FUNDAMENTALS A Complete Guide for Beginners
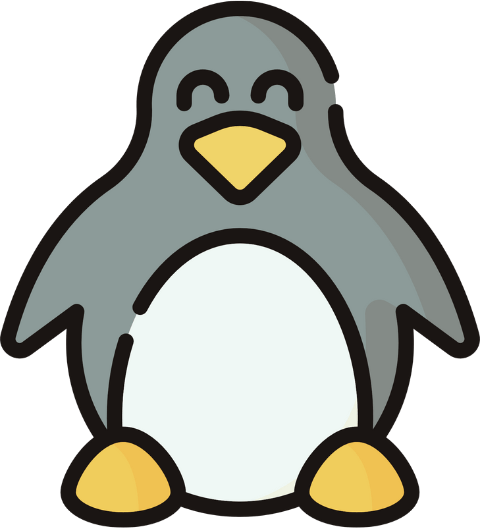
Iteration of Bash arrays refers to accessing each element one by one within the arrays. It usually involves using loops to go through the elements to perform operations on each element individually.
This tutorial provides an extensive guide on 3 loop-based methods (for, while, until loops) of how to iterate a Bash array. It will also walk you through the necessary commands and syntax to iterate through the elements of the bash array.
1. Using “for” Loop
The Bash for loop is a control flow statement that allows the iteration over the items of an array repeatedly. The basic conditional syntax for the Bash for loop is:
for variable in "${your_array[@]}"
do
# Commands to execute for each array element
done
Here’s an example of how to iterate an array in Bash using the for loop:
#!/bin/bash
#Declaring an indexed array and assigning it with values
users=(ubuntu redHat kali)
#looping through the elements to print
for item in ${users[@]}
do
echo $item
done
After declaring the array users, the loop for item in ${users[@]}
iterates the array to access each element individually within the item variable and prints them on the screen with the assistance of echo $item
.
Using C-Style “for” Loop
The C-style for loop in Bash has a construction similar to the loop used in C programming. With the combination of 3 parts, initialization, condition, and increment/decrement, the C-style for loop with the format for ((initialization, condition, and increment/decrement)) provides a more controlled iteration of elements in a Bash indexed array. For instance, the loop for ((i=1; i<=4; i++))
iterates starting from index 1 till index 4 with each increment.
To iterate an array in Bash with the C-style for loop, see the example below:
#!/bin/bash
# Indexed Array
fruits=("apple" "banana" "orange")
#array length
len=${#fruits[@]}
# Using C-style for loop to iterate through indexed array
echo "Iterating through indexed array using C-style for loop:"
for ((i = 0; i < len; i++)); do
echo "${fruits[i]}"
done
In this Bash script, the len variable stores the array length (3) and the loop for ((i = 0; i < len; i++))
starts iterating over the elements from index 0 to the end (index 2) and prints the elements on the prompt using echo "${fruits[i]}"
where fruits[i] store the array values one at a time to print.
Iterate an Associative Array Using “for” loop
The Bash for loop is also capable of iterating over an associative array. The only thing that differs is the for loop iterates over the keys instead of direct values to smartly access the elements of that array. Here’s how:
#!/bin/bash
# declare an Associative Array and initialize with elements
declare -A assoc_array
assoc_array=([key1]="value1" [key2]="value2" [key3]="value3")
# Using for loop to iterate through associative array (keys)
echo "Iterating through an associative array using for loop (keys):"
for key in "${!assoc_array[@]}"; do
echo "Key: $key, Value: ${assoc_array[$key]}"
done
Here, the for-loop for key in "${!assoc_array[@]}"
iterates through the keys of the assoc_array and the command echo "Key: $key, Value: ${assoc_array[$key]}"
prints both the keys and the corresponding values to the screen. All the key-value pairs are printed with new lines.
NOTE: To iterate through and print only the keys, use: for key in "${!assoc_array[@]}"
and print keys using the echo command: echo $key
.
2. Using “while” Loop
A Bash while loop repeatedly executes the pre-defined task until the specified condition is true. Let’s see an example of how a while loop works to iterate an array in Bash:
#!/bin/bash
# Indexed Array
users=("apple" "banana" "orange")
# Get the length of the array
array_length=${#users[@]}
# Initialize counter
counter=0
# Using while loop to iterate through indexed array
echo "Iterating through users array using while loop:"
while [ $counter -lt $array_length ]; do
echo "${users[counter]}"
((counter++))
done
Here, the counter variable keeps track of the array index whereas the while [ $counter -lt $array_length ]
loop continues iterating the array until it has reached the entire length (3). While iterating over, the echo "${users[counter]}"
prints each element and immediately ((counter++))
increments the value of the counter by 1 each time to access the next element and so on.
Iterate Bash Associative Array Using “while” Loop
To iterate a Bash associative array using a while loop and print the key-value pairs, see the following example:
#!/bin/bash
# declare an associative array
declare -A thespian
thespian=([tom]="cruise" [john]="cena" [christian]="bale")
# Using while loop to iterate through associative array
echo "Iterating through associative array using while loop:"
while IFS= read -r key && IFS= read -r value <&3; do
echo "Key: $key, Value: $value"
done < <(printf '%s\n' "${!thespian[@]}" | sort) 3< <(printf '%s\n' "${thespian[@]}" | sort)
Here, the while loop reads key-value pairs by leveraging file descriptors (<&3) and manages key and value retrieval via IFS settings. It begins by sorting and printing the keys using (${!thespian[@]})
and values with (${thespian[@]})
of the associative array using printf and process substitution (< <()). Then, the while loop reads these sorted lists, simultaneously capturing keys as key and values as value within each iteration.
3. Using “until” Loop
The until loop in Bash executes the given task until the mentioned condition remains false. It’s the opposite of the while loop. It provides straightforward syntax until [ condition ] followed by the command to run to iterate through Bash arrays.
See the below example to understand how the until loop iterates over an array:
#!/bin/bash
# Indexed Array
declare -a fruits=("apple" "banana" "orange")
# Get the length of the array
array_length=${#fruits[@]}
# Initialize counter
counter=0
# Using until loop to iterate through indexed array
echo "Iterating through indexed array using until loop:"
until [ $counter -ge $array_length ]; do
echo "${fruits[counter]}"
((counter++))
done
In the script above, the loop until [ $counter -ge $array_length ]
iterates the array until the counter value equals the array length to print the elements.
Iterating Bash Associative Arrays Using “until” Loop
To iterate an associative array, use the until loop in the format: until [ $counter -ge $num_keys ]
followed by the command to execute. The -ge
operator denoting “greater than or equals” compares the current iteration value counter with num_keys (holds the number of keys to determine the terminating condition).
Here’s an example:
#!/bin/bash
# Associative Array
declare -A my_assoc_array
my_assoc_array=([key1]="value1" [key2]="value2" [key3]="value3")
# Get the keys of the associative array
keys=("${!my_assoc_array[@]}")
# Get the number of keys
num_keys=${#keys[@]}
# Initialize counter
counter=0
# Using until loop to iterate through keys of associative array
until [ $counter -ge $num_keys ]; do
key="${keys[counter]}"
echo "Key: $key, Value: ${my_assoc_array[$key]}"
((counter++))
done
In the script above, the declare -A my_assoc_array
command declares an associative array my_assoc_array and initializes key-value pairs. Then the loop until [ $counter -ge $array_length ]
iterates the array until the counter value equals the array length to print the elements.
NOTE: Though it’s possible to iterate over the Bash associative array using the “until” loop, it’s not recommended due to its intricacy and challenge to maintain code.
How to Iterate Through a Range of Array Elements?
Apart from iterating an entire array, the Bash loops can iterate through any specified range of that array. I have shown an instance below on how to use a c-style for loop, for ((initialization, condition, and increment/decrement)), to iterate a Bash array over a given range:
#!/bin/bash
# Indexed Array with 5 elements
digits=("one" "two" "three" "four" "five")
#print array
echo "all elements: ${digits[@]}"
echo
# Iterating through the 3rd and 4th elements using a for loop
echo "Iterating through the 3rd and 4th elements of the array:"
for ((i = 2; i < 4; i++)); do
echo "${digits[i]}"
done
The script starts by printing all elements of the array using echo "all elements: ${digits[@]}"
. It then focuses on iterating specifically through the 3rd and 4th elements (three & four) of the array using a for loop with a C-style.
Using “Slicing” Method
The array-slicing technique ${array[@]:start_index:count}
accesses elements from the start_index up to the number of elements defined by count. For example: ${array[@]:2:3}
will access 3 elements beginning from index 2 inclusive.
However, you can employ the slicing formula within a Bash for loop condition to iterate over a specific range. Here’s how:
#!/bin/bash
# Indexed Array
my_array=("one" "two" "three" "four" "five" "six" "seven")
#print array
echo "elements: ${my_array[@]}"
echo
# Define the range to iterate (start_index:count)
start_index=2 # Start index
count=3 # Number of elements to iterate
# Iterate from index 2 upto 3 elements inclusive with slicing and for loop
echo "Iterating from index 2 upto 3 elements inclusive in the array:"
for elem in "${my_array[@]:start_index:count}"; do
echo "$elem"
done
The for loop in the format for elem in "${my_array[@]:start_index:count}"
above starts to iterate my_array from index 2 and continues until it reaches the 4th element (index 3).
Conclusion
To sum up, this article has gone through in-depths on 3 loop-based methods to iterate over an array in Bash mentioning both the indexed and associative arrays along with practical examples. Hope this guides you to be adept in iterating arrays in Bash for effective data handling and manipulation. Still, if you face any difficulty, feel free to share in the comment section. Happy scripting!
People Also Ask
How do I iterate an array in Bash?
To iterate an array in Bash, use for loop with the ${your_array[@]} syntax:
for elem in "${your_array[@]}"; do
echo "$elem"
done
This will iterate over your_array to print the array elements.
Is it possible to iterate through an associative array in Bash?
Yes, just like indexed arrays, it’s possible to iterate through a Bash associative array. Associative arrays use keys (string indices) in lieu of numerical indices. The for-loop syntax looks a bit different though. See the example below to iterate the associative array: country_capital=([“Bangladesh”]=”Dhaka” [“France”]=”Paris” [“United Kingdom”]=”London”):
# Loop over the array
for key in "${!country_capital[@]}"
do
echo "Key: $key, Value: ${country_capital[$key]}"
done
Output:
Key: United Kingdom, Value: London
Key: France, Value: Paris
Key: Bangladesh, Value: Dhaka
How to iterate over a specific range of elements in a Bash array?
You can use the for loop with the array slicing expression in the format for elem in “${my_array[@]:start_index:count}” to iterate over a range of elements in a Bash array. Here, start_index is the starting position and count is the number of elements to iterate. For instance, to iterate from the 2nd element to 4th element of the array num=(1 2 3 4 5 6 7):
for elem in "${num[@]:1:3}"; do
echo "$elem"
done
Output:
2
3
4
Can I iterate through the keys of a Bash associative array?
Use a for loop with ${!associative_array[@]} to iterate through the keys. For example, to iterate over the keys of an associative array named declare -A food=([“eat”]=”rice” [“take”]=”tea” [“drink”]=”juice”), use the loop:
for key in "${!food[@]}"
do
echo "Key: $key"
done
Output:
Key: drink
Key: take
Key: eat
Can I use the “printf” command in the for loop to print an indexed array?
Yes, you can use the printf command within the for loop to print an indexed array like the following:
myArray=("element1" "element2" "element3")
for element in "${myArray[@]}"; do
printf "%s " "$element"
done
Output:
element1 element2 element3
What’s the difference between an indexed array and an associative array in Bash?
Index array uses numerical indices (0 1 2) to store and retrieve elements. Contrarily, the Bash associative arrays allow the customization of indices by employing user-defined keys with unique values providing a more non-sequential approach to data handling.
How to print the length of an indexed array in Bash?
The length of an indexed array means the number of elements stored in the array. To print the length of an indexed array, use the command echo ${#array[@]}
Related Articles
<< Go Back to Elements of Bash Array | Bash Array | Bash Scripting Tutorial