FUNDAMENTALS A Complete Guide for Beginners
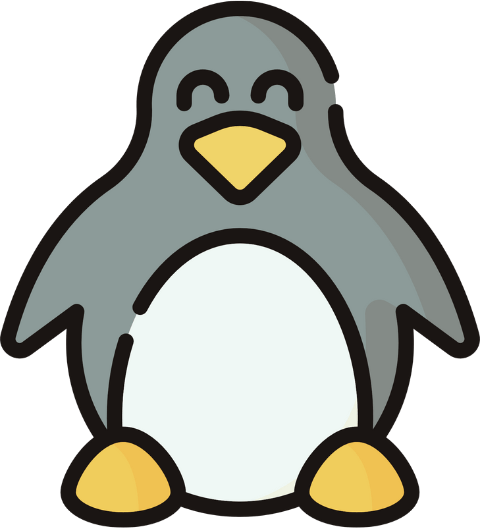
In the dynamic landscape of Bash scripting, managing script termination efficiently is crucial for ensuring script reliability and system integrity. This is where the bash trap EXIT command comes into play. It allows users to ensure the script termination occurs with finesse. With the trap EXIT command, the bash scripts gain the ability to execute pre-defined actions before gracefully terminating. This article will dive into various aspects of the bash trap EXIT command.
What is “trap EXIT” Command in Bash Scripting?
In Bash scripting, the trap EXIT is a command used to set up a signal handler for the EXIT signal. This means that when the script is about to exit the specified commands or functions are executed. This happens when the script reaches the end or encounters an error. This is used to perform cleanup tasks, close files, or process or release resources before the script terminates. It ensures that the script will take certain actions before it exits regardless of the exit condition.
The basic syntax of the trap EXIT command is as follows:
trap 'commands_to_execute_before_exit' EXIT
function some_function(){
#some commands
}
trap some_function EXIT
The trap command can trigger any command or function when it receives the EXIT signal
. Here, in the first syntax, the trap command executes the commands_to_execute_before_exit
command just before the script exits. In the second syntax, it triggers the some_function
function which is a user-defined function with some combinations of commands before the script terminates.
2 Practical Examples of Bash “trap EXIT”
The trap EXIT command in bash scripting sets up a signal handler to execute specified commands or functions just before a script exits. It is commonly used for cleanup tasks, error handling, logging, etc. This article will go through 2 useful examples of such cases.
1. Remove Temporary Files
Removing temporary files in Bash scripting is necessary to avoid cluttering the filesystem and consuming unnecessary disk space. If temporary files are not removed, they accumulate over time and potentially cause issues such as running out of disk space or confusion when navigating directories.
Using the bash trap EXIT command, temporary files can be removed automatically when the script exits, whether it finishes successfully or encounters an error. By setting up a trap for the EXIT signal and defining a function to delete the temporary files within it, cleanup occurs reliably before script termination. This approach ensures that temporary files are consistently removed, regardless of script execution outcomes.
The following example showcases such a bash script to remove temporary files before the script terminates:
#!/bin/bash
# Function to clean up temporary files
cleanup() {
echo "Cleaning up before exit..."
rm -f user_info.txt
IFS=$' \t\n' # Reset IFS to default value
}
# Trap EXIT signal to call the cleanup function
trap cleanup EXIT
# Main script logic
echo "Welcome to the script!"
read -p "Please enter your name: " username
read -p "Please enter your favorite Linux distribution: " distro
# Save user info to a temporary file
echo "Name: $username" > user_info.txt
echo "Favorite Linux Distro: $distro" >> user_info.txt
# Read user info from the temporary file and display
echo "Reading user info from file..."
while IFS= read -r line; do
echo "$line"
done < user_info.txt
echo "Script execution completed."
This bash script collects user information such as their name and favorite Linux distribution. It then saves this information to a temporary file named user_info.txt
. The cleanup function removes this temporary file upon the script exit. Additionally, it resets the IFS variable to its default value to prevent any unintended behavior in subsequent commands that rely on it. Finally, the script reads the user information from the file and displays it back to the user.
Here, the script cleans all temporary files before it terminates.
2. Error Handling and Logging
Error handling and logging are necessary in bash scripting to identify and troubleshoot issues, ensure script reliability, and provide insight into script execution. Without proper error handling and logging, it is challenging to diagnose problems. Which leads to unexpected behavior and potential data loss.
Using the bash trap EXIT command, error handling and logging can be implemented by defining functions to handle errors and log messages and setting up traps for the EXIT signal to invoke these functions. When an error occurs during script execution, the error handling function is triggered. Then it logs the error message and terminates the script gracefully. Here is how to do so:
#!/bin/bash
# Define log file path
script_dir=$(dirname "$0") # Get the script's directory
log_file="$script_dir/error.log"
# Function to write log message with timestamp
log_message() {
local message="$1"
echo "$(date +'%Y-%m-%d %H:%M:%S') - $message" >> "$log_file"
}
# Trap EXIT to write a final message to the log
trap 'log_message "Script execution finished."' EXIT
# Function to handle errors and log them
handle_error() {
local exit_code="$1"
local error_message="$2"
log_message "ERROR: Script exited with code $exit_code. Message: $error_message"
exit "$exit_code"
}
# Script logic here (example: check if a file exists)
if [[ ! -f important_file.txt ]]; then
handle_error 1 "Critical file 'important_file.txt' not found."
fi
# Perform other script operations here
echo "Processing data..."
sleep 20
# Script execution completed successfully
log_message "Script completed successfully."
exit 0
This Bash script manages error handling and logging for a series of operations. It begins by defining a log file path which is in the bash script’s directory and a function log_message()
to append timestamped messages to the log. The trap command is set to write a final message to the log upon script exit. In case of errors, the handle_error()
function logs the error message and exits with the appropriate exit code. Then the main script checks for the existence of a critical file and processes some data. Upon successful execution of the bash script, a success message is logged into the log file before the script exits.
Here, as you can see, the script logs all of its actions in a log file instead of echoing them in the terminal.
From this image, see the log file that shows the occurrence of the script execution and the error log.
Tips on Using the “trap EXIT” Command
The “trap EXIT” command is not free of any limitation. Here are some potential pitfalls and best practices of using the “trap EXIT” command:
Pitfalls:
- Limited Execution: Commands within the trap are executed only once just before the script terminates. This means it can not be used for ongoing tasks or monitoring.
- Non-Standard Exits: Signals like SIGINT (Ctrl+C) can cause script termination without triggering the EXIT trap.
- Nested Traps: Traps can be designed in nested form. If not careful it can cause potential unintended consequences.
- Resource Consumption: Commands within the trap can cause resource leaks, especially when it involves long-running operations.
- Log Verbosity: Excessive logging within the trap can bloat the log file with useless information.
Best Practices:
- Clarity and Conciseness: Keep the trap command focused on essential tasks like cleanup or logging the final message.
- Proper Cleanup: Focus on critical cleanup actions like removing temporary files or closing child processes.
- Logging Strategy: Develop a strategy that balances details and overwhelming log files.
- Error Handling Integration: Integrate error handling mechanisms with the trap command for a cohesive approach.
- Testing Thoroughly: Do rigorous testing of the trap handler under various scenarios to uncover potential issues and validate expected behavior.
- Proper documentation: Keep clear documentation of the trap exit handler including its purpose, expected behavior, and dependencies to facilitate understanding and future modifications.
Conclusion
In summary, the significance of trap EXIT shines brightly in bash scripting. Using the power of this command the user can ensure that every script terminates a well-orchestrated symphony of cleanup, error handling, logging, etc. I hope this article helps you end your script more gracefully.
People Also Ask
What does the trap exit do?
The trap exit command in bash scripting sets up a signal handler to execute specified commands or functions just before a script exits. This ensures that certain actions are taken before script termination, such as cleanup tasks, error handling, or logging. It provides a reliable way to manage resources and ensure script stability.
How does exit work in bash?
In bash, the exit command terminates the script’s execution. When encountered, it immediately stops the script and returns an exit status to the calling environment. This exit status indicates whether the script was completed successfully or encountered an error. The exit command is commonly used to gracefully end script execution and return control to the user or calling process.
What is the difference between trap err and trap exit in Bash?
In bash scripting, the trap err command and trap exit command serve different purposes. The trap err command is used to handle errors or failures within a script. Which allows scripts to take custom responses when errors occur. On the other hand, the trap exit command is specifically designed to execute commands or functions just before the script exits. It happens regardless of whether it finishes successfully or encounters an error. While the trap err focuses on error handling during script execution, the trap exit command is concerned with executing cleanup tasks or finalizing actions before the script terminates.
What is the trap command in bash?
The trap command in bash is a built-in command used to intercept and respond to signals received by a script or shell session. It allows users to specify actions or commands to execute when a particular signal is received. Common signals include interrupts from the keyboard or signals sent by other processes. By using the trap command the users can define custom behavior for their scripts, such as cleanup actions, error handling, or specific responses to signals like termination or suspension, etc.
How do I see if a trap is executed before exit?
To determine if a trap is executed before exit in a bash script, you can include debugging statements or echo commands within the trap function. These statements will be displayed when the trap command is triggered indicating that it has been executed before the script exits. Additionally, you can test the behavior of the trap by intentionally causing the script to exit. Observing the script’s output behavior will confirm whether the trap function is executed before the script terminates.
Related Articles
<< Go Back to Bash Signals Handling | Bash Process and Signal Handling | Bash Scripting Tutorial