FUNDAMENTALS A Complete Guide for Beginners
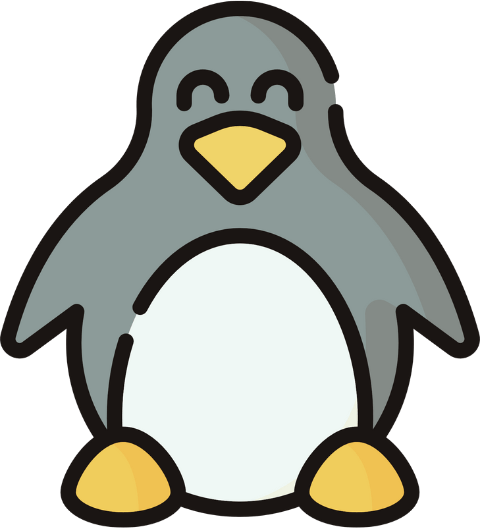
In Bash, one way to interact with users is by reading user input, which allows scripts and programs to accept data directly from the keyboard or other input sources. So, reading user input is a crucial aspect of creating interactive scripts. From this article, you will know how to read bash user inputs using the read command with some practical cases.
Syntax of “read” Command
In Bash, read
is a built-in command used to take input from the user or a specified input source. It then assigns the entered value to one or more variables for further script processing. Here’s a basic syntax of the read command:
read [OPTION]... Variable…
Options of “read” Command
Some useful options for the “read” command are provided below:
Option | Description |
---|---|
-s | Let the command perform in the ‘silent’ mode. Does not echo the user’s input. |
-n (number) | Returns after reading the specified number of characters. |
-N (number) | Returns after reading the specified number of characters while ignoring the delimiter. |
-d (delimiter) | Reads line until the given delimiter instead of a new line. |
-t (time) | Sets the time in seconds that the script should wait for the input from the user. |
-r | Disables backlashes to escape characters. |
-p (prompt) | Shows a message to the user before the input prompt. |
-a (array) | Assigns the given word sequence to a variable named array. |
-e | Begins an interactive shell session to get the line to read. |
-i (prefix) | Adds initial text before a line is read as a prefix. |
-u (file descriptor) | Reads from file descriptor instead of standard input. |
5 Cases of Reading User Inputs Using the “read” Command
Bash uses the read command to read user inputs. In this article, I will discuss some practical cases of how to create bash scripts to read user inputs.
1. Read Single User Input Using Bash Script
In this case, I will show you how you can simply use the read
command to read a single user input. Follow the script to accomplish this task:
#! /bin/bash
echo "What’s your name?"
read name
echo "Hello, $name! Nice to meet you."
Here, the echo
command echoes the quoted message. After that, the read
command reads & stores the input from the user and assigns it to the variable name. In the last line, the echo command echoes the value stored in the name variable along with other parts of the message.
In the above image, you can see that, I successfully ran the created intro.sh script. The script shows a user interactive message What’s your name?. Later it has been read & displayed after the user has inserted the value ‘Joy’.
2. Read Multiple User Inputs Using Bash Script
The read command in Bash can read multiple variables at a time. For that, just provide a list of variable names separated by spaces, and the command will assign the corresponding inputs to each variable. Let’s see a bash script to read multiple user inputs using the read
command:
#! /bin/bash
echo "Enter your name, age, and gender: "
read name age gender
echo "Name: $name"
echo "Age: $age"
echo "Gender: $gender"
First, the echo
command prints a prompt message to the user, specifying the inputs expected. After that, The read
command reads the user’s input, which is then executed to contain three values separated by spaces. It assigns these values to the variables name, age, & gender respectively. The next three lines display the value stored in these variables.
In the above image, you can see that the script is showing a user interactive message for multiple inputs. Later these values have been read & displayed after the user has inserted the values joy 20 male (separated by space).
3. Read Input from a File in Bash
You can use the read command within a loop to process each line of a file. In this case, the script will simply echo each line with a processing message, but you can perform any desired operations. Here’s a bash script to see how the read
command works to read input from a file:
#! /bin/bash
filename="input.txt"
while IFS=read -r line
do
echo "Processing: $line"
# Performs actions on each line
done < "$filename"
First, assign the file name you want to read from to the variable filename. Here, the file name is set to input.txt. Make sure the file exists in the same directory as the script or provide the full path to the file. Then, the After that, while IFS=read -r line
line initiates a while loop
that iterates over each line of the file. Where in IFS=read -r line, the read
command reads each line from the file and assigns it to the variable line. By setting ‘IFS= ’ and using the -r
option, it is ensured that leading & trailing whitespaces are preserved, and backlashes are not interpreted as escape characters. And do
sets actions for the command.echo “Processing: $line”
, this line prints the current line being processed. Lastly, done < “$filename”
redirects the contents of the file specified by the $filename variable as input for the while loop. Each line of the file will be read and processed until the end of the file is reached.
In the above image, you can see that the script is reading & processing each line of the file input.txt. After reading, it is also displaying them one by one.
4. Read User Password Securely Using Bash
Using the read command with option --silent
or -s
you can assign any sensitive user information as the command option hides them without being displayed on the screen. Here’s a bash script to read the user password securely using the read
command:
#! /bin/bash
echo -n Enter your password:
read -s password
echo
# Perform actions in silent mode
echo "Password entered: $password"
The echo -n “Enter your password: ”
echoes the quoted message with, -n
being used to prevent a newline character from being added. Then in read -s password
, the option -s
lets operate the read
command in silent mode. Thus, the user can enter a password without displaying the characters on the screen. After that echo
prints a newline just to maintain the readability. Finally, echo “Password entered: $password”
prints out the password that the user entered.
In the above image, you can see that the script is showing a user interactive message Enter your password. Later, the user enters the password but the command reads it in silent mode without displaying it. Which we can see after printing the value.
5. Create an Interactive Prompt for Multiple Input Types
You can also write a bash program to generate prompts for multiple inputs. In this case, the prompt will ask the user three different questions and print them at the end. Check the bash script below to create an interactive prompt for multiple input types:
#!/bin/bash
echo "Welcome. What should we call you: "
read var_name
echo "It’s nice to meet you" $var_name
read -p "Enter your age: " number
echo "So, you are a $number year old!"
echo "Do you like bash programming?(y/n)"
read
echo "That’s good to know, thank you."
The first line echoes a message. In the next line, the script takes the input as a variable and prints it. Then, in the next line, the read
command prints the prompt and takes a numeric value from the user using the -p
(prompt) option, and prints this number in the next line. At last, comes the yes/no question to the user, which is read by default, and the program ends with a thank-you message.
First, it welcomed the user and asked for the user name, in the next line the user inserted the value jane. After that, the program prints “It’s nice to meet you jane”. Where jane is the variable value. Then asks for the user’s age by Entering your age and the user inserted 12. The next line prints the user’s age with the line “So, you are a 12 year old!”. Finally, asks a yes/ no question, and the user inserts Yes by entering the letter “y”. After reading the message “That’s good to know, thank you.” is displayed.
Conclusion
To sum up, learning to create interactive scripts is a mandatory Bash scripting task. And for that, you have to learn to read user inputs. Hope this article helps in attaining the necessary knowledge.
People Also Ask
Related Articles
- How to Use Input Argument in Bash [With 4 Practical Examples]
- How to Use Bash Input Parameter? [5 Practical Cases]
- How to Wait for User Input in Bash [With 4 Practical Examples]
- How to Read CSV Files in Bash? [2 Methods]
<< Go Back to Bash Input | Bash I/O | Bash Scripting Tutorial
This was a scripting method that I vaguely understood. yet i use it in almost every script I write.
I was very happy to stumble across this read in my late night linux research , And after reading it,..
I am humbled, thankful, and inspired.
Thank you for this knowledge,
I am already trying a few of these out, more in depth than I was able to before.
You are most welcome! It felt awesome hearing your positive review! We always try to break down each of the scripts with explanations for users for their better understanding. You can also go through our other readings if you need any help on any particular topic. Happy scripting!
This is my most used version of the read command ..
reply=”memory”
while IFS= read line
do
shuf “memory” > “tmp-mem” &&
shuf “tmp-mem” > “memory” &&
shuf -n 1 –random-source=”memory”
done < "$reply" | espeak-ng -p13 -s88 -a 200 -v en-US+whisper
Yes, the read command is an efficient and simple tool for creating interactive scripts. Your code looks fine, just that you can use the ‘-r’ option with the read command, so that backlashes are treated as literal characters and don’t get interpreted as escape characters.
reply=”memory”
while IFS= read -r line
do
shuf “memory” > “tmp-mem” &&
shuf “tmp-mem” > “memory” &&
shuf -n 1 –random-source=”memory”
done < "$reply" | espeak-ng -p13 -s88 -a 200 -v en-US+whisper