FUNDAMENTALS A Complete Guide for Beginners
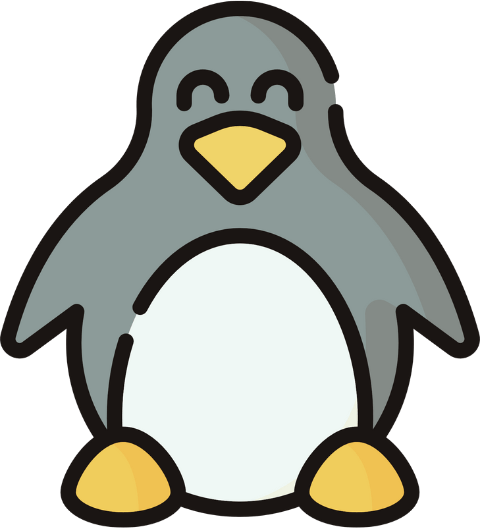
In the realm of information, each and every part of our computing system has data saved inside a file. For data handling, files and directories need to be created or removed. Moreover, if any new information arrives, the existing files need to be updated by appending. It is often necessary to copy and move files and directories to sort them topicwise. In this article, I will show you some bash file example on how to manipulate your file systems efficiently with some practical bash script examples.
10 File Manipulation Examples in Bash
As a user, you might need to do some file manipulation tasks. These tasks help you to accommodate any update in data, find the file size, and back up important files by coping or moving them. Or, you may just need to create and delete files and folders whenever necessary, overwrite one, read or check the existence of a file, etc. Here in this section, I have listed these 10 basic practical bash file manipulation examples:
1. Create & Remove Bash Directory and File
Bash provides various commands to create and remove directories and files. For example, you can use the mkdir command and the touch command to create a directory and a file. Moreover, the rm command to remove these files & directories. Now, to create a file or directory, just type the corresponding command followed by the file or directory name. Like these, mkdir folder_name
and touch file_name
. The same goes for removing the folder or file, rm -rf folder_name
or rm -f file_name
.
Here is a complete bash script to create a new file, and a folder & then remove them one by one:
#!/bin/bash
echo "Before creating folder_1 and file_1"
ls
#creating file and folder
mkdir folder_1
touch file_1
echo "After creating folder_1 and file_1"
ls
#deleting file and folder
rm -rf folder_1
rm -f file_1
echo "After removing folder_1 and file_1"
ls
In the script, the mkdir folder_1
& touch file_1
commands will create folder_1 directory and file_1 file respectively, inside the current working directory. Then, the rm -rf folder_1
& rm -f file_1
commands will delete the folder_1 and the file_1. The -f
option stands for the forceful removal of the file and the folder whether they are write-protected or are in use.
2. Append String to File in Bash
Appending a string to a file is just like adding a new string to an already existing string. To append a string to a file, the redirection operator >>
is used. The appropriate syntax for this purpose is: echo "string to append" >> file_where_to_add.txt
. In this syntax, >>
redirection indicates appending “string to append” to the file_where_to_add.txt file.
Here is a practical demonstration of overwriting a file:
#!/bin/bash
#printing contents
echo "Content of file3.txt"
cat file3.txt
#appending line
echo "Hello from command line" >> file3.txt
#printing contents
echo "Contents of file3.txt after appending"
cat file3.txt
The echo "Hello from command line" >> file3.txt
command will append the “Hello from command line” string to the “file3.txt” file.
3. Read Lines From File
The read command is used to read input from the user or from a file. Its primary purpose is to assign values entered by the user or read from a file to variables. So to read from a file, the file name has to be specified with the read command. For reading the first line from a file, use the syntax: read -r variable_name < file_name
. Here the ‘<’ redirection operator will redirect file_name to variable_name which is an argument of the read command. Then echo "$variable_name"
will print the first line.
Moreover, you can also read all the lines from the file one by one just using the read command inside a while loop. Check out the below bash script to understand the process practically:
#!/bin/bash
echo "Reading the first line "
read -r var < file1.txt
echo "The first line is stored in the variable is: $var"
echo "Reading and printing each line one by one"
count=1
while read -r var; do
echo "Line $count"
echo $var
count=$((count+1))
done < file1.txt
The read -r var < file1.txt
command will read the first line from the file1.txt file and keep it in the var variable. Then the echo "The first line is stored in the variable is: $var"
will print the value on the terminal. After that, the while read -r var; do
command starts a while loop, reads each line one by one, and assigns it to the var variable. The echo $var
command prints each line. Finally, the done < file1.txt
marks the end of the while loop.
4. Copy a File in Bash
Bash provides the cp command, which stands for ‘copy’. It copies a file from one folder to another folder. All you have to do is give the folder’s current location followed by the next destination path. For instance, a simple syntax explaining this would be, cp /folder/location/file_name /destination/location
.
To copy a file from one place to another, please go through the following bash script:
#!/bin/bash
echo "Copying file1.txt to a backup folder"
echo "Files before copying: "
ls /home/susmit/Backup
cp /home/susmit/file1.txt /home/susmit/Backup
echo "Files after copying: "
ls /home/susmit/Backup
The cp /home/susmit/file1.txt /home/susmit/Backup
command has copied the file1.txt file to the /home/susmit/Backup directory.
5. Move a File in Bash
Moving a file means replacing a file from one directory to another directory. The mv command moves files from one directory to another directory. To accomplish this, use the syntax: mv /old/directory/file_name /new/directory/file_name
.
Here is a real example of this scenario:
#!/bin/bash
echo "Moving file1.txt to a backup folder"
echo "Files in Backup before moving: "
ls /home/susmit/Backup
echo "Files in original folder before moving: "
ls /home/susmit
mv /home/susmit/file1.txt /home/susmit/Backup
echo "Files after moving: "
ls /home/susmit/Backup
echo "Files in original folder after moving: "
ls /home/susmit
The mv /home/susmit/file1.txt /home/susmit/Backup
command will move the file1.txt file from the /home/susmit/ to the /home/susmit/Backup directory.
6. Find the Size of the File in Bash
The ls command with the -l option shows the attributes of the files and folders of a certain directory. Then piping it to awk command with a specified filed number will extract the file size. Follow the syntax to find the file size: file_size=$(ls -l file_name | awk '{print $number}')
Here the attributes of the file_name will be passed to the awk command which will fetch the information of the number’th field.
Here is a complete bash script to find the size of a file:
#!/bin/bash
echo "Finding File Size of File1.txt"
file_size=$(ls -l file1.txt | awk '{print $5}')
echo "Size of the file is: $file_size bytes"
In the file_size=$(ls -l file1.txt | awk '{print $5}')
, the output of the ls command will be piped into the awk command, and the awk command will extract the fifth field which is the size of the file1.txt, and keep the result into the file_size variable.
7. Rename Directory and File by Bash Script
The mv command also changes file and directory names besides just moving a file from one directory to another directory. To change the file or directory name, use the below syntax: mv old_file/directory_name new_file/directory_name
.
Here is a bash script on how to change file and folder name:
#!/bin/bash
echo “Before renaming directory_1 and file_1”
ls
mv file_1 file_2
mv directory_1 directory_2
echo “After renaming directory_1 and file_1”
ls
The mv file_1 file_2
command will change the name of the file_1 file to file_2. Similarly, the mv directory_1 directory_2
command will change the name of the directory_1 to directory_2.
8. Count Characters, Words & Lines in the File
The wc command accompanied by a specific option counts characters, words, and lines in a file. For counting the word number, use the syntax: count=`cat $filename | wc -w'
. Here, the contents of the filename will be piped to the wc command which will count the total word number with the -w option.
Here is a practical example of counting characters, words, and lines in a file:
#! /bin/bash
echo “Enter the filename”
read file
c=`cat $file | wc -c`
w=`cat $file | wc -w`
l=`grep -c "." $file`
echo Number of characters in $file is $c
echo Number of words in $file is $w
echo Number of lines in $file is $l
The read command will read the file. Then the c=`cat $file | wc -c`
command will pass the output of the cat command to the wc command where the wc command with the -c
option will count the total characters and keep it in the c variable. Similarly, the -w
option will count word numbers. Finally, the l=`grep -c "." $file`
command will find the total number of the period (.) which counts the total sentence numbers.
9. Check If a File Exists in Bash
The test command with the -f
option looks for the specified file in the specified directory. For this use the syntax: test -f /path/to/find/file
inside the condition of the if statement. This will print the result about the existence of the file based on the exit status of the executed command.
Here is a practical demonstration of how to check the existence of a file:
#!/bin/bash
if test -f /home/susmit/file1.txt; then
echo "File exists."
else
echo “File does not exist”
fi
The if test -f /home/susmit/file1.txt; command with -f option will look for the file1.txt file inside the /home/susmit/ directory. Then it will print a message according to the result.
10. Bash Split String by Symbol
A symbol-separated string can be split into parts utilizing the Internal Field Separator (IFS). At first, set the delimiter using the syntax: IFS=','
Here the specified delimiter is the comma(,). Then read the string as an array utilizing the syntax: read -a array <<<"$entry"
. Here every comma separate word of the given input (entry) will be passed to the array as an array element part by part.
Here is a practical bash script example to split a string by symbol:
#!/bin/bash
#Example for bash split string by Symbol (comma)
#reading string value
read -p "Enter Name, State, and Age separated by a comma: " entry
#setting comma as delimiter
IFS=','
#reading string as an array as tokens separated by IFS
read -a array <<<"$entry"
echo "Name : ${array[0]} "
echo "State : ${array[1]} "
echo "Age : ${array[2]}"
The IFS=','
will set comma (,) as the delimiter. After that, the read -a array <<<"$entry"
command will keep every comma-separated word of the entered string entry as an array element. Finally, the echo "Name : ${array[0]} "
command will print the array element sequentially.
Conclusion
In conclusion, learning the skill of file manipulation is a fundamental skill for anyone working with data. In this article, I have explored some bash file examples of the creation, deletion, updating, renaming, coping, and moving of files and directories. Whether you are a beginner or an advanced programmer of bash scripting, this article will equip you with the tools to flourish your scripting skills.
People Also Ask
What is the file format for bash?
The file format for bash is the .sh file extension. It usually accomplishes a specific task whereas a text file just contains information.
How do I get the line number of a file in bash?
To get the line number of a file use the wc command in bash. This command can be used to find the number of lines, characters, words, and bytes of a file. Follow the below syntax to find the number of lines: wc -l file_name
What is the first line of a Bash file?
The first line of a Bash file is “shebang” (#!). It instructs the program loader which interpreter to run when reading the script.
How do I open a file in bash?
To open a file in Bash, use the cat command. It simply prints the content of the file on the terminal without enabling it for editing.
Where is bashrc stored?
The bashrc is located in the home directory. The bashrc file is read in and executed whenever a bash script or bash shell is started.