used long command, then an alias is recommended.
For which command should I create an alias?
You should consider creating an alias for commands that you frequently use or ones that have long or complex syntax. This can streamline your workflow and make it easier to execute these commands quickly with a shorter, more memorable alias. To find which command deserves an alias, you can search history using the following command:
history | awk '{CMD[$2]++} END {for (a in CMD) print CMD[a], CMD[a]/NR*100 "%", a}' | grep -v "./" | sort -nr | head -n10
It counts the occurrence of each command and calculates its percentage relative to the total number of commands. Finally, it filters out the top 10 commands from the history list.
Where should I put my aliases used in a Bash script?
If a Bash script contains both functions and aliases, it’s advisable to place the aliases before the definition of any function. This ensures that the aliases are available for use within the functions defined in the script.
Related Articles
- How to Define a Function in Bash [2 Methods]
- Variables in Bash Function [Easy Reference]
- How to Call a Function in Bash [7 Cases]
- How to Add Options in a Bash Script [2 Methods]
- Argument in Bash Script
- Return Values From Bash Function
- Overriding Commands in Bash
- Bash Function Examples
<< Go Back to Bash Functions | Bash Scripting Tutorial