FUNDAMENTALS A Complete Guide for Beginners
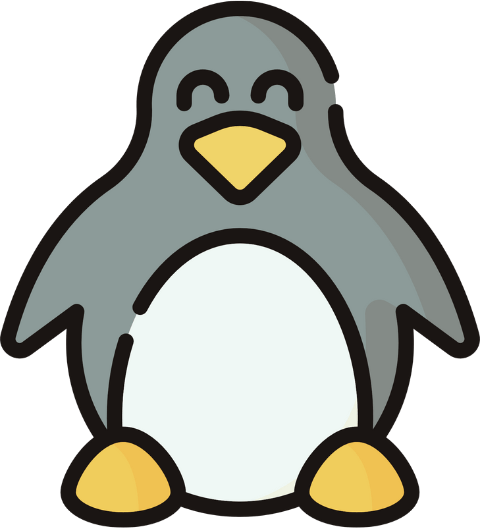
While working with the bash function, you may encounter difficulties in exiting the function. However, there are some effective methods such as utilizing return, exit, break, etc., commands within scripts to resolve this issue. This article will demonstrate five ways to exit bash functions, along with addressing some common problems and solutions that may encountered during the process.
5 Easy Ways to Exit From Bash Function
In bash scripting, exiting a function is one of the most common tasks that everyone needs to know. In this section, I will go through 5 ways to exit the bash function in detail, so let’s know them.
1. Using “return” Statement
To exit from a bash function, use the return
statement. Once the function encounters the return statement, it stops executing the function. Moreover, the “return” statement returns an integer value between 0 to 255 from the bash function. Value 0 indicates success and others are used to indicate failures. Follow the script to see how it works to exit the function:
#!/bin/bash
calculate_sum() {
local number1=$1
local number2=$2
# Check if both numbers are provided
if [ -z "$number1" ] || [ -z "$number2" ]; then
echo "Error: Please provide two numbers."
return 1
fi
# Calculate the sum
local sum=$((number1 + number2))
return $sum
}
# Call the function with two numbers
calculate_sum 5 3
# Check the return value of the function
return_value=$?
echo "Sum of the two numbers: $return_value"
This function calculate_sum
takes two parameters, number1 and number2. It checks if both numbers are provided using the -z operator in the if statement. If either of the numbers is not provided, it prints an error message, “Error: Please provide two numbers.”, returns a non-zero value (return 1), and ends the function.
When both numbers are provided, it calculates the sum and stores it in the sum variable. The function then returns the sum using the return $sum. calculate_sum 5 3
calls the function with the arguments 5 and 3. The return value is captured in the variable return_value using $?.
As you can see, the bash function returns 8 by adding the numbers 5 and 3.
The return
statement can also be used without a return value. Here’s an example bash script:
#!/bin/bash
my_function() {
echo "Welcome to Linuxsimply"
return
echo "Hello, world!"
}
my_function
After running the script, it prints “Welcome to Linuxsimply” and then ends the script when the return
command inside the function is encountered:
2. Using “exit” Command
The exit command is used to exit the script or shell session. However, users need extra caution while using this command as it not only terminates the function but also the entire shell script. Additionally, it has an exit status from 0 to 255 like the return
statement. Now, see an example to learn how to exit from the bash function using the exit
command:
#!/bin/bash
function file_existence() {
if [[ -f "$1" ]]; then
# File exists
echo "File '$1' found."
else
# File not found
echo "Error: File '$1' not found."
exit 1 # This exits the entire script
fi
}
if file_existence "hello.txt"; then
echo "File exists"
fi
The file_existence
function takes a filename as an argument and checks whether it exists using the -f
test. If the file exists, it prints a message; otherwise, it prints an error message and exits the script with an exit status of 1. The script then calls the file_existence
function with the argument “hello.txt”. If the defined file exists, it prints “File exists”.
Since the “hello.txt” is absent in the system, it shows an error and exits the entire shell script.
Note: To exit the function as well as the whole script, the exit
command can be used without an argument (0-255) like the return command.
3. Exit Function Based on User Input
Based on user input, any function can be ended. In this example, the script uses a conditional if
statement to decide when to exit the function. The function will end as soon as a user writes “exit” to the console. Here’s how:
#!/bin/bash
check_user_input() {
echo "Enter 'exit' to terminate the function:"
read user_input
if [[ "$user_input" == "exit" ]]; then
echo "Exit the function."
return
else
echo "Continue the function."
fi
}
# Call the function
check_user_input
Here, the Bash function named “check_user_input” is created. Inside the function, it prompts the user to enter an input. Then the read
command reads the user input. "$user_input" == "exit"
checks if the user input is “exit”, if it is, it prints the “Exit the function.” using the echo command and exits the function due to the return command. If the user writes other than “exit”, it continues the function and echoes “Continue the function.”.
In the first execution, I entered “exit” so the function ended. But when I entered “hello” in the 2nd execution of the script, the function continued.
4. Using “break” Command
In bash, the break
statement is used to break loops. Here, a for
loop is created inside the function where the beak command exits both from the loop and the function. Here’s how it works:
#!/bin/bash
function break_function() {
for i in {1..6}; do
echo $i
if [ $i -eq 4 ]; then
break # Exit the loop and function
fi
done
}
break_function
The script defines a function called break_function
that contains a for
loop. Inside the loop, it prints the value of i, and if i is equal to 4 ($i -eq 4)
, the break
statement is executed, causing the loop and the function to terminate.
The picture illustrates the termination of both the loop and function when the value of i becomes 4.
5. Using “trap” Command
Exiting from the bash function can be achieved using the trap command. In this example, the trap
command catches the SIGINT
signal which is usually generated by pressing CTRL+C, and exits the function. Here’s the bash script to accomplish this task:
#!/bin/bash
my_function() {
trap "cleanup_and_exit" SIGINT # Call cleanup_and_exit function on Ctrl+C
echo "Function started. Press Ctrl+C to exit gracefully."
sleep 10
# This line will not be reached if Ctrl+C is pressed during sleep
echo "Function completed."
}
cleanup_and_exit() {
echo "Ctrl+C detected. Cleaning up and exiting."
exit 1
}
# Call the function
my_function
# This line will not be reached if Ctrl+C is pressed during my_function
echo "Script continues after function call."
The my_function
function sets up a trap
using trap "cleanup_and_exit" SIGINT
. It indicates that when the SIGINT signal (CTRL+C) is received, the cleanup_and_exit
function should be called.
The cleanup_and_exit
function is responsible for cleaning up resources or performing any necessary actions before exiting the script with a status of 1.
Then the function simulates some work with sleep 10
. If Ctrl+C is pressed during this sleep, the trap
will be triggered, and the cleanup_and_exit
function will be executed. The subsequent line “Script continues after function call.” will not be reached if CTRL+C is pressed during the execution of my_function
.
You can see that after starting the function as soon as I entered CTRL+C, the function terminated.
Differentiating “exit” and “return” in Bash Function Exit
Though both exit
and return
commands have exit codes between 0 and 255, they have a couple of differences:
“exit” Command | “return” Command |
---|---|
The exit command is used to terminate the entire shell script. | The return command does not end the entire shell session, it terminates the function and returns control to the calling code. |
It can be called inside and outside of a function. | It is used only within functions. |
The exit can be used interactively. | The return statement can not be used interactively. |
Note: However, the exit
command is not recommended for use inside a function, as it can lead to unexpected behavior if the function is called from within another function.
Common Issues While Exiting from Bash Function
You may encounter some problems while exiting from the bash function. Let’s take a look at these problems and how to solve them:
A. Using “exit” Instead of “return”
It is not a good practice to use the exit
command instead of the return
command to exit out of a bash function. Because it will terminate the whole shell session, not just the function preventing any subsequent code from being executed. So if you want to only exit the function and continue with the rest of the script, use the return
statement instead of the exit
command.
B. Unexpected Script Termination
Unexpected script termination can occur if you misplace the exit
command inside the function in bash like this:
#!/bin/bash
function example_function() {
if [ "$some_condition" = "true" ]; then
exit 0 # Incorrect: Exits the entire script, not just the function
fi
}
# Call the function
example_function
# The script continues here after the function call
echo "Script continues."
In this script, if some_condition
is equal to “true”, no output will be generated after the function call because the script exits within the function. Therefore, be mindful of using the exit
command.
C. Writing “return” Command Outside Function
If you write a return
command outside the function, it may not produce the results as expected. So to avoid this issue, always write this command inside the bash function.
Practice Tasks on How to Exit from Bash Function
To enhance your Bash scripting abilities and learn how to exit a Bash function, try the following:
- Define a Bash function that checks if the number is odd, if so, exit the function.
- Write a function that simulates a simple calculator. It should take two numbers and an operator as input, perform the operation (addition, subtraction, multiplication, or division), and then exit with 0 on success or a non-zero code for errors (division by zero, invalid operator).
- Create a function that reads lines from a file until it encounters a specific keyword. Each line should be printed, and the function should exit with 0 when the keyword is found or 1 when the end of the file is reached without finding the keyword.
- Generate a function that acts like a timer. It should take a duration in seconds as input, wait for that amount of time, and then exit with 0. However, if the user presses CTRL+C during the wait, the function should exit immediately with a specific non-zero code.
Conclusion
To wrap up, I have listed 5 ways to exit from function in Bash. In addition, I have also discussed some common problems encountered while trying to exit from the bash function. Read this article carefully to improve your bash scripting skills. Good luck!
People Also Ask
What is exit command in Bash?
The exit
command in bash is used to end a script or a shell session. It assigns an exit status, ranging from 0 to 255, where 0 signifies success and values from 1 to 255 denote various error types. This is the syntax of the exit
command:
exit n
, where n represents the numeric value from 0 to 255.
What is return statement in Bash?
The return
statement is used within functions to end a function and returns values to the caller script. When a function meets the return statement, it immediately exits the function. This is the syntax of the return
statement:
return n
, where n represents the numeric value from 0 to 255. A value of 0 is considered a successful operation, while values ranging from 1 to 255 indicate failures.
Bash exit vs return?
The main difference between an exit
and a return
command is that an exit command terminates the execution of a bash script while a return command terminates the execution of a bash function. An ‘exit’ command can be executed inside or outside of a bash function, whereas a ‘return’ command should be executed inside a bash function.
How to exit bash function if a certain condition occurs?
To exit the bash function based on a certain condition, use the if
statement inside the function to check the condition. If the condition is true, use the exit
command with an appropriate exit code (0-255) to signal the reason for early termination. Here’s an example bash script for this:
#!/bin/bash
function check_file_size() {
local file_name="$1"
local max_size=1024 # In kilobytes
if [[ ! -f "$file_name" ]]; then
echo "Error: File '$file_name' does not exist."
exit 1
fi
local file_size=$(stat -c%s "$file_name")
if [[ $file_size -gt $(($max_size * 1024)) ]]; then
echo "Error: File '$file_name' is too large (exceeds $max_size KB)."
exit 2
fi
# Rest of the function logic...
}
This script checks if the file exists and is within the size limit. If either condition fails, it exits with an informative message and a specific exit code.
Related Articles
- How to Return Exit status “0” in Bash [6 Cases]
- How to Return String From Bash Function [4 Methods]
- How to Return Boolean From Bash Function [6 Methods]
- How to Return Array From Bash Function? [7 Methods]
<< Go Back to Return Values From Bash Function | Bash Functions | Bash Scripting Tutorial