FUNDAMENTALS A Complete Guide for Beginners
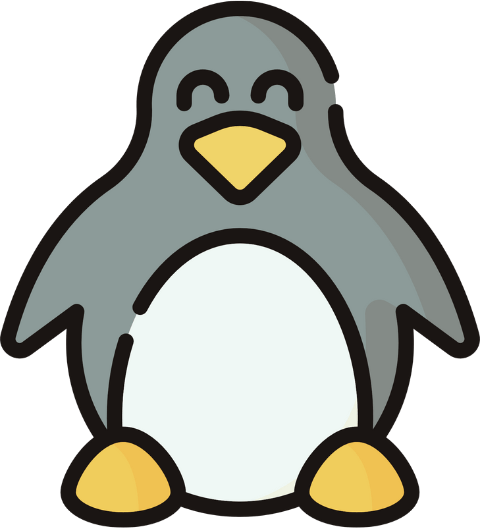
The ‘rm’ command is a powerful tool for deleting files, but when it comes to scenarios where the file’s existence is uncertain, executing ‘rm’ without precautionary measures can lead to unintended consequences. If you want to be sure before deletion, you can use the -i
option with rm
. The complete syntax is: rm -i file_name
. For forcefully deleting a file whether the file exists on the system or the file is write-protected, you can use the syntax: rm -f file_name
. In this article, I will discuss some cases of bash ‘rm’ if file exists.
6 Cases to Remove the File If Exists
There are several cases or scenarios where you might want to remove a file if it exists. Here are the cases:
Case 1: Delete File If Exists Using the ‘rm’ Command
The rm command can be used to delete a file if exits. First, create a file named file1.txt using:
touch file1.txt
Now, this script will delete the file1.txt:
#!/bin/bash
# Set the filename
filename='file1.txt'
# Check whether the file exists or not
if [ -f “$filename” ]; then
rm file1.txt
echo "$filename is removed"
fi
The conditions of if command -f “$filename”
checks whether the file inside the filename variable is a regular file. If the condition is true then it will execute the rm file1.txt file and print a message.
The above script has checked for the existence of the “file1.txt” file. Then the rm command deleted the file.
Case 2: Delete File Interactively Using ‘rm’ Command with ‘-i’ Option
The -i
option is the simplest syntax to be sure about the removal before removing a file. The complete syntax is: rm -i file_name
. This command will ask whether you want to delete the intended file before finally deleting it. Here is how to delete a file interactively with the rm -i
command:
#!/bin/bash
# Take the filename
read -p 'Enter the filename to delete: ' filename
# Check whether the file exists or not
if [ -f "$filename” ]; then
# Remove the file with permission
rm -i "$filename"
# Check whether the file is removed or not
if [ -f “$filename” ]; then
echo "$filename is not removed"
else
echo "$filename is removed"
fi
else
echo "File does not exist"
fi
Here the rm -i "$filename"
command will ask for confirmation from an interactive prompt and if the user presses “Y” it will delete the file and will not delete in case of pressing “N”.
The rm command has removed the “file1.txt” file after getting “Y” as confirmation from the terminal.
Case 3: Delete File Using ‘rm’ Command with ‘-v’ Option
To delete a file that is passed as an argument from the command line and print a remove message, use the rm command with the -v option. Here is how to delete the file and print a delete message with the rm command with the -v option:
#!/bin/bash
#Check whether the file exists or not
if [[ $1 != "“ && -f $1 ]]; then
#Print remove message
rm -v $1
else
echo "Filename is not provided or filename does not exist"
fi
Here the rm -v $1
command will delete the file passed as argument and print a remove message on the terminal.
The -v option with the rm command has removed the “file1.txt” file and printed a delete message on the terminal.
Case 4: Forcefully Delete a File Using ‘rm’ with ‘-f’ Option
To delete a file by executing a one-line command whether the file is write-protected or the file does not exist you have to use the -f
option along with the rm command. Here is an example script of how to delete a file by one-line command.
#!/bin/bash
filename=’file1.txt’
ls | grep “$filename”
rm -f “$filename”
ls | grep “$filename”
The rm -f “$filename”
command will forcefully remove the file inside the filename variable whether the file is write-protected or does not exist.
See the “file1.txt” file has not been printed on the second line which means the rm command with -f has removed it.
Note: You can also use [ -f file ] && rm file
to remove a file executing a one-line command.
Case 5: Delete Multiple Files Using ‘rm’ Command
You can easily delete multiple files by passing the file name as an argument. Then incorporate an if condition with a for loop which will concatenate all the files together and then the rm command will delete all the files together.
Here is the bash script for how to delete multiple files:
#!/bin/bash
files="”
space=" "
# Check the multiple filenames are given or not
if [ "$#" -gt 2 ]; then
# Reading argument values using a loop
for argval in "$@"
do
if [ -f "$argval" ]; then
files+="$argval$space"
else
echo "$argval does not exist"
fi
done
# Remove files
rm $files
echo "Files are removed."
else
echo "Filenames are not provided, or filename does not exist"
fi
The if [ -f "$argval" ]
command finds if the argument file is regular or not. Then the files+="$argval$space"
command will concatenate all the file names one after another and save them to the files variable. Then the rm $files
command has deleted all the files saved inside the files variable.
See all the files have been deleted together.
Case 6: Check Existence then Delete the File
Check the existence first and then delete the file, just use the -e
option with the file name inside the condition of the if command and put the rm command inside the if command.
Here is the bash script on how to check existence and then delete the file:
#!/bin/bash
if [ -e file1.txt ]
then
rm file1.txt
fi
Here the -e file1.txt
inside if condition checks if the file exists.
See the “file1.txt” file has been deleted.
Note: You can also use the below single-line code syntax for checking existence of a file and remove it:
[ -e file_name ] && rm file_name
Conclusion
Removing unnecessary files has been one of the most important skills to keep the user’s system clean and storage efficient. I believe after going through this article, you have become skillful enough to delete your unnecessary files.
People Also Ask
How do you delete a file if it exists in Linux?
You can delete a file using the rm command if it exists in Linux. Let’s try to delete the filex.txt file. For this notion, you have to follow the syntax: rm filex.txt
How do I delete a folder in bash?
To delete empty folders, you use any of the following syntax:
rmdir folder_name
rm -d folder_name
rm -r folder_name
Is it possible to reverse rm?
No, it is apparently impossible to reverse the rm. Because the rm
command deletes the file or directory permanently.
What is the silent rm command?
Usually, the rm
command silently deletes files and directories. When you use the -v
or --verbose
option it tells us about each file and directory it deletes.
How do I delete multiple files in bash?
To delete multiple files at once, you can use the rm command followed by the name of the files. The file names should be separated by a space.
Related Articles
- Check If a Variable Exists in Bash If Statement
- Check If An Input Argument Exists in Bash [4 Methods]
- Check If a String Contains Substring, Number & Letter in Bash
- Check If a String is Empty or Not in Bash [5 Methods]
- How to Compare Strings in Bash With if Statement [6 Methods]
- How to Compare Numbers in Bash With If Statement [2 Methods]
- Check If Array Contains an Element in Bash [6 Methods]
- Bash if-else Condition in One-Line [2 Ways]
- Using grep in if Statement in Bash [4 Cases]
- How to Use Regex in Bash If Condition? [4 Cases]
- What is ‘$?’ in the Bash if Statement? [8 Examples]
<< Go Back to If Else in Bash | Bash Conditional Statements | Bash Scripting Tutorial