FUNDAMENTALS A Complete Guide for Beginners
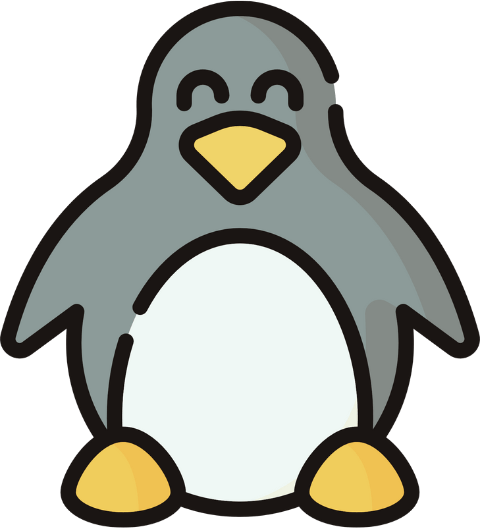
Bash refers to the Bourne-again-shell, a popular script used in Unix-like operating systems such as Ubuntu. In bash, a string is a series of characters enclosed with quotes. If a string contains a substring, letter, number, or any special character, can be checked with the if else condition. Checking string containment is essential if you are working with text data, implementing conditional logic, or parsing files. So no more worries. Here, I have explored different ways of checking string contents.
Why is It Useful to check if a String Contains Substring, Number & Letter in Bash?
In Bash scripting, processing and manipulating text data is one of the important tasks. Therefore, to control the bash script, it is vital to learn how to check a substring, number, letter, and special character from a string in bash. Some of the important uses are mentioned below:
- Data Extraction: If you need to extract any specific information from a lengthy string or any configuration files/log files, you can extract them by using the methods explained in this article.
- Data Validation: When you wish to check if a file contains valid data or the desired data you wanted, use these methods to validate the information.
- Text Processing and Manipulation: Manipulating text and extracting valuable information from a large text can be done by finding string contents. You can also format, transform, or filter text according to your needs.
- Log Parsing and Pattern Matching: To analyze error codes, timestamps, etc. from log files, checking whether a string contains another string or any errors is the easiest way. That’s how you can control the bash script. Moreover, for efficient pattern matching, this is a reliable way.
- Data Searching and Cleaning: If you want to remove any particular character, number, or string from a file, first search the specific data and then delete them to keep your dataset clean.
12 Methods to Find Substring, Number, Letter and Special Character in Bash
A string may contain a substring, numbers, letters, or special characters which can be checked in numerous ways. You can use different operator, special sign, or command to search the specific word, digit, or letter from the main string.
In this section, I will explain to you 12 easy methods to check string contents in bash that will allow you to process or parse any log/text files. You will have better control over the bash script after reading this article. Additionally, a case statement can also be used to compare strings which are explained here. Now let’s dive into the details.
1. Checking With “[[” Operator and Wildcards
Enclosing with the [[ operator and wildcards, the condition is set to find the substring, numbers, letters, and special characters from the main string. If you are working with simple text, this method will be efficient for its simple and flexible nature. You don’t need to import any external libraries as this feature is built-in in the bash shell.
To process large text, you can check the other methods explained in this article. Now, open the Ubuntu Terminal and write the following script inside the nano text editor to check string content with [[ operator and wildcards:
#!/bin/bash
string="Hi@123,I am using ubuntu"
substring="ubuntu"
numbers="123"
letters="Hi I am using ubuntu"
special_chars="@,"
# Substring Check
if [[ $string == *"$substring"* ]]; then
   echo "Substring '$substring' found in string"
else
   echo "Substring '$substring' not found in string"
fi
# Number Check
if [[ $string =~ [0-9] ]]; then
   echo "String contains numbers '$numbers'"
else
   echo "String does not contain numbers '$numbers'"
fi
# Letter Check
if [[ $string =~ [a-zA-Z] ]]; then
  echo "String contains letters '$letters'"
else
   echo "String does not contain letters '$letters'"
fi
# Special Character Check
if [[ $string =~ [^a-zA-Z0-9] ]]; then
   echo "String contains special characters '$special_chars'"
else
   echo "String does not contain special characters '$special_chars'"
fi
Here, the main string is ‘Hi@123,I am using ubuntu’ in the script. From this string, the contents will be found. ‘[[ $string == *”$substring”* ]]’ checks if the main string contains the substring ‘ubuntu’. Similarly, ‘[[ $string =~ [0-9] ]]’ checks the digit’s presence in the string. ‘[[ $string =~ [a-zA-Z] ]]’ condition is for checking letters. Finally, ‘[[ $string =~ [^a-zA-Z0-9] ]]’ checks whether the string has any special characters. The echo command echoes the quoted message.
In this image, you can see that the main string contains the substring ‘ubuntu’, numbers ‘123’, all the alphabetic letters ‘Hi I am using ubuntu’, and special characters ‘@,’ except all the alphanumeric characters. For more complex pattern matching, you can use the following methods.
2. Using “grep” Command for Checking String Contents
This method is useful for analyzing large text and maintaining complex patterns. You can search for multiple parameters using the pipe (|) operator along with grep. Though this powerful command is case-sensitive, use it carefully. Now check the following to learn the entire process of checking substrings, numbers, letters, and special characters:
#!/bin/bash
string="Hello@123, i am using ubuntu"
# Check if the input string contains a substring, numbers, letters, special characters
if [ -n "$string" ]; then
   # Find substring using grep
   substring=$(echo "$string" | grep -o "ubuntu")
   # Find numbers using grep
   numbers=$(echo "$string" | grep -o "[0-9]*")
   # Find letters using grep
   letters=$(echo "$string" | grep -o "[A-Za-z]*")
   # Find special characters using grep
   special_chars=$(echo "$string" | grep -o "[^A-Za-z0-9]*")
   echo "Substring: $substring"
   echo "Numbers: $numbers"
   echo "Letters: $letters"
   echo "Special Characters: $special_chars"
else
   echo "The input string is empty."
fi
Here, main string=‘Hello@123, i am using ubuntu’ and I am extracting the substring ‘ubuntu’ and digits, letters, and special characters from this string using grep. The grep -o option is used to instruct taking only the matched pattern as an output.
This picture shows that the substring, numbers, letters, and special characters are extracted from the main string.
3. Using “expr” Command for Checking String Contents
The ‘expr’ command is used to search string contents such as substrings, numbers, etc. Though it is not usually used in modern bash script, it can be useful to handle simple text. The compatibility of this method is higher as it is available in almost all UNIX-like operating systems. Therefore, follow the script below to know the whole process of checking string content:
#!/bin/bash
full_string="Hello@123, I am using Ubuntu"
# Initialize variables to store extracted values
substring="Ubuntu"
numbers="123"
letters="Hello I am using Ubuntu"
special_chars="@ ,"
# Check if the full string is not empty
if [ -n "$full_string" ]; then
# Find a substring using `expr`
substring_expr="\(Ubuntu*\)"
expr_result=$(expr "$full_string" : ".*$substring_expr")
if [ -n "$expr_result" ]; then
substring="${substring}"
fi
# Find numbers using `expr`
numbers_expr=" \([0-9]*\)"
expr_result=$(expr "$full_string" : ".*$numbers_expr")
if [ -n "$expr_result" ]; then
numbers="${numbers}"
fi
# Find letters using `expr`
letters_expr=" \([A-Za-z ]*\)"
expr_result=$(expr "$full_string" : ".*$letters_expr")
if [ -n "$expr_result" ]; then
letters="${letters}"
fi
# Find special characters using `expr`
special_chars_expr="\(.*\)"
expr_result=$(expr "$full_string" : ".*$special_chars_expr")
if [ -n "$expr_result" ]; then
special_chars="${special_chars}"
fi
else
echo "The input string is empty."
fi
echo "Substring: $substring"
echo "Numbers: $numbers"
echo "Letters: $letters"
echo "Special Characters: $special_chars"
Firstly, you have to take the full string and define the variables that you want to extract from the full string. After that, the expr command takes the full string as input and searches the defined substring, numbers, letters, and special characters in the full string. If it finds the matched patterns, it will print them otherwise it will show the input string is empty.
After running the bash expr.sh, you will get the output as shown in this picture. You can see that the expr command successfully finds the defined substring, numbers, letters, and special characters.
4. Checking With Regular Expression (regex) in Bash
The efficiency of this method made it a popular text-parsing tool. It is a widely used technique to check the contents of the string or text files. You can check complex patterns like email addresses, and urls with this regular expression method. For complex searches, it can be a bit slower. However, everyone feels comfortable using this well-structured text-processing kit. Check the bash script to know how to use regex
for checking string content:
#!/bin/bash
string="Hi@123, I am using ubuntu"
substring="ubuntu"
numbers="123"
letters="Hello I am using ubuntu"
special_chars="@ ,"
# Find Substring
if [[ $string =~ $substring ]]; then
echo "Substring '$substring' found in string"
else
echo "Substring '$substring' not found in string"
fi
# Find Numbers
if [[ $string =~ [0-9] ]]; then
echo "String contains numbers '$numbers'"
else
echo "String does not contain numbers '$numbers'"
fi
# Find Letters
if [[ $string =~ [a-zA-Z] ]]; then
echo "String contains letters '$letters'"
else
echo "String does not contain letters '$letters'"
fi
# Find Special Characters
if [[ $string =~ [^a-zA-Z0-9] ]]; then
echo "String contains special characters '$special_chars'"
else
echo "String does not contain special characters '$special_chars'"
fi
The ‘=~’ operator is used for pattern matching in the regular expression method. With this operator, it starts to match the specified pattern in the string and prints as an output otherwise it will print the the line defined in the echoes under the else section.
The printed output is shown in the picture. You can see the substring, numbers, letters, and special characters are successfully printed as defined in the variable section in the bash script.
5. Using Function for Checking String Contents
Once you define a function, it can be used multiple times when you need it. This reusability of function made this method convenient to users. In this example, I will explain how you can create a function to check the string contents. See the script below:
#!/bin/bash
# Function to check for a substring
substring_check() {
local string="$1"
local substring="$2"
if [[ $string =~ $substring ]]; then
echo "Substring '$substring' found in string"
else
echo "Substring '$substring' not found in string"
fi
}
# Function to check for numbers
numbers_check() {
local string="$1"
if [[ $string =~ [0-9] ]]; then
echo "String contains numbers"
else
echo "String does not contain numbers"
fi
}
# Function to check for letters
letters_check() {
local string="$1"
if [[ $string =~ [a-zA-Z] ]]; then
echo "String contains letters"
else
echo "String does not contain letters"
fi
}
# Function to check for special characters
special_chars_check() {
local string="$1"
if [[ $string =~ [^a-zA-Z0-9] ]]; then
echo "String contains special characters"
else
echo "String does not contain special characters"
fi
}
string="Hi@123, I am using ubuntu"
# Call the functions to perform the checks
substring_check "$string" "ubuntu"
numbers_check "$string"
letters_check "$string"
special_chars_check "$string"
Here, 4 different functions is generated to search substring, numbers, letters, and special characters. The substring_check function checks the substring in the input string. Similarly, numbers_check, letters_check, and special_chars_check functions do similar their respective task.
This is the output of the function.sh bash script. Here, you can see that the input string contains the specified substring, numbers, letters, and special characters.
6. Working With “awk” Command to Check String Contents
The awk command offers several advantages in analyzing and parsing structured or unstructured text data. It can be used directly in the command line which makes it more suitable. Moreover, it has several built-in variables that allow you to perform efficient data manipulation. Now, check the following to know the detailed procedure:
#!/bin/bash
string="Hello@123, I am using ubuntu"
# Extracting a substring
substring=$(echo "$string" | awk 'match($0, /ubuntu/) {print substr($0, RSTART, RLENGTH)}')
if [ -n "$substring" ]; then
echo "Substring found in the string: $substring"
else
echo "Substring not found in the string"
fi
# Extracting numbers
numbers=$(echo "$string" | awk '{gsub(/[^0-9]/, " "); print $0}')
if [ -n "$numbers" ]; then
echo "Numbers found in the string: $numbers"
else
echo "Numbers not found in the string"
fi
# Extracting letters
letters=$(echo "$string" | awk '{gsub(/[0-9@,]/, " "); print $0}')
if [ -n "$letters" ]; then
echo "Letters found in the string: $letters"
else
echo "Letters not found in the string"
fi
# Extracting special characters
special_chars=$(echo "$string" | awk '{gsub(/[A-Za-z0-9 ]/, " "); print $0}')
if [ -n "$special_chars" ]; then
echo "Special characters found in the string: $special_chars"
else
echo "Special characters not found in the string"
fi
Here, the awk command initiates substring matching using the ‘match’ function and finds the starting of the match with RSTART. Then find the match length with RLENGTH and subtract the specific substring from the input string. In search of numbers, it utilizes gsub to remove other characters except for numbers and replace them with blank spaces. It does the same task in search of letters and special characters using gsub.
Here, you can see the output has been printed successfully. Additionally, you can see some extra spaces with the defined substring, numbers, letters, and special characters for using the gsub function in the awk command.
7. Using “sed” Command for Checking String Contents
Checking string contents with the sed command is a powerful way in bash scripting. You can search substrings, numbers, letters, and special characters from a large text file. It is available in all Unix-like operating systems like Ubuntu. In this example, I will show you how to use the ‘sed’ command to check string contents. Check the script mentioned below to accomplish this task:
#!/bin/bash
string="Hi@123, I am using ubuntu"
# Extract 'ubuntu' substring
substring=$(echo "$string" | sed -n 's/.* \([[:alnum:]]*\)$/\1/p')
if [ "$substring" == "ubuntu" ]; then
echo "Substring 'ubuntu' found in the string: $substring"
else
echo "Substring 'ubuntu' not found in the string"
fi
# Extracting numbers
numbers=$(echo "$string" | sed 's/[^0-9]//g')
if [ -n "$numbers" ]; then
echo "Numbers found in the string: $numbers"
else
echo "Numbers not found in the string"
fi
# Extracting letters
letters=$(echo "$string" | sed 's/[^a-zA-Z ]//g')
if [ -n "$letters" ]; then
echo "Letters found in the string: $letters"
else
echo "Letters not found in the string"
fi
# Extracting special characters
special_chars=$(echo "$string" | sed 's/[a-zA-Z0-9 ]//g')
if [ -n "$special_chars" ]; then
echo "Special characters found in the string: $special_chars"
else
echo "Special characters not found in the string"
fi
Here, sed command is used to extract the particle string contents from the original string. The sed with -n option suppresses the automatic printing and matches the substring then prints the output into the substring variable. In a similar way, it prints the numbers, letters, and special characters in their defined variables.
You can see the bash sed.sh script’s output in this image. The expected string contents are found after running the bash script.
8. Checking With “perl” Command in Bash
For sting manipulation like checking substring, numbers, etc. perl is a great tool to use. This versatile text processing tool allows users to match precise patterns precisely. Additionally, it has regular expression capabilities which makes it suitable for processing text data sets. However, if you want to have more simpler tool, you can go for sed or grep. Now, check the procedure of checking string contents with perl
command:
#!/bin/bash
string="Hi@123, I am using ubuntu"
# Extract 'ubuntu' substring
substring=$(echo "$string" | perl -nle 'print $1 if /(\bubuntu\b)/')
if [ "$substring" == "ubuntu" ]; then
echo "Substring 'ubuntu' found in the string: $substring"
else
echo "Substring 'ubuntu' not found in the string"
fi
# Extracting numbers
numbers=$(echo "$string" | perl -nle 's/\D/ /g; print')
if [ -n "$numbers" ]; then
echo "Numbers found in the string: $numbers"
else
echo "Numbers not found in the string"
fi
# Extracting letters
letters=$(echo "$string" | perl -nle 's/[^a-zA-Z ]/ /g; print')
if [ -n "$letters" ]; then
echo "Letters found in the string: $letters"
else
echo "Letters not found in the string"
fi
# Extracting special characters
special_chars=$(echo "$string" | perl -nle 's/[a-zA-Z0-9 ]//g; print')
if [ -n "$special_chars" ]; then
echo "Special characters found in the string: $special_chars"
else
echo "Special characters not found in the string"
fi
First, a string is taken as input. The perl with -nle option runs perl code for each line of input. If the line contains Ubuntu, it will print the substring in the output otherwise it will show that the substring is not found in the string. Similarly, it checks numbers, letters, and special characters.
The bash perl.sh output contains all the string contents.
9. Using “tr” Command to Check String Contents
tr stands for translate and it is primarily used for translating and deleting specific text from the texts. Moreover, it can be used to find string contents including substrings, numbers, etc. However, this command is unable to provide error messages in detail. Therefore, you can opt for the sed or awk command to avoid this problem. Now, see the bash script for finding string contents with tr
command:
#!/bin/bash
string="Hi@123, I am using ubuntu"
# Initialize variables to store extracted values
substring=""
numbers=""
letters=""
special_chars=""
# Extract 'ubuntu' substring
if [[ $string == *ubuntu* ]]; then
substring="ubuntu"
fi
# Extract numbers
numbers=$(echo "$string" | tr -cd '0-9')
# Extract letters
letters=$(echo "$string" | tr -cd 'a-zA-Z ')
# Extract special characters
special_chars=$(echo "$string" | tr -cd '@,')
# Check if values were found and print them
if [ -n "$substring" ]; then
echo "Substring 'ubuntu' found in the string: $substring"
else
echo "Substring 'ubuntu' not found in the string"
fi
if [ -n "$numbers" ]; then
echo "Numbers found in the string: $numbers"
else
echo "Numbers not found in the string"
fi
if [ -n "$letters" ]; then
echo "Letters found in the string: $letters"
else
echo "Letters not found in the string"
fi
if [ -n "$special_chars" ]; then
echo "Special characters found in the string: $special_chars"
else
echo "Special characters not found in the string"
fi
First of all, variables are taken to store the output values. Then as an input, a string is taken. After that, the tr command finds the specified ubuntu substring from the string and deletes the other characters with the -cd option. To find numbers, it prints the digit 0-9 and deletes the other characters. In the same way, it finds letters and special characters.
This image shows that all the string contents are extracted properly from the input string.
10. Checking With Parameter Expansion
Parameter expansion provides a straightforward way to perform string operations such as checking string contents. It is usually efficient for small or medium-sized strings. Moreover, it has the potential to make bash script readable and simple to all. To know the procedure of how can you use it, do the following:
#!/bin/bash
string="Hi@123, I am using ubuntu"
# Extract 'ubuntu' substring
substring="${string##* }"
if [ "$substring" == "ubuntu" ]; then
echo "Substring 'ubuntu' found in the string: $substring"
else
echo "Substring 'ubuntu' not found in the string"
fi
# Extracting numbers
numbers="${string//[^0-9]/ }"
if [ -n "$numbers" ]; then
echo "Numbers found in the string: $numbers"
else
echo "Numbers not found in the string"
fi
# Extracting letters
letters="${string//[^a-zA-Z ]/ }"
if [ -n "$letters" ]; then
echo "Letters found in the string: $letters"
else
echo "Letters not found in the string"
fi
# Extracting special characters
special_chars="${string//[^@,]/ }"
if [ -n "$special_chars" ]; then
echo "Special characters found in the string: $special_chars"
else
echo "Special characters not found in the string"
fi
Here, parameter expansion is used to extract the substring after the last space in the string, and  “${string##* }” trims everything before the last space. Thus, substring ubuntu is extracted. Numbers, letters, and special characters are also found similarly.
You can see in the picture that the string contents are extracted from the input string.
11. Using “read” Command for Checking String Contents in Bash
The read command interacts with the user by reading a line from the user’s input. It provides an easy way to assign variables. Therefore, it can be used to check substring, letters, and other characters from any input string. Let’s dive into the detailed procedure of checking string content with read
command:
#!/bin/bash
string="Hi@123, I am using ubuntu"
# Initialize variables to store extracted values
substring="ubuntu"
numbers="123"
letters="Hi I am using ubuntu"
special_chars="@ ,"
# Create a temporary file to hold the string
temp_file=$(mktemp)
echo "$string" > "$temp_file"
# Read words from the string
while read -ra words; do
for word in "${words[@]}"; do
if [[ "$word" == "ubuntu" && -z "$substring" ]]; then
substring="$word"
elif [[ "$word" =~ ^[0-9]+$ ]]; then
numbers="$numbers"
elif [[ "$word" =~ ^[a-zA-Z[:space:]]+$ ]]; then
letters="$letters"
elif [[ ! "$word" =~ ^[a-zA-Z0-9[:space:]]+$ ]]; then
special_chars="$special_chars"
fi
done
done < "$temp_file"
# Check if values were found and print them
if [ -n "$substring" ]; then
echo "Substring 'ubuntu' found in the string: $substring"
else
echo "Substring 'ubuntu' not found in the string"
fi
if [ -n "$numbers" ]; then
echo "Numbers found in the string: $numbers"
else
echo "Numbers not found in the string"
fi
if [ -n "$letters" ]; then
echo "Letters found in the string: $letters"
else
echo "Letters not found in the string"
fi
if [ -n "$special_chars" ]; then
echo "Special characters found in the string: $special_chars"
else
echo "Special characters not found in the string"
fi
# Clean up temporary file
rm "$temp_file"
Here, the variables are initialized to store the extracted values. Then a temporary file is created to hold the original string content. The read
command with the -ra
option within the while loop reads the temporary file line and converts the line intro array of words using this statement ${words[@]}. After that, the categories of the variable are differentiated based on the contents of each word and check whether it is a substring, any letter, or any other character. The -n
option checks for the non-empty value of the variable and prints the result accordingly. Lastly, the temporary file is cleaned by the rm command.
You can see in this image that the read.sh bash script gives the accurate output.
12. Performing Case Statement for Checking String Contents
You can check if a string contains a substring, numbers, letters, or special characters with a case statement in bash. The ability to match multiple patterns of the case statement increases its efficiency. In this example, I will show you the step-by-step process of checking string contents. Let’s start checking string content using case
statement:
#!/bin/bash
string="Hi@123, I am using ubuntu"
# Check if the string contains 'ubuntu'
case "$string" in
   *ubuntu*)
       echo "String contains 'ubuntu'"
       ;;
   *)
       echo "String does not contain 'ubuntu'"
       ;;
esac
# Check if the string contains numbers
case "$string" in
   *[0-9]*)
       echo "String contains numbers"
       ;;
   *)Â
      echo "String does not contain numbers"
       ;;
esac
# Check if the string contains letters
case "$string" in
   *[a-zA-Z]*)
       echo "String contains letters"
       ;;
   *)
       echo "String does not contain letters"
       ;;
esac
# Check if the string contains special characters
case "$string" in
   *[!a-zA-Z0-9]*)
       echo "String contains special characters"
       ;;
   *)
       echo "String does not contain special characters"
       ;;
esac
Here, four case statements are used to check string contents. 1st case checks if the string contains substring ubuntu, 2nd case checks numbers 0-9. The 3rd case checks letters and the 4th case checks special characters. With the esac command, the case statement is ended.
You can see in this image that the string contains Ubuntu, numbers, letters, and special characters.
Conclusion
In this article, I have explained 12 methods to check string contents including substring, numbers, letters, and special characters. Under the if-else condition, grep, expr, awk, sed, tr, and many more commands are utilized here. The case statement is used in method 12 for checking string contents. Every method has its pros and cons. Therefore, read the description of each method carefully to know more. Accordingly, choose the best possible method to accomplish your job. Good luck!
People Also Ask
Related Articles
- Check If a Variable Exists in Bash If Statement
- Check If An Input Argument Exists in Bash [4 Methods]
- Check If a String is Empty or Not in Bash [5 Methods]
- How to Compare Strings in Bash With if Statement [6 Methods]
- How to Compare Numbers in Bash With If Statement [2 Methods]
- Check If Array Contains an Element in Bash [6 Methods]
- Bash if-else Condition in One-Line [2 Ways]
- Using grep in if Statement in Bash [4 Cases]
- How to Use Regex in Bash If Condition? [4 Cases]
- Bash rm If Exists [All Case Explained]
- What is ‘$?’ in the Bash if Statement? [8 Examples]
<< Go Back to If Else in Bash | Bash Conditional Statements | Bash Scripting Tutorial