FUNDAMENTALS A Complete Guide for Beginners
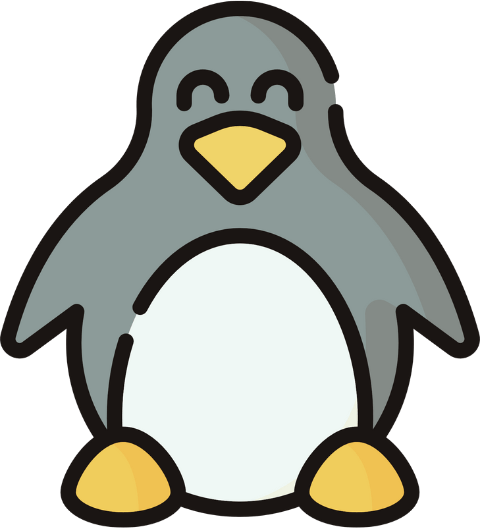
To check if a string is equal to another string, Bash uses comparison operators like double equals operator (==), equal (=) operator, or not (!=) operator, test command, and case statement.
To check if strings are equal or not, you can use the following 5 methods:
- Using equal “=” operator:
[ "$string1" = "$string2" ]
- Using double equal “==” operator:
[ "$string1" == "$string2" ]
- Using not equal operator:
[ "$string1" != "$string2" ]
- Using the “test” command:
test "$string1" = "$string2"
- Using the “case” statement:
case "$string1" in
$string2 )
Let’s dive into the 5 methods to check string equality in Bash:
1. Using Equal “=” Operator
Bash offers an equal =
operator to compare two strings to check their equality. To check if strings are equal, use the equal operator within the if-else statement. If the strings are equal, the condition evaluates true. Otherwise, the condition evaluates false.
Follow the below script to check if the two strings are equal:
#!/bin/bash
#Define two strings
string1="Linuxsimply"
string2="Linuxsimply"
#Print the declared strings
echo "Frist string: $string1"
echo "Second String: $string2"
# Check if string1 is equal to string2
if [ "$string1" = "$string2" ]; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
"$string1" = "$string2"
condition checks whether the value of string1 is equal to the value of string2. The equal operator =
is used for this equality comparison.The output shows that string1 and string2 are equal after comparing the strings.
2. Using Double Equal “==” Operator
You can check the equality of two strings using the double equal ==
operator within an if statement. Follow the script below to check if two strings are equal using the double equal operator:
#!/bin/bash
#Define two strings
string1="Linuxsimply"
string2="Bash"
#Print the declared strings
echo "Frist string: $string1"
echo "Second String: $string2"
#Check the strings
if [ "$string1" == "$string2" ]; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
Here, "$string1" == "$string2"
condition returns true if it finds the string1 and string2 equal. If the strings are different then the condition returns False.
3. Using Not Equal “!=” Operator
In Bash, the not-equal operator examines whether the entities being compared are different. In Bash, the not equal !=
operator along with the if-else statement can check if two strings are equal. When the strings are not equal, the condition is true; otherwise, it is false.
To check the string’s equality using not equal operator, follow the script below:
#!/bin/bash
#Define two strings
string1="Linux"
string2="linux"
#Print the declared strings
echo "Frist string: $string1"
echo "Second String: $string2"
#check the string's equality
if [ "$string1" != "$string2" ]; then
echo "Strings are not Equal."
else
echo "Strings are Equal."
fi
"$string1" != "$string2"
condition checks whether the value of string1 is not equal to the value of string2. If string1 becomes different from string2, the condition evaluates true and executes the echo "Strings are not Equal."
. Otherwise, it evaluates false and executes echo "Strings are Equal."
.4. Using the “test” Command
In Bash, you can compare two strings to check equality using the test command instead of using [ ] or [[ ]]. Follow the script below to check if the strings are equal:
#!/bin/bash
#Define two strings
string1="LinuxSimply"
string2="Linux"
#Print the declared strings
echo "Frist string: $string1"
echo "Second String: $string2"
#check two strings equality
if test "$string1" = "$string2"; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
if test "$string" = "$string2"
statement checks whether string1 and string2 are equal using the test command within the if statement. The test command evaluates the expression.To check if two strings are equal in one line, use the test command with the equal =
operator and conditional operator (&&, ||). Follow the script below to check string equality in one line:
test "LinuxSimply" = "LiNUXsimply" && echo "Strings are equal." || echo "Strings are not equal."
test “LinuxSimply” = “LiNUXsimply”
checks if the two strings are equal. If the preceding command succeeds and returns true, the command after the &&
operator (echo “strings are equal”) will execute. If the preceding command fails and returns false, the command after the ||
operator (echo “Strings are not equal”) will execute.The output shows the result after checking two strings’ equality using the “test” command in one line.
5. Using “case” Statement
In Bash, the case statement allows for conditional branching based on the value of a variable. It evaluates the variable against different patterns and executes the code block associated with the first matching pattern. You can use a case statement in Bash to check strings for equality. Follow the script below to check if the two strings are equal:
#!/bin/bash
#Define two strings
string1="Linux"
string2="LinuxSimply"
#Print the declared strings
echo "Frist string: $string1"
echo "Second String: $string2"
#Check the strings
case "$string1" in
$string2 )
echo "Strings are equal"
;;
*)
echo "Strings are not equal."
;;
esac
Note: So far, the 5 methods discussed in this article, all check strings’ equality in a case-sensitive manner.
In the following section, I’ll explain approaches for performing equality checks without considering the case of the characters involved.
Case Insensitive Equality Check
To check the case-insensitive equality of strings, you can convert the strings into lowercase (using ,,
) or uppercase (using ^^
) or use the nocasematch
option and after that compare them to check their equality. In this section 3 ways have been discussed to check string in a case-insensitive manner.
1. Lowercase Conversion: To convert the string’s case into lowercase and check their equality as below:
#!/bin/bash
#Define two strings
string1="LinuxSimply"
string2="linuxsiMpLy"
#Print the declared strings
echo "Frist string: $string1"
echo "Second String: $string2"
#Convert the strings into lowercase
string1=${string1,,}
string2=${string2,,}
#Print the declared strings
echo "The strings after converting into lowercase"
echo "Frist string: $string1"
echo "Second String: $string2"
#compare the strings
if [[ $string1 == $string2 ]]; then
echo "The strings are equal."
else
echo "The strings are not equal."
fi
${string1,,}
and ${string2,,}
are used to convert the strings to lowercase using the comma ,
operator inside the double square brackets [[ ]]
.2. Uppercase Conversion: To convert both strings in uppercase and compare them. Check the script below to check the equality of two strings:
#!/bin/bash
#Define two strings
string1="LinuxSimply"
string2="linuxsiMpLy"
#Print the declared strings
echo "Frist string: $string1"
echo "Second String: $string2"
#Convert the strings into uppercase
string1=${string1^^}
string2=${string2^^}
#Print the declared strings
echo "The strings converted into uppercase"
echo "Frist string: $string1"
echo "Second String: $string2"
#compare the strings
if [[ $string1 == $string2 ]]; then
echo "The strings are equal."
else
echo "The strings are not equal."
fi
${string1^^}
and ${string2^^}
expressions are used to convert the strings to uppercase. This is done by using parameter expansion with ^^
.3. Using “nocasematch” Option: Bash provides the nocasematch
option to check the strings’ equality in a case-insensitive manner. To check string equality in case insensitive manner, follow the script below:
#!/bin/bash
#Define two strings
string1="Linux"
string2="LinuxSimply"
#Print the declared strings
echo "Frist string: $string1"
echo "Second String: $string2"
shopt -s nocasematch
#Check the strings
case "$string1" in
$string2 )
echo "Strings are equal"
;;
*)
echo "Strings are not equal."
;;
esac
shopt -s nocasematch
is used to set the nocasematch
option, which makes the subsequent string comparisons in the script case-insensitive.Conclusion
To sum up, this article has illustrated 5 methods in detail, including the case-insensitive approach. Employing appropriate comparison techniques, such as using the == operator or the != operator with double brackets and employing the [[ … ]] construct, allows for effective string equality checks in Bash scripts. Hope this guide will help to sharpen your knowledge of the equality check of strings in Bash.
People Also Ask
What’s the difference between the ‘!=’ and the ‘-ne’ operator?
In Bash, the !=
operator is used for string comparison whereas the -ne
operator is used for only numerical comparison. When considering which operator to use, you have to consider the type of values you are comparing.
What’s the key difference between “[[ ]]” and “[ ]” syntax?
The key difference between [[ ]]
and [ ]
syntax in Bash is that ‘[[ ]]’ is a modern and flexible conditional construct. It supports advanced string comparisons, pattern matching, and logical operators, while ‘[ ]’ is a traditional test command with more limited functionality. [[ ]] is generally recommended for conditional expressions in modern Bash scripts.
How to check if a string starts or ends with a specific substring in Bash?
To check if a string starts or ends with a specific substring, use the [[ ]] construct with the ==
operator and the appropriate wildcard characters (* and ?). Here is an example bash script for checking the start and end of a string:
#!/bin/bash
string="Hello, World!"
#Checking if the string starts with "Hello"
if [[ "$string" == "Hello"* ]]; then
echo "The string starts with 'Hello'."
fi
#Checking if the string ends with "HWorld!"
if [[ "$string" == *"World!" ]]; then
echo "The string ends with 'World!'."
fi
##Output
The string starts with 'Hello'.
The string ends with 'World!'.
How can I check if two strings are equal in Bash?
To check if two strings are equal in Bash, use the equal (=) operator to compare the strings. For example, if two strings are string1 and string2, the syntax will be if [string1=string2]
to compare the strings. If the two strings are equal the condition returns true, on the contrary, it returns false. This is how you can check if two strings are equal in Bash.
How to compare two strings using their length?
To compare two strings using their length, use the parameter expansion expression ${#string}
to determine the length of the strings and compare the strings using the If statement. The syntax to compare two strings using their length is if [ ${#string1}=${#string2} ]
.
How to check if a string is empty or not in Bash?
To check if a string is empty or not, use the -z
option within the if-else statement. Follow the script below to check for an empty string:
#!/bin/bash
#initializing empty string
string=""
#Checking if the string is empty
if [[ -z "$string" ]]; then
echo "The string is empty."
else
echo "The string is not empty."
fi
##Output
The string is empty.
Related Articles
<< Go Back to Check String in Bash | Bash String | Bash Scripting Tutorial