FUNDAMENTALS A Complete Guide for Beginners
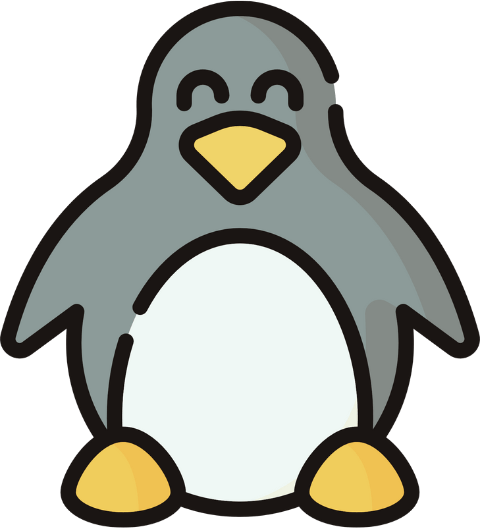
The length of a string refers to finding the number of characters in a string. It is essential to find length for text processing, input validation, and parse text. The basic syntax to find the length of a string is the ${#string}. The syntax is straightforward and does not require any external command to find the length.
Let’s take a look at different methods to find the length of a string.
6 Ways to Find the Length of a String
Besides the basic syntax hash(#
)with curly brackets, there are some other commands like the wc command, awk command, and expr command used to find the length of a string. In this part, I will show 6 methods to find the length of a string in bash.
1. Using Parameter Expansion With “#”
Parameter expansion refers to the process of manipulating and expanding the values of the variable. Using this # symbol with the parameter expansion, you can get the length of the given variable’s value. Generally, the hash(#) symbol is used for commenting, but it is also used as a variable substitution and special parameter in bash.
To know the length of a string, go through the following code:
#!/bin/bash
String="Hello, World!"
length=${#String}
echo "The length of the string is: $length"
The string has been declared in a variable string. Then the length of the string is stored in the length variable. The#
in the${#String}
calculates the length of the following string variable.
From the output, you can see the length is counted using the parameter expansion. Rename the file name length.sh according to your preference.
Note: The {#$string} syntax, expr command, while loop counts the leading white space of the first line of a text as a character but the wc and awk commands do not count these spaces as characters.
A. Using Loop to Find the Length of a String
To find the length of a large string use the loop with the {#string} syntax for its efficient and simple structure. Here’s how:
#!/bin/bash
string='Hello, Bash!'
length=0
# Iterate through each character in the string
for (( i=0; i<${#string}; i++ )); do
length=$((length + 1))
done
echo "The length of the string is $length"
The for loop iterates over each character in a line. The loop is initialized with i=0 and continues as long as “i” is less than the length of the string(${#string})
. It will increment thei
with each iteration.
B. Using Function to Find the Length of String in Bash
To count the length frequently in the bash script, explore the following code:
#!/bin/bash
function string_length {
string=$1
echo ${#string}
}
string_length 'Hello, World!'
Thefunction string_length
line declared a function whose name is the string length. The argument in the function has been accessed using the position variable$1
and storing the input in the string variable The{#string}
prints the length of the string.
Using this function, one can find different string lengths by replacing the input argument.
2. Using “wc” Command
The wc command is the command line utility which stands for word count. You can use the command to count the number of lines, words, and characters.
To find the length of a string using thewc
command, follow the below script:
#!/bin/bash
string="Hello, Bash!"
length=$(echo -n $string | wc -m)
echo "The length of the string is $length"
The string is declared in a string variable with a double quote. The-n
option with the echo command prints the string without a newline character. The output of theecho
command has been piped to thewc
command using the pipe(|) operator. The wc command with the-m
option counts the character of the output of theecho
command.
3. Using “expr” Command
The expr command is the command line utility that evaluates the expression following this command. The basic syntax of the expr command isexpr EXPRESSION
. Replace the expression according to user preference.
To find the length of a string use the length operator with theexpr
command. Here is how:
#!/bin/bash
my_string="Hello, World!"
length=$(expr length "$my_string")
echo $length
The$(expr length "$my_string")
returns the length of the my_string. The length “$my_string” is the expression in theexpr
command.
Note: To find the length of a string you can use the expr command with the: '.*'
. Here is how:
#!/bin/bash
string="Hello, World!"
length=$(expr "$string" : '.*')
echo "The length of the string is: $length"
The comparison operator:
count the common characters between the previous and latter variable of “:”. The.*
matches the entire string. The “.*” can be replaced by the string itself. However, the regular expression may not be portable with different versions of theexpr
command.
4. Using the “awk” Command
The awk command is the command line utility for text processing and pattern matching. The basic syntax of the awk command isawk 'pattern { action }' input_file
. Here the pattern indicates the conditions or regular expression and the action to be performed when the pattern matches. If the pattern is missing then the action will be performed on the whole string. The input_file can be piped with the echo command.
To find the length of the string, use theawk
command with the length operator. Here is how:
#!/bin/bash
my_string="This is a sample string"
echo $my_string | awk '{print length}'
The output of theecho $my_string
command is piped to theawk
command. Here the print length is the action that will be performed on the string. The awk command calculates the length using the built-inlength
operator and prints the output to the terminal.
5. Using “perl” to Find the Length of String
To find the length of a string, use theperl
command with the pipe operator. Here’s how it looks like:
#!/bin/bash
string="It is the new string. The length of the string should be counted."
echo "$string" | perl -nle "print length"
The pipe operator takes the output from theecho
command and uses it as the input for theperl
command. The-n
option loops around every line of input. But in this case, it calculates the length of entire the string. The-l
option is used to add a newline character to each print statement automatically. The-e
option allows execution of the script.
From the image, you can see the number of characters is about 65.
6. Using the While loop
The while loop with the read command allows to read input, iterate through data, and perform operations. But in this case, it simply reads the string character by character and counts the length.
To find the length using the while loop, follow this script:
#!/bin/bash
string="This is a new string"
n=0
while read -n1 character; do
n=$((n+1));
done <<(echo -n "$string")
echo "Length of the string is $n "
Then
variable is initialized with a value of 0 and the variable counts the characters in the string. Theread
command reads each character from the input and stores it into character. Inside the loop, the variable n is increased by 1 in each iteration. In thedone <<(echo -n "$string")
, the output of theecho
command which is the string, is redirected as input into the while loop. The-n
option is used to print characters without newline characters.
Here in the image, you can see the length of the string which is 20. Rename the script according to your preference.
Find the Length of String Without White Space in Bash
The white space refers to the blank characters that represent the vertical or horizontal space in the text. If a line contains white space, the spaces are considered a character and counted as a string length.
To find the length of a string without counting the space, explore the following code:
#!/bin/bash
originalString="Hello, this is a string with spaces."
# Remove whitespaces from the string
stringWithoutSpaces=$(echo "$originalString" | tr -d ' ')
# Calculate the length of the string without whitespaces
lengthWithoutSpaces=${#stringWithoutSpaces}
echo "Original string: $originalString"
echo "String without spaces: $stringWithoutSpaces"
echo "Length of the string without spaces: $lengthWithoutSpaces"
The original string is piped to the tr command in echo “$originalString” | tr. The tr command is used to delete the white space in the string. With the -d option the tr command deletes the (‘ ‘) space. Then the {#string} is used to count the length of the string.
Conclusion
The article shows a few methods by which the user can easily learn how to find the length for efficient text processing in bash scripting. Among these methods, the parameter expansion is the simplest and easiest method to calculate the length, for large strings, use the for loop with the parameter expansion to find the length.
People Also Ask
How to verify the length of a string?
Verifying a string length refers to the process of checking if a string fulfills a minimum length. It is important to verify the length of a string for handling data and prevent invalid results.
To verify the length, explore the following code:
#!/bin/bash
str="Hello, World!"
[ $(echo -n "$str" | wc -c) -gt 6 ] && echo 'valid' || echo 'invalid'
Here, The output of theecho
command has been piped to thewc
command as input. The test conditional[...-gt 6]
is used to check if the value obtained in the$(echo -n "$str" | wc -c)
is greater than 6. If the result of the[$(echo -n "$str" | wc -c) -gt 6 ]
command is true then the logical AND && operator will be executed. If it returns false then it will execute the logical OR || operator.
How to check string length 0 in Bash?
To check if a string length is 0 or an empty string, use the-z
conditional which returns true if the string length is 0 and returns false if the string length is not equal to 0. You can also use the==
operator when comparing with an empty string to check if the string length is 0. Here how it looks like:
#!/bin/bash
string=""
if [ -z "$string" ]; then
echo "String is empty."
else
echo "String is not empty."
fi
To check if a string is empty or not, use the-z
option. If it is empty then it returns the true value and if the string is not empty it will return false.
How to compare string length in bash?
To compare the length of two strings whether it is equal or not, use the following code:
#!/bin/bash
if [[ ${#string} == ${#string_two} ]]; then
echo "Strings are equal by length."
else
echo "Strings are not equal by length."
fi
You can use the double bracket ([[ ]]) for the conditional operation. And use the double equal sign for equality comparison within the double bracket. In the double bracket if the condition is true then it prints the block in the if statement otherwise it prints the block of the else statement.
Related Articles
<< Go Back to Bash String Operations | Bash String | Bash Scripting Tutorial