FUNDAMENTALS A Complete Guide for Beginners
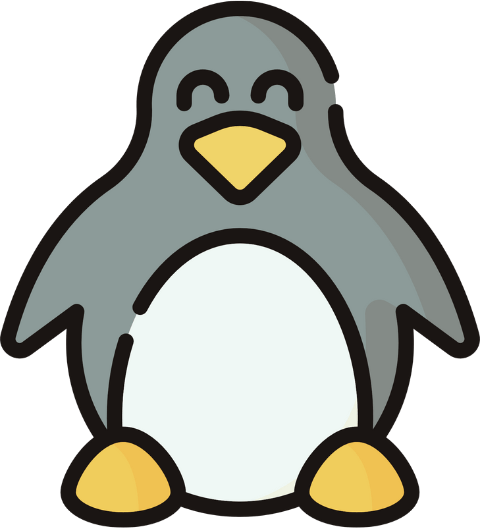
Comments are lines in a script that are ignored by the interpreter and are used for documentation or to temporarily disable code. You can comment in a Bash script by using the # symbol. Comments can be used to explain the purpose of certain code segments, provide instructions for future modifications, or temporarily exclude code from execution without deleting it.
In this article, you will get introductory ideas about the basics of Bash comments.
When to Use Bash Comments?
It’s common to write a Bash script, set it aside, and not revisit it for several months. When you eventually return to it, you will need to acquaint yourself with the whole code again. Comments clarify the process and help you to keep the scripting track here.
Again, suppose, you have written a script for other users. Comments, in this case, will simplify the less obvious parts of the program and make the whole script organized to them. Anyone by exploring the whole script written with comments can easily understand and interpret your thoughts.
How to Comment in Bash?
Use the # symbol at before the line you want to comment in a Bash script. Here’s how:
-
Create a file named file.sh to write a bash script with comment:
nano file.sh
-
Afterward, write programs in the script and adorn your script by using any kind of commenting style within the codes according to the requirement:
#!/bin/bash #This script is for printing information #Printing a user’s name echo “Nadiba Rahman” #Printing a website’s name echo “linuxsimply.com”
EXPLANATIONHere, the first line denotes a shebang. This is not a comment. But the next line “#This script is for printing information” is a comment that is written by the programmer to understand the purpose of the whole script later. Then, “#Printing a user’s name”, and “#Printing a website’s name” explains the step-by-step code.
-
Press CTRL+S to save the file and then CTRL+X to exit.
- Now, executing the script will print “Nadiba Rahman” and “linuxsimply.com” and ignore the rest of the commented lines.
Types of Comments in Bash
There are two types of comments in Bash script: Single-line comments & multiple-line comments. A little viewpoint regarding this:
- Single Line Comments in Bash: A single-line comment starts with a ‘#’ symbol.
- Multiple Line Comments in Bash: There is no built-in method to write multi-line comments in the Bash script.
To know more about the Bash comments, visit – Single-line comments, Multiple-line comments, and Block comments.
Comparative Analysis of Block Comments and Single Line Comment in Bash
A little comparative analysis chart between single-line & multiple-line comments in Bash is here:
Types | Pros | Cons |
---|---|---|
Single Line Comments |
|
|
Multiple Line Comments |
|
|
Which type of comment needs to prefer, depends on the requirement of the detailing level of users and the organizations. For a fast-forward explanation, you may choose a single-line commenting style. Otherwise, for detailed and comprehensive documentation, you may approach multiple-line commenting. As you have already got the pros and cons of both styles, now you can strike a good balance of comments.
In-depth Exploration of Bash Commenting
Master the Art of Commenting by deeply exploring several commenting aspects in Bash like the following section:
A. Explaining Script Purpose
Suppose, you have started coding right after opening a Bash script. Except for you, no one will understand the code at first glance. After a few days, even you won’t get the reason for what you wanted to do that code. So, for self and others’ betterment, it’s a must thing to comment out the purpose of what you are going to do at the beginning of any script shortly. Check the following script for the practical approach:
#!/bin/bash
#Script name: purpose.sh
#Purpose:
#Codes here
Here, in “#Purpose:”, you will write the purpose and target of your script.
From the above image, you can see the marked portion where you must write the purpose of your script to make it more engaging.
B. Commenting Functions
A function within a code contains some components like input parameters, arguments, return values, etc. Function comments play an indispensable role in this case. Comments disclose the purpose of every component & clear each level inside a function. See the below script as an example:
#!/bin/bash
#Function: new_user
#Purpose: This function greets new users
#Parameters:
# $1: Name of the first user
#Returns: No return values
new_user() {
name =$1
#Print the welcome message
echo “Hello, $name! Welcome.”
}
#Greet the user X
new_user X
Here, “#Function: new_user” tells what the function name is. And “#Purpose: This function greets new users” indicates the target of the script. Then, “#Parameters:” and “# $1: Name of the first user” dictates the parameters used in the code. After that, “#Returns: No return values” shows the return values if the function has any. Now, “#Print the welcome message” displays to print the message inside the echo command. Finally, “#Greet the user X” tells us to add user X as the new user.
In the above snapshot, you can see the function comments written in the highlighted areas.
C. Commenting Variables
Variables with enigmatic names inside the Bash script are quite confounding for the readers. Comments expound on the usage of those variables to make the script more appealing to the users. Go through the below script to check the process:
#!/bin/bash
#Purpose: This script calculates the area of a rectangle.
#Variables
#length: This stores the length of the rectangle in units.
#width: This stores the width of the rectangle in units.
length=3 #Rectangle’s length
width=2 #Rectangle’s width
#Calculate the area by multiplying the length & the width
area=$((length * width))
#Display the result
echo “The area is: $area square units.”
Here, “#Purpose: This script calculates the area of a rectangle.” indicates the goal of the script. In the “#Variables” section, “#length: This stores the length of the rectangle in units.”, and “#width: This stores the width of the rectangle in units.” represent the variables used in the script and what they mean here. Then, “#Rectangle’s length”, and “#Rectangle’s width” define inserted values to the variables. Afterward, ‘#Calculate the area by multiplying the length & the width” declares to calculate the desired task by using the variables. And finally, “#Display the result” shows the calculated result.
The above screenshot shows the usage of variable comments in a script.
D. Commenting Tags or Conventions
Tags or conventions are particular notations or labels that are used to provide extra information and instructions to improvise the commenting structure. Some commenting tags in Bash are:
#!/bin/bash
#Constants
Readonly max_retries=3 #Maximum number of retries for a failed case
Readonly log_file="script.log" #The log file path
#NOTE: This for loop may hamper performance
for i in {1..50}; do
#codes here
done
#TODO: Append error handling for edge cases
#FIXME: Find issues with file permissions
#Observe behavior:
#HACK: Though this method works, it's not the efficient one.
#suggestions:
Here, “#NOTE: This for loop may hamper performance”, “#TODO: Append error handling for edge cases”, “#FIXME: Find issues with file permissions”, and “#HACK: Though this method works, it’s not the efficient one.” are the convention comments which add some additional information to the script.
The above image displays the convention comments in a script.
E. Documentation Tools
Using some tools like BashDoc, Doxygen, Mardkwon, etc., you can generate documentation from the comments in your Bash script. But while using these tools it’s very important to follow some specific commenting patterns which are applicable to the tool you are using.
Tips for Checking & Updating Bash Comments
Outdated comments can mislead and create algorithmic errors. So, it’s very important to check regularly if the comments are updated or not. Follow the tips below to review & update Bash comments properly:
- Review the Bash comments regularly to make sure that they state the current condition of the code.
- Remove the inaccurate comments and update them by changing the variables, functions, algorithms, etc. as per requirement.
- Use the same phrasing format throughout the comments to keep consistency.
- Simply avoid the fusion of complex languages. Only use plain and manageable terms while commenting.
- Use version control to review the changes over time and track the state of Bash comments to spot potential errors.
Conclusion
By now, you have got that the Bash comments are nothing but an important interpretation of Bash codes. So, use more comments as logically as possible to keep track of your script.
People Also Ask
How Can I Comment in Bash?
To comment in Bash, use a hash (#) symbol before the line you want to comment. Everything following the #
symbol on the same line is considered a comment and is ignored by the Bash interpreter.
Are comments visible while listing up a directory’s contents?
No, comments are not visible during a directory’s contents listing execution.
Can I add metadata in Bash script using comments?
Yes, you can add metadata by commenting in the Bash script. This can include details such as the script’s purpose, author, creation date, and any other relevant information.
Is it mandatory to use comments in Bash?
No, it’s not mandatory to use comments in Bash. Actually, it’s just for the clarification of the script.
Can I remove comments once I insert them in Bash?
Yes, you can remove comments from Bash whenever you want. But you have to do it manually.
Can I use both single-line & multiple-line comments in a Bash script?
Of course! You can use both. Actually, it’s up to you how you will organize your script.
Do comments affect a running program?
No, comments are text used to explain the code logic. They don’t have any impact on the performance of your running program.
Related Articles
- The Art of Commenting in Bash
- Bash the Hash! – a Symbolic Feature of Bash Comment
- What Are Single-line Comments in Bash? [2 Cases With Examples]
- Multiple Line Comments in Bash [With Shortcut Keys]
- How to Use Block Comment in Bash? [2 Cases]
<< Go Back to Bash Scripting Tutorial