FUNDAMENTALS A Complete Guide for Beginners
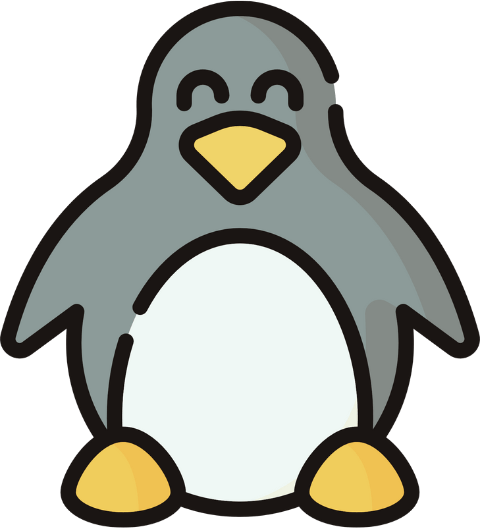
To return an array from the function in Bash, use the following methods:
- Using Global Variable:
my_function() declare my_array { echo my_array;}
- Using Command Substitution:
variable=$(my_function)
- Using Process Substitution:
variable=$(< <(my_function))
- Using IFS Variable:
IFS=' ' variable=$(my_function)
- Using “nameref” Feature:
my_function() {local -n my_array=$1;} declare ref_array
- Using Here-document:
my_function() {cat EOF my_array EOF;}
- Using “printf” Command:
my_function() {printf "%s\n" "${my_array[@]}";}
Bash does not have a built-in way to return an array directly from the function. Nevertheless, declaring a global variable or using the command substitution method helps to get an array from the bash function. In this article, I will explore 7 methods to seamlessly return arrays from functions in Bash, assisting you to unleash your scripting potential.
1. Using Global Variable
One of the most common approaches to return an array from the function in bash is to define a global variable that can be called from both inside and outside of the function. It offers more simplicity, making the script easy to understand. Here’s a Bash script to return an array using a global variable:
#!/bin/bash
declare -a array
function get_array(){
array=("apple" "banana" "orange" "cherry")
}
get_array
echo "${array[0]}"
echo "${array[1]}"
echo "${array[2]}"
echo "${array[3]}"
Firstly,declare -a array
declares a global variable “array” using the declare command and the -a
option indicates that the variable “array” is an indexed array. After that, a function named get_array
is defined. Inside the function, array=("apple" "banana" "orange" "cherry")
assigns the four elements to the variable “array”. When the function is called, echo "${array[0]}"
prints the 1st element of the array. Similarly, “${array[1]}” “${array[2]}” “${array[3]}” are used to print 2nd, 3rd, and 4th array element.
The script returns all the array elements from the function using a global variable.
Note: You can print the entire array in one line by placing the echo "${array[@]}"
line after the function call.
2. Using Command Substitution
Integrating the command substitution method with the echo
command is a straightforward way to catch the output of a function. In this case, the output of the function is an array. Check this script to know how to return an array using the echo command with the command substitution method:
#!/bin/bash
function return_array(){
local array=(1 2 3 4 5 6)
for element in "${array[@]}"; do
echo "$element"
done
}
# Call the function and capture its output using command substitution
result=$(return_array)
# Print the captured output, with each element on a separate line
echo "$result"
Inside the return_array
function, a local variable “array” with values (1 2 3 4 5 6) is declared first. Then a for loop iterates over each array element and prints them separately using echo "$element"
. After that, result=$(return_array)
uses command substitution to capture the function’s output. Finally, echo "$result"
displays the captured output.
You can see that the function returns each array element using command substitution with the echo command.
3. Using Process Substitution
To gracefully handle the function’s output, consider utilizing the process substitution method with the echo
command. This strategy effectively returns the array from a function using the $(< <(function_name))
syntax. Here’s how:
#!/bin/bash
function get_array() {
local array=("apple" "banana" "orange" "cherry")
for element in "${array[@]}"; do
echo "$element"
done
}
result=$(< <(get_array))
echo "$result"
In this script, the get_array
function includes an array with 4 elements (“apple” “banana” “orange” “cherry”). The for loop
iterates over the array elements and shows each array in the terminal using the echo
command. Later, result=$(< <(get_array))
uses the process substitution approach to catch the function’s output and print it on the console with echo "$result"
.
Returning the array elements from the function smoothly.
4. Using IFS Variable
IFS stands for Internal Field Separator which is a special variable in bash used to split the input string into fields based on the character set to an IFS. In this example, the IFS is set to a single space character that will separate the array into individual elements using space. Afterward, the individual array element will be returned with the help of command substitution and echo
command.
Check the full bash script to know how the IFS variable works to return an array from the Bash function:
#!/bin/bash
function return_array(){
local array=(1 2 3 4 5 6)
echo "${array[@]}"
}
IFS=' '
array=$(return_array)
individual_elements=($array)
for element in "${individual_elements[@]}"; do
echo "$element"
done
This Bash script first defines a function called return_array
that creates a local array variable named “array” containing integers from 1 to 6. echo "${array[@]}"
echoes all the array elements separated by spaces.
The script then captures the output of the return_array
function into a variable “array” using command substitution. Afterward, individual_elements=($array)
splits the “array” into individual elements using the IFS value.
Finally, it iterates over each element of the “individual_elements” array using a for loop
and prints each element of the “individual_elements” array.
As you can see, the integer values of the array are printed in the terminal.
5. Using “nameref” Feature
Even though Bash cannot directly return arrays from functions, it offers the option to generate a nameref using the -n
parameter to manipulate the array directly. Nameref is a useful feature in bash that allows one to create a reference to a variable with another name, where the reference variable behaves like an alias of the original variable. Changes to the reference variable affect the original variable, and vice versa. Check out the script below to return an array using nameref
:
#!/bin/bash
# Define function to operate on an array
function get_array {
local -n array=$1 # Create a nameref
array=("apple" "banana" "orange" "cherry") # Use the nameref to assign values
}
# Declare an array to hold the result
declare -a result_array
# Call the function with the reference to the result_array
get_array result_array
for element in "${result_array[@]}"; do
echo "$element"
done
Inside the get_array
function, local -n array=$1
declares a local variable “array” and the -n
option makes it a nameref. This nameref is then assigned the value of the first argument ($1)
provided to the function. This means that the “array” which contains 4 elements (“apple” “banana” “orange” “cherry”) becomes a reference to the array passed to the function.
After that, declare -a result_array
declares a “result_array” that holds the result returned by the “get_array” function. Consequently, get_array result_array
calls the get_array function and passes “result_array” as an argument, allowing “get_array” to modify the “result_array” directly via the nameref. Lastly, the for loop
iterates over the array elements and prints them in the terminal.
Upon executing the script, it shows all the elements of the array.
6. Using Here-document
The here-document passes text into a command or script. To return an array from the function using here-doc, incorporate the mapfile
command that converts the text of the here-document into an array. Here’s how:
#!/bin/bash
# Function that uses a here-document to return an array
function return_array {
cat <<EOF
1
2
3
4
5
EOF
}
# Capture the output in an array using mapfile
mapfile -t my_array < <(return_array)
# Access the array
echo "Array elements: ${my_array[@]}"
In this script, the return_array
function uses the cat command to display the content within the delimiter EOF…EOF
. Then, mapfile -t my_array < <(return_array)
captures the output of the function and converts the text into an array. Here, the -t
option removes trailing newline characters from each line read. Lastly, echo "Array elements: ${my_array[@]}"
prints out all the elements of the “my_array” array.
You can notice that the script has displayed all the array elements.
7. Using “printf” Command
The printf
command in Bash prints the text or variables to the standard output. Blending the “printf” command with the function provides a compelling way to return an array from the Bash function. See the following script to accomplish this task:
#!/bin/bash
get_array() {
local array=("apple" "banana" "orange" "cherry")
printf "%s\n" "${array[@]}"
}
get_array
The get_array
function contains a local variable “array” which has 4 elements. After calling the function, printf "%s\n" "${array[@]}"
prints all the array elements on a separate line in the terminal.
Getting the array from the function using the “printf” command.
Conclusion
Returning arrays from Bash functions is a daunting task as Bash does not provide any direct ways to do it. But don’t worry, I have shown you 7 methods that can be used to return an array from a function in bash. Choose the method that works best for you. Good luck!
People Also Ask
Can a Bash function return an array?
Bash function can’t return an array like other programming languages. However, you can simulate the task using a global or IFS variable. Moreover, you can apply command substitution or nameref feature to achieve this task.
How can a function return an array in Bash?
To return an array from a function in bash, create a function named my_function
, then define a global variable global_var=(1 2 3 4)
containing all the array elements (1 2 3 4). After that, call the function and use echo "${global_var[@]}"
to print the array values in the terminal.
Can a function return two arrays?
Yes definitely, a function can return two arrays. To do so, define two arrays as follows: array1=(red blue green) and array2=(apple banana orange), where “array1” and “array2” are the names of the global variables. After that, print the array elements with echo "${array1[@]}"
and echo "${array2[@]}"
.
How to convert array into string in Bash?
To convert an array into a string, you can use parameter expansion that expands each element of the array into separate words. Suppose, a variable named “array” has 3 elements (“This” “is” “Linux”), to make them a string “This is Linux”, use echo "${array[@]}"
syntax.
How to return an associative array from Bash function?
An associative array is a collection of key-value pairs where each key is unique within the array. To return an associative array from the function in bash, check the script below:
#!/bin/bash
# Define a function to populate an associative array
populate_array() {
local -n array_ref=$1 # Create a reference to the array passed as argument
array_ref["key1"]="value1"
array_ref["key2"]="value2"
array_ref["key3"]="value3"
}
# Declare an empty associative array
declare -A my_array
# Call the function to populate the array
populate_array my_array
# Access the elements of the array
echo "Key1: ${my_array["key1"]}"
echo "Key2: ${my_array["key2"]}"
echo "Key3: ${my_array["key3"]}"
Related Articles
- How to Exit From Function in Bash? [5 Ways]
- How to Return Exit status “0” in Bash [6 Cases]
- How to Return String From Bash Function [4 Methods]
- How to Return Boolean From Bash Function [6 Methods]
<< Go Back to Return Values From Bash Function | Bash Functions | Bash Scripting Tutorial