FUNDAMENTALS A Complete Guide for Beginners
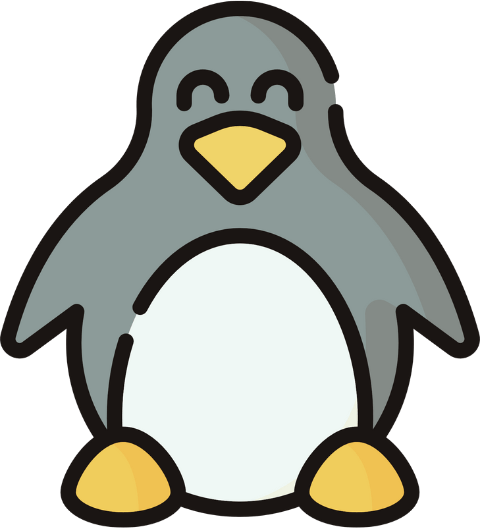
To check whether a string is empty, compare the string with empty string (” “):
if [ "$string" == " " ]; then
echo "Empty"
else
echo "Not empty"
fi
Apart from that, Bash offers multiple operators such as -z, -n, ==, !=, and so on that can be used to check if a string is empty or not. Additionally, you can use the parameter expansion method which provides a straightforward way to check the string content.
In this article, I will provide 5 different methods to check whether a string is empty or not in bash, thus empowering you to write more effective scripts with fewer errors.
What is a String in Bash?
A string is a collection of characters that are enclosed within a single quotation mark (‘’) or double quotation mark (“”). These strings may include letters, digits, unique characters, and spaces. Strings store data or text that you can use to control, show, or work with inside a bash script. For instance:
string=“Hello, world 123”
A string with zero length that contains no letters, digits, characters, or gaps is called an empty string. For example:
empty_string=""
1. Check If a String is Empty Using “-n” Option
The -n option of the if-else statement actually checks if the input string length is not zero. If it is zero, it evaluates to false, otherwise gives the true output and returns if expression block.
To check if a string is empty using the -n option, use this syntax [ -n “$string” ]. Here’s how it works:
#!/bin/bash
string=""
if [ -n "$string" ]; then
echo "The string is not empty."
else
echo "The string is empty."
fi
When the string length is zero or empty, the script will display the else block code “The string is empty.” otherwise, it will show the if code block “The string is not empty.”.
In this image, you can see that the string is empty as no values are specified within the string of the bash script.
2. Check If a String is Not Empty Using “-z” Option
The -z option tests if the input string is empty or not. It returns a true result if the input string is empty. Conversely, it evaluates to false if the input string is not empty.
This section provides a step-by-step explanation of two distinct types of string content verifications in bash. Check out the following cases:
2.1 Using Single Square Bracket With “-z”
To check if a string is not empty, use the -z option along with the if-else conditional statement. Here, the single square bracket “[“ is used to test the condition inside the brackets:
#!/bin/bash
string="hello,i am using Ubuntu"
if [ -z "$string" ]; then
echo "empty string."
else
echo "The string is not empty."
fi
If the defined string length is zero, then it will print the if block expression. On the other hand, it will print the else block expression.
The output indicates that the string is a non-empty string, as it contains specified values like “hello, i am using Ubuntu”.
2.2 Using Double Square Brackets With “-z”
Using the double square bracket “[[“ for checking conditional statements is preferable to the single square one “[“, as it is more sophisticated than the latter.
To check if a string is not empty, use the -z $string inside the double square brackets. Here’s the bash script for this:
#!/bin/bash
string="hello,i am using Ubuntu"
if [[ -z $string ]]; then
echo "Empty string."
else
echo "Non-empty string."
fi
If the input string is empty, it will display the if code block. Otherwise, it will print else block code “Non-empty string.”.
After running the above script, it shows that it is a non-empty string because the string contains “hello,i am using Ubuntu”.
3. Check if a String is Not Empty Using Parameter Expansion
The parameter expansion method uses curly braces “{}” prefixed with a $
sign to find, replace, and modify the value of a parameter. Here, I will employ parameter expansion inside the if condition to check whether the string is set or not empty. This syntax ${string:+test}
replaces the string value with test if the parameter string is non-empty otherwise, it expands to an empty string.
To check if the string is not empty using the parameter expansion method, see the bash script:
#!/bin/bash
string="Linuxsimply"
if [ ${string:+test} ]
then
echo "Non-empty string"
else
echo "Empty string"
fi
If the test command finds the string is empty, it returns the false expression. Otherwise, it will return the true expression which is “Non-empty string“.
Running the script shows that it is a Non-empty string because it has the words “Linuxsimply” in it.
4. Check If a String is Empty Using Equal “==” Operator
The equal == operator checks whether the string is equal to another string. So, this operator can be used to check if a string is empty by comparing it with an empty string. The expression [ “$string”==”” ] within the if condition checks if a string is empty. Let’s see how it works:
#!/bin/bash
string=""
if [ "$string"=="" ]; then
echo "Empty string"
else
echo "Non-empty string"
fi
If both strings are equal, it will execute the if block code. Contrarily, it will print the else block expression “Non-empty string”.
As you can see the output displays an empty string as the input string contains nothing in it.
5. Check If a String is Not Empty Using Not Equal “!=” Operator
The operator != is a negation of == operator that checks for inequality. Unlike, the equal operator, this operator gives the true outcome if both strings are not equal to one another. To check if a string is not empty by using the != operator, look at the script below:
#!/bin/bash
string="hello,i am using Ubuntu"
if [ "$string" != "" ]; then
echo "Non-empty string."
else
echo "Empty string."
fi
If the predefined string is equal to the empty string, it returns false expression “Empty string.”, otherwise returns true expression “Non-empty string.”.
This image indicates that the string is non-empty because the string contains “hello, i am using Ubuntu”.
Conclusion
I hope this article helps you understand the procedure to check if the string is empty or not. Here, I have explained 5 methods of checking the contents of a string variable. You can choose any of them to accomplish your task according to your preferences. Best of luck.
People Also Ask
Is it possible to use “-v” option to check if a string is empty?
NO, it is not possible to use -v
option to check an empty string. You can use -v
option to check if a variable is empty in bash, but not for checking strings. Instead, you can use -z
, -n
, and other options to check if a string is empty.
How to Check if the String Contains Only Digits?
You can apply the regular expression =~
and ^[0-9]+$
within the if
condition. It checks whether the string contains only digits 0 to 9. Write the following syntax [[ $string =~ ^[0-9]+$ ]]
inside the if condition of your bash script to complete this task.
Can I use the “-z” option to check if a variable is empty in bash?
Yes, definitely. You can check if a variable is empty or not using the -z
option of the if else statement. If the option finds that the variable is empty, it returns the if block expression otherwise displays the else block expression.
How do you check if a string is in a list in bash?
To check if a string in a list is in bash, you can try using a for loop
. It will go through all the elements in the list and keep comparing each one until you find the string you want. If it matches, you will find the desired string. Check out this example to see how it works:
#!/bin/bash
string_list=("apple" "banana" "cherry" "date" "grape") # List of strings
target_string="cherry" # Target string to check
found=false # Flag to indicate if the target string is found in the list
for element in "${string_list[@]}"; do # Iterate through the list elements
if [ "$element" == "$target_string" ]; then # Compare the
current element with the target string
found=true
break
fi
done
if [ "$found" = true ]; then # Check if the target string was found in the list
echo "The target string '$target_string' is in the list."
else
echo "The target string '$target_string' is not in the list."
fi
As the “cherry” string is present in the string_list=(“apple” “banana” “cherry” “date” “grape”), the output will be: The target string ‘cherry’ is in the list.
Related Articles
- Check If a Variable Exists in Bash If Statement
- Check If An Input Argument Exists in Bash [4 Methods]
- Check If a String Contains Substring, Number & Letter in Bash
- How to Compare Strings in Bash With if Statement [6 Methods]
- How to Compare Numbers in Bash With If Statement [2 Methods]
- Check If Array Contains an Element in Bash [6 Methods]
- Bash if-else Condition in One-Line [2 Ways]
- Using grep in if Statement in Bash [4 Cases]
- How to Use Regex in Bash If Condition? [4 Cases]
- Bash rm If Exists [All Case Explained]
- What is ‘$?’ in the Bash if Statement? [8 Examples]
<< Go Back to If Else in Bash | Bash Conditional Statements | Bash Scripting Tutorial