FUNDAMENTALS A Complete Guide for Beginners
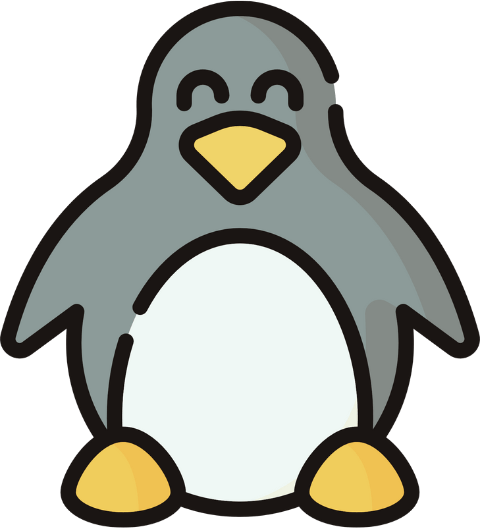
Bash, the popular command line interpreter and scripting language, offers powerful capabilities to customize and control scripts using input parameters. In this article, I’ll explore practical examples to demonstrate how to handle bash input parameter effectively. Whether you’re new to Bash or an experienced user, mastering input parameters can enhance your scripting skills and streamline your workflow. Let’s delve into the world of Bash input parameters and unlock their full potential.
Key Takeaways
- Getting familiar with the bash input parameter.
- Accessing bash input.
- Passing multiple inputs into a bash script.
Special Characters and Bash Input Parameter
Bash programming follows some special characters. Some are listed below.
- $ → This symbol is used when we assign any value or get any value.
- $@ → Represents all the command line arguments provided.
- $# → This counts the total number of passed parameters.
Free Downloads
5 Practical Cases of Input Parameter in Bash Scripting
The bash input parameter is one of the most integral parts of bash scripting. Here I have listed some scripts that include bash input parameters.
Case 01: Display the Passed Parameter
You can easily display the parameter you passed to the terminal. Here I will pass two parameters while running a script. This script will print the passed parameters to the terminal. to do the same follow the steps below:
➊ At first, open the Ubuntu Terminal.
➋ Then, execute the following command into the terminal.
nano parameter.sh
➌ This will open a file named parameter.sh. Now, type the following line into the file, press CTRL+S to save, then CTRL+X to close the file.
#!/bin/bash
echo "First Parameter: $1" #Printing the first parameter
echo "Second Parameter: $2" #Printing the second parameter
➍ Now, run the following command into the terminal to run the parameter.sh bash script passing 10 and 95 as parameters.
bash parameter.sh 10 95
The above image shows that two parameters 10 and 95 are successfully passed to the bash script.
Case 02: Assign Provided Arguments to Bash Variable
While executing the Bash script, it creates the necessary bash variables. Here I will pass two parameters to the bash script. And the bash script will eventually create a bash variable and print it to the terminal. You can follow the scripts to do the same.
Script (bashvar.sh) >
#!/bin/bash
a=$1
b=$2
p=$(($a*$b))
echo "Product of $a and $b is: $p"
Now, execute the following command into the terminal to pass the two-parameter 2 and 5 to the bashvar.sh bash script.
bash bashvar.sh 2 5
The above image shows that your two passed parameters have created a bash variable which has been printed on the terminal.
Case 03: Check Whether the Passed Parameter is NULL
You can check whether your passed parameter is NULL or not. Here I will develop a bash script and then pass a parameter to this script to check whether the parameter is NULL or not. To achieve so you can follow the given script.
Script (nullchk.sh) >
#!/bin/bash
if [[ -z $1 ]];
then
echo 'No Parameter Passed!'
else
echo "The Parameter is: $1"
fi
Now, execute the following command into the terminal to run the bash script and pass a parameter.
bash nullchk.sh
bash nullchk.sh 5
The former image illustrates that the bash scripts can check whether a parameter is NULL or not.
Case 04: Reading Multiple Arguments With “for” Loop
Here I will develop a bash script that can take multiple parameters from the terminal and then print them on the terminal. To do the same, follow the below script.
Script (multiarg.sh) >
#!/bin/bash
for i in $@
do
echo -e "$i\n"
done
Now, execute the following command into the terminal to multiple parameters to bash the script.
bash multiplearg.sh 1 2 33 45 1 “hi”
The above image illustrates that the bash script takes multiple parameters and prints them on the terminal.
Case 05: Accessing the Number of Parameters Passed
Here I will develop a bash script that can count the number of parameters passed. To achieve so, you can follow the below script
Script (parameternum.sh) >
#!/bin/bash
echo "The Number of Parameter Passed In are: $#"
Now, execute the following command into the terminal to multiple parameters to bash the script.
bash parameternum.sh 1 5 3 5
The above image illustrates that the bash script counts the total number of parameters passed.
Conclusion
In conclusion, mastering Bash input parameters is essential for enhancing your scripting capabilities. By utilizing them effectively, you can create dynamic and versatile scripts that handle various scenarios. Through practical examples, I’ve demonstrated the power and flexibility of input parameters in Bash. Incorporating these parameters into your scripts will streamline your process, improve efficiency, and empower you as a Bash programmer. Unlock the full potential of Bash by leveraging input parameters. Happy scripting!
People Also Ask
Related Articles
- How to Use Input Argument in Bash [With 4 Practical Examples]
- How to Read User Input in Bash [5 Practical Cases]
- How to Wait for User Input in Bash [With 4 Practical Examples]
- How to Read CSV Files in Bash? [2 Methods]
<< Go Back to Bash Input | Bash I/O | Bash Scripting Tutorial